首先在yml里面配置数据
spring:
datasource:
driver-class-name: com.mysql.cj.jdbc.Driver
username: root
password: 123456
url: jdbc:mysql://192.168.56.101:3306/orderfood?useSSL=false&useUnicode=true&characterEncoding=UTF8&serverTimezone=GMT
jpa:
show-sql: true
一般来说是一张表一个接口,正常情况下 一张表对应一个接口 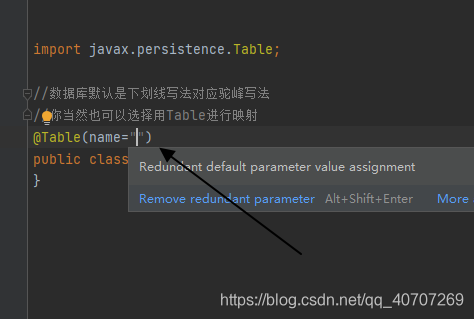 里面写数据库名字可以完成映射
主键和自增映射
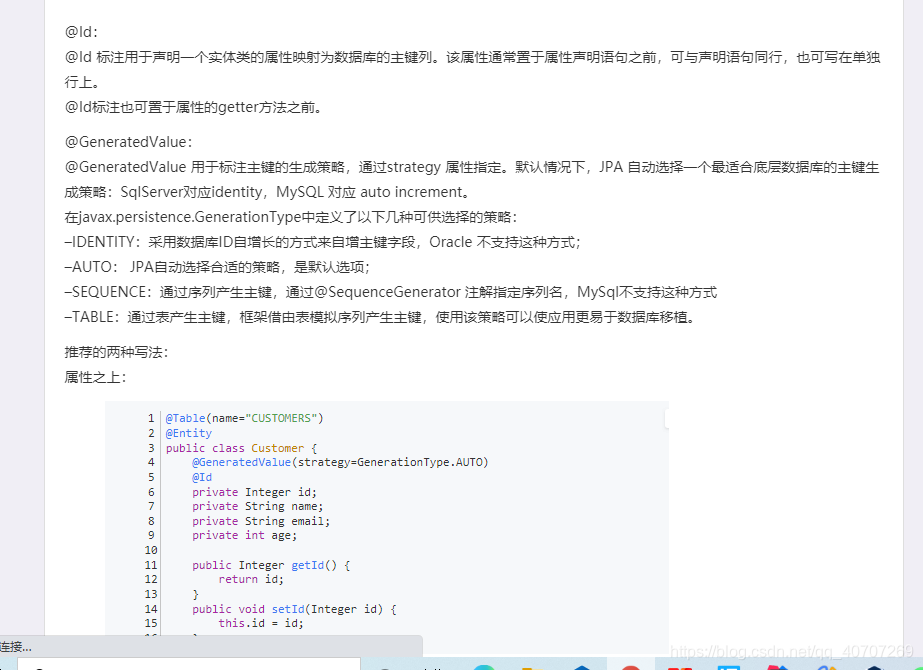 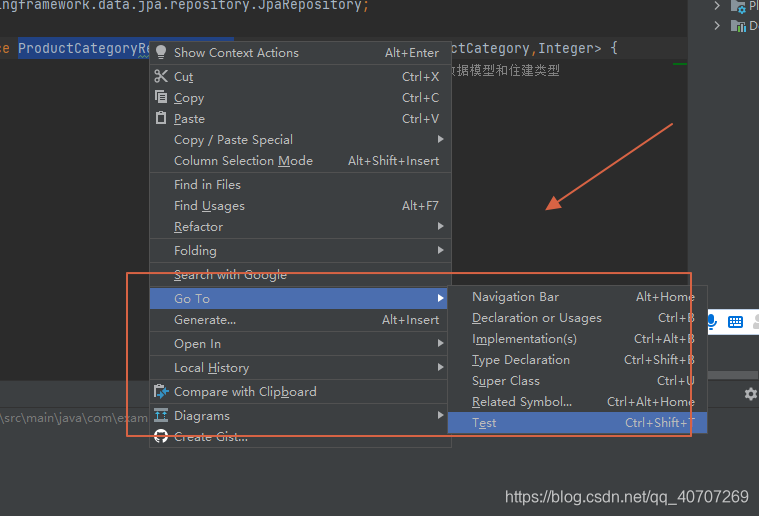 写完接口之后直接去测试
url连接最好加上
jdbc:mysql://localhost:3306/test?serverTimezone=GMT&allowPublicKeyRetrieval=true&useSSL=false&characterEncoding=utf8;
规则就是先写一个一张表写一个模型 一张表要写一个模型
package com.example.wetchatorder.dateObject;
import javax.persistence.Entity;
import javax.persistence.GeneratedValue;
import javax.persistence.Id;
import javax.persistence.Table;
@Entity
public class ProductCategory {
@Id
@GeneratedValue
private Integer categoryId;
private String categoryName;
private Integer categoryType;
public Integer getCategoryId() {
return categoryId;
}
public void setCategoryId(Integer categoryId) {
this.categoryId = categoryId;
}
public String getCategoryName() {
return categoryName;
}
public void setCategoryName(String categoryName) {
this.categoryName = categoryName;
}
public Integer getCategoryType() {
return categoryType;
}
public void setCategoryType(Integer categoryType) {
this.categoryType = categoryType;
}
@Override
public String toString() {
return "ProductCategory{" +
"categoryId=" + categoryId +
", categoryName='" + categoryName + '\'' +
", categoryType=" + categoryType +
'}';
}
}
直接根据模型去写接口 写完接口之后可以直接进行测试 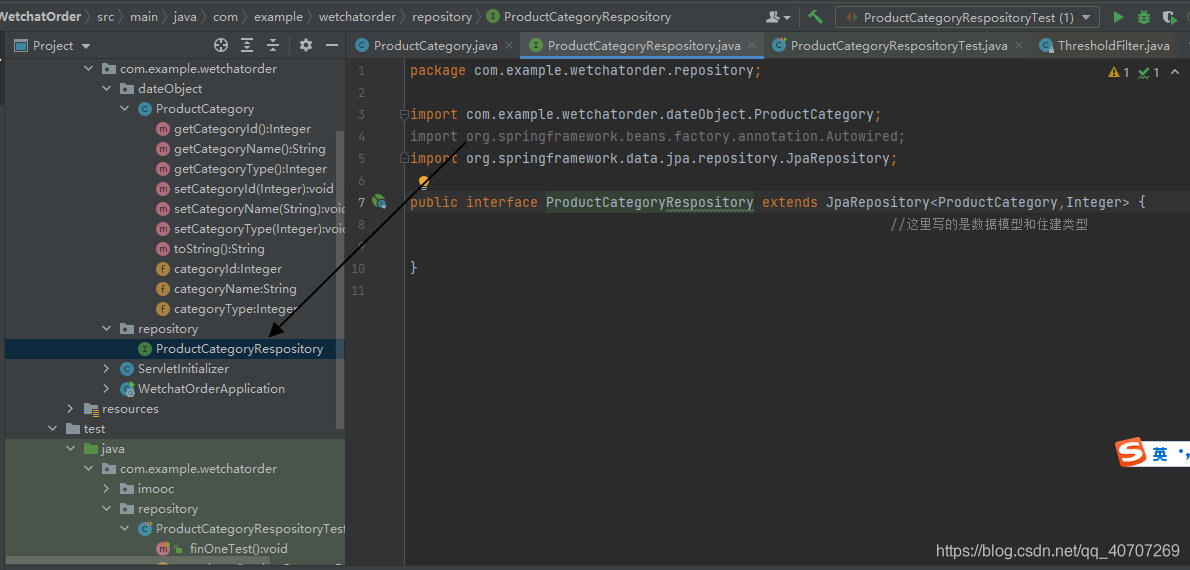
package com.example.wetchatorder.repository;
import com.example.wetchatorder.dateObject.ProductCategory;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.jpa.repository.JpaRepository;
public interface ProductCategoryRespository extends JpaRepository<ProductCategory,Integer> {
