1. 算术运算符
1.1 运算符和表达式
- 运算符:对常量或者变量进行操作的符号
- 表达式:用运算符把常量或者变量连接起来符合java语法的式子就可以称为表达式。不同运算符连接的表达式体现的是不同类型的表达式。
- 举例说明:
int a = 10; int b = 20; int c = a + b; +∶是运算符,并且是算术运算符 a +b:是表达式,由于+是算术运算符,所以这个表达式叫算术表达式
1.2 算术运算符
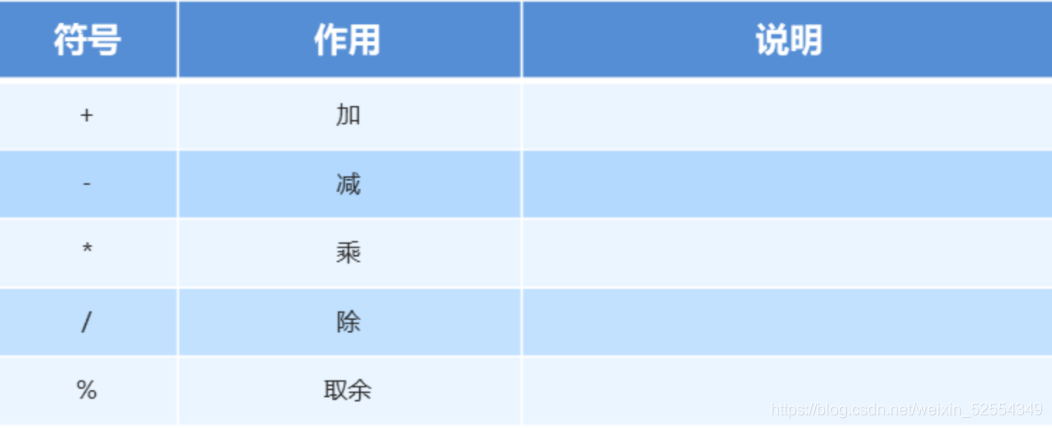
public class VariableDemo02 {
public static void main(String[] args) {
int a = 10;
int b = 20;
System.out.println(a + b);
System.out.println(a - b);
System.out.println(a * b);
System.out.println(a / b);
System.out.println(a % b);
}
}
除法得到的是商,取余得到是余数 整数相除只能得到整数,要想得到小数,必须有浮点数的参与
注意事项
/和%的区别:两个数据做除法,/取结果的商,%取结果的余数。整数操作只能得到整数,要想得到小数,必须有浮点数参与运算。
1.3. 字符的+操作
拿字符在计算机底层对应的数值来进行计算的  算术表达式中包含多个基本数据类型的值的时候,整个算术表达式的类型会自动进行提升。 提升规则:
- byte类型,short类型和char类型将被提升到int类型
- 整个表达式的类型自动提升到表达式中最高等级操作数同样的类型
- 等级顺序: byte,short,char → int → long → float → double
public class VariableDemo02 {
public static void main(String[] args) {
int a = 10;
char b = 'A';
b = 'a';
b = '0';
System.out.println(a + b);
}
}
1.4. 字符串的+操作
当“+”操作中出现字符串时,这个”+”是字符串连接符,而不是算术运算。
- “diannao” + 666
在”+”操作中,如果出现了字符串,就是连接运算符,否则就是算术运算。当连续进行“+”操作时,从左到右逐个执行。 - 6 + 66 + “电脑”
public class VariableDemo02 {
public static void main(String[] args) {
System.out.println("dian" + "nao");
System.out.println("diannao" + 666);
System.out.println(666 + "diannao");
System.out.println("电脑" + 6 + 66);
