今天大佬给我Spring配置,然后让我实现订单在未付款状态,N秒后自动失效 ↓
一、大佬给的配置
spring:
activemq:
inMemory: false
broker-url: tcp://47.108.204.1:61616
user: admin
password: admin
二、添加依赖和俩文件
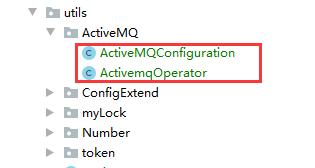
ActiveMQ依赖
<!--activeMq-->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-activemq</artifactId>
</dependency>
第一个配置文件------ActiveMQConfiguration
package com.nxt.order.utils.ActiveMQ;
import org.apache.activemq.ActiveMQConnection;
import org.apache.activemq.ActiveMQConnectionFactory;
import org.apache.activemq.RedeliveryPolicy;
import org.springframework.beans.factory.annotation.Qualifier;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import org.springframework.context.annotation.Primary;
import org.springframework.jms.config.JmsListenerContainerFactory;
import org.springframework.jms.config.SimpleJmsListenerContainerFactory;
@Configuration
public class ActiveMQConfiguration {
@Bean(name = "innerConnectionFactory")
@Primary
public ActiveMQConnectionFactory firstConnectionFactory(
@Value("${spring.activemq.broker-url}") String brokerUrl,
@Value("${spring.activemq.user}") String username,
@Value("${spring.activemq.password}") String password) {
ActiveMQConnectionFactory factory = new ActiveMQConnectionFactory();
factory.setBrokerURL(brokerUrl);
factory.setUserName(username);
factory.setPassword(password);
factory.setTrustAllPackages(true);
factory.setMaxThreadPoolSize(ActiveMQConnection.DEFAULT_THREAD_POOL_SIZE);
RedeliveryPolicy redeliveryPolicy = new RedeliveryPolicy();
redeliveryPolicy.setUseExponentialBackOff(true);
redeliveryPolicy.setMaximumRedeliveries(1);
redeliveryPolicy.setInitialRedeliveryDelay(1000);
redeliveryPolicy.setBackOffMultiplier(2);
redeliveryPolicy.setMaximumRedeliveryDelay(1000);
factory.setRedeliveryPolicy(redeliveryPolicy);
return factory;
}
@Bean("topicJmsListenerContainerFactory")
public JmsListenerContainerFactory<?> topicJmsListenerContainerFactory(
@Qualifier("innerConnectionFactory") ActiveMQConnectionFactory connectionFactory) {
SimpleJmsListenerContainerFactory factory = new SimpleJmsListenerContainerFactory();
factory.setConnectionFactory(connectionFactory);
factory.setSessionTransacted(true);
factory.setPubSubDomain(true);
return factory;
}
@Bean("queueJmsListenerContainerFactory")
public JmsListenerContainerFactory<?> queueJmsListenerContainerFactory(
@Qualifier("innerConnectionFactory") ActiveMQConnectionFactory connectionFactory) {
SimpleJmsListenerContainerFactory factory = new SimpleJmsListenerContainerFactory();
factory.setConnectionFactory(connectionFactory);
factory.setSessionTransacted(true);
factory.setPubSubDomain(false);
return factory;
}
}
第二个配置文件------ActivemqOperator
package com.nxt.order.utils.ActiveMQ;
import org.apache.activemq.ScheduledMessage;
import org.apache.activemq.command.ActiveMQQueue;
import org.apache.activemq.command.ActiveMQTopic;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.autoconfigure.jms.JmsProperties;
import org.springframework.jms.core.JmsMessagingTemplate;
import org.springframework.stereotype.Component;
import javax.jms.*;
@Component
public class ActivemqOperator {
private final JmsMessagingTemplate jmsTemplate;
@Autowired
public ActivemqOperator(JmsMessagingTemplate jmsTemplate) {
this.jmsTemplate = jmsTemplate;
}
public void sendMsgTopic(String topic, String message) {
Destination destination = new ActiveMQTopic(topic);
jmsTemplate.convertAndSend(destination, message);
}
public void sendMsgQueue(String queue, String message) {
Destination destination = new ActiveMQQueue(queue);
jmsTemplate.convertAndSend(destination, message);
}
public <T> void delaySend(String queue, String message, Long time) {
Connection connection = null;
Session session = null;
MessageProducer producer = null;
ConnectionFactory connectionFactory = jmsTemplate.getConnectionFactory();
Destination destination = new ActiveMQQueue(queue);
try {
connection = connectionFactory.createConnection();
connection.start();
session = connection.createSession(Boolean.TRUE, Session.AUTO_ACKNOWLEDGE);
producer = session.createProducer(destination);
producer.setDeliveryMode(JmsProperties.DeliveryMode.PERSISTENT.getValue());
ObjectMessage objectMessage = session.createObjectMessage(message);
objectMessage.setLongProperty(ScheduledMessage.AMQ_SCHEDULED_DELAY, time);
producer.send(objectMessage);
session.commit();
} catch (Exception e) {
throw new RuntimeException("delaySend exception : " + e.getMessage());
} finally {
try {
if (producer != null) {
producer.close();
}
if (session != null) {
session.close();
}
if (connection != null) {
connection.close();
}
} catch (Exception e) {
e.printStackTrace();
}
}
}
}
三、演示效果
package com.nxt.order.controller;
import com.nxt.order.utils.ActiveMQ.ActivemqOperator;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.jms.annotation.JmsListener;
import org.springframework.web.bind.annotation.GetMapping;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
import java.text.SimpleDateFormat;
import java.util.Date;
@RequestMapping("/testMq")
@RestController
public class Testaaaaa {
@Autowired
ActivemqOperator activemqOperator;
@GetMapping("/queue")
public String testMqQueue() {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
System.out.println("当前订单【123456789】生成时间: "+df.format(new Date()));
activemqOperator.delaySend("testQueue","延时10S",10000L);
return "OK";
}
@JmsListener(destination = "testQueue", containerFactory = "queueJmsListenerContainerFactory")
public void listenerMq(String message) {
SimpleDateFormat df = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss");
System.out.println("当前订单【123456789】失效时间: " + df.format(new Date()));
}
}
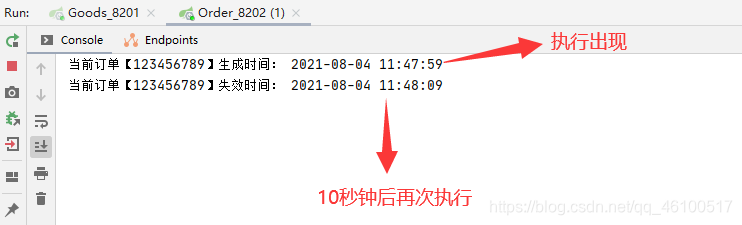 如果我们把生成时间改成订单创建,失效时间改成订单结束时间到了 订单失效,那么此功能就算实现了
|