一、Object类
Object 类是所有类的最终根类。 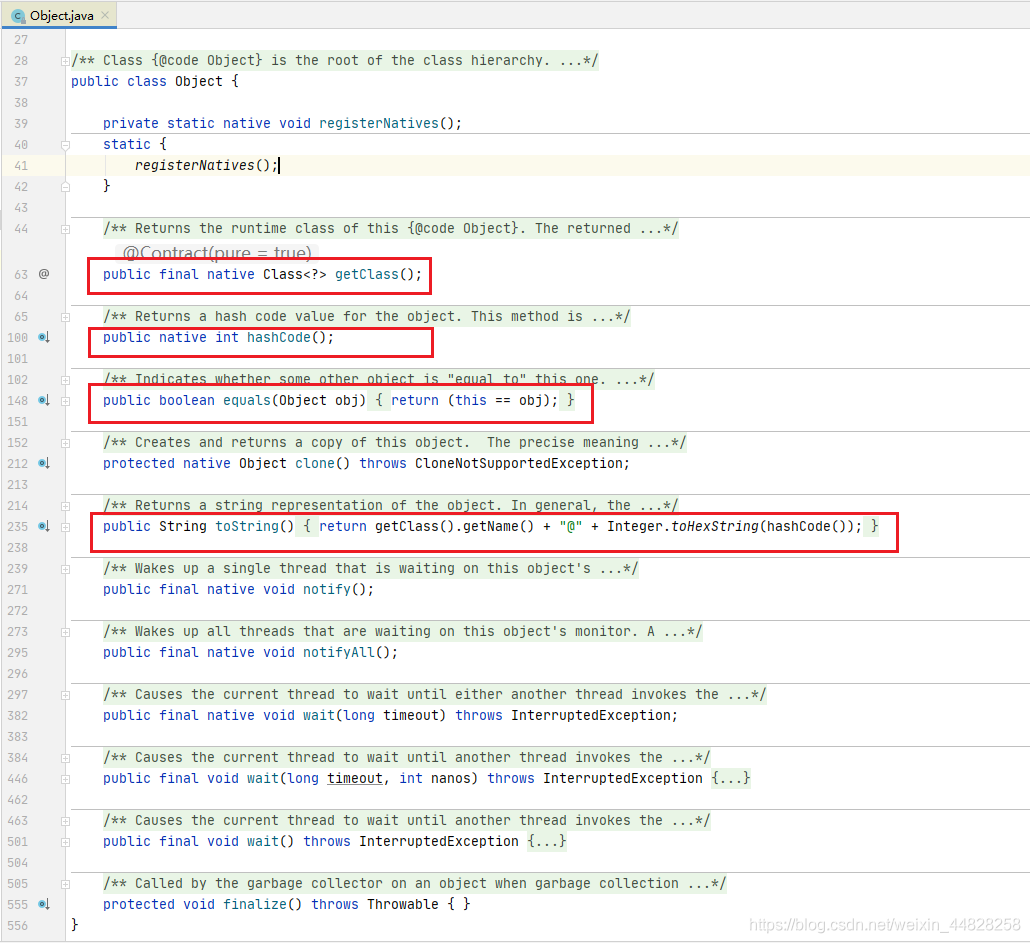 其中我们使用最多就是图片中标识的几个:
方法 | 描述 |
---|
public boolean equals(Object obj) | 表示某个其它对象是否“等于”此对象 | public final class<?> getClass() | 返回此Object的运行时类 | public int hashCode() | 返回对象的哈希码值 | public String toString() | 返回对象的字符串表示形式 |
二、Arrays类
描述: 该类包含用于操作数组的各种方法
方法 | 描述 | 举例 |
---|
public static List asList(T… a) | 返回由指定数组支持的固定大小的列表 | List stooges = Arrays.asList(“Larry”, “Moe”, “Curly”); | public static String toString(int[] a) | 返回指定数组的内容的字符串表示形式 | int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; String as = Arrays.toString(a); | public static void sort(int[] a) | 按照数字升序顺序排列指定的数组 | int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; | public static void sort(int[] a, int fromIndex, int toIndex) | 按照数字升序顺序对数组的指定范围进行排序 | int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a, 2, 7); | public static int binarySearch(int[] a, int key) | 使用二分查找算法在指定的int数组中搜索指定的值 | int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a); int index = Arrays.binarySearch(a, 5); | public static int binarySearch(int[] a, int fromIndex, int toIndex, int key) | 使用二分查找算法在指定的int数组中的指定范围搜索指定的值 | int[] a = { 10, 20, 30, 4, 5, 60, 70, 80 }; Arrays.sort(a); int index = Arrays.binarySearch(a, 2, 7, 60); |
三、Math类
描述: 该类包含执行基本数字运算的方法,如基本指数,对数,平方根和三角函数
成员变量:
变量 | 描述 |
---|
public static final double E | 比其它任何一个更接近 e ,自然对数的基数 | public static final double PI | 比其它任何一个更接近 pi ,圆周长与其直径的比率 |
成员方法:
方法 | 描述 |
---|
public static int abs(int a) | 返回值为int绝对值 | public static int max(int a, int b) | 返回两个int的较大值 | public static int min(int a, int b) | 返回两个int的较小值 | public static double floor(double a) | 返回小于或等于参数的最大整数 | public static double ceil(double a) | 返回大于或等于参数的最小整数 | public static long round(double a) | 返回四舍五入后的整数值 | public static double pow(double a, double b) | 返回a的b次幂 | public static double log(double a) | 返回log以e为底的值 | public static double sqrt(double a) | 返回a的正平方根 | public static double random() | 返回一个[0.0 , 1.0)之间的随机数 public static int getRand(double min, double max) { return (int) (Math.random() * (max - min + 1) + min); } |
四、Date类
描述: 该类是一个日期类
构造方法:
方法 | 描述 |
---|
public Date() | 构造一个 Date对象,它代表当前的毫秒值 | public Date(long date) | 使用给定的毫秒时间值构造一个Date对象 |
五、SimpleDateFormat类
