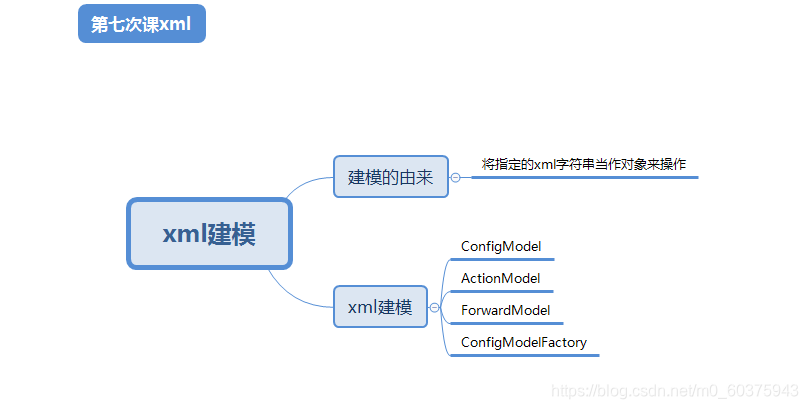
1.什么是XML建模 将XML配置文件中的元素、属性、文本信息转换成对象的过程叫做XML建模
2. XML建模的步骤 建模分两步: 1、以面向对象的编程思想,描述xml资源文件 2、将xml文件中内容封装进model实体对象
ConfigModel
ActionModel
ForwardModel
首先config.xml里面的元素是从里(①forward? ?②action? ?③config)到外取出
<?xml version="1.0" encoding="UTF-8"?> ?? ?<!-- ?? ??? ?config标签:可以包含0~N个action标签 ?? ?--> <config> ?? ?<!-- ?? ??? ?action标签:可以饱含0~N个forward标签 ?? ??? ?path:以/开头的字符串,并且值必须唯一 非空 ?? ??? ?type:字符串,非空 ?? ?--> ?? ?<action path="/regAction" type="test.RegAction"> ?? ??? ?<!-- ?? ??? ??? ?forward标签:没有子标签;? ?? ??? ??? ?name:字符串,同一action标签下的forward标签name值不能相同 ; ?? ??? ??? ?path:以/开头的字符串 ?? ??? ??? ?redirect:只能是false|true,允许空,默认值为false ?? ??? ?--> ?? ??? ?<forward name="failed" path="/reg.jsp" redirect="false" /> ?? ??? ?<forward name="success" path="/login.jsp" redirect="true" /> ?? ?</action>
?? ?<action path="/loginAction" type="test.LoginAction"> ?? ??? ?<forward name="failed" path="/login.jsp" redirect="false" /> ?? ??? ?<forward name="success" path="/main.jsp" redirect="true" /> ?? ?</action> </config>
? ?A.config节点下有多个子action节点,无节点属性 ? ?B.action节点下有多个子forward节点,有节点属性 ? ?C.forward下无子节点,有节点属性
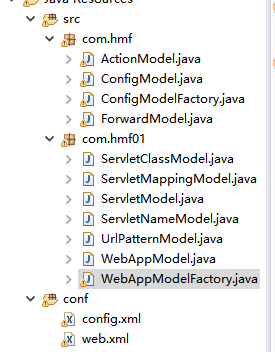
?
ForwardModel
package com.hmf;
import java.io.Serializable;
/** ?* config点xmlforwar节点对应的建模实体类ForwardModel ?* @author T440s ?* ?*/ public class ForwardModel implements Serializable{ ?? ?//forwar节点对应的name属性 ?? ?private String name; ?? ?//forwar节点对应的path属性 ?? ?private String path; ?? ?//forwar节点对应的redirect属性 ?? ?private boolean redirect; ?? ? ?? ?public String getName() { ?? ??? ?return name; ?? ?} ?? ?public void setName(String name) { ?? ??? ?this.name = name; ?? ?} ?? ?public String getPath() { ?? ??? ?return path; ?? ?} ?? ?public void setPath(String path) { ?? ??? ?this.path = path; ?? ?} ?? ?public boolean isRedirect() { ?? ??? ?return redirect; ?? ?} ?? ?public void setRedirect(boolean redirect) { ?? ??? ?this.redirect = redirect; ?? ?} } ?
