public static void main(String[] args) {
Student a = new Student();
Student b = new Student();
a.setName("zhangsan");
b.setName("lisi");
System.out.println(a);
System.out.println(b);
swap1(a,b);
System.out.println(a);
System.out.println(b);
swap(a,b);
System.out.println(a);
System.out.println(b);
int c = 10;
int d = 20;
Integer e = 10;
Integer f = 20;
swap(c,d);
System.out.println(c);
System.out.println(d);
swap(e,f);
System.out.println(e);
System.out.println(f);
}
private static void swap1(Student e, Student f){
String name = e.getName();
e.setName(f.getName());
f.setName(name);
}
private static void swap(Student e, Student f){
Student a1 = e;
e = f;
f = a;
}
private static void swap(int a,int b){
int c = a;
a = b;
b = c;
}
private static void swap(Integer a,Integer b){
Integer c = a;
a = b;
b = c;
}
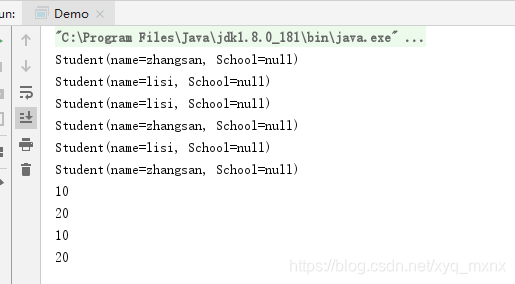
?
?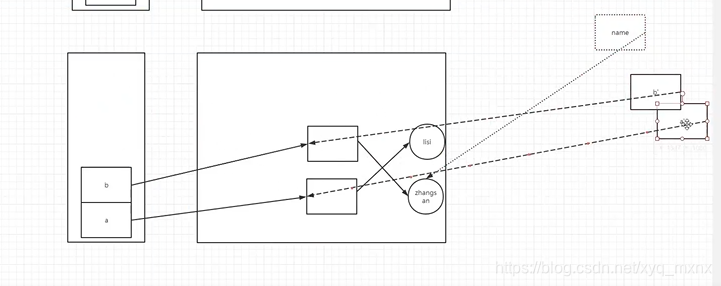
引用类型 a b 在执行swap1方法时,会新引入栈针,name是指向e(也就是图中的a',a'与a指向同一个对象)的name的,所以e和f的name值会交换(也就是图中的a' b'对应的name值),方法执行结束,name,e(a'),f(b')会出栈,此时a b 内部的 name 值已经改变。?
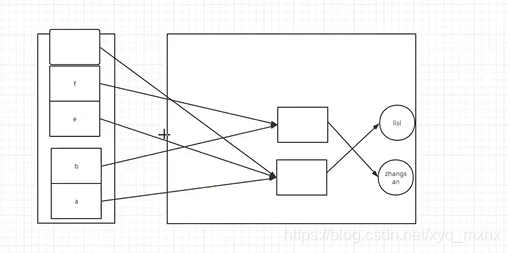
?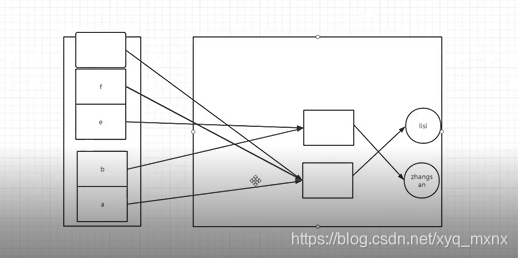
?
而在执行swap方法时,a1指向的是e这个对象,也就是a指向的对象,当e和f指向的对象交换时,并不影响a和b,方法执行完后,a1,e和f出栈,a和b仍然指向之前的对象,所以a和b不会改变。
int类型的数据是整型值,所以直接在栈里分配。
执行swap方法时,会引入新栈针,方法里的所有操作都是对a和b做的,并没有影响c和d的值,所以 swap(c,d); swap(e,f); 之后,输出的值和初始值一样。
执行方法时,会新引入栈针,无论对栈针的指向做什么操作,都不会影响方法外数据的值,但是通过栈针的指向改了里面对象的内容会对方法外的数据的值产生影响。
java中全都是值传递 而不是对象传递。因为执行方法时,栈针里新入栈的变量是复制参数的值,而不是参数指向的对象。
|