9、Lombok
- Lombok 项目是一个 java 库,可自动插入编辑器并构建工具,将 java 向上弹出。
切勿再编写其他获取器或等于方法,用一个注释,您的类有一个功能齐全的建设者,自动记录变量,等等。
使用步骤
-
在IDEA中安装插件! 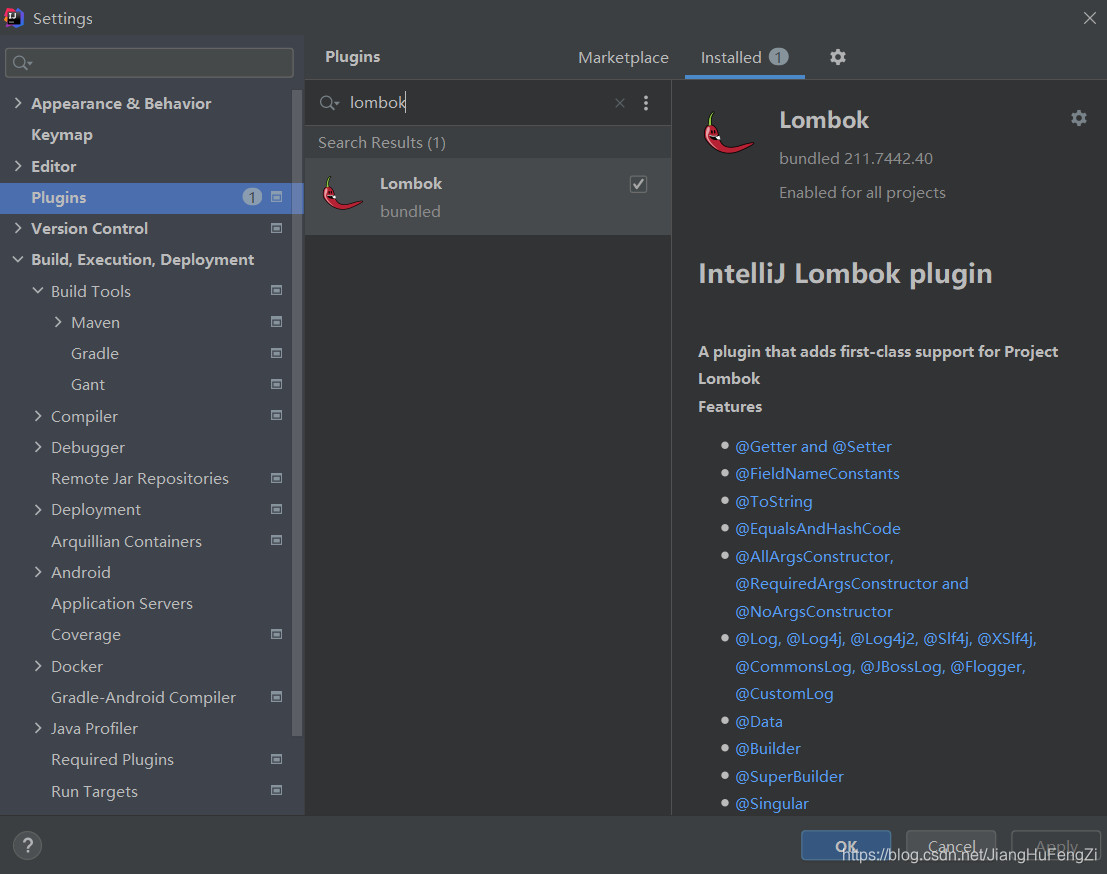 -
在项目中导入lombok的jar包 <dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.18.20</version>
</dependency>
-
注解列表: @Getter and @Setter
@FieldNameConstants
@ToString
@EqualsAndHashCode
@AllArgsConstructor, @RequiredArgsConstructor and @NoArgsConstructor
@Log, @Log4j, @Log4j2, @Slf4j, @XSlf4j, @CommonsLog, @JBossLog, @Flogger, @CustomLog
@Data
@Builder
@SuperBuilder
@Singular
@Delegate
@Value
@Accessors
@Wither
@With
@SneakyThrows
@val
@var
experimental @var
@UtilityClass
Lombok config system
Code inspections
Refactoring actions (lombok and delombok)
-
重点掌握:
-
@Data 他会帮你生成get、set,equals,toString,hashCode等方法!!