}
直接进行测试
package com.example.wetchatorder.repository;
import com.example.wetchatorder.dateObject.ProductCategory;
import lombok.extern.slf4j.Slf4j;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.*;
@RunWith(SpringRunner.class)
@SpringBootTest
@Slf4j
public class ProductCategoryRespositoryTest {
@Autowired
private ProductCategoryRespository repository;
@Test
public void finOneTest(){
Optional<ProductCategory> productCategory = repository.findById(1);
ProductCategory productCategory1 = productCategory.get();
System.out.println(productCategory1);
}
}
先测试好能不能正确联通和交互。
package com.example.wetchatorder.repository;
import com.example.wetchatorder.dateObject.ProductCategory;
import lombok.extern.slf4j.Slf4j;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringRunner;
import javax.transaction.Transactional;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import static org.junit.jupiter.api.Assertions.*;
@RunWith(SpringRunner.class)
@SpringBootTest
@Slf4j
public class ProductCategoryRespositoryTest {
@Autowired
private ProductCategoryRespository repository;
@Test
@Transactional
public void finOneTest(){
Optional<ProductCategory> productCategory = repository.findById(1);
ProductCategory productCategory1 = productCategory.get();
productCategory1.setCategoryType(15);
repository.save(productCategory1);
System.out.println(productCategory1);
}
@Test
@Transactional
public void saveTest(){
ProductCategory productCategory1 = new ProductCategory();
productCategory1.setCategoryId(2);
productCategory1.setCategoryName("可爱的狗狗");
productCategory1.setCategoryType(10);
repository.save(productCategory1);
}
@Test
public void findByCategoryTypeIn(){
List<Integer> testList = Arrays.asList(1,3,7);
List<ProductCategory> result = repository.findByCategoryTypeIn(testList);
for (ProductCategory p: result
) {
System.out.println(p);
}
}
}
|