1 @ControllerAdvice
1.1 @ControllerAdvice 全局异常处理
- 文件上传案例
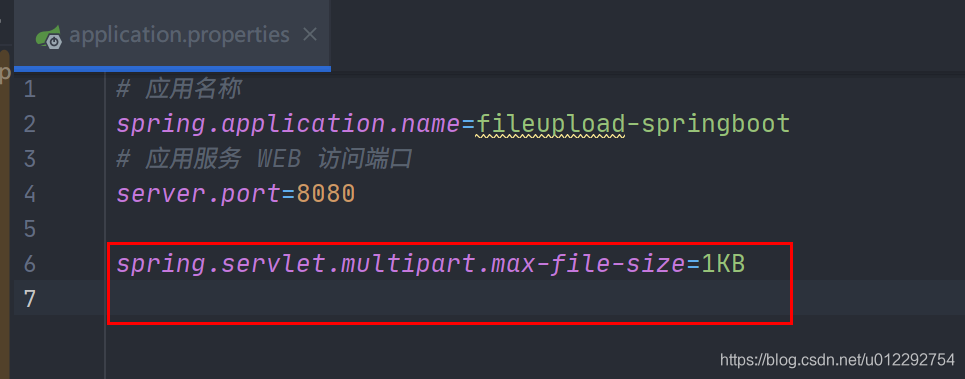
package com.tzb.exception;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.RestControllerAdvice;
@RestControllerAdvice
public class MyGlobalException {
@ExceptionHandler(Exception.class)
public String customException(Exception e) {
return e.getMessage();
}
}

1.2 全局数据绑定
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ModelAttribute;
import java.util.HashMap;
import java.util.Map;
@ControllerAdvice
public class MyGlobalData {
@ModelAttribute(name = "info")
public Map<String,String> mydata(){
Map<String,String> info = new HashMap<>();