-
@AllArgsConstructor 会帮你生成有参构造
-
@NoArgsConstructor 会帮你生成无参构造
基本上一个实体类,用这三个注解就够了,而且用了这个注解依然可以自己在手写定义其它的重载的方法!
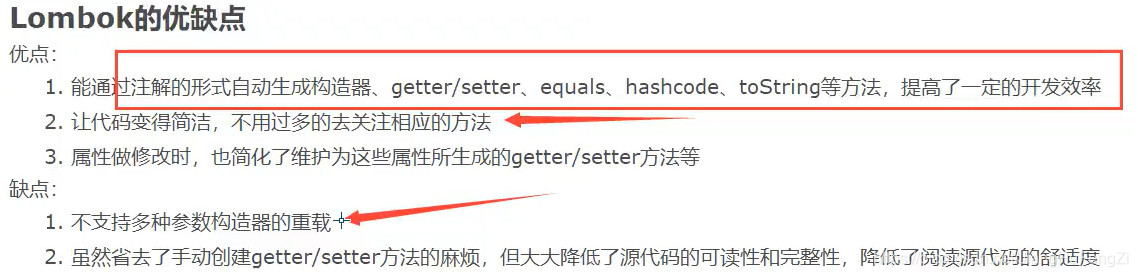
10、多对一处理
多对一:
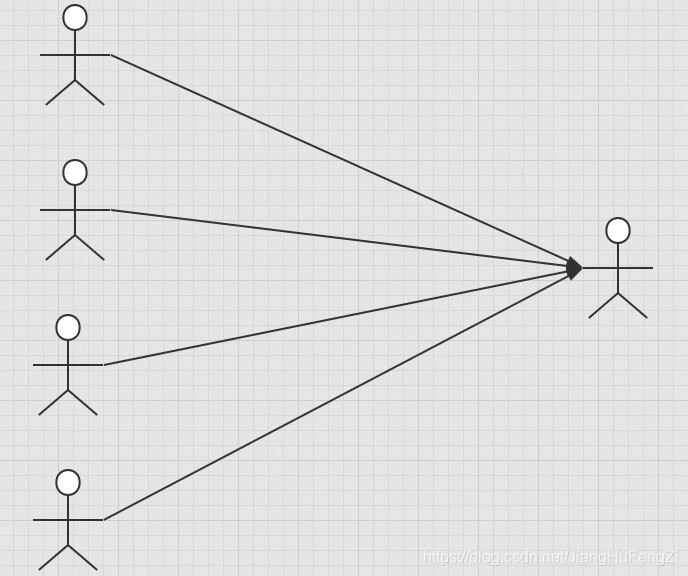
- 多个学生,对应一个老师
- 对于学生这边而言,叫做关联.. 多个学生,关联一个老师【多对一】
- 对于老师而言,集合,一个老师,有很多学生 【一对多】
SQL:
CREATE TABLE `teacher` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
PRIMARY KEY (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8
INSERT INTO teacher(`id`, `name`) VALUES (1, '秦老师');
CREATE TABLE `student` (
`id` INT(10) NOT NULL,
`name` VARCHAR(30) DEFAULT NULL,
`tid` INT(10) DEFAULT NULL,
PRIMARY KEY (`id`),
KEY `fktid` (`tid`),
CONSTRAINT `fktid` FOREIGN KEY (`tid`) REFERENCES `teacher` (`id`)
) ENGINE=INNODB DEFAULT CHARSET=utf8
INSERT INTO `student` (`id`, `name`, `tid`) VALUES (1, '小明', 1);
INSERT INTO `student` (`id`, `name`, `tid`) VALUES (2, '小红', 1);
INSERT INTO `student` (`id`, `name`, `tid`) VALUES (3, '小张', 1);
INSERT INTO `student` (`id`, `name`, `tid`) VALUES (4, '小李', 1);
INSERT INTO `student` (`id`, `name`, `tid`) VALUES (5, '小王', 1);
测试环境搭建
- 导入lombok
- 新建实体类 Teacher Student
- 建立Mapper接口
- 建立Mapper.xml文件
