1.概述
Sping是一个开源的JavaEE应用程序框架,由Rod Jahnson通过其设计的interface21框架演化改进而来。Spring的核心是控制反转(IoC)和面向切面(AOP)。 简而言之,Spring是一个轻量级的控制反转(IoC)和面向切面(AOP)的框架。 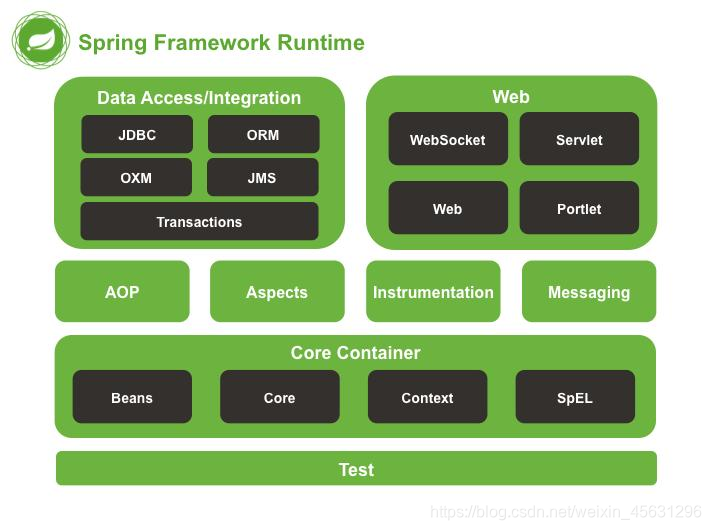
2.IoC
IoC是面向对象编程中的一种设计原则,可以用来减低代码之间的耦合度。 最常见的方式叫做依赖注入,通过控制反转,对象在被创建的时候,由一个调控系统内所有的外界实体将其所依赖的对象引用传递给它,也可以说依赖被注入对象中。 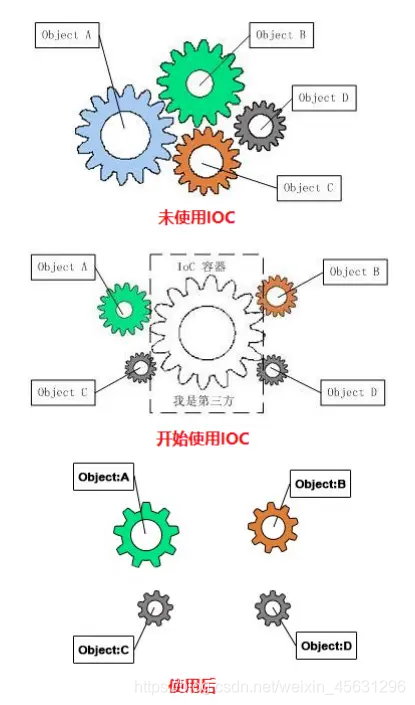
IoC的创建方式 <1>通过无参构造方式来创建 实体类:
public class Hello {
private String string;
public String getString() {
return string;
}
public void setString(String string) {
this.string = string;
}
@Override
public String toString() {
return "Hello{" +
"string='" + string + '\'' +
'}';
}
}
配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="hello" class="demo1.Hello">
<property name="string" value="Spring"/>
</bean>
</beans>
测试
public class MyTest {
public static void main(String[] args) {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("beans,xml");
Hello hello = (Hello) applicationContext.getBean("hello");
System.out.println(hello.toString());
}
}
结果  <2>通过有参数构造创建 实体类
public class User {
private String name;
public User() {
System.out.println("无参构造!");
}
public User(String name) {
this.name = name;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public void show() {
System.out.println("name=" + name);
}
}
配置文件 共有三种方式 通过类赋值
<bean id="user" class="demo1.User">
通过下标赋值
<bean id="user" class="demo1.User">
<constructor-arg index="0" value="test"/>
</bean>
通过参数名赋值
<bean id="user" class="demo1.User" name="user">
<constructor-arg name="name" value="测试"/>
</bean>
测试
public class MyTest {
public static void main(String[] args) {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("beans.xml");
User user = (User) applicationContext.getBean("user");
user.show();
}
}
通过类赋值测试结果 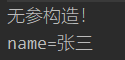 通过下标赋值测试结果 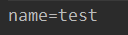 通过参数名赋值测试结果 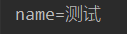
3.HelloSpring
(1)实体类
public class Hello {
private String string;
public String getString() {
return string;
}
public void setString(String string) {
this.string = string;
}
@Override
public String toString() {
return "Hello{" +
"string='" + string + '\'' +
'}';
}
}
(2)配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="hello" class="demo1.Hello">
<property name="string" value="Spring"/>
</bean>
</beans>
(3)测试
public class MyTest {
public static void main(String[] args) {
ClassPathXmlApplicationContext applicationContext = new ClassPathXmlApplicationContext("beans,xml");
Hello hello = (Hello) applicationContext.getBean("hello");
System.out.println(hello.toString());
}
}
结果 
|