Exception可以被程序处理的异常
异常指的是程序在运行中出现了无法预料的状况,异常发生在程序运行期间。程序中可以选择捕获异常,也可以不处理使用默认的异常
算术异常
通常是由于算术计算错误导致的
public class Gui003 {
public static void main(String[] args) {
int i=0;
int x=100;
System.out.println(x/i);
}
}
Exception in thread "main" java.lang.ArithmeticException
异常统分为两类:运行时异常和非运行时异常
常见的运行时异常: 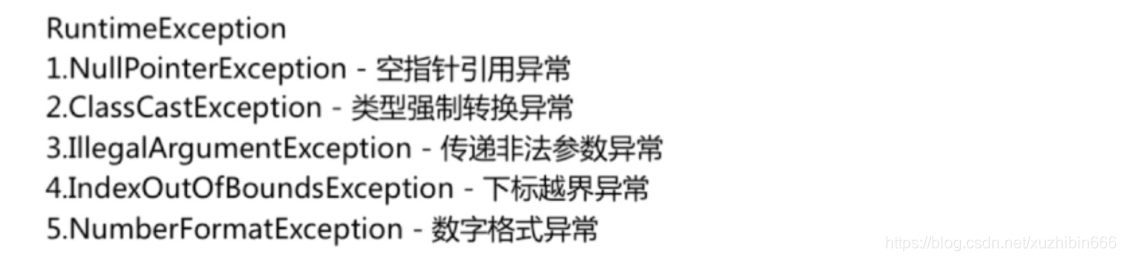
异常框架
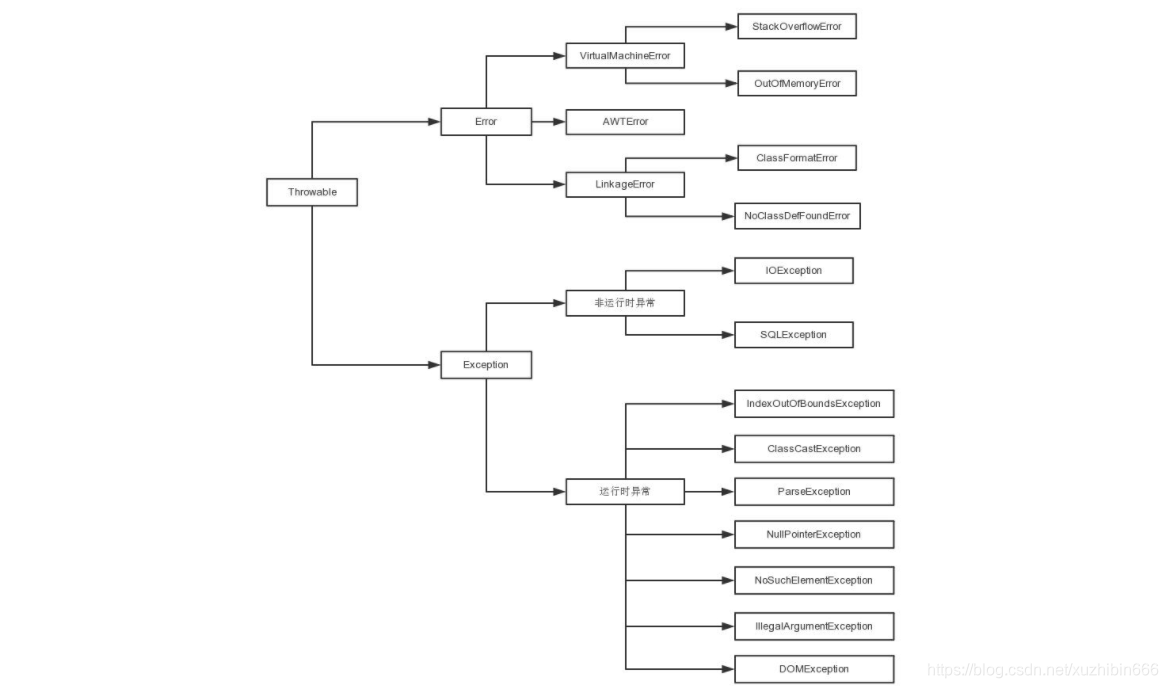
异常处理机制
处理异常的5个关键字
try catch finally throw throws
try监控代码块中的异常
try {
System.out.println(x/i);
}
catch在try代码块之后用于捕获异常
catch(ArithmeticException e){
System.out.println("算术异常");
}
捕获异常,0不能作为被除数,所以100除以0会出现异常错误
 手动捕获异常抛出
int i=0;
int x=100;
try {
System.out.println(x/i);
}catch(ArithmeticException e){
System.out.println("算术异常");
}
执行结果:
算术异常
finally关键字
finally在catch代码块之后,finally无论程序出不出异常都会执行finally代码块中的内容
finally {
System.out.println("执行finally");
}
执行结果:
执行finally
如下定义数组长度为6,输出下标为6的元素
public class exception {
public static void main(String[] args) {
int [] IntArray={1,23,45,6,7,46};
System.out.println(IntArray[6]);
}
}
提示下标越界异常  手动捕获异常
public class exception {
public static void main(String[] args) {
int [] IntArray={0,23,45,6,7,46};
try {
System.out.println(IntArray[6]);
}catch (ArrayIndexOutOfBoundsException E1){
System.out.println("数组下标越界");
}
try {
System.out.println(IntArray[5]/IntArray[0]);
}
catch (ArithmeticException E2){
System.out.println("被除数不能为0");
}
}
}
catch可以捕获多个异常,catch也相当于if else增增递进
int [] IntArray={0,23,45,6,7,46};
try {
System.out.println(IntArray[6]);
}catch (ArrayIndexOutOfBoundsException E1){
System.out.println("数组下标越界");
}
catch (ArithmeticException E2){
System.out.println("被除数不能为0");
}
在使用多条异常判断时将异常框架中最大的异常爱放置最下面
package Exception;
public class Ex1 {
public static void main(String[] args) {
int [] Array= {1,2,3,4,5};
try {
System.out.println(Array[5]);
}catch (Error error){
System.out.println("错误Error");
}catch (ArrayIndexOutOfBoundsException A){
System.out.println("数组下标越界");
}catch (Exception exception){
System.out.println("异常事项【Exception】");
}catch (Throwable throwable){
System.out.println("异常事项【Throwable】");
}
}
}
如上将最高级的异常类写置最下
执行结果:
数组下标越界
快捷键Ctrl+Alt+T快速创建 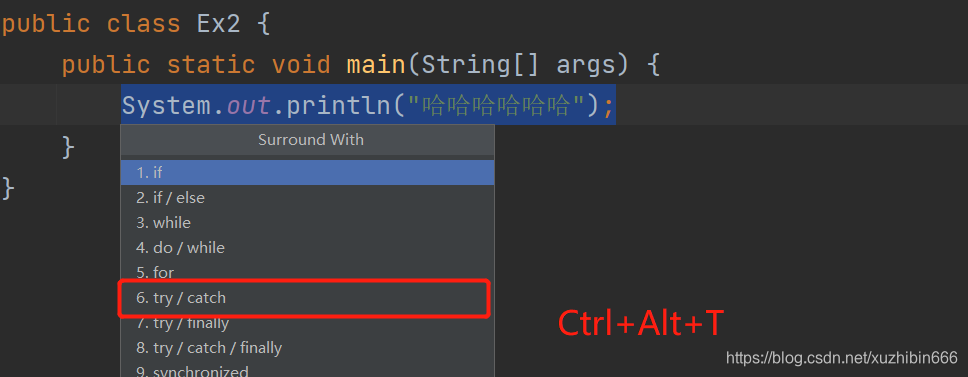