- 在核心配置文件中绑定注册我们的Mapper接口或者文件
- 测试查询是否成功
按照查询嵌套处理
<select id="getStudent" resultMap="studentTeacher">
select * from student
</select>
<resultMap id="studentTeacher" type="Student">
<result property="id" column="id"/>
<result property="name" column="name"/>
<association property="teacher" column="tid" select="getTeachers" javaType="Teacher"/>
</resultMap>
<select id="getTeachers" resultType="Teacher">
select * from teacher where id = #{id}
</select>
测试结果:
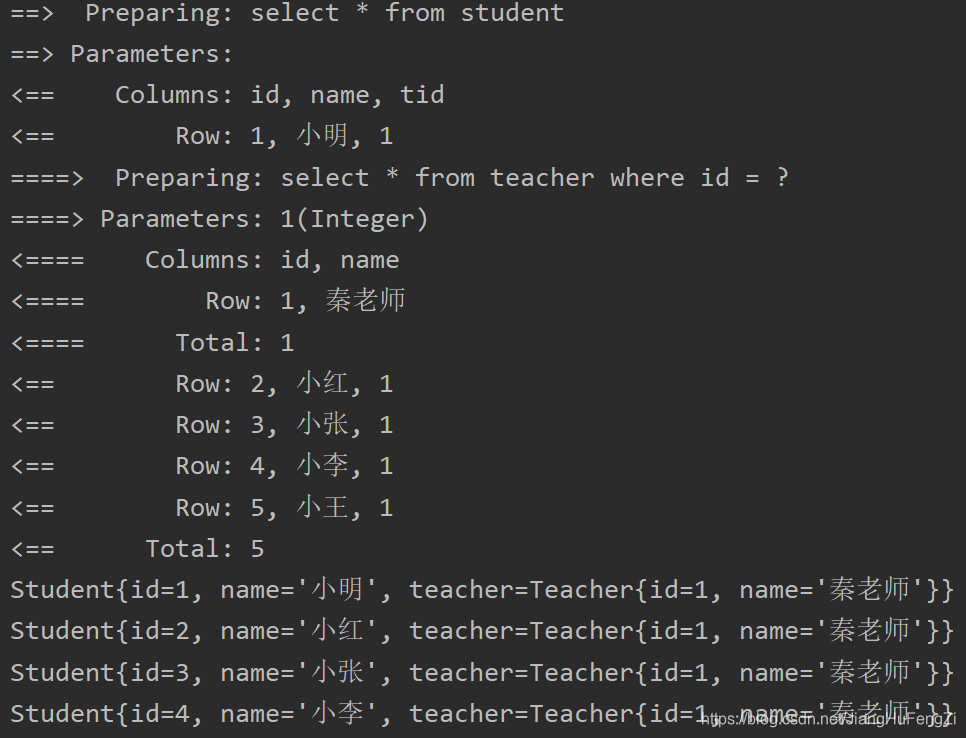
按照结果嵌套处理
<select id="getStudent2" resultMap="getST">
select student.id,student.name,teacher.name as tname from student,teacher where student.tid=teacher.id
</select>
<resultMap id="getST" type="Student">
<result property="id" column="id"/>
<result property="name" column="name"/>
<association property="teacher" javaType="Teacher">
<result property="name" column="tname"/>
</association>
</resultMap>
测试结果
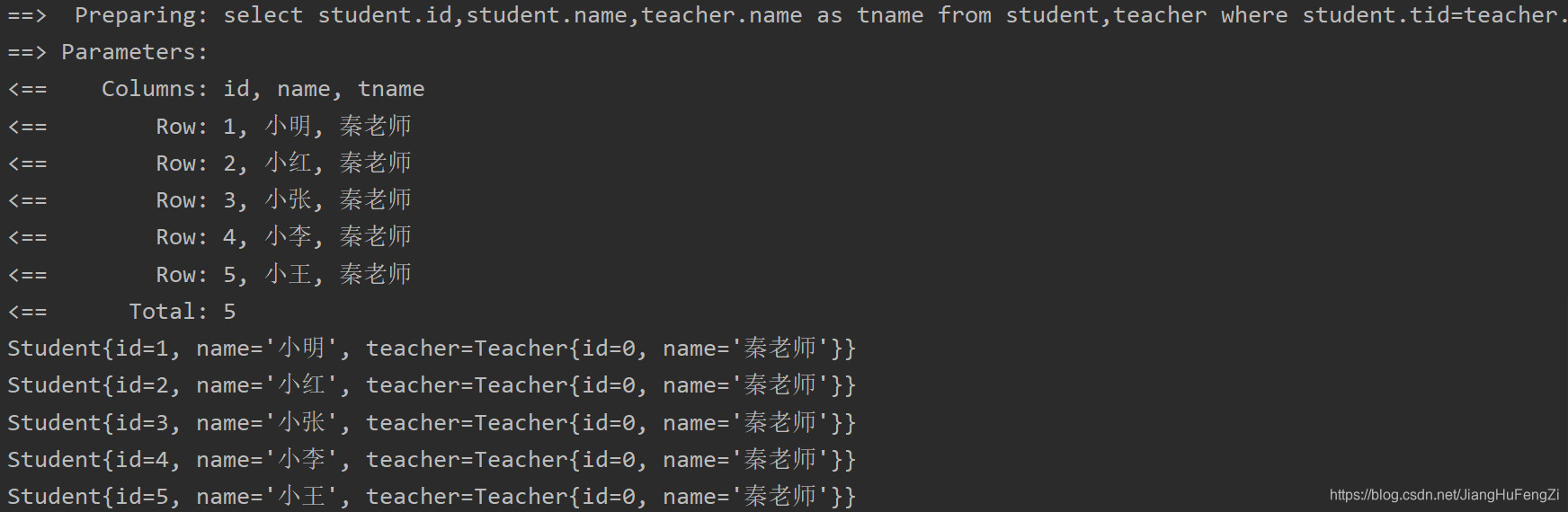
多对一就是:
11、一对多处理
比如:一个老师拥有多个学生!
对于老师而言,就是一对多的关系!
环境搭建
数据库和刚才一样
实体类
public class Student {
private int id;
private String name;
private int tid;
}
public class Teacher {
private int id;
private String name;
