需求:有一堆英语考试真题(PDF格式),读取PDF,然后分析每个单词出现的词频,输出到一个txt文本中,每行是“单词 词频”,结果按照词频降序排列。
思路:使用一个LinkedHashMap,Key是单词,Value是词频,逐个遍历每个pdf文件,读取pdf中的所有文本,对于文本按照各种标点符号进行分割,将全部单词转为小写,遍历每个单词,看在map中是否存在,存在则词频+1,不存在则put增加新的单词。
代码如下:
import com.yzk18.commons.IOHelpers;
import com.yzk18.docs.PDFHelpers;
import org.apache.pdfbox.pdmodel.PDDocument;
import java.util.*;
public class WordFrequency {
public static void main(String[] args) {
String dir = "E:\\英语\\六级英语\\2020年7月";
String[] pdfFiles = IOHelpers.getFilesRecursively(dir,"pdf");
LinkedHashMap<String,Integer> map = new LinkedHashMap<>();
for (String pdfFile : pdfFiles)
{
PDDocument doc = PDFHelpers.openFile(pdfFile);
String text = PDFHelpers.parseText(doc);
String[] words = text.toLowerCase().split("\\s|\\.|\\,|\\:|\\!|\\?|;|\\(|\\)");
for(String word : words)
{
if(!isEnglishWord(word))
{
continue;
}
if(word.equals(""))
{
continue;
}
Integer freq = map.get(word);
if(freq==null)
{
map.put(word,1);
}
else
{
map.put(word,freq+1);
}
}
PDFHelpers.close(doc);
}
String outputString="";
List<Map.Entry<String, Integer>> infoIds =new ArrayList<Map.Entry<String, Integer>>(map.entrySet());
Collections.sort(infoIds, new Comparator<Map.Entry<String, Integer>>() {
@Override
public int compare(Map.Entry<String, Integer> o1, Map.Entry<String, Integer> o2) {
Integer p1 = o1.getValue();
Integer p2 = o2.getValue();;
return Integer.valueOf(p2)-Integer.valueOf(p1);
}
});
LinkedHashMap<String, Integer> newMap = new LinkedHashMap <String, Integer>();
for(Map.Entry<String,Integer> entity : infoIds){
newMap.put(entity.getKey(), entity.getValue());
}
for(String word : newMap.keySet())
{
int freq = map.get(word);
outputString = outputString+word+" "+freq+"\r\n";
}
IOHelpers.writeAllText("d:/1.txt", outputString);
}
public static boolean isEnglishWord(String s)
{
for(int i=0;i<s.length();i++)
{
char ch = s.charAt(i);
if(Character.isLowerCase(ch)==false)
{
return false;
}
}
return true;
}
}
输出结果如下: 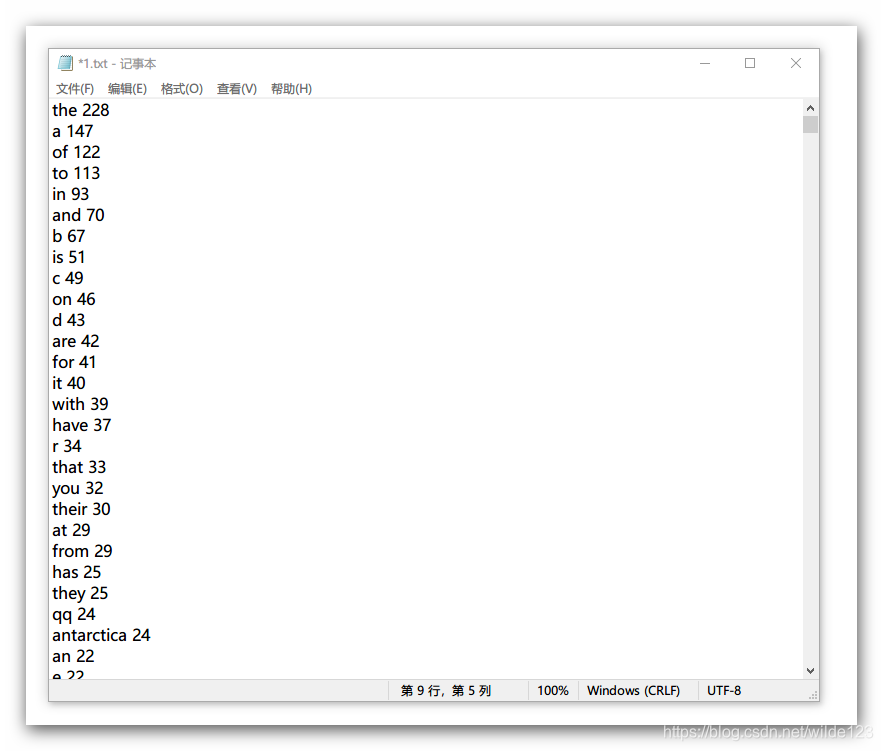 这样,就可以自己网上下载四六级真题,通过词频背单词啦,( ̄▽ ̄)~*。
|