Java集合可分为Collection和Map两种体系
- Collection接口:单列数据,应以了存取一组对象的方法的集合
- List:元素有序,可重复的集合
- Set:元素无序,不可重复的集合
- Map接口:双列数据,保存具有映射关系“key-value对”的集合
Collection接口继承树 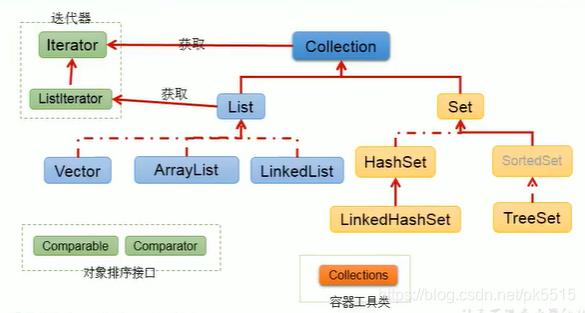 Map接口继承树 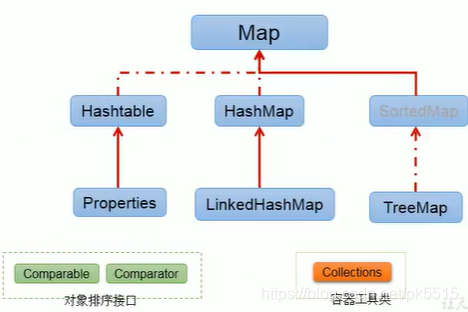
Collection接口的常用方法
package com.pan;
import org.junit.Test;
import java.util.*;
class Person{
String name;
int age;
public Person() {
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public Person(String name, int age) {
this.name = name;
this.age = age;
}
@Override
public boolean equals(Object o) {
System.out.println("Person equals...");
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
Person person = (Person) o;
return age == person.age && Objects.equals(name, person.name);
}
@Override
public int hashCode() {
return Objects.hash(name, age);
}
}
public class CollectionTest {
@Test
public void test1(){
Collection coll = new ArrayList();
coll.add("aa");
coll.add("ss");
coll.add(233);
coll.add(new Date());
System.out.println(coll.size());
Collection coll1 = new ArrayList();
coll1.add(456);
coll.addAll(coll1);
System.out.println(coll.size());
System.out.println(coll);
System.out.println(coll.isEmpty());
coll.clear();
System.out.println(coll.isEmpty());
}
@Test
public void test2(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(new String("Tom"));
coll.add(456);
coll.add(false);
coll.add(new Person("Jerry",10));
boolean contains = coll.contains(123);
System.out.println(contains);
System.out.println(coll.contains(new String("Tom")));
System.out.println(coll.contains(new Person("jerry",10)));
coll.remove(123);
System.out.println(coll);
System.out.println("*************************");
coll.remove(new Person("Jerry",10));
System.out.println(coll);
Collection coll1 = Arrays.asList(123,456);
coll.removeAll(coll1);
System.out.println(coll);
}
@Test
public void test3(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(new String("Tom"));
coll.add(false);
coll.add(456);
coll.add(new Person("Jerry",10));
Collection coll1 = Arrays.asList(123,456,789);
coll.retainAll(coll1);
System.out.println(coll);
}
@Test
public void test4(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(new String("Tom"));
coll.add(false);
coll.add(456);
coll.add(new Person("Jerry",10));
System.out.println(coll.hashCode());
Object[] objects = coll.toArray();
for(int i = 0; i < objects.length;i++){
System.out.println(objects[i]);
}
List<String> list = Arrays.asList(new String[]{"aa","bb","cc"});
System.out.println(list);
}
}
使用迭代器和remove删除集合中的数据
package com.pan;
import org.junit.Test;
import java.util.ArrayList;
import java.util.Collection;
import java.util.Iterator;
public class IteratorTest {
@Test
public void test1(){
Collection coll = new ArrayList();
coll.add(123);
coll.add(new String("Tom"));
coll.add(false);
coll.add(456);
coll.add(new Person("Jerry",10));
Iterator iterator = coll.iterator();
while(iterator.hasNext()){
Object obj = iterator.next();
if("Tom".equals(obj)){
iterator.remove();
}
}
Iterator iterator1 = coll.iterator();
while(iterator1.hasNext()) {
System.out.println(iterator1.next());
}
}
}
|