SpringBoot
加油兄弟们!!!!!!今天也要继续努力!
每日格言:
锲而舍之,朽木不折;锲而不舍,金石可镂。
run执行原理
run
开启服务
package com.hyc.spring01helloworld;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class Spring01HelloworldApplication {
public static void main(String[] args) {
SpringApplication.run(Spring01HelloworldApplication.class, args);
}
}
SpringBootApplication.run分析
主要是两个部分,一个是SpringBootApplication实例化,一个是run的执行
SpringBootApplication
执行的步骤:
- 推断应用类型是普通的java项目还是web项目
- 查找并加载所有可用的初始化器,设置到initializers实行中
- 找出所有的应用程序监听器,设置到listeners属性中
- 推断并且设置main方法定义类,找到运行的主类
查看构造器
public SpringApplication(ResourceLoader resourceLoader, Class<?>... primarySources) {
this.sources = new LinkedHashSet();
this.bannerMode = Mode.CONSOLE;
this.logStartupInfo = true;
this.addCommandLineProperties = true;
this.addConversionService = true;
this.headless = true;
this.registerShutdownHook = true;
this.additionalProfiles = Collections.emptySet();
this.isCustomEnvironment = false;
this.lazyInitialization = false;
this.applicationContextFactory = ApplicationContextFactory.DEFAULT;
this.applicationStartup = ApplicationStartup.DEFAULT;
this.resourceLoader = resourceLoader;
Assert.notNull(primarySources, "PrimarySources must not be null");
this.primarySources = new LinkedHashSet(Arrays.asList(primarySources));
this.webApplicationType = WebApplicationType.deduceFromClasspath();
this.bootstrapRegistryInitializers = this.getBootstrapRegistryInitializersFromSpringFactories();
this.setInitializers(this.getSpringFactoriesInstances(ApplicationContextInitializer.class));
this.setListeners(this.getSpringFactoriesInstances(ApplicationListener.class));
this.mainApplicationClass = this.deduceMainApplicationClass();
}
SpringBoot配置
- 官方配置太多,