System.out.println(6 + 66 + "电脑");
}
}
2. 赋值运算符
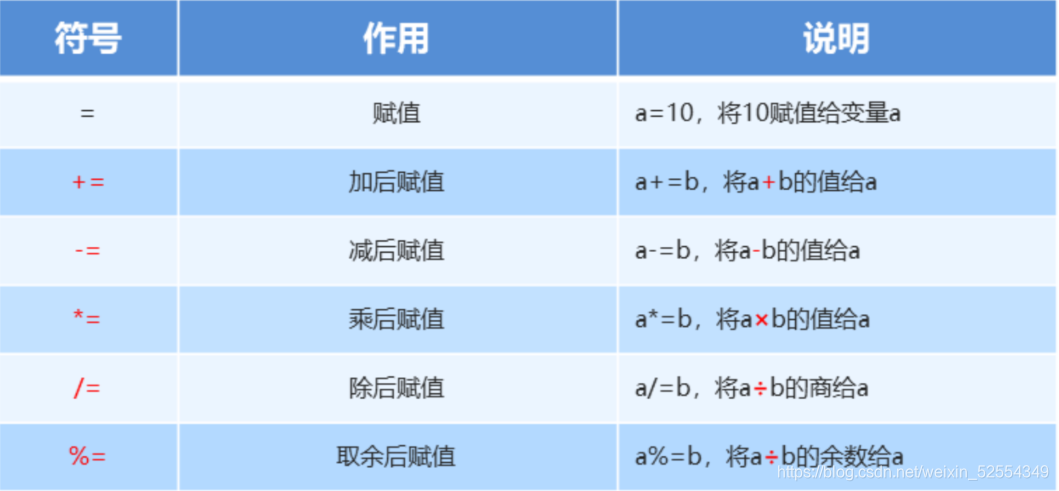
public class VariableDemo02 {
public static void main(String[] args) {
int i = 10;
System.out.println("i:" + i);
i += 20;
System.out.println("i: " + i);
short s = 10;
s += 20;
s = (short) (s + 20);
System.out.println("s : " + s);
}
}
注意事项:
3. 自增自减运算符
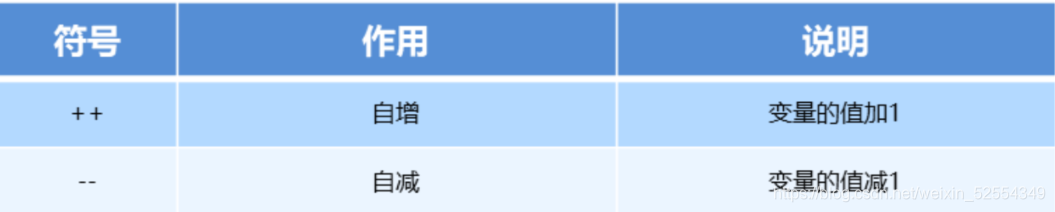 注意事项:
- ++和–既可以放在变量的后边,也可以放在变量的前边。
- 单独使用的时候,++和–无论是放在变量的前边还是后边,结果是一样的。
- 参与操作的时候,如果放在变量的后边,先拿变量参与操作,后拿变量做++或者–。
- 参与操作的时候,如果放在变量的前边,先拿变量做++或者–,后拿变量参与操作。
最常见的用法:单独使用
public class VariableDemo02 {
public static void main(String[] args) {
int i = 10;
System.out.println("i:" + i);
int j = i++;
System.out.println("i:" + i);
System.out.println("j:" + j);
int j = ++i;
System.out.println("i:" + i);
System.out.println("j:" + j);
}
}
4. 关系运算符
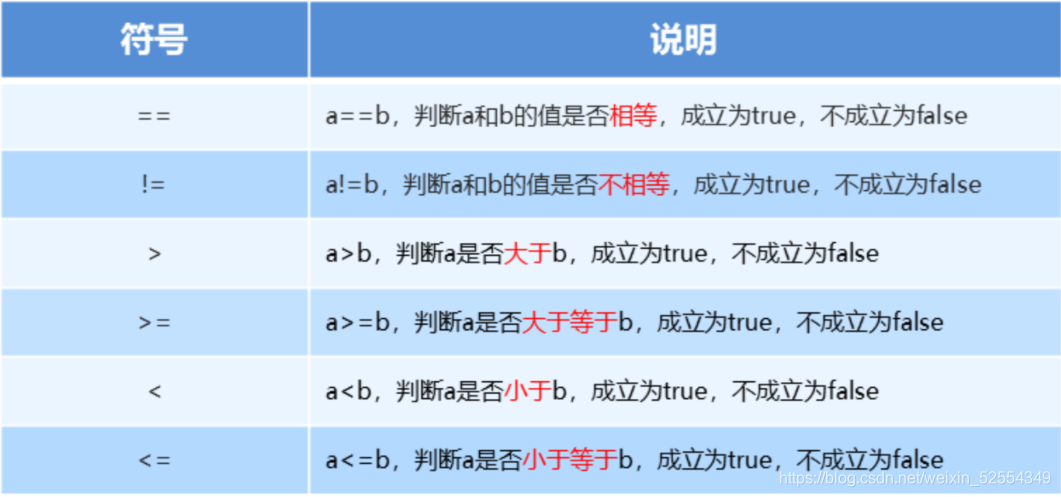 注意事项:
- 关系运算符的结果都是boolean类型,要么是true,要么是false。
- 千万不要把“==”误写成“=”。
public class VariableDemo02 {
public static void main(String[] args) {
int i = 10;
int j = 20;
int k = 10;
System.out.println(i == j);
System.out.println(i == k);
System.out.println(i != j);
System.out.println(i != k);
System.out.println(i > j);
System.out.println(i > k);
System.out.println(i >= j);
System.out.println(i >= k);
System.out.println(i = j);
}
}