info.put("name", "Mike");
info.put("address","ShangHai");
return info;
}
}
@RestController
public class HelloController {
@GetMapping("/hello")
public void hello(Model model ){
Map<String, Object> asMap = model.asMap();
Map<String, String> info = (Map<String, String>)asMap.get("info");
System.out.println(info);
}
}

1.3 请求参数预处理
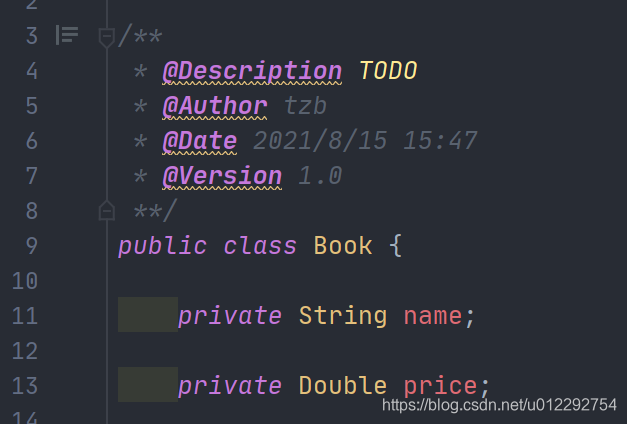 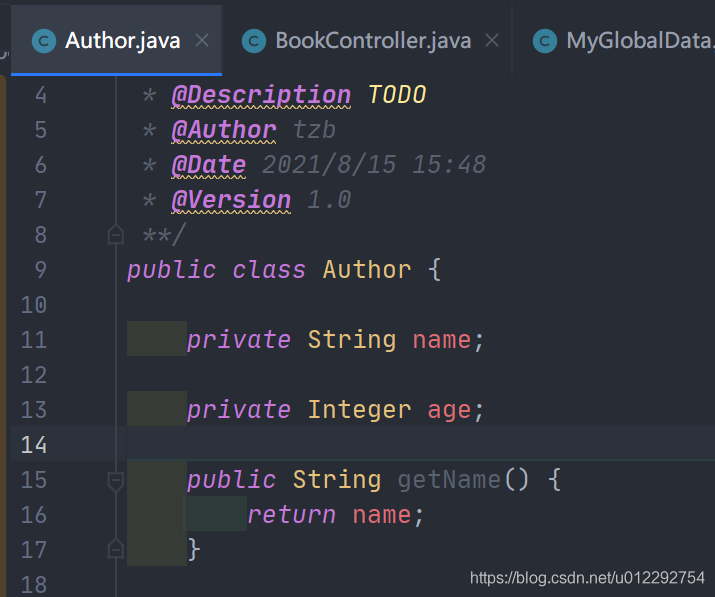
@ControllerAdvice
public class MyGlobalData {
@ModelAttribute(name = "info")
public Map<String,String> mydata(){
Map<String,String> info = new HashMap<>();
info.put("name", "Mike");
info.put("address","ShangHai");