- 如何配置tml
- 学习原理,一通百通
官方配置链接:https://docs.spring.io/spring-boot/docs/current/reference/html/application-properties.html#application-properties
yml是什么?
YAML是"YAML Ain’t a Markup Language"(YAML不是一种标记语言)的递归缩写。在开发的这种语言时,YAML 的意思其实是:“Yet Another Markup Language”(仍是一种标记语言),但为了强调这种语言以数据做为中心,而不是以标记语言为重点,而用反向缩略语重命名。
标记语言:
以前的配置文件,大多数都是用xml来配置,比如一个简单的端口配置,我们来对比下yaml和xml的区别
yml:
server:
port: 8080
xml:
<server>
<port>8080</port>
</server>
yml:特点
- K:V
- 对空格的要求十分高
- 普通的key-value
- 可以注入到我们的配置类中
两种赋值方式
1.注解赋值
@Component
public class Dog {
@Value("郑文杰")
private String name;
@Value("3")
private Integer age;
2.YML注入
实体类:
@Component
@ConfigurationProperties(prefix = "person")
public class person {
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
YML:
person:
name: hyc
age : 3
happy: false
birth: 2019/11/02
maps: {k1: 123, k2: 456}
lists:
-code
-music
-girl
dog:
name: 旺财
age: 3
@ConfigurationProperties()作用:
将配置文件中每个属性的值,映射这个组件中;
告诉SpringBoot将奔雷所有的属性和配置文件中相关的配置进行绑定
参数perfix = “person” 将person组件(类)和yml中person的值对应绑定
@PropertySource
指定配置文件:properties文件
@PropertySource(value = "classpath:application.properties")
用@value 赋值
SpringBoot推荐我们用yml
对比:
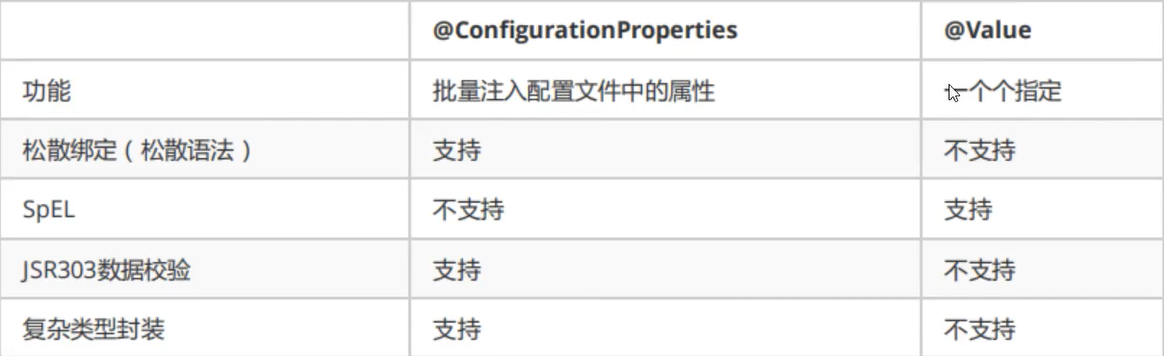
应为yml十分灵活:
person:
name: hyc
age : ${random.int}
happy: false
birth: 2019/11/02
maps: {k1: 123, k2: 456}
lists:
-code
-music
-girl
dog:
name: 旺财
age: 3
java类
package com.hyc.pojo;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.context.annotation.PropertySource;
import org.springframework.stereotype.Component;
import org.springframework.validation.annotation.Validated;
import javax.validation.constraints.Email;
import java.util.Date;
import java.util.List;
import java.util.Map;
@Component
