1.Error和Exception
1.简单分类:
1.检查性异常:这些异常在编译时不能被简单地忽略。
2.运行时异常:可能被程序员避免的异常。
3.错误Error:错误不是异常,它脱离了程序员的控制,例如当栈溢出了,一个错误就发生了,它在编译时是检测 不到的。
?
2.异常体系结构
java把异常当作对象处理,并定义了一个基类java.lang.Throwable作为所有异常的超类。
Throwable分为Exception和Error
?
3.Error(致命性的)
Error类对象由虚拟机生成并抛出,大多数错误与代码执行者所执行的操作无关。
Java虚拟机运行错误(Virtual MachineError),当JVM不在有继续执行操作所需要的内存资源时,将出现OutOfMemoryError。这些异常发生时,java虚拟机一般会选择线程终止。
还有发生在虚拟机试图执行应用时,如类定义错误(NoClassDefFoundError)、连接错误(LinkageError)。这些错误是不可查的,因为他们在应用程序的控制和处理能力之外,而且绝大多数是程序运行时不允许出现的状况。
?
4.Exception
一个重要子分支RuntimeException:
? ????? 数组下标越界:ArrayIndexOutOfBoundsException
? ????? 空指针异常:NullPointerException
? ????? 算术异常:ArithmeticException
? ????? 丢失资源:MissingResourceException
? ????? 找不到类:ClassNotFoundException
这些异常一般是由于程序逻辑错误引起的,程序应该从逻辑角度尽可能避免这类异常的发生。
? ?
2.捕获和抛出异常
1.try,catch,finally
public class Demo01 {
public static void main(String[] args) {
int a = 1;
int b = 0;
try {
System.out.println(a/b);
}
catch (ArithmeticException e){
System.out.println("出现算术异常,b不能为0");
}
finally {
System.out.println("finally");
}
}
}
?
2.捕获多个异常,顺序要从小到大进行捕获
public class Demo02 {
public static void main(String[] args) {
int a = 1;
int b = 0;
try {
System.out.println(a/b);
}catch (Error e){
System.out.println("Error");
}catch (Exception e1){
System.out.println("Exception");
}catch (Throwable t){
System.out.println("Throwable");
}finally {
System.out.println("finally");
}
}
}
?
3.快捷键
选中当前代码Ctrl+Alt+t,自动生成捕获异常代码
?
4.throw和throws
public class Demo03 {
public static void main(String[] args) {
new Demo03().test(1,0);
}
public void test(int a, int b){
if (b==0){
throw new ArithmeticException();
}
}
}
?
public class Demo03 {
public static void main(String[] args) {
try {
new Demo03().test(1,0);
} catch (ArithmeticException e) {
e.printStackTrace();
}
}
public void test(int a, int b) throws ArithmeticException{
if (b==0){
throw new ArithmeticException();
}
}
}
? ?
3.自定义异常
需要继承Exception
public class MyException extends Exception {
private int detail;
public MyException(int a) {
this.detail = a;
}
@Override
public String toString() {
return "MyException{" + "detail=" + detail + '}';
}
}
public class Test {
static void test(int a) throws MyException {
System.out.println("传递的参数为"+a);
if (a>10) {
throw new MyException(a);
}
System.out.println("ok");
}
public static void main(String[] args) {
try {
test(11);
} catch (MyException e) {
System.out.println("MyException>"+e);
}
}
}
输出结果为:
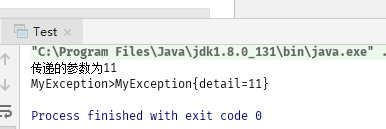
? ?
4.异常总结
1.在多重异常时,可以加一个catch(Exception)来处理可能·会·遗漏的异常。
2.对于不稳定代码,可以加上try-catch,处理潜在异常。
3.尽量去处理异常,切记不要只是调用printStackTrace()去打印输出。
4.尽量添加finally语句去释放占用的资源。IO流。
|