private List<Student> students;
}
按照结果嵌套处理
- 尽量使用这种情况,因为这种情况出错还可以调试sql语句
<select id="getTeacher" resultMap="teacher_student" parameterType="_int">
select teacher.id as tid,teacher.name as tname,student.id as sid,student.name as sname
from student ,teacher
where student.tid=teacher.id and teacher.id=#{tid}
</select>
<resultMap id="teacher_student" type="Teacher" >
<result property="id" column="tid"/>
<result property="name" column="tname"/>
<collection property="students" ofType="Student" >
<result property="id" column="sid"/>
<result property="name" column="sname"/>
<result property="tid" column="tid"/>
</collection>
</resultMap>
测试结果:
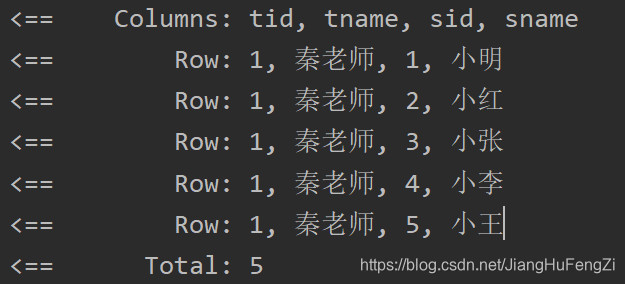
按照查询嵌套处理
<select id="getTeacher2" resultMap="ts" parameterType="_int">
select * from teacher where id = #{teacher_id}
</select>
<resultMap id="ts" type="Teacher">
<result property="id" column="id"/>
<result property="name" column="name"/>
<collection property="students" ofType="Student" javaType="ArrayList" select="getStudent" column="id"/>
</resultMap>
<select id="getStudent" resultType="Student">
select * from student where tid =#{123456}
</select>
测试结果