ActionModel
package com.hmf;
import java.io.Serializable; import java.util.HashMap; import java.util.Map;
/** ?* 将xml放入到action实体类 ?* @author T440s ?* ?*/ public class ActionModel implements Serializable{ ?? ?private String path; ?? ?private String type; ?? ? ?? ?//在同一个action下的name? ?? ?//key 代表forward节点的name属性,唯一 ?? ?//value,将整个forward节点所对应的建模实体类ForwardModel作为value的值 ?? ?private Map<String, ForwardModel> forwards=new HashMap<String, ForwardModel>(); ?? ? ?? ?//压栈 ?? ?public void push(ForwardModel ForwardModel1) { ?? ??? ?forwards.put(ForwardModel1.getName(),ForwardModel1); ?? ?} ?? ? ?? ?//弹栈 ?? ?public ForwardModel get(String name) { ?? ??? ?return forwards.get(name); ?? ?} ?? ? ?? ?public String getPath() { ?? ??? ?return path; ?? ?} ?? ?public void setPath(String path) { ?? ??? ?this.path = path; ?? ?} ?? ?public String getType() { ?? ??? ?return type; ?? ?} ?? ?public void setType(String type) { ?? ??? ?this.type = type; ?? ?} ?? ?@Override ?? ?public String toString() { ?? ??? ?return "ActionModel [path=" + path + ", type=" + type + "]"; ?? ?} ?? ? ?? ?public ActionModel(String path, String type) { ?? ??? ?super(); ?? ??? ?this.path = path; ?? ??? ?this.type = type; ?? ?} ?? ?public ActionModel() { ?? ??? ?// TODO Auto-generated constructor stub ?? ?} } ?
ConfigModel
package com.hmf;
import java.io.Serializable; import java.util.HashMap; import java.util.Map;
public class ConfigModel implements Serializable{ ?? ? ?? ?private Map<String, ActionModel> actions=new HashMap<String, ActionModel>(); ?? ? ?? ?public Map<String, ActionModel> getAction(){ ?? ??? ?return actions; ?? ?} ?? ? ?? ?public void setAction (Map<String, ActionModel> actions){ ?? ??? ?this.actions=actions; ?? ?} ?? ? ?? ?@Override ?? ?public String toString() { ?? ??? ?return "ConfigModel [actions=" + actions + "]"; ?? ?} ?? ? ?? ?public ConfigModel() { ?? ??? ?// TODO Auto-generated constructor stub ?? ?}
?? ?public ConfigModel(Map<String, ActionModel> actions) { ?? ??? ?super(); ?? ??? ?this.actions = actions; ?? ?} ?? ? ?? ?//压栈 ?? ?public void push(ActionModel actionModel) { ?? ??? ?actions.put(actionModel.getPath(),actionModel); ?? ?} ?? ?//弹栈 ?? ?public ActionModel get(String path) { ?? ??? ?return actions.get(path);
? ? }
????public static void main(String[] args) { ?? ??? ?????????//ConfigModel对象里没有xml内容 ?? ??? ?????????ConfigModel configModel=new ConfigModel(); ????????????? } ?? ?}
ConfigModelFactory
package com.hmf;
import java.io.InputStream; import java.util.List;
import org.dom4j.Document; import org.dom4j.DocumentException; import org.dom4j.Element; import org.dom4j.io.SAXReader;
/** ?* 建模思路: ?* 1.将原有的config.xml进行解析 ?* 2.对应标签内容,将其封装赋值给相应的对象 ?* ?forward标签值 赋值给forwardModel对象 ?* ?action标签值 赋值给ActionModel对象 ?* ?config标签值 赋值给ConModel对象 ?* @author T440s ?* ?*/ public class ConfigModelFactory { ?? ?public static ConfigModel build() throws DocumentException { ?? ??? ?return build("config.xml"); ?? ?} ?? ? ?? ?public static ConfigModel build(String resourcepath) throws DocumentException { ?? ??? ?InputStream in = ConfigModelFactory.class.getResourceAsStream(resourcepath); ?? ??? ?SAXReader sax=new SAXReader(); ?? ??? ?Document doc = sax.read(in); ?? ??? ?ConfigModel config=new ConfigModel(); ?? ??? ?List<Element> acList = doc.selectNodes("/config/action"); ?? ??? ?for (Element actEle : acList) { ?? ??? ??? ?ActionModel actionModel=new ActionModel(); ?? ??? ??? ?//将xml文件解析的来的path值赋值给actionModel对象中的path属性 ?? ??? ??? ?actionModel.setPath(actEle.attributeValue("path")); ?? ??? ??? ?actionModel.setType(actEle.attributeValue("type")); ?? ??? ??? ?List<Element> forwardEles = actEle.selectNodes("forwardEles"); ?? ??? ??? ?for(Element forwardEle : forwardEles) { ?? ??? ??? ??? ?ForwardModel forwardModel=new ForwardModel(); ?? ??? ??? ??? ?forwardModel.setName(forwardEle.attributeValue("name")); ?? ??? ??? ??? ?forwardModel.setPath(forwardEle.attributeValue("path")); ?? ??? ??? ??? ?//redirect只有在配置文件赋值false的时候 是代表(转发) ?? ??? ??? ??? ?//其他都是代表重定向 ?? ??? ??? ??? ?forwardModel.setRedirect(!"false".equals(forwardEle.attributeValue("redirect"))); ?? ??? ??? ??? ?config.push(actionModel); ?? ??? ??? ?} ?? ??? ??? ?config.push(actionModel); ?? ??? ?} ?? ??? ?return config; ?? ?} } ?
