package Genericity;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.List;
/*
泛型
*/
public class Test01 {
public static void main(String[] args) {
//不使用泛型时,分析程序存在的缺点
// List list = new ArrayList();
// Cat cat = new Cat();
// Bird bird = new Bird();
// list.add(cat);
// list.add(bird);
// //迭代器
// Iterator iterator = list.iterator();
// while(iterator.hasNext()){
// Object next = iterator.next();
// if (next instanceof Animal) {
// Animal animal = (Animal) next;//不使用泛型 每次迭代出的元素都需要强制转换成Animal才能使用.move方法
// animal.move();
// }
// }
/*
JDK5之后的泛型机制
而使用泛型的好处是:
1.集合中存储的元素类型统一了
2.从集合中取出的元素类型都是泛型指定的类型,不需要每次都进行强转
缺点?
导致集合中的元素缺乏多样性,只能存一种类型。
*/
List<Animal> list = new ArrayList<Animal>();
Cat cat = new Cat();
Bird bird = new Bird();
list.add(cat);
list.add(bird);
Iterator<Animal> iterator = list.iterator();
while (iterator.hasNext()) {
Animal next = iterator.next();
next.move();
//但是如果需要调用子类的特有方法,还是需要强转!
if (next instanceof Cat){
Cat cat1=(Cat)next;
cat1.catchMouse();
}
if (next instanceof Bird){
Bird bird1=(Bird)next;
bird1.fly();
}
}
}
}
class Animal {
public void move() {
System.out.println("动物在移动");
}
}
class Cat extends Animal {
public void catchMouse() {
System.out.println("猫抓老鼠");
}
}
class Bird extends Animal {
public void fly() {
System.out.println("鸟儿在飞翔");
}
}
自定义泛型:
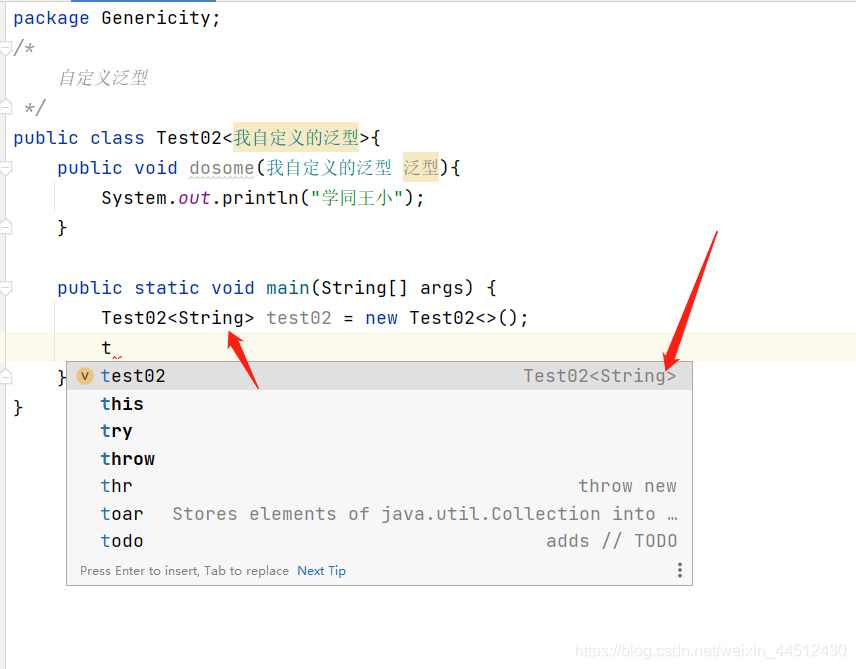
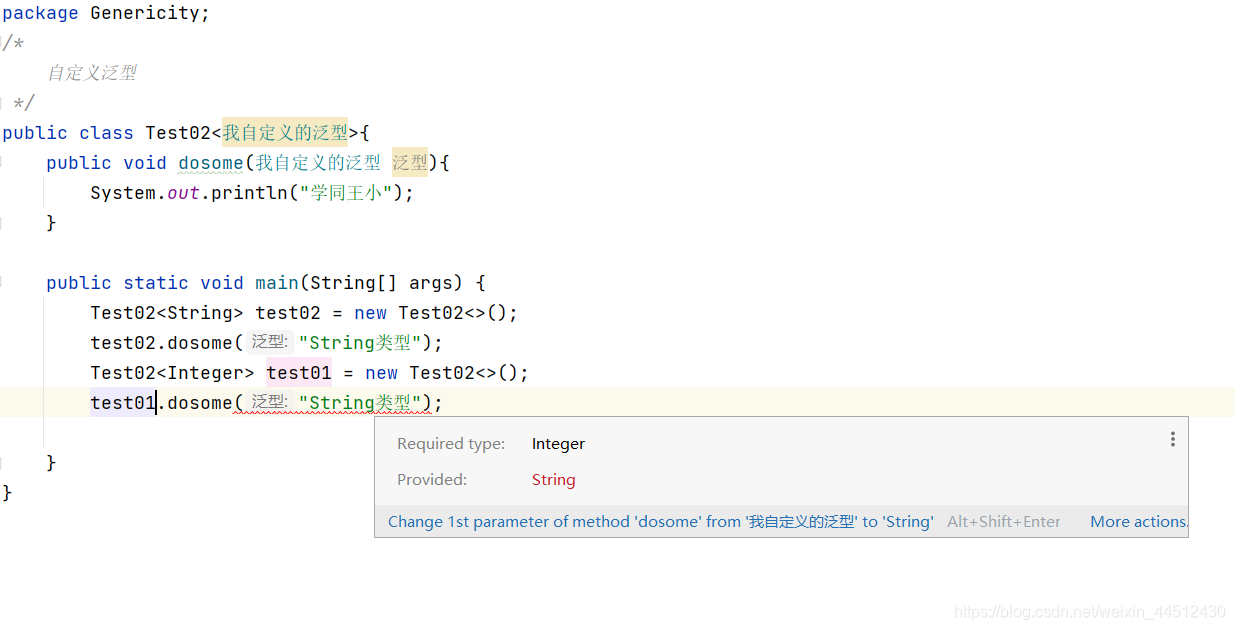
此时的test01在调用dosome方法时只能传入Integer类型
?
?
|