5. 逻辑运算符
5.1 逻辑运算符概述
在数学中,一个数据x,大于3,小于6,我们可以这样来进行表示:3<x<6。 在Java中,需要把上面的式子先进行拆解,再进行合并表达。
- 拆解为: x>3和x<6
- 合并后: x>3&& x<6
&&其实就是一个逻辑运算符。
1.我们可以这样说,逻辑运算符,是用来连接关系表达式的运算符。
2.当然,逻辑运算符也可以直接连接布尔类型的常量或者变量。
5.2 逻辑运算符
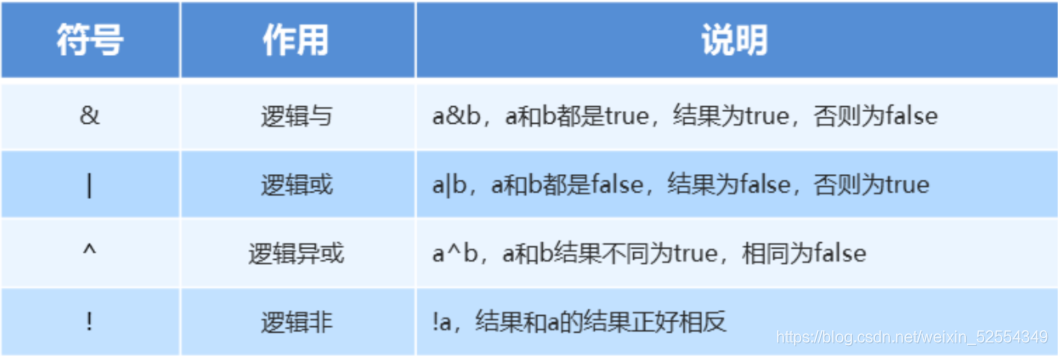
public class VariableDemo02 {
public static void main(String[] args) {
int i = 10;
int j = 20;
int k = 30;
System.out.println((i > j) & (i > k));
System.out.println((i < j) & (i > k));
System.out.println((i > j) & (i < k));
System.out.println((i < j) & (i < k));
System.out.println("=============================");
System.out.println((i > j) | (i > k));
System.out.println((i < j) | (i > k));
System.out.println((i > j) | (i < k));
System.out.println((i < j) | (i < k));
System.out.println("=============================");
System.out.println((i > j) ^ (i > k));
System.out.println((i < j) ^ (i > k));
System.out.println((i > j) ^ (i < k));
System.out.println((i < j) ^ (i < k));
System.out.println("=============================");
System.out.println((i > j));
System.out.println(!(i > j));
System.out.println(!!(i > j));
System.out.println(!!!(i > j));
}
}
5.3 短路逻辑运算符
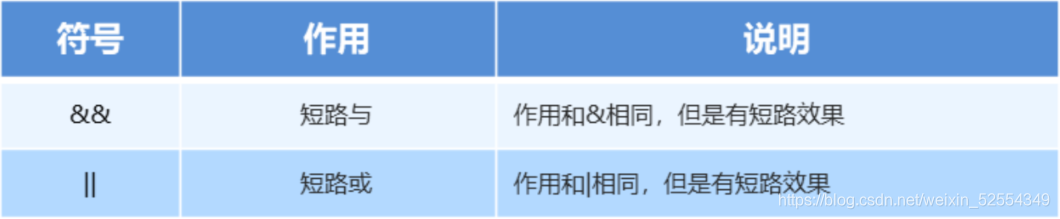 注意事项:
- 逻辑与&,无论左边真假,右边都要执行。
- 短路与&&,如果左边为真,右边执行;如果左边为假,右边不执行。
- 逻辑或|,无论左边真假,右边都要执行。
- 短路或||,如果左边为假,右边执行;如果左边为真,右边不执行。
最常用的逻辑运算符:&&,ll,!