例如:
创建一个servlet进行建模
通过url-pattern读取到servlet-class的值
<?xml version="1.0" encoding="UTF-8"?> <web-app> ? <servlet> ? ?? ?<servlet-name>jrebelServlet</servlet-name> ? ?? ?<servlet-class>com.zking.xml.JrebelServlet</servlet-class> ? </servlet> ?? ? <servlet-mapping> ? ?? ?<servlet-name>jrebelServlet</servlet-name> ? ?? ?<url-pattern>/jrebelServlet</url-pattern> ? </servlet-mapping> ?? ? <servlet> ? ?? ?<servlet-name>jrebelServlet2</servlet-name> ? ?? ?<servlet-class>com.zking.xml.JrebelServlet2</servlet-class> ? </servlet> ?? ? <servlet-mapping> ? ?? ?<servlet-name>jrebelServlet2</servlet-name> ? ?? ?<url-pattern>/jrebelServlet2</url-pattern> ? ?? ?<url-pattern>/jrebelServlet3</url-pattern> ? </servlet-mapping> </web-app>
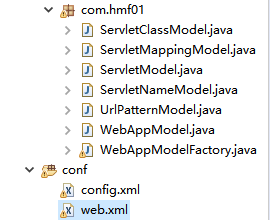
package com.hmf01;
import java.io.InputStream; import java.util.List;
import org.dom4j.Document; import org.dom4j.DocumentException; import org.dom4j.Element; import org.dom4j.Node; import org.dom4j.io.SAXReader;
public class WebAppModelFactory { ?? ?public static WebAppModel buildWebAppModel() throws Exception{ ?? ??? ?return buildWebAppModel("/web.xml"); ?? ?}
?? ? ?? ?public static WebAppModel buildWebAppModel(String xmlPath) { ?? ??? ?InputStream in = WebAppModelFactory.class.getResourceAsStream(xmlPath); ?? ??? ?SAXReader saxReader = new SAXReader(); ?? ??? ?WebAppModel webAppModel = new WebAppModel(); ?? ??? ?try { ?? ??? ??? ?Document doc = saxReader.read(in); ?? ??? ??? ? ?? ??? ??? ?List<Element> servletEles = doc.selectNodes("/web-app/servlet"); ?? ??? ??? ?for (Element servletEle : servletEles) { ?? ??? ??? ??? ?ServletModel servletModel = new ServletModel();
?? ??? ??? ??? ? ?? ??? ??? ??? ?Element servletNameEle = (Element) servletEle.selectSingleNode("servlet-name"); ?? ??? ??? ??? ?Element servletClassEle = (Element) servletEle.selectSingleNode("servlet-class"); ?? ??? ??? ??? ?ServletNameModel servletNameModel = new ServletNameModel(); ?? ??? ??? ??? ?ServletClassModel servletClassModel = new ServletClassModel(); ?? ??? ??? ??? ?servletNameModel.setContext(servletNameEle.getText()); ?? ??? ??? ??? ?servletClassModel.setContext(servletClassEle.getText()); ?? ??? ??? ??? ?servletModel.setServletNameModel(servletNameModel); ?? ??? ??? ??? ?servletModel.setServletClassModel(servletClassModel);
?? ??? ??? ??? ?webAppModel.pushServletModel(servletModel); ?? ??? ??? ?}
?? ??? ??? ? ?? ??? ??? ?List<Element> servletMappingEles = doc.selectNodes("/web-app/servlet-mapping"); ?? ??? ??? ?for (Element servletMappingEle : servletMappingEles) { ?? ??? ??? ??? ?ServletMappingModel servletMappingModel = new ServletMappingModel();