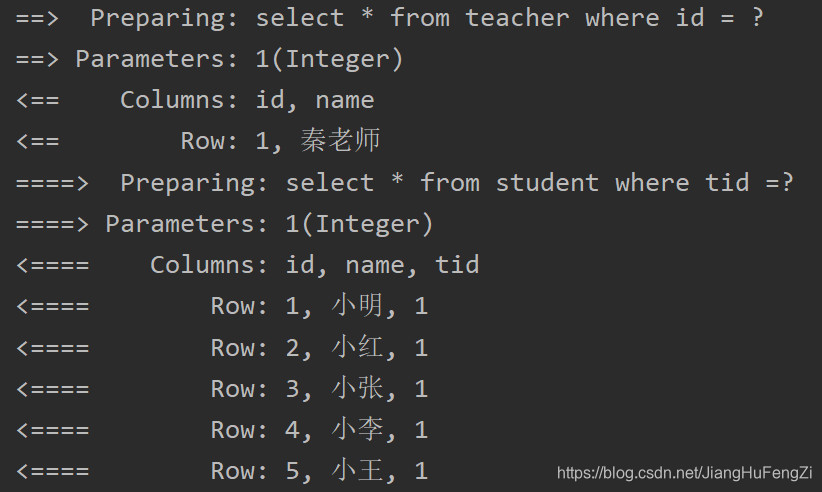
小结
- 关联 - association 【多对一】
- 集合 - collection 【一对多】
- javaType & ofType
- javaType 用来指定实体类中属性的类型
- ofType 用来指定映射到List或者集合中的pojo类型,泛型中的约束类型!
注意点:
- 保证sql的可读性,尽量保证通俗易懂
- 注意一对多和多对一中,属性名的字段的问题!
- 如果问题不好排查错误,可以使用日志,建议使用Log4j
面试高频
- MySQL引擎
- InnoDB的底层原理
- 索引
- 索引优化!
12、动态SQL
- 什么是动态SQL:动态SQL就是指根据不同的条件生成不同的SQL语句
- 利用动态SQL这一特性可以彻底摆脱拼接SQL语句的痛苦
如果你之前用过 JSTL 或任何基于类 XML 语言的文本处理器,你对动态 SQL 元素可能会感觉似曾相识。在 MyBatis 之前的版本中,需要花时间了解大量的元素。借助功能强大的基于 OGNL 的表达式,MyBatis 3 替换了之前的大部分元素,大大精简了元素种类,现在要学习的元素种类比原来的一半还要少。
- if
- choose (when, otherwise)
- trim (where, set)
- foreach
搭建环境
CREATE TABLE `blog`(
`id` VARCHAR(50) NOT NULL COMMENT 博客id,
`title` VARCHAR(100) NOT NULL COMMENT 博客标题,
`author` VARCHAR(30) NOT NULL COMMENT 博客作者,
`create_time` DATETIME NOT NULL COMMENT 创建时间,
`views` INT(30) NOT NULL COMMENT 浏览量
)ENGINE=INNODB DEFAULT CHARSET=utf8
创建一个基础工程
-
导包 -
编写配置文件 -
编写实体类 package com.feng.pojo;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import java.util.Date;
@Data
@AllArgsConstructor
@NoArgsConstructor
public class Blog {
private int id;
private String title;
private String author;
private Date createTime;