return info;
}
@InitBinder("b")
public void b(WebDataBinder binder){
binder.setFieldDefaultPrefix("b.");
}
@InitBinder("a")
public void a(WebDataBinder binder){
binder.setFieldDefaultPrefix("a.");
}
}
@RestController
public class BookController {
@PostMapping("/book")
public void addBook(@ModelAttribute("b") Book book, @ModelAttribute("a") Author author) {
System.out.println("book:" + book);
System.out.println("author:" + author);
}
}
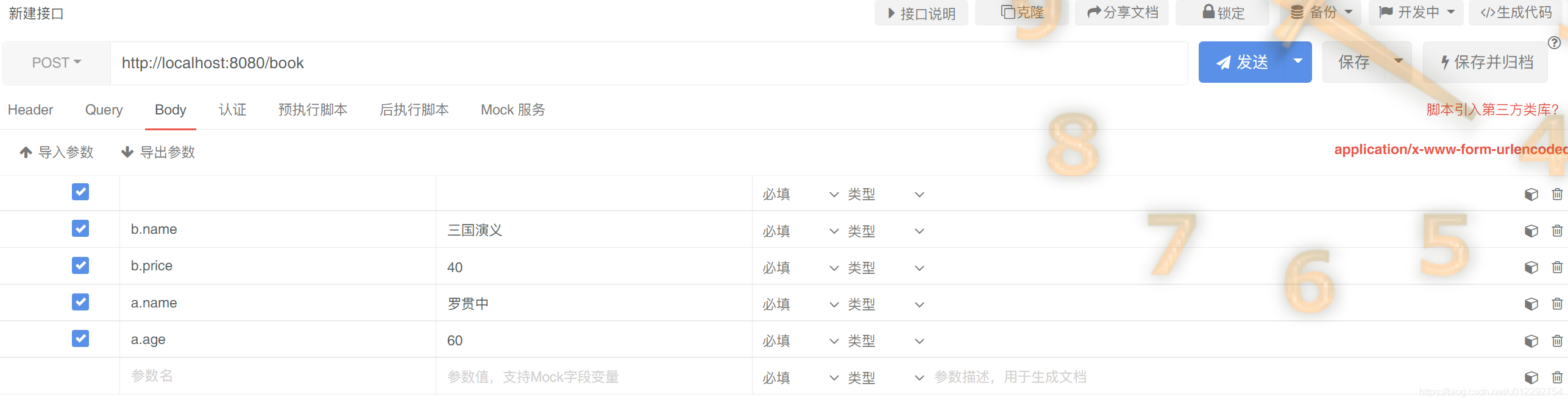 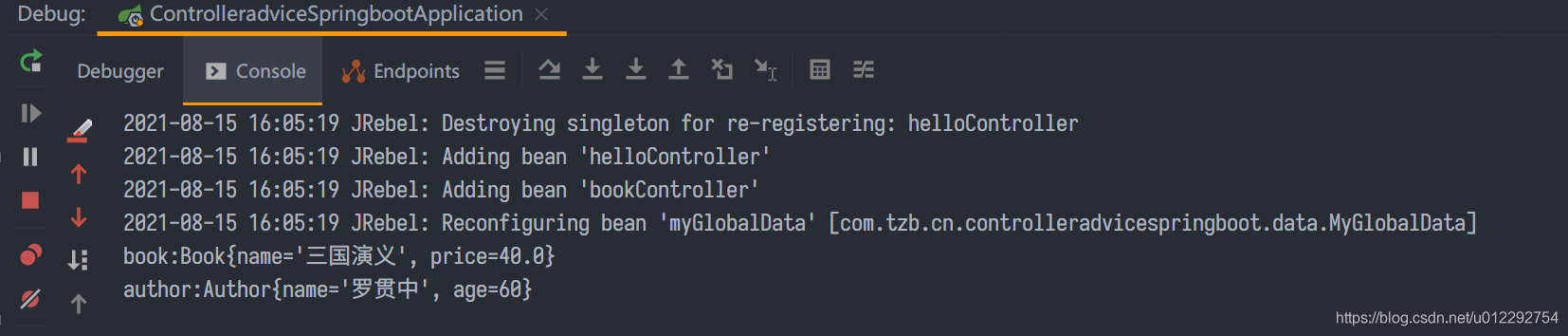
2 异常页面定义
2.1 直接根据错误码定义页面
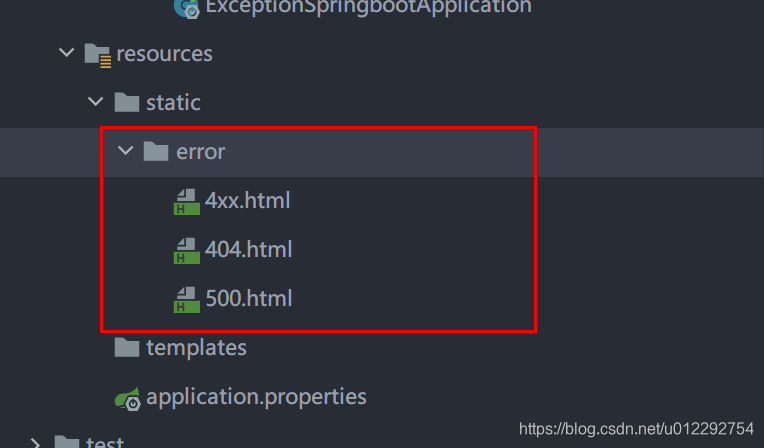