public class VariableDemo02 {
public static void main(String[] args) {
int i = 10;
int j = 20;
int k = 30;
System.out.println((i > j) && (i > k));
System.out.println((i < j) && (i > k));
System.out.println((i > j) && (i < k));
System.out.println((i < j) && (i < k));
System.out.println("=============================");
System.out.println((i > j) || (i > k));
System.out.println((i < j) || (i > k));
System.out.println((i > j) || (i < k));
System.out.println((i < j) || (i < k));
System.out.println("=============================");
System.out.println((i++ > 100) && (j++ > 100));
System.out.println("i:" + i);
System.out.println("j:" + j);
}
}
6. 三元运算符
6.1三元运算符
- 格式:关系表达式?表达式1:表达式2;
- 范例: a> b ? a : b;
计算规则:
- 首先计算关系表达式的值
- 如果值为true,表达式1的值就是运算结果
- 如果值为false,表达式2的值就是运算结果
public class VariableDemo02 {
public static void main(String[] args) {
int a = 10;
int b = 20;
int max = a > b ? a : b;
System.out.println("max:" + max);
}
}
6.2. 两只老虎案例
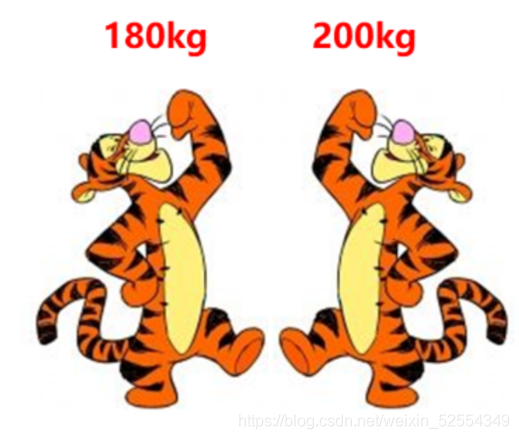
分析: ① 定义两个变量用于保存老虎的体重,单位为kg,这里仅仅体现数值即可。 int weight1 = 180; int weight2 = 200; ② 用三元运算符实现老虎体重的判断,体重相同,返回true,否则,返回false。 (weight1 == weight2) ? true : false; ③输出结果
public class VariableDemo02 {
public static void main(String[] args) {
int weight1 = 180;
int weight2 = 200;
boolean flag = (weight1 == weight2) ? true : false;