5.1 将日期格式化为字符串
Date d = new Date();
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
String s = sdf.format(d);
5.2 将字符串格式化为日期
SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd hh:mm:ss");
Date d = sdf.parse("2020-07-15 01:02:03");
六、Calendar类
Calendar c = Calendar.getInstance();
int year = c.get(Calendar.YEAR);
int month = c.get(Calendar.MONTH) + 1;
int day = c.get(Calendar.DATE);
int hour = c.get(Calendar.HOUR);
int minute = c.get(Calendar.MINUTE);
int second = c.get(Calendar.SECOND);
System.out.println(year + "-" + month + "-" + day + " " + hour + ":" + minute + ":" + second);
七、String类
描述: 该类代表字符串
构造方法:
方法 | 描述 |
---|
public String() | 初始化构造一个空白字符串 | public String(char[] value) | 通过字符数组初始化字符串 | public String(byte[] bytes) | 通过使用平台的默认字符集解码指定的字节数组来构造新的String | public String(byte[] bytes, Charset charset) | 通过使用自定义的字符集解码指定的字节数组来构造新的String | public String(StringBuffer buffer) | 字符串缓冲区的内容被复制,重新分配一个新的字符串 | public String(StringBuilder builder) | 字符串构建器的内容被复制,重新分配一个新的字符串 |
成员方法:
方法 | 描述 |
---|
public int length() | 返回此字符串的长度 | public int indexOf(int ch) | 返回指定字符第一次出现的字符串内的索引 | public int lastIndexOf(int ch) | 返回指定字符的最后一次出现的字符串中的索引 | public int indexOf(String str) | 返回指定子字符串第一次出现的字符串内的索引 | public int lastIndexOf(String str) | 返回指定子字符串最后一次出现的字符串中的索引 | public char charAt(int index) | 返回char指定索引处的值 | public char[] toCharArray() | 将此字符串转换为新的字符数组 | public static String valueOf(int i) | 返回int参数的字符串int形式 | public String trim() | 返回一个删除前后空格的字符串 | public String toString() | 返回当前字符串 | public String concat(String str) | 将指定的字符串连接到该字符串的末尾 | public String toLowerCase() | 返回一个转换为小写的字符串 | public String toUpperCase() | 返回一个转换为大写的字符串 | public String substring(int beginIndex) | 返回一个以指定索引处的字符开头到该字符串的末尾的子字符串 | public String substring(int beginIndex, int endIndex) | 返回一个以指定索引处的字符开头到指定索引处的字符结尾的子字符串 | public String replace(char oldChar, char newChar) | 返回一个替换所有旧字符后的新字符串 | public String replaceAll(String regex, String replacement) | 返回一个替换所有符合正则表达式字符串后的新字符串 | public String[] split(String regex) | 根据正则表达式拆分字符串并返回拆分后的字符串数组 | public boolean startsWith(String prefix) | 判断此字符串是否以指定的前缀开头 | public boolean endsWith(String suffix) | 判断此字符串是否以指定的后缀结尾 | public boolean matches(String regex) | 根据正则表达式判断当前字符串是否符合要求 | public boolean isEmpty() | 判断当前字符串是否为空字符串 | public boolean contains(CharSequence s) | 判断当前字符串是否包含s字符 | public boolean equals(Object anObject) | 判断当前字符串是否和anObject相等 |