private int views;
}
在Mybatis中开启驼峰命名规则: < setting name="mapUnderscoreToCamelCase" value="true"/>
-
编写实体类对应Mapper接口和Mapper.XML文件
IF
<select id="getBlogIf" parameterType="map" resultType="Blog">
select * from blog where 1=1
<if test="title !=null">
and title=#{title}
</if>
<if test="author !=null">
and author=#{author}
</if>
</select>
修改上面的SQL语句
<select id="getBlogIf" parameterType="map" resultType="Blog">
select * from blog
<where>
<if test="title !=null">
title=#{title}
</if>
<if test="author !=null">
and author=#{author}
</if>
</where>
</select>
测试类
@Test
public void textIf(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
Map map = new HashMap<>();
map.put("author","小明");
List<Blog> blogIf = mapper.getBlogIf(map);
for (Blog blog : blogIf) {
System.out.println(blog);
}
sqlSession.close();
}
choose (when, otherwise)
- choose中只会有一个语句的到满足,即选择一个sql语句拼接,而if是判断拼接的条件
<select id="getBlogChoose" parameterType="map" resultType="Blog">
select * from blog
<where>
<choose>
<when test="title!=null">
title=#{title}
</when>
<when test="author!=null">
and author=#{author}
</when>
<otherwise>
and views=#{views}
</otherwise>
</choose>
</where>
