一元运算符
int b = a++;
int c = ++a;
幂运算需要使用工具类
Math.pow(3,2); //9
逻辑与会造成短路运算
public class Text05 {
public static void main(String[] args) {
boolean a = true;
boolean b = false;
System.out.println("a && b: "+ (a && b));
System.out.println("a || b: "+ (a || b));
System.out.println("!(a && b): "+ !(a && b));
int c = 5;
boolean d = (c<4) && (c++<4);
System.out.println(d);
System.out.println(c);
}
}
位运算是对二进制位的运算
A&B 与
A|B 或
A^B 异或(相同为0,不同为1)
~B 非
最快速度运算2*8(位运算,效率高)
0000 0000 0
0000 0001 1
0000 0010 2
0000 0100 4
0000 1000 8
<< 左移 (*2) >>右移(/2)
a+=b; //a=a+b
a-=b; //a=a-b
public class Text06 {
public static void main(String[] args) {
int a = 10;
int b = 20;
System.out.println(a+b);
System.out.println(""+a+b);
System.out.println(a+b+"");
}
}
三元运算符 条件运算符
x ?y :z //如果x==ture,则结果为y,否则结果为z
public class Text07 {
public static void main(String[] args) {
int score = 80;
String type = score < 60 ? "不及格" : "及格";
System.out.println(type);
}
}
优先级
Java 语言中运算符的优先级共分为 14 级,其中 1 级最高,14 级最低。在同一个表达式中运算符优先级高的先执行。
优先级 | 运算符 | 结合性 |
---|
1 | ()、[]、{} | 从左向右 | 2 | !、+、-、~、++、– | 从右向左 | 3 | *、/、% | 从左向右 | 4 | +、- | 从左向右 | 5 | ?、?、>>> | 从左向右 | 6 | <、<=、>、>=、instanceof | 从左向右 | 7 | ==、!= | 从左向右 | 8 | & | 从左向右 | 9 | ^ | 从左向右 | 10 | | | 从左向右 | 11 | && | 从左向右 | 12 | || | 从左向右 | 13 | ?: | 从右向左 | 14 | =、+=、-=、*=、/=、&=、|=、^=、~=、?=、?=、>>>= | 从右向左 |
包机制
包的本质就是一个文件夹
一般使用公司域名倒置作为包名:com.baidu.www
为了使用某一个包的成员,我们需要再Java程序中明确导入该包。使用“import”语句可以完成此功能。
import package1.package2.classname;
import package1.package2.*; //导入这个包下所有的类
JavaDoc(/**… */)
加在类上面就是类的注释,加在方法上面就是方法的注释
@author
@version 版本号
@since 指明需要最早使用的jdk版本
@param 参数名
@return 返回值情况
@throws 异常抛出情况
打开cmd,进入该类所在的目录,然后输入
javadoc -encoding UTF-8 -charset UTF-8 Text08.java
- -encoding UTF-8:表示设置编码。
- -charset UTF-8:也表示设置编码。
IDEA
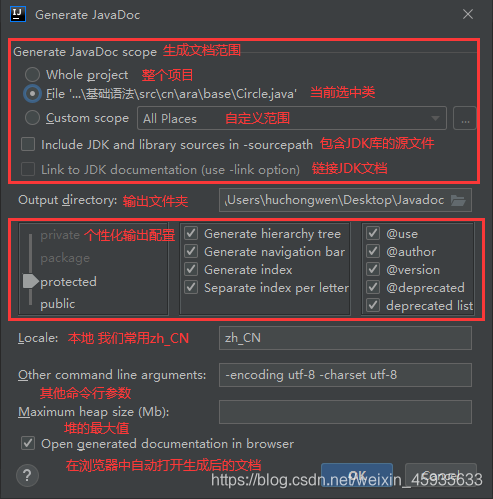
Java流程控制
Scanner对象
实现程序和人的交互,java.util.Scanner是Java5的新特征,可以通过Scanner类来获取用户的输入。
Scanner s = new Scanner(Syttem.in);
通过Scanner类的next()与nextLine()方法获取输入的字符串
String str = scanner.nextLine();
读取前,需要使用hasNext()与hasNextLine()判断是否还有输入数据。
凡是属于IO流的类如果不关闭会一直占用资源 即输入输出流
scanner.close();
package com.wang.operator;
import java.util.Scanner;
public class Demo01 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("使用next方式接收:");
if(scanner.hasNext()){
String str = scanner.next();
System.out.println("输入的内容为:"+str);
}
scanner.close();
}
}
next()读取到空格结束;
nextLIne()读取到enter结束;
|