一,日期
Date类
Date date1 = new Date();
System.out.println(date1.toString());
Date date2 = new Date(60000);
System.out.println(date2);
运行结果:
Fri Jul 30 23:04:48 CST 2021
Thu Jan 01 08:01:00 CST 1970
Date 类表示系统特定的时间戳,可以精确到毫秒。Date 对象表示时间的默认顺序是星期、月、日、小时、分、秒、年。注:Date(long date):GMT 时间(格林尼治时间)1970 年 1 月 1 日 0 时 0 分 0 秒开始经过参数 date 指定的毫秒数
? ? ? ? ----SimpleDateFormat
????????????????输出的格式并不是我们预期的格式可以使用这个类改变输出格式
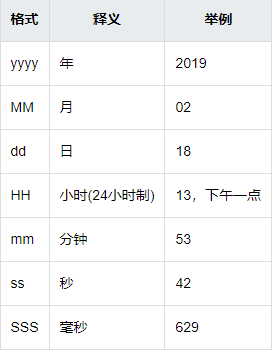
SimpleDateFormat simpleDateFormat1 = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss.SSS");
SimpleDateFormat simpleDateFormat2 = new SimpleDateFormat("yyyy-MM-dd");
SimpleDateFormat simpleDateFormat3 = new SimpleDateFormat("yyyy/MM/dd");
System.out.println(simpleDateFormat1.format(date2)); // 输出2021-07-30 23:04:50.629
System.out.println(simpleDateFormat2.format(date2)); // 输出2021-07-30
System.out.println(simpleDateFormat3.format(date2)); // 输出2021/07/30
?
Calendar类
由于Calendar是一个抽象类所以不能使用new方法创建对象
Calendar calendar = Calendar.getInstance();
Calendar c1 = Calendar.getInstance();
// 获得年份
int year = c1.get(Calendar.YEAR);
// 获得月份
int month = c1.get(Calendar.MONTH) + 1;
// 获得日期
int date = c1.get(Calendar.DATE);
// 获得小时
int hour = c1.get(Calendar.HOUR_OF_DAY);
// 获得分钟
int minute = c1.get(Calendar.MINUTE);
// 获得秒
int second = c1.get(Calendar.SECOND);
// 获得星期几(注意(这个与Date类是不同的):1代表星期日、2代表星期1、3代表星期二,以此类推)
int day = c1.get(Calendar.DAY_OF_WEEK);
jdk8以后官方不推荐使用上述类
新的时间API ?? ?|-- LocalDate ?? ?|-- LocalTime ?? ?|-- LocatDateTime ?? ?|-- DateTimeFormatter ?? ?|-- Instant
LocalDate代表IOS格式(yyyy-MM-dd)的日期:如:2020-06-16
?LocalTime表示一个时间,而不是日期,如:08:05:58.464
?LocalDateTime是用来表示日期和时间的,这是一个最常用的类之一,如:2020-06-16T08:05:58.464 ?? ??? ?
Instant:时间线上的一个瞬时点。 这可以被用来记录应用程序中的事件时间戳。
?Instant表示时间线上的一点,而不需要任何上下文信息,例如,时区。 概念上讲,它只是简单的表示自1970年1月1日0时0分0秒(UTC)开始的秒 数。
LocalDate localDate = LocalDate.now();
System.out.println(localDate);
System.out.println(localDate.getDayOfMonth());
System.out.println(localDate.getDayOfYear());
// 本地时间
LocalTime localTime = LocalTime.now();
System.out.println(localTime);
// 日期+时间的对象
LocalDateTime dateTime = LocalDateTime.now();
System.out.println(dateTime);
// 专门用来格式化日期时间的对象
String str = dateTime.format(DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"));
System.out.println(str);
// 将日期字符串解析为日期对象
LocalDateTime ldt = LocalDateTime.parse("2000年03月08日 14:52:18", DateTimeFormatter.ofPattern("yyyy年MM月dd日 HH:mm:ss"));
System.out.println(ldt);
// 伦敦标准时间,就是经度为0的,0时区时间
Instant instant = Instant.now();
System.out.println(instant);
——————BigDecimal
该类用来对超过16位有效位的数进行精确的运算
// 计算机中的浮点数,并不像整数那样存储,是通过一种算法得到
// 这样的如果我们使用浮点数计算时,可能得到是一个相似值
System.out.println(0.1 + 0.2);
// 高精度行业:航天、经济、银行、金融
// 根据不同行业需求,选择对应的构造函数进行计算
BigDecimal bigDecimal1 = new BigDecimal("0.1");
BigDecimal bigDecimal2 = new BigDecimal("0.2");
BigDecimal add = bigDecimal1.add(bigDecimal2);
System.out.println(add);
System.out.println(bigDecimal1.subtract(bigDecimal2));
System.out.println(bigDecimal1.divide(bigDecimal2));
System.out.println(bigDecimal1.multiply(bigDecimal2));
NumberFormat
NumberFormat帮助您格式化和解析任何区域设置的数字。您的代码可以完全独立于小数点,千位分隔符的区域设置约定,甚至是使用的特定十进制数字,或者数字格式是否为十进制。
double num = 23465.1264566;
// # 主要是在.之后,表示要保留的小数位
DecimalFormat df = new DecimalFormat("0.0000");
String format = df.format(num);
System.out.println(format);
DecimalFormat df2 = new DecimalFormat("0E0");
System.out.println(df2.format(num));
double pi = 3.1415927;
System.out.println(new DecimalFormat("0").format(pi));
System.out.println(new DecimalFormat("0.00").format(pi));
//取两位整数和三位小数,整数不足部分以0填补。
System.out.println(new DecimalFormat("00.000").format(pi));// 03.142
//取所有整数部分
System.out.println(new DecimalFormat("#").format(pi));
//以百分比方式计数,并取两位小数
System.out.println(new DecimalFormat("#.##%").format(pi));
long c = 299792458;
System.out.println(new DecimalFormat("#.#####E0").format(c));// 2.99792E8
System.out.println(new DecimalFormat("00.####E0").format(c));// 29.9792E7
System.out.println(new DecimalFormat(",###").format(c));// 299,792,458
|