</select>
测试
@Test
public void textChoose(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
Map map = new HashMap<>();
map.put("title","编程改变人生");
map.put("author","小明");
map.put("views",999);
List<Blog> blogIf = mapper.getBlogChoose(map);
for (Blog blog : blogIf) {
System.out.println(blog);
}
sqlSession.close();
}
trim (where, set)
where:
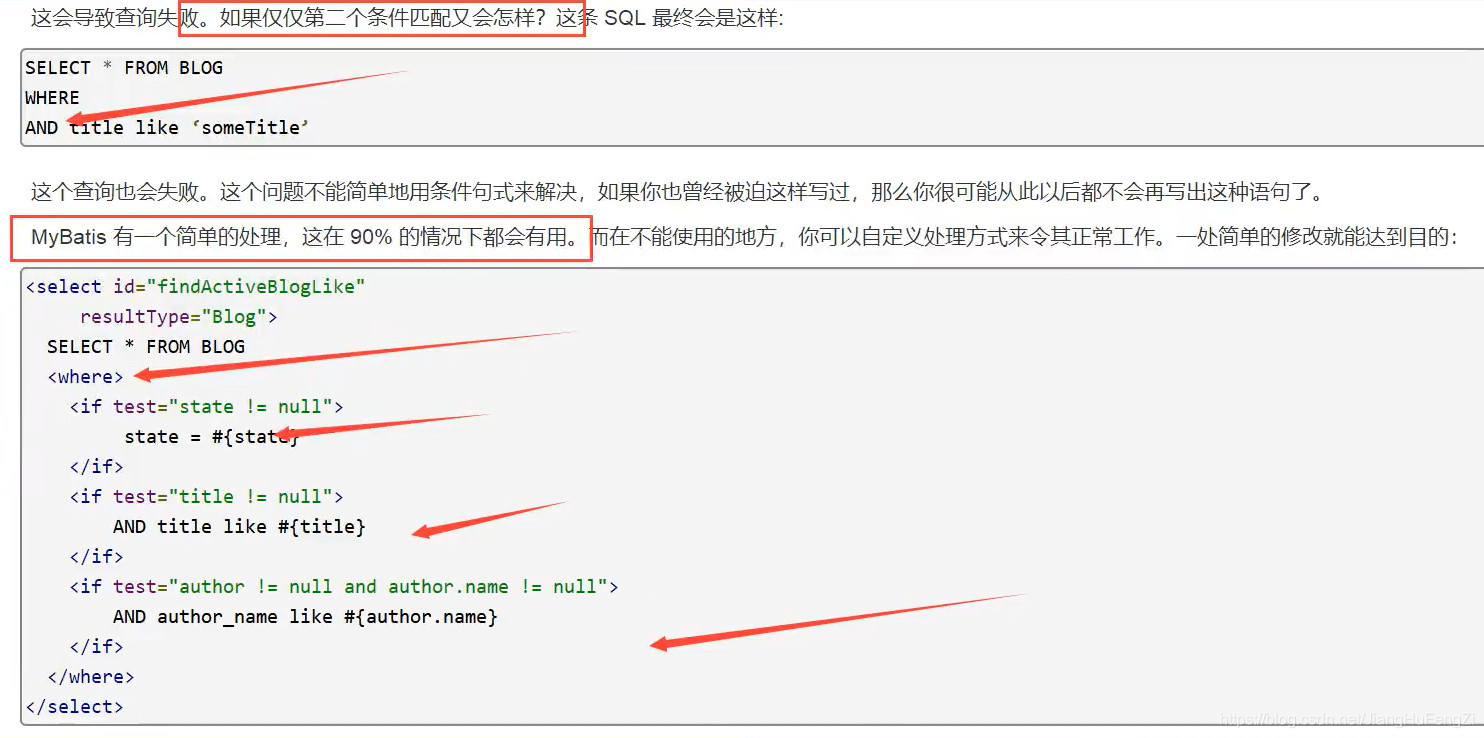
<select id="getBlogIf" parameterType="map" resultType="Blog">
select * from blog
<where>
<if test="title !=null">
title=#{title}
</if>
<if test="author !=null">
and author=#{author}
</if>
</where>
</select>
set:
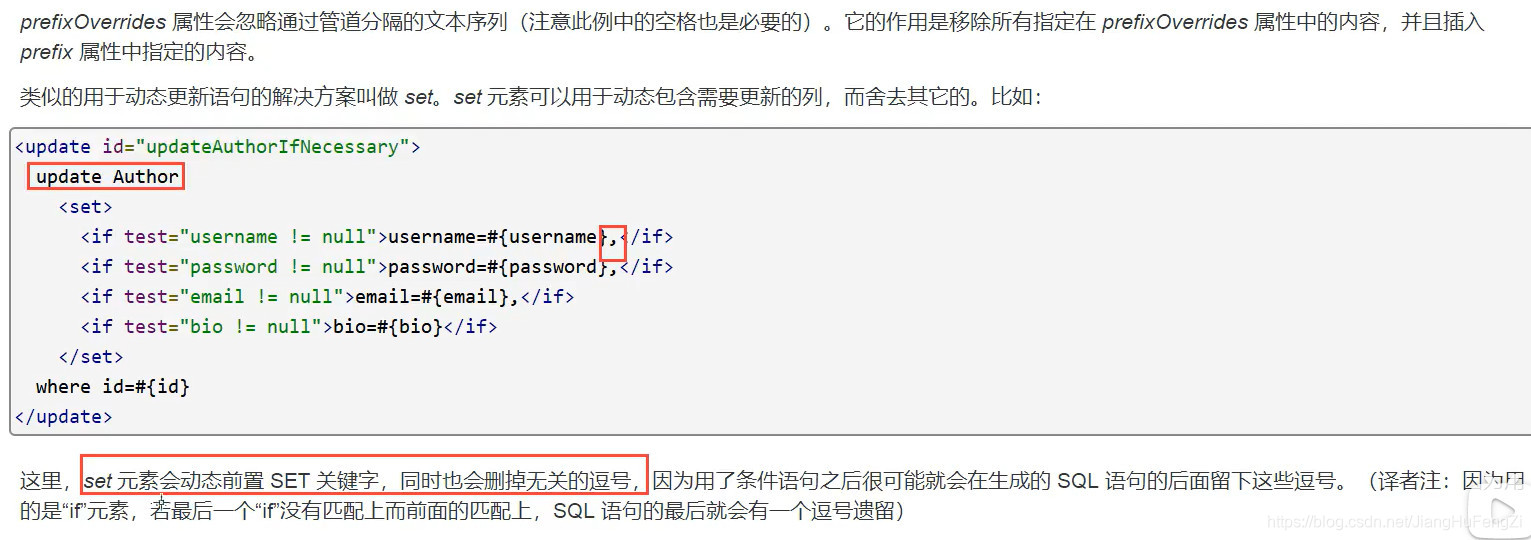
<update id="updateBlog" parameterType="map" >
update blog
<set>
<if test="title !=null">
title = #{title},
</if>
<if test="author">
author =#{author}
</if>
</set>
where id =#{id}
</update>
@Test
public void textset(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
Map map = new HashMap<>();
map.put("title","编程改变人生2");
map.put("id","92ed752144e7491cacfa7e29fae4fc64");
mapper.updateBlog(map);
sqlSession.close();
}

这里它自己去除了逗号。
所谓的动态SQL,本质还是SQL语句,只是我们可以在SQL层面,去执行逻辑代码
SQL片段
有的时候,我们可能会将一些功能的部分抽取出来,方便复用!