System.out.println("flag:" + flag);
}
}
6.3 三个和尚案例
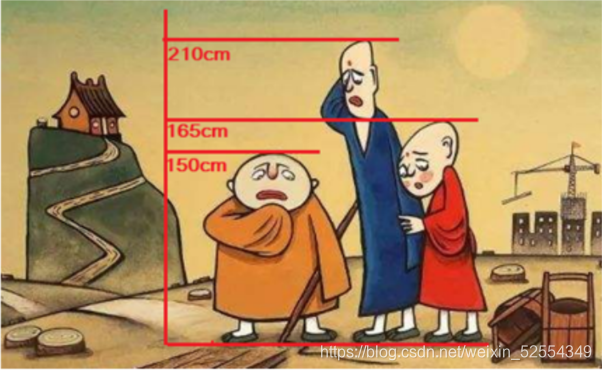
需求:一座寺庙里住着三个和尚,已知他们的身高分别为150cm.210cm、165cm,
请用程序实现获取这三个和尚的最高身高。
分析:
① 定义三个变量用于保存和尚的身高,单位为cm,这里仅仅体现数值即可。
int height1 = 150;
int height2 = 210;
int height3= 165;
② 用三元运算符获取前两个和尚的较高身高值,并用临时身高变量保存起来。
(height1 > height2) ? height1 : height2;
③ 用三元运算符获取临时身高值和第三个和尚身高较高值,并用最大身高变量
保存。
(tempHeight > height3) ? tempHeight : height3;
public class VariableDemo02 {
public static void main(String[] args) {
int height1 = 150;
int height2 = 210;
int height3 = 165;
int tempHeight = height1 > height2 ? height1 : height2;
int maxHeight = tempHeight > height3 ? tempHeight : height3;
System.out.println("maxHeight : " + maxHeight);
}
}
7. 数据输入
7.1 数据输入概述
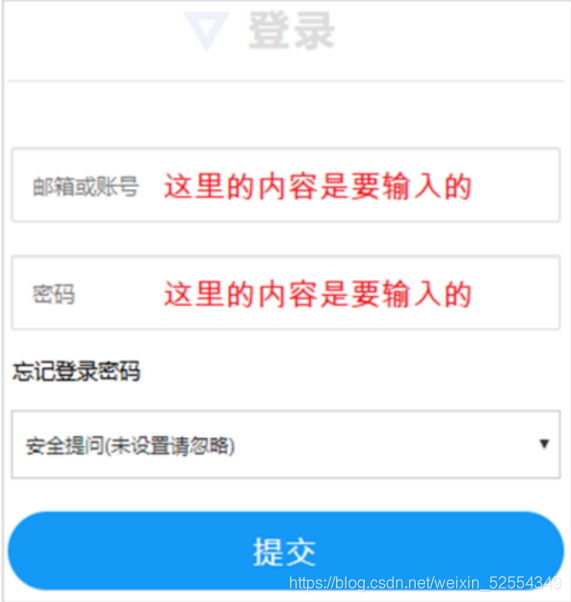
7.2 Scanner使用的基本步骤
① 导包
import java.util. Scanner ;
导包的动作必须出现在类定义的上边
② 创建对象
Scanner sc = new Scanner (System.in) ;
上面这个格式里面,只有sc是变量名,可以变,其他的都不允许变。
③ 接收数据
int i = sc.nextInt () ;
上面这个格式里面,只有i是变量名,可以变,其他的都不允许变。
public class VariableDemo02 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
int x = sc.nextInt();
System.out.println("x:" + x);
}
}
7.3 三个和尚升级版案例
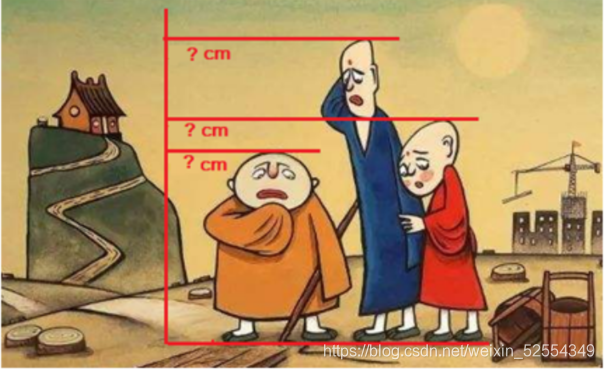
分析:
① 身高未知,采用键盘录入实现。首先导包,然后创建对象。
import java.util.Scanner;
Scanner sc = new Scanner(System.in);
② 键盘录入三个身高分别赋值给三个变量。
int height1 = sc.nextInt();