注意事项:
StringBuffer | StringBuilder |
---|
内容可以变 | 内容可以变 | 线程安全 | 线程不安全 | 多线程操作字符串 | 单线程操作字符串 | StringBuffer sb = new StringBuffer(); sb.append(“Hello”); sb.append(" "); sb.append(“World”); System.out.println(sb.toString()); | StringBuilder sb = new StringBuilder(); sb.append(“Hello”); sb.append(" "); sb.append(“World”); System.out.println(sb.toString()); |
八、八种包装类
基本类型| 包装类
byte | Byte |
---|
short | Short | int | Integer | long | Long | float | Float | double | Double | char | Character | boolean | Boolean |
自动装箱:把基本数据类型转换为对应的包装类类型
自动拆箱:把包装类类型转换为对应的基本数据类型
九、BigInteger类
BigInteger bi1 = new BigInteger("100");
BigInteger bi2 = new BigInteger("50");
System.out.println("add:" + bi1.add(bi2));
System.out.println("subtract:" + bi1.subtract(bi2));
System.out.println("multiply:" + bi1.multiply(bi2));
System.out.println("divide:" + bi1.divide(bi2));
十、BigDecimal类
BigDecimal bd1 = new BigDecimal("0.09");
BigDecimal bd2 = new BigDecimal("0.01");
System.out.println("add:" + bd1.add(bd2));
BigDecimal bd3 = new BigDecimal("1.0");
BigDecimal bd4 = new BigDecimal("0.32");
System.out.println("subtract:" + bd3.subtract(bd4));
BigDecimal bd5 = new BigDecimal("1.015");
BigDecimal bd6 = new BigDecimal("100");
System.out.println("multiply:" + bd5.multiply(bd6));
BigDecimal bd7 = new BigDecimal("1.301");
BigDecimal bd8 = new BigDecimal("100");
System.out.println("divide:" + bd7.divide(bd8));
十一、异常类
**概述:**异常就是程序出现了不正常的情况 体系: 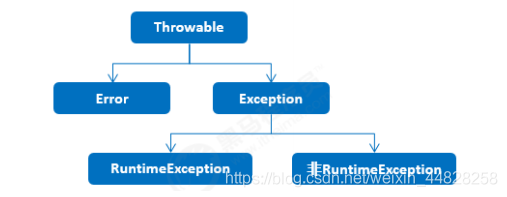 解决:
11.1 方案一:用try-catch方式处理异常
try {
可能出现异常的代码;
} catch(异常类名 变量名) {
异常的处理代码;
}
11.2 方案二:用throws方式处理异常
public void 方法名() throws 异常类名 {
}
注意:  案例:
class ScoreException extends Exception {
public ScoreException() {}
public ScoreException(String message) {
super(message);
}
}
class Teacher {
public void checkScore(int score) throws ScoreException {
if (score < 0 || score > 100) {
throw new ScoreException("你给的成绩有误,分数应该在0-100之间");
} else {
System.out.println("你给的成绩正常");
}
}
}
public class Main {
public static void main(String[] args) {
try {
Teacher s = new Teacher();
s.checkScore(120);
} catch (ScoreException e) {
e.printStackTrace();
}
}
}
|