-
使用SQL标签抽取公共的部分 <sql id="if-title">
<if test="title !=null">
title=#{title}
</if>
<if test="author !=null">
and author=#{author}
</if>
</sql>
-
在需要使用的地方使用Include标签引用即可 <select id="getBlogIf" parameterType="map" resultType="Blog">
select * from blog
<where>
<include refid="if-title"></include>
</where>
</select>
注意事项:
- 最好基于单表来定义SQL标签
- 不要存在where标签
- 一般只用一些if语句在里面
Foreach
select * from user where 1=1 and ( id=1 or id=2 or id=3 )
<foreach item="item" index="index" collection="list"
open="(" separator="," close=")">
</foreach>
<foreach item="id" collection="ids"
open="(" separator="or" close=")">
</foreach>
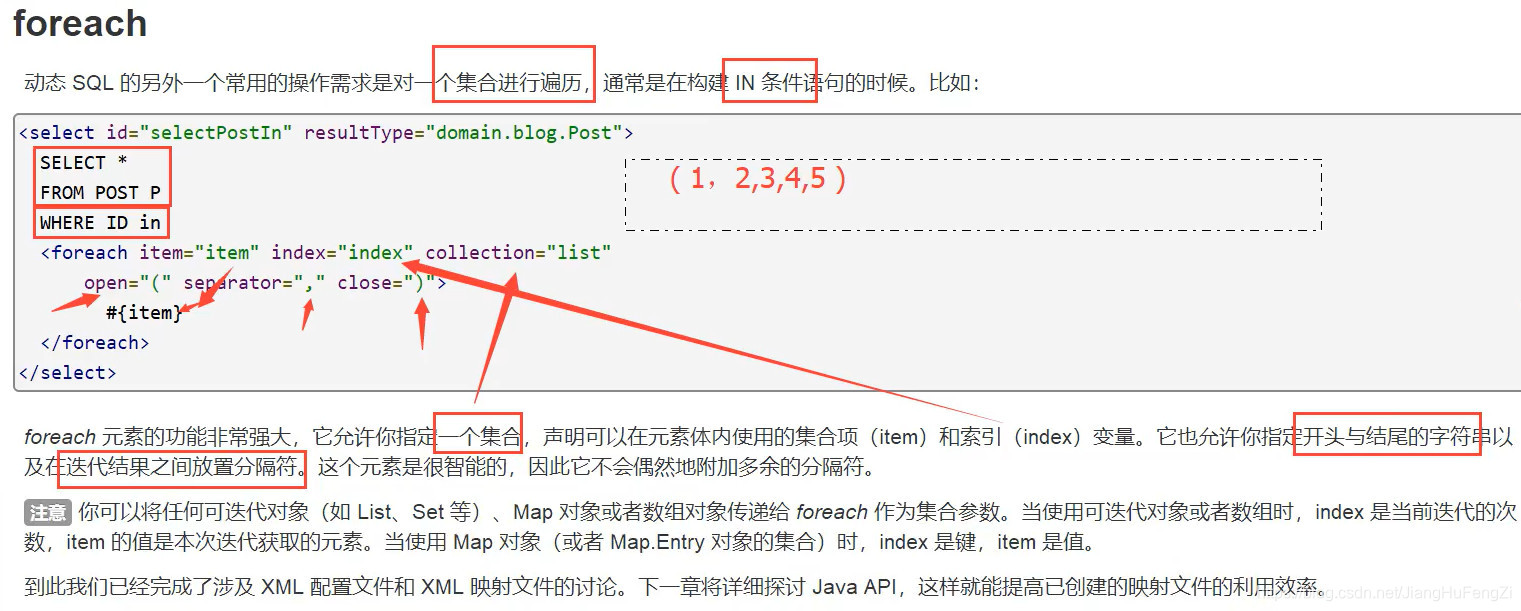
<select id="getBlog" parameterType="map" resultType="Blog">
select * from blog
<where>
<foreach collection="ids" item="id" open="(" close=")" separator="or">
id =#{id}
</foreach>
</where>
</select>
测试
@Test
public void getBlog(){
SqlSession sqlSession = MybatisUtils.getSqlSession();
BlogMapper mapper = sqlSession.getMapper(BlogMapper.class);
Map map = new HashMap<>();
ArrayList list = new ArrayList<>();
map.put("ids",list);
List<Blog> blog = mapper.getBlog(map);
for (Blog blog1 : blog) {
System.out.println(blog1);
}
sqlSession.close();
}
动态SQL就是在拼接SQL语句,我们只要保证SQL的正确性,按照SQL的格式,去排列组合就可以了
建议:
- 先在Mysql中写出完整的SQL语句在对应的去修改成为我们的动态SQL语句即可!
|