int height2 = sc.nextInt();
int height3 = sc.nextlnt();
③用三元运算符获取前两个和尚的较高身高值,并用临时身高变量保存起来。
(height1 > height2) ? height1 : height2;
④用三元运算符获取临时身高值和第三个和尚身高较高值,并用最大身高变量
保存。
(tempHeight > height3) ? tempHeight : height3;
⑤输出结果。
public class VariableDemo02 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入第一个和尚的身高:");
int heightl = sc.nextInt();
System.out.println("请输入第二个和尚的身高:");
int height2 = sc.nextInt();
System.out.println("请输入第三个和尚的身高:");
int height3 = sc.nextInt();
int tempHeight = heightl > height2 ? heightl : height2;
int maxHeight = tempHeight > height3 ? tempHeight : height3;
System.out.println("这三个和尚中身高最高的是: " + maxHeight + "cm");
}
}
8 流程控制
8.1 流程控制语句概述
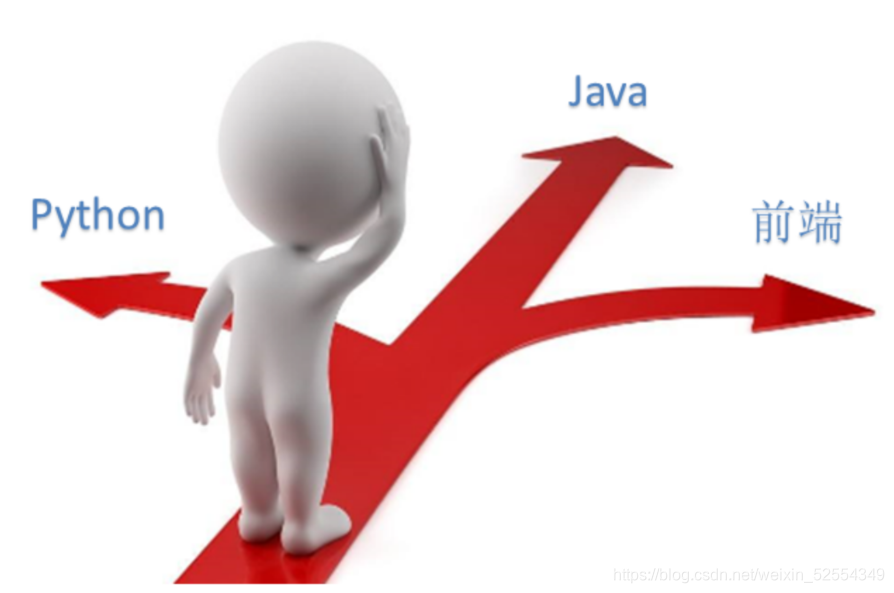
8.2 流程控制语句分类
- 顺序结构
- 分支结构(if,switch)
- 循环结构(for,while,do…while)
8.3 顺序结构
顺序结构是程序中最简单最基本的流程控制,没有特定的语法结构,按照代码的先后顺序,依次执行,程序中大多数的代码都是这样执行的。
顺序结构执行流程图: 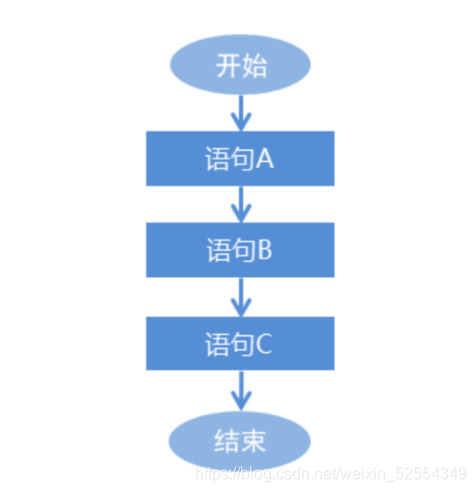
public class VariableDemo02 {
public static void main(String[] args) {
System.out.println("开始");
System.out.println("语句A");
System.out.println("语句B");
System.out.println("语句c");
System.out.println ("结束");
}
}
8.4 if语句
(1)if语句格式1
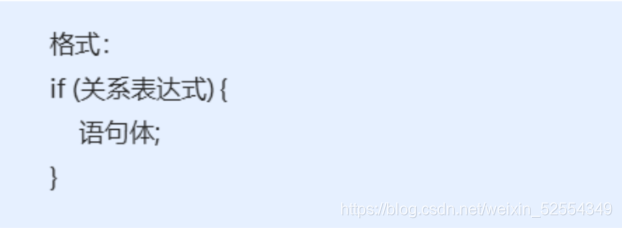 执行流程:
- 首先计算关系表达式的值
- 如果关系表达式的值为tiue就执行语句体
- 如果关系表达式的值为false就不执行语句体
- 继续执行后面的语句内容
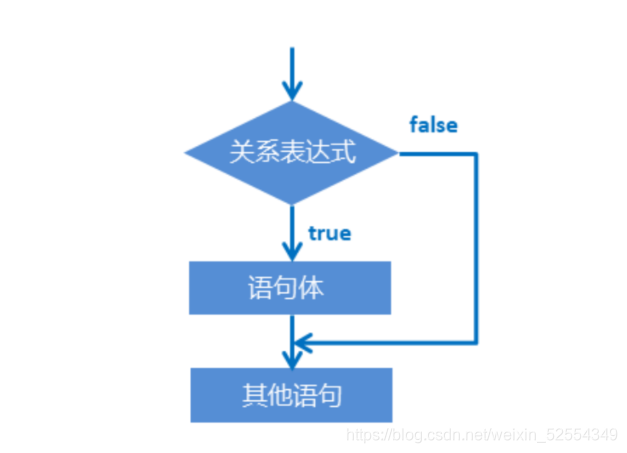