@ConfigurationProperties(prefix = "person")
@Validated //数据校验
public class person {
@Email(message = "邮箱格式错误")
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
@Override
public String toString() {
return "person{" +
"name='" + name + '\'' +
", age=" + age +
", happy=" + happy +
", birth=" + birth +
", maps=" + maps +
", lists=" + lists +
", dog=" + dog +
'}';
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public Boolean getHappy() {
return happy;
}
public void setHappy(Boolean happy) {
this.happy = happy;
}
public Date getBirth() {
return birth;
}
public void setBirth(Date birth) {
this.birth = birth;
}
public Map<String, Object> getMaps() {
return maps;
}
public void setMaps(Map<String, Object> maps) {
this.maps = maps;
}
public List<Object> getLists() {
return lists;
}
public void setLists(List<Object> lists) {
this.lists = lists;
}
public Dog getDog() {
return dog;
}
public void setDog(Dog dog) {
this.dog = dog;
}
}
jsr303校验注解:
@Validated
校验示例:
java类:
@Component
@ConfigurationProperties(prefix = "person")
@Validated //数据校验
public class person {
@Email(message = "邮箱格式错误")
private String name;
private Integer age;
private Boolean happy;
private Date birth;
private Map<String,Object> maps;
private List<Object> lists;
private Dog dog;
YML:此时我们的name并不是邮箱格式
person:
name: hyc
age : ${random.int}
happy: false
birth: 2019/11/02
maps: {k1: 123, k2: 456}
lists:
-code
-music
-girl
dog:
name: 旺财
age: 3
执行效果:

使用了@Validated //数据校验注解的类可以对自己的属性设置格式数据校验
全部注解:
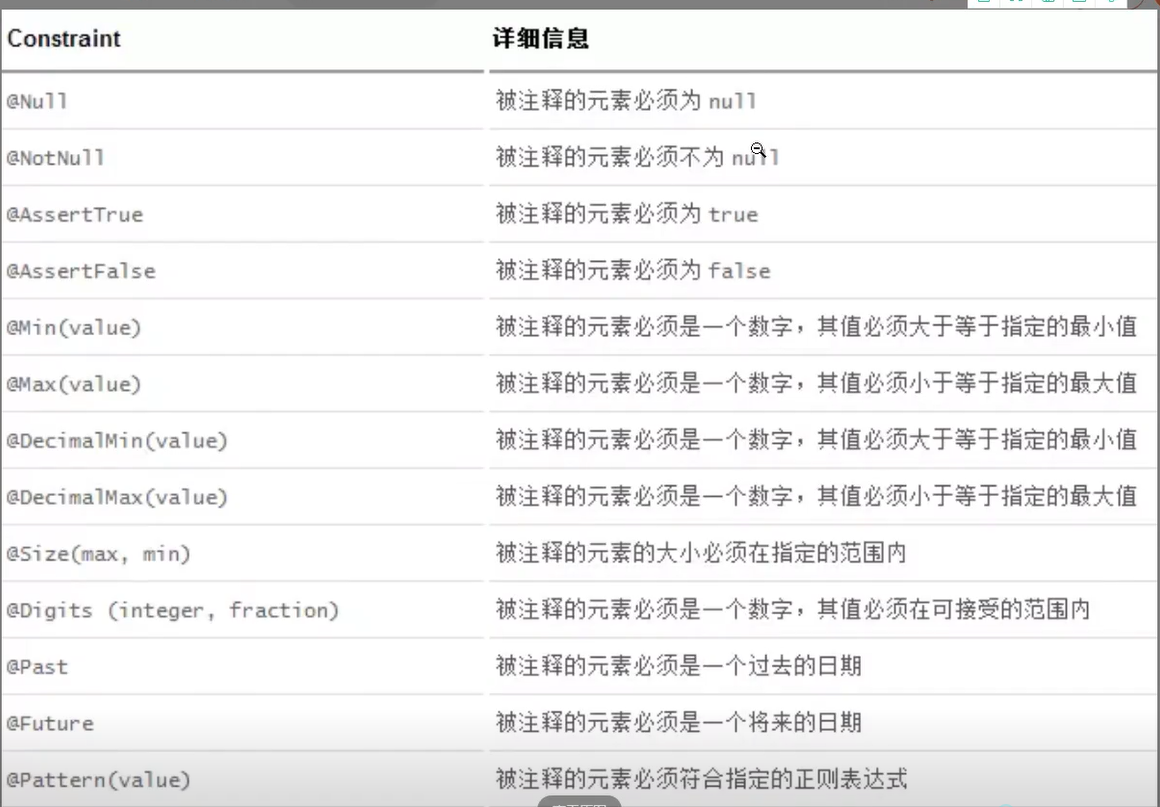
常用注解:
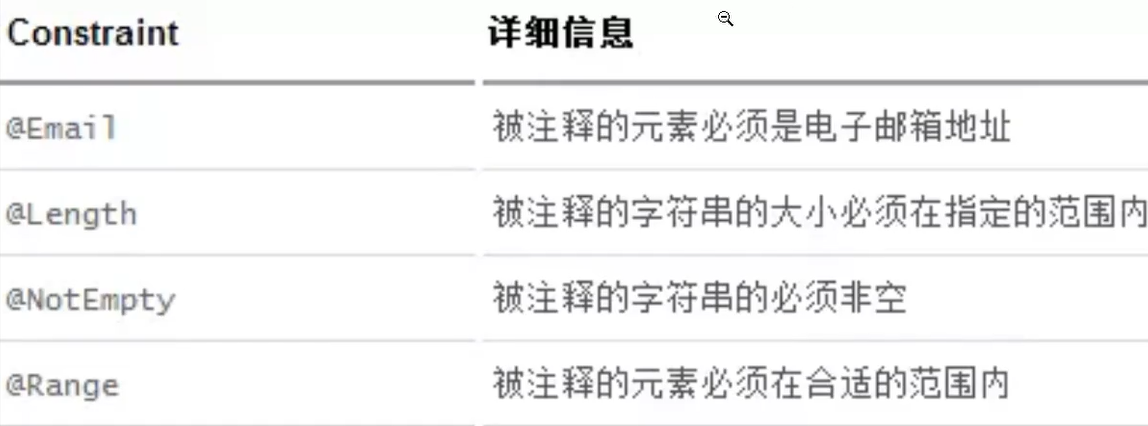
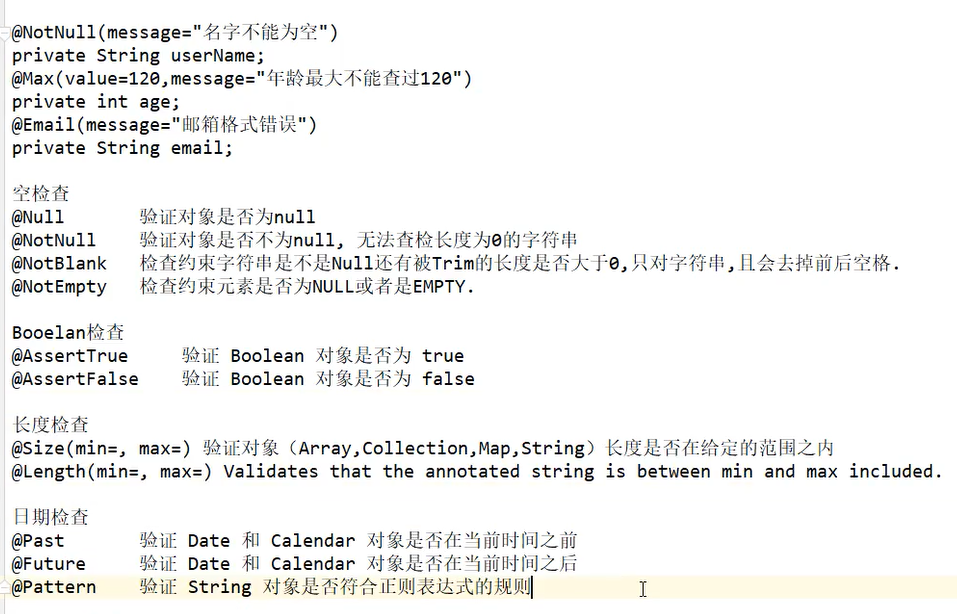
正则表达式:相对核心
源码位置:
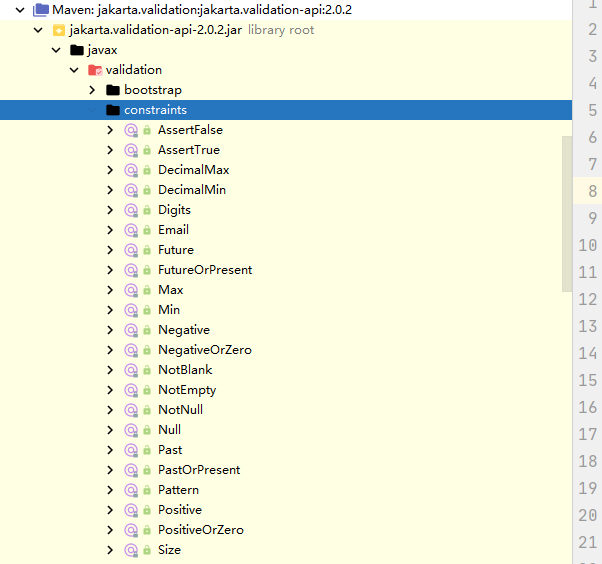
小结:
学会找到源码位置,尝试自己阅读源码,探索别人写的巧妙之处,来提升自己
|