环境
springboot2.4.3?+ thymeleaf2.4.3?+ bootstrap3
需求
浏览器中访问:http://localhost:8080/employee/list 列表员工数据
操作步骤
步骤1:创建项目 springboot-thymeleaf
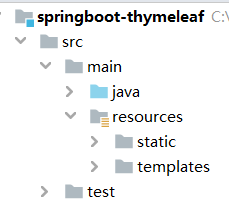
?
步骤2:在/项目/resourcess/static 文件中导入bootstrap3相关文件
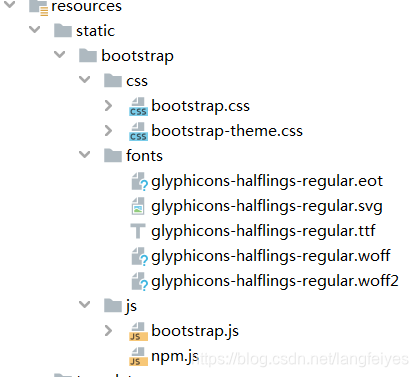
步骤3:导入依赖
核心依赖有3个:springboot parent? + web环境 + thymeleaf 依赖
在pom.xml文件中导入
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.3</version>
<relativePath/>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
步骤4:在/项目/resources/application.properties配置thymeleaf
#开启thymeleaf视图解析
spring.thymeleaf.enabled=true
#编码
spring.thymeleaf.encoding=utf-8
#前缀
spring.thymeleaf.prefix=classpath:/templates/
#是否使用缓存
spring.thymeleaf.cache=false
#严格的HTML语法模式
spring.thymeleaf.mode=HTML
#后缀名
spring.thymeleaf.suffix=.html
步骤5:编写相关相关代码
代码结构:
实体类:Employee
package com.langfeiyes.thymeleaf.entities;
/**
* 员工对象
*/
public class Employee {
private Long id;
private String name;
private int age;
public Employee() {
}
public Employee(Long id, String name, int age) {
this.id = id;
this.name = name;
this.age = age;
}
public Long getId() {
return id;
}
public void setId(Long id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
}
接口类:EmployeeController
package com.langfeiyes.thymeleaf.controller;
import com.langfeiyes.thymeleaf.entities.Employee;
import org.springframework.stereotype.Controller;
import org.springframework.ui.Model;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.ArrayList;
import java.util.List;
@Controller
@RequestMapping("employee")
public class EmployeeController {
//假装是从数据库中查询出来的
private List<Employee> queryList(){
List<Employee> list = new ArrayList<>();
list.add(new Employee(1L, "zhangsan", 16));
list.add(new Employee(2L, "lisi", 17));
list.add(new Employee(3L, "wangwu", 18));
list.add(new Employee(4L, "zhaoliu", 19));
list.add(new Employee(5L, "qianqi", 20));
return list;
}
/**
* 员工列表
* @return
*/
@RequestMapping("/list")
public String list(Model model){
model.addAttribute("emps", queryList());
return "employee/list"; //返回员工模板
}
}
启动类:App
package com.langfeiyes.thymeleaf;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class App {
public static void main(String[] args) {
SpringApplication.run(App.class, args);
}
}
步骤6:编写员工列表模板
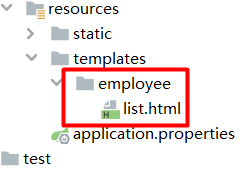
代码结构:
<html lang="en" xmlns:th="http://www.w3.org/1999/xhtml">
<head>
<title>员工列表</title>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<link rel="stylesheet" href="/bootstrap/css/bootstrap.css" type="text/css" />
<script type="text/javascript" src="/bootstrap/js/bootstrap.js"></script>
</head>
<body>
<div class="container-fluid " style="margin-top: 20px">
<div class="row">
<div class="col-sm-10">
<table class="table table-striped table-hover" >
<thead>
<tr>
<th>序号</th>
<th>员工名称</th>
<th>员工年龄</th>
<th>操作</th>
</tr>
</thead>
<tr th:each="e,eStat:${emps}">
<td th:text="${eStat.index+1}"></td>
<td th:text="${e.name}"></td>
<td th:text="${e.age}"></td>
<td>
<a class="btn btn-info btn-xs " href="javascript:;" >
<span class="glyphicon glyphicon-edit"></span> 编辑
</a>
<a href="javascript:;" class="btn btn-danger btn-xs ">
<span class="glyphicon glyphicon-trash"></span> 删除
</a>
</td>
</tr>
</table>
</div>
</div>
</div>
</body>
</html>
?
步骤7:测试
右键执行App类
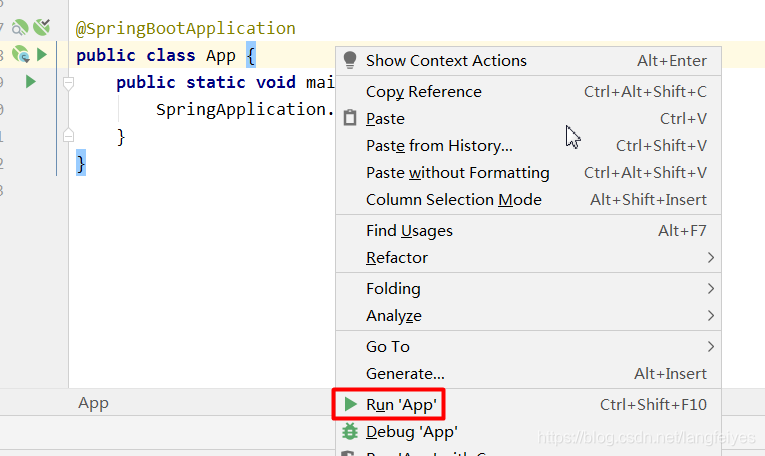
浏览器输入:http://localhost:8080/employee/list
显示最终效果:
?到这,Springboot集成thymeleaf成功。
案例源码:传送门
|