public class VariableDemo02 {
public static void main(String[] args) {
System.out.println("开始");
int a = 10;
int b = 20;
if (a == b) {
System.out.println("a等于b");
}
int c = 10;
if (a == c) {
System.out.println("a等于c");
}
System.out.println("结束");
}
}
(2)if语句格式2
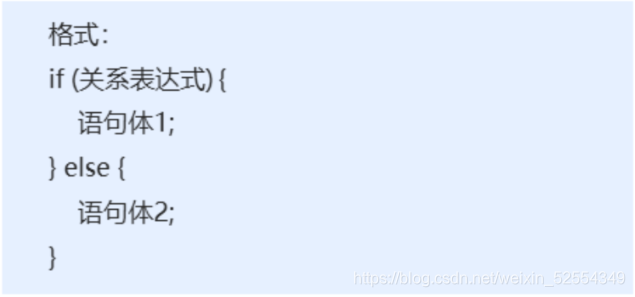 执行流程:
- ①首先计算关系表达式的值
- ②如果关系表达式的值为true就执行语句体1
- ③如果关系表达式的值为false就执行语句体2
- 继续执行后面的语句内容
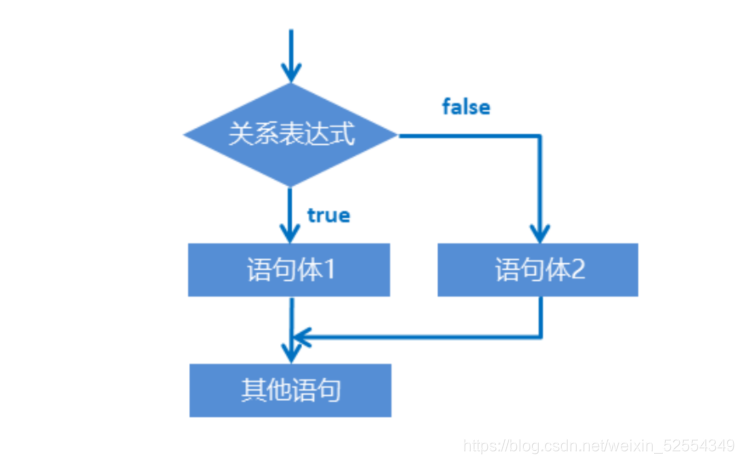
public class VariableDemo02 {
public static void main(String[] args) {
System.out.println("开始");
int a = 10;
int b = 20;
if (a > b) {
System.out.println("a的值大于b");
} else {
System.out.println("a的值不大于b");
}
System.out.println("结束");
}
}
案例:奇偶数
需求:任意给出一个整数,请用程序实现判断该整数是奇数还是偶数,并在控制台输出该整数是奇数还是偶数。
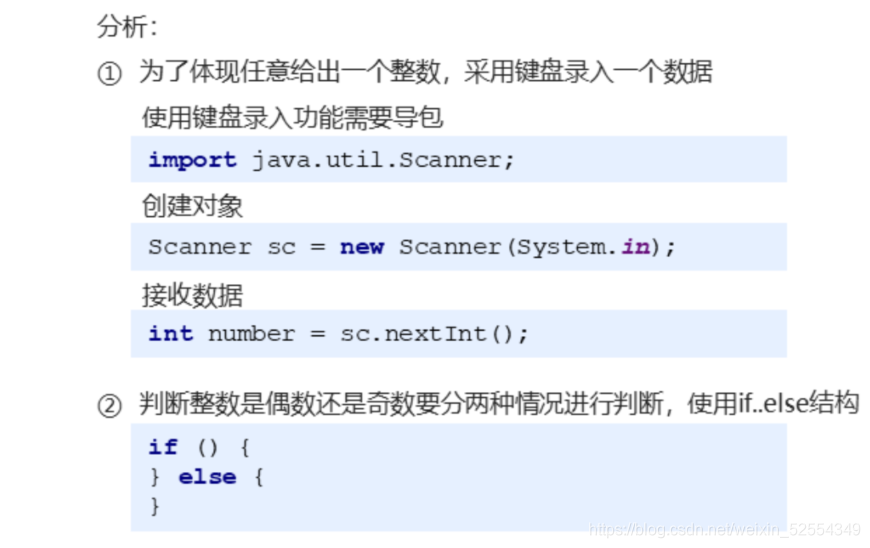 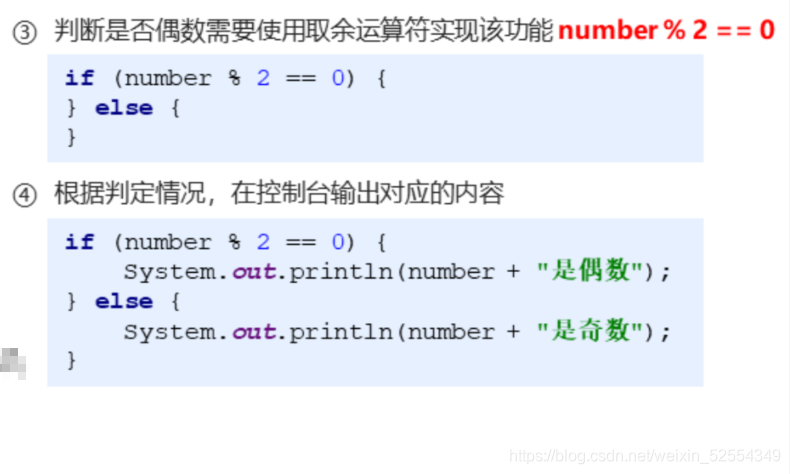
public class VariableDemo02 {
public static void main(String[] args) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个整数:");
int number = sc.nextInt();
if (number % 2 == 0) {
System.out.println(number + "是偶数");
} else {
System.out.println(number + "是奇数");
}
}
}
(3)if语句格式3
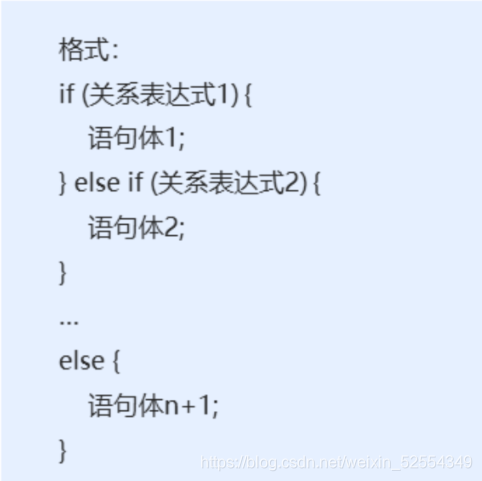 执行流程:
- ① 首先计算关系表达式1的值
- ②如果值为true就执行语句体1;如果值为false就计算关系表达式2的值
- ③如果值为true就执行语句体2;如果值为false就计算关系表达式3的值
- ④ …
- ⑤ 如果没有任何关系表达式为true,就执行语句体n+1。
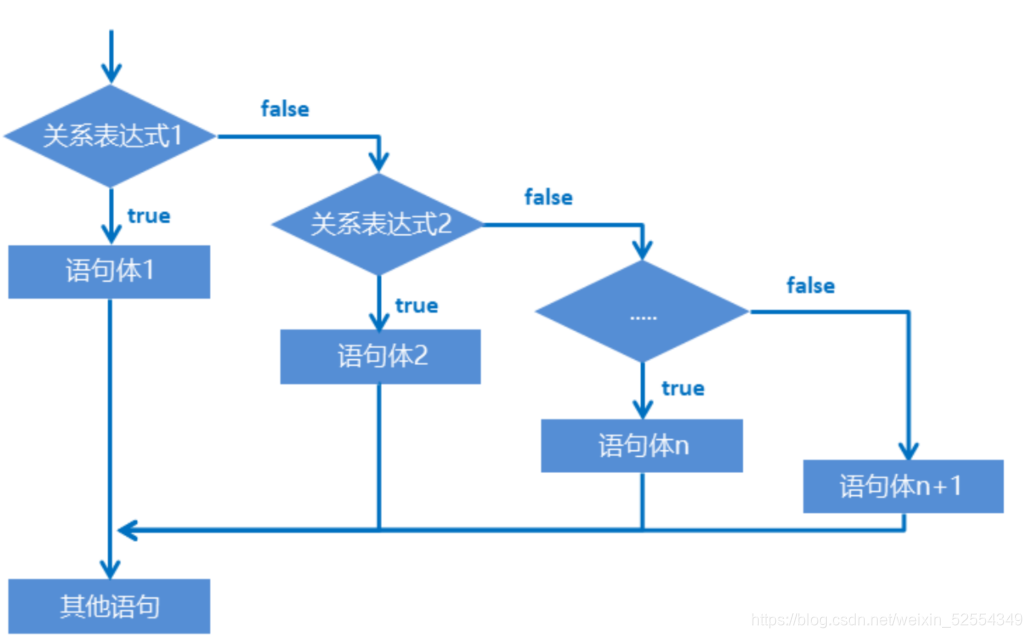