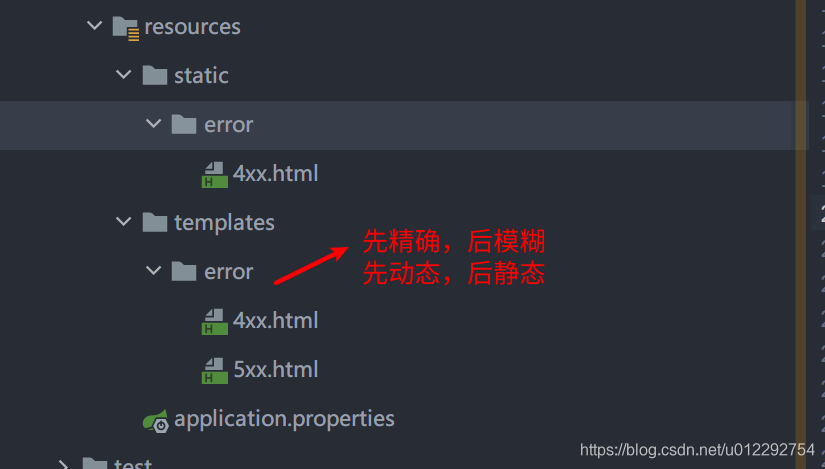 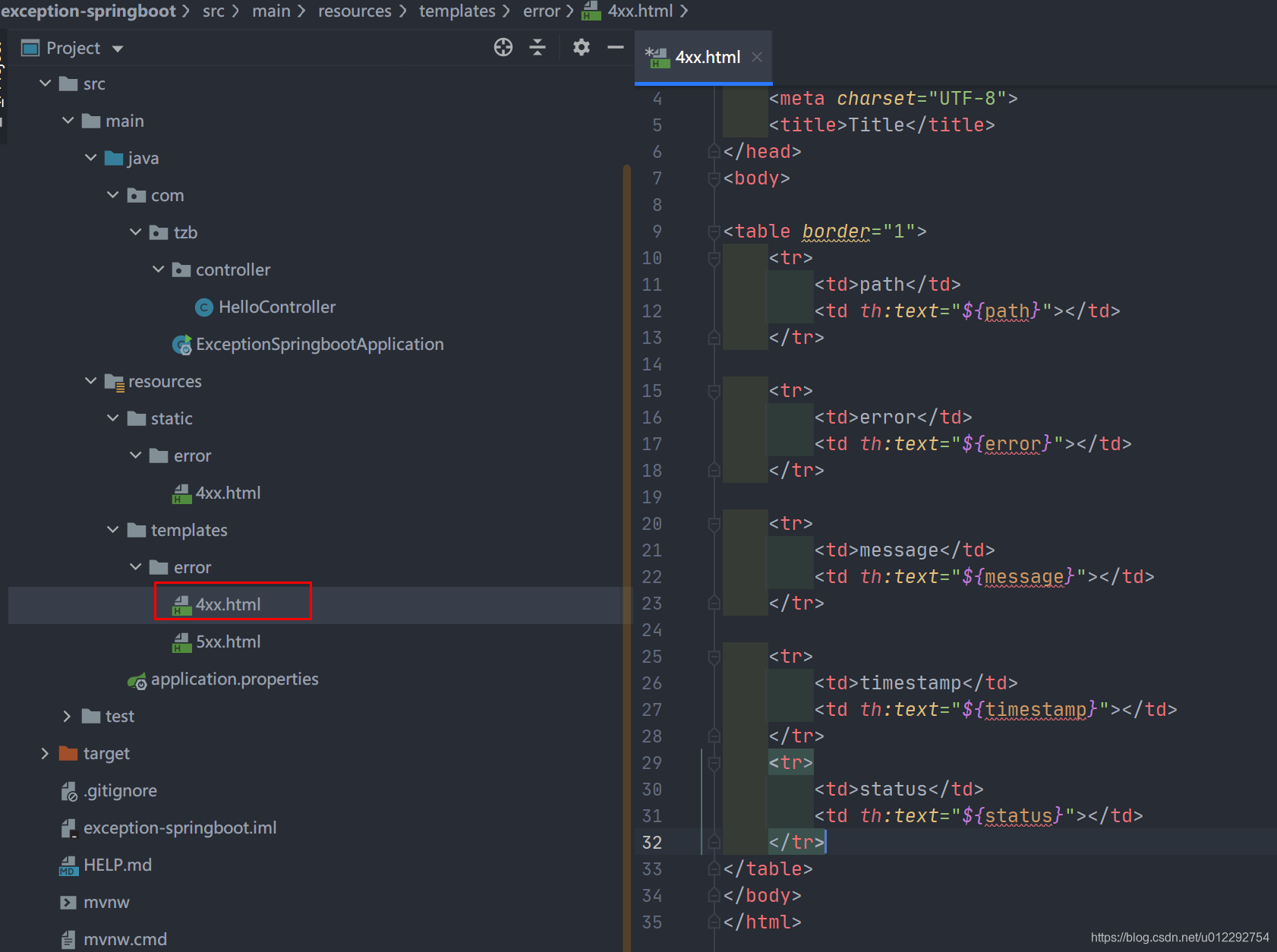 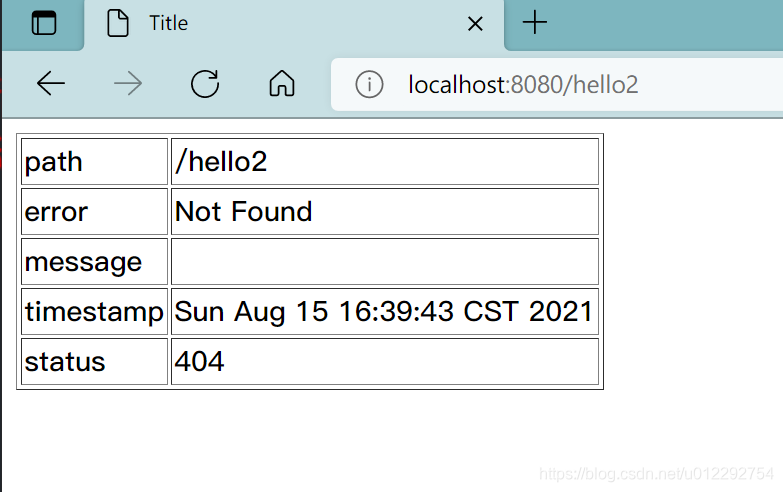
2.2 异常处理原理
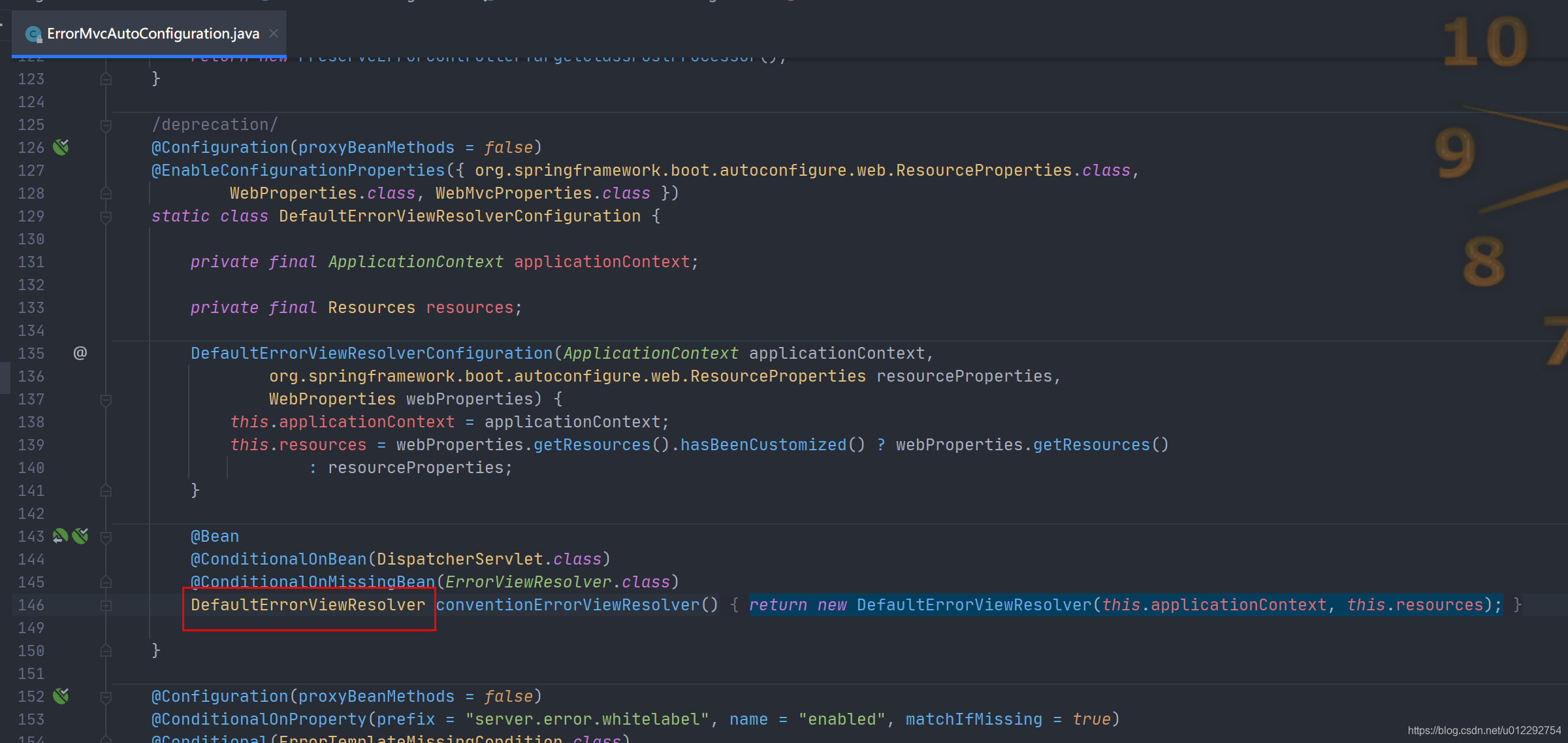
2.3 自定义异常
2.3.1 自定义数据
package com.tzb.exception;
import org.springframework.boot.web.error.ErrorAttributeOptions;
import org.springframework.boot.web.servlet.error.DefaultErrorAttributes;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.WebRequest;
import java.util.Map;
@Component
public class MyErrorAttribute extends DefaultErrorAttributes {
@Override
public Map<String, Object> getErrorAttributes(WebRequest webRequest, ErrorAttributeOptions options) {
