前提
本计算器是基于Swing编写。可以完成加法、减法、乘法、除法和取余运算。且有小数点、退格、清零和求倒数功能。
1.启动类
public class GuiCaculator {
public static void main(String[] args) {
new CaculatorPanel().init();
}
}
2.常量类
public class Const {
public static int Screen_W= Toolkit.getDefaultToolkit().getScreenSize().width;
public static int Screen_Y=Toolkit.getDefaultToolkit().getScreenSize().height;
public static int Frame_W=300;
public static int Frame_Y=300;
public static int frame_x=(Screen_W-Frame_W)/2;
public static int frame_y=(Screen_Y-Frame_Y)/2;
public static String Title="简易计算器";
public static String btnString="+-*/789C456X123R0.%=";
public static String regex="[\\+\\-*%/.R=CX]";
}
3.计算器类
class CaculatorPanel extends JFrame implements ActionListener {
private String operator=null;
private String firstInput=null;
private Double firstNum=0d;
private Double secondNum=0d;
private Double result=0d;
private String wrongNum;
private String rightNum;
private int length;
JTextArea jTextArea = new JTextArea(8, 24);
public void init() {
Container container = this.getContentPane();
JPanel jPanel = new JPanel();
JPanel jPane2 = new JPanel();
jTextArea.setFont(new Font("monospaced", Font.BOLD, 20));
jPanel.add(jTextArea);
jPane2.setLayout(new GridLayout(5,4));
for (int i = 0; i < Const.btnString.length(); i++) {
String btnString=Const.btnString.substring(i, i+1);
JButton jButton = new JButton();
jButton.setText(btnString);
jButton.setFont(new Font("微软雅黑",Font.PLAIN , 16));
if(btnString.matches(Const.regex)){
jButton.setForeground(Color.RED);
}
jButton.addActionListener(this);
jPane2.add(jButton);
}
container.setLayout(new GridLayout(2, 1));
container.add(jPanel);
container.add(jPane2);
setTitle(Const.Title);
setResizable(false);
setSize(Const.Frame_W, Const.Frame_Y);
setLocation(Const.frame_x, Const.frame_y);
setDefaultCloseOperation(WindowConstants.EXIT_ON_CLOSE);
setVisible(true);
}
@Override
public void actionPerformed(ActionEvent e) {
String clickStr=e.getActionCommand();
if(clickStr.equals("C")){
this.jTextArea.setText("");
}
else if("X".equals(clickStr)){
wrongNum=this.jTextArea.getText();
length=wrongNum.length();
rightNum=wrongNum.substring(0, length-1);
System.out.println(rightNum);
this.jTextArea.setText(rightNum);
}
else if(".0123456789".indexOf(clickStr)!=-1){
this.jTextArea.setText(jTextArea.getText()+clickStr);
}
else if(clickStr.matches("[\\+\\-*/%]{1}")){
operator=clickStr;
firstInput=this.jTextArea.getText();
this.jTextArea.setText("");
}
else if(clickStr.equals("=")){
firstNum=Double.valueOf(firstInput);
secondNum=Double.valueOf(this.jTextArea.getText());
switch (operator){
case "+":
result=firstNum+secondNum;
break;
case "-":
result = firstNum - secondNum;
break;
case "*":
result=firstNum*secondNum;
break;
case "/":
if (secondNum!=0)
result=firstNum/secondNum;
break;
case "%":
if (secondNum!=0)
result=firstNum%secondNum;
break;
}
this.jTextArea.setText(result.toString());
}
else if("R".equals(clickStr)&&result!=0) {
result = 1 / result;
this.jTextArea.setText(result.toString());
}
}
}
4、计算器截图
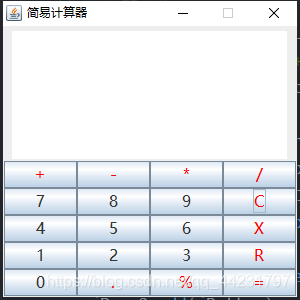
|