public class VariableDemo02 {
public static void main(String[] args) {
System.out.println("开始");
Scanner sc =new Scanner (System.in) ;
System.out.println ("请输入一个星期数(1-7): ");
int week = sc.nextInt() ;
if (week == 1){
System.out.println("星期一");
}else if (week == 2){
System.out.println("星期二");
}else if (week == 3){
System.out.println("星期三");
}else if (week == 4){
System.out.println("星期四");
}else if (week == 5){
System.out.println("星期五");
}else if (week == 6){
System.out.println("星期六");
}else {
System.out.println("星期日");
}
System.out.println("结束");
}
}
8.5 考试奖励案例
需求:小明快要期末考试了,小明爸爸对他说,会根据他不同的考试成绩,送他不同的礼物,
假如你可以控制小明的得分,请用程序实现小明到底该获得什么样的礼物,并在控制台输出。
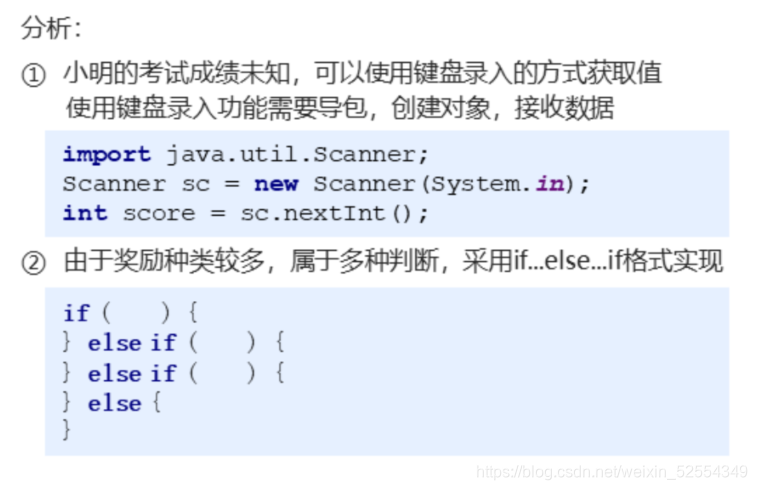 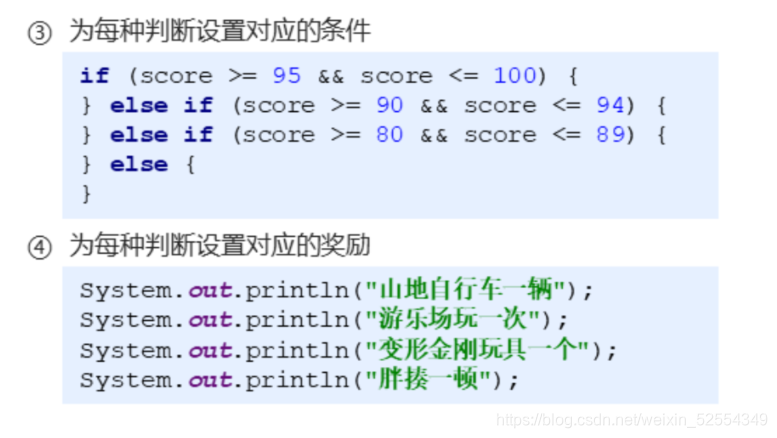
public class VariableDemo02 {
public static void main(String[] args) {
Scanner sc = new Scanner (System.in) ;
System.out.println ("请输入一个分数: ") ;
int score = sc.nextInt () ;
if (score >= 95 && score <= 100){
System.out.println("小明得分:" + score + "分,奖励:山地自行车一辆");
}else if (score >= 90 && score <= 94){
System.out.println("小明得分:" + score + "分,奖励:游乐场玩一次");
}else if (score >= 80 && score <= 95){
System.out.println("小明得分:" + score + "分,奖励:变形金刚玩具一个");
}else if (score < 80 && score >= 0){
System.out.println("小明得分:" + score + "分,奖励:胖揍一顿");
}else {
System.out.println("您输入的分数不合法");
}
}
}
|