Map<String, Object> map = super.getErrorAttributes(webRequest, options);
if ((Integer) map.get("status") == 404) {
map.put("message", "页面不存在");
}
return map;
}
}
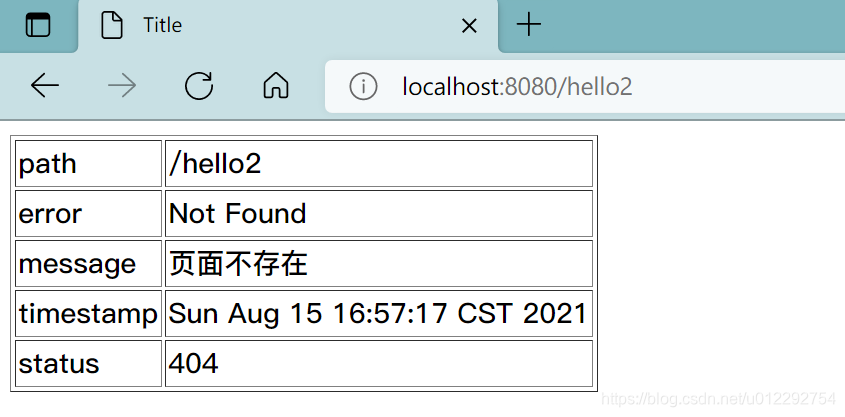
2.3.2 自定义视图
package com.tzb.exception;
import org.springframework.boot.autoconfigure.web.ResourceProperties;
import org.springframework.boot.autoconfigure.web.servlet.error.DefaultErrorViewResolver;
import org.springframework.context.ApplicationContext;
import org.springframework.http.HttpStatus;
import org.springframework.stereotype.Component;
import org.springframework.web.servlet.ModelAndView;
import javax.servlet.http.HttpServletRequest;
