1 整合redis