?? ??? ??? ??? ? ?? ??? ??? ??? ?Element servletNameEle = (Element) servletMappingEle.selectSingleNode("servlet-name"); ?? ??? ??? ??? ?ServletNameModel servletNameModel = new ServletNameModel(); ?? ??? ??? ??? ?servletNameModel.setContext(servletNameEle.getText()); ?? ??? ??? ??? ?servletMappingModel.setServletNameModel(servletNameModel); ?? ??? ??? ??? ? ?? ??? ??? ??? ?List<Element> urlPatternEles = servletMappingEle.selectNodes("url-pattern"); ?? ??? ??? ??? ?for (Element urlPatternEle : urlPatternEles) { ?? ??? ??? ??? ??? ?UrlPatternModel urlPatternModel = new UrlPatternModel(); ?? ??? ??? ??? ??? ?urlPatternModel.setContext(urlPatternEle.getText()); ?? ??? ??? ??? ??? ?servletMappingModel.pushUrlPatternModel(urlPatternModel); ?? ??? ??? ??? ?}
?? ??? ??? ??? ?webAppModel.pushServletMappingModel(servletMappingModel); ?? ??? ??? ?} ?? ??? ?} catch (Exception e) { ?? ??? ??? ?// TODO Auto-generated catch block ?? ??? ??? ?e.printStackTrace(); ?? ??? ?} ?? ??? ?return webAppModel; ?? ?} ?? ? ?? ? ?? ?public static String getServletClassByUrl(WebAppModel webAppModel, String url) { ?? ??? ?String servletClass = ""; ?? ??? ?String servletName = ""; ?? ??? ?List<ServletMappingModel> servletMappingModels = webAppModel.getServletMappingModels(); ?? ??? ?for (ServletMappingModel servletMappingModel : servletMappingModels) { ?? ??? ??? ?List<UrlPatternModel> urlPatternModels = servletMappingModel.getUrlPatternModels(); ?? ??? ??? ?for (UrlPatternModel urlPatternModel : urlPatternModels) { ?? ??? ??? ??? ?if(url.equals(urlPatternModel.getContext())) { ?? ??? ??? ??? ??? ?ServletNameModel servletNameModel = servletMappingModel.getServletNameModel(); ?? ??? ??? ??? ??? ?servletName = servletNameModel.getContext(); ?? ??? ??? ??? ?} ?? ??? ??? ?} ?? ??? ?} ?? ??? ? ?? ??? ? ?? ??? ?List<ServletModel> servletModels = webAppModel.getServletModels(); ?? ??? ?for (ServletModel servletModel : servletModels) { ?? ??? ??? ?ServletNameModel servletNameModel = servletModel.getServletNameModel(); ?? ??? ??? ?if(servletName.equals(servletNameModel.getContext())) { ?? ??? ??? ??? ?ServletClassModel servletClassModel = servletModel.getServletClassModel(); ?? ??? ??? ??? ?servletClass = servletClassModel.getContext(); ?? ??? ??? ?} ?? ??? ?} ?? ??? ?return servletClass; ?? ?} ?? ? ?? ?public static void main(String[] args) throws Exception { ?? ??? ?WebAppModel webAppModel = WebAppModelFactory.buildWebAppModel(); ?? ??? ?String res = getServletClassByUrl(webAppModel, "/jrebelServlet"); ?? ??? ?String res2 = getServletClassByUrl(webAppModel, "/jrebelServlet2"); ?? ??? ?String res3 = getServletClassByUrl(webAppModel, "/jrebelServlet3"); ?? ??? ?System.out.println(res); ?? ??? ?System.out.println(res2); ?? ??? ?System.out.println(res3); ?? ?} } ?
好了今天到这里了?
|