import java.util.HashMap;
import java.util.Map;
@Component
public class MyErrorViewResolver extends DefaultErrorViewResolver {
public MyErrorViewResolver(ApplicationContext applicationContext, ResourceProperties resourceProperties) {
super(applicationContext, resourceProperties);
}
@Override
public ModelAndView resolveErrorView(HttpServletRequest request, HttpStatus status, Map<String, Object> model) {
Map<String, Object> map = new HashMap<>();
map.putAll(model);
if((Integer)model.get("status") ==500){
map.put("message", "服务器内部错误");
}
return new ModelAndView("myerror/999", map);
}
}
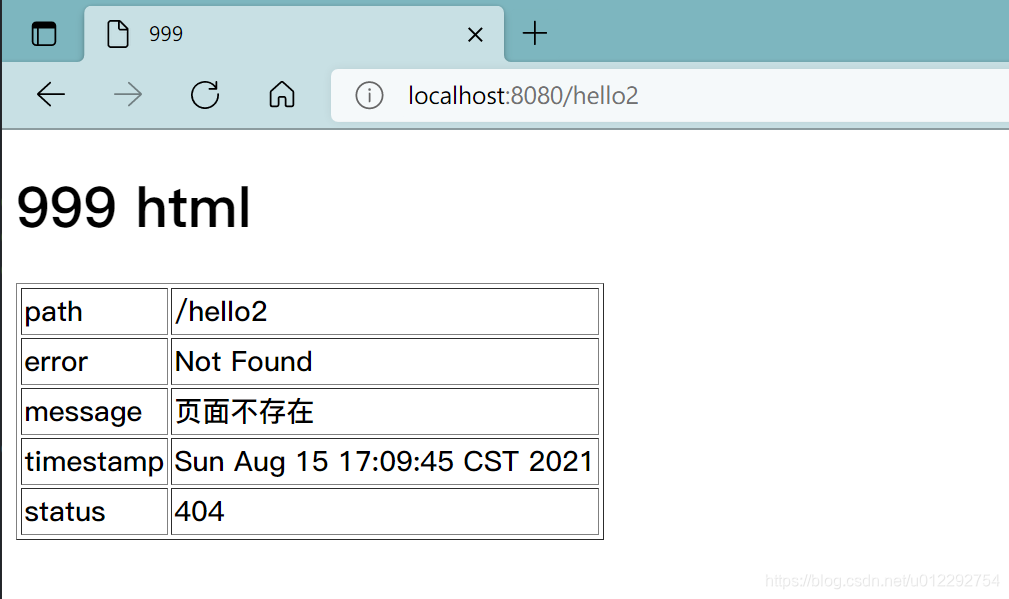
|