主动抛出异常
throw和 throws是运行在方法体里面的
如下代码,使用throw抛出异常,当a或b等于0时,抛出错误异常
package Exception;
public class Ex3 {
public static void main(String[] args) {
Ex3.Sum(0,2);
}
public static int Sum(int a,int b){
if (a==0||b==0){
throw new Error();
}else {
System.out.println(a / b);
} return a+b;
}
}
执行结果:
Exception in thread "main" java.lang.Error
at Exception.Ex3.Sum(Ex3.java:8)
at Exception.Ex3.main(Ex3.java:4)
主动在方法中抛出异常是throw,在方法上是throws
如下方法中定义100/0在方法中抛出异常
package Exception;
public class Ex3 {
public static void main(String[] args) {
try {
new Ex3().Demo();
}catch (ArithmeticException arithmeticException){
System.out.println("Ex:算术异常");
}
}
public void Demo() throws ArithmeticException{
System.out.println(100/0);
}
}
自定义异常
java中内置的异常类可以描述在编程时大部分的异常情况,除此,用户可以自定义异常,只需继承exception类即可
package Exception;
public class Ex4 {
public static void main(String[] args) throws ExceptionClass {
Ex4.Demo(91);
}
static void Demo(int a) throws ExceptionClass {
if(a>=10){
throw new ExceptionClass(a);
}else {
System.out.println(a);
} }
}
class ExceptionClass extends Exception{
private int detail;
public ExceptionClass( int a) {
this.detail = a;
}
@Override
public String toString() {
return "ExceptionClass{" + "detail=" + detail + '}'+"[您的值大于10]";
}
}
执行结果:
Exception in thread "main" ExceptionClass{detail=91}[您的值大于10]
at Exception.Ex4.Demo(Ex4.java:9)
at Exception.Ex4.main(Ex4.java:4)
自定义异常类手动抛出
package Exception;
import java.util.Scanner;
public class Ex5 {
public static void main(String[] args) throws MyExceptionClass {
Scanner scanner =new Scanner(System.in);
System.out.print("请输入数字:");
int num= scanner.nextInt();
if (num==0){
throw new MyExceptionClass(num);
}else if (num >10){
throw new MyExceptionClass(num);
}else {
System.out.println("数字是"+num);
}
}
}
class Ex{
}
class MyExceptionClass extends Exception{
private int number;
public MyExceptionClass(int i) {
this.number=i;
}
@Override
public String toString() {
return "MyExceptionClass{"+"number="+number+'}';
}
}
执行结果:
请输入数字:11
Exception in thread "main" MyExceptionClass{number=11}
at Exception.Ex5.main(Ex5.java:11)
请输入数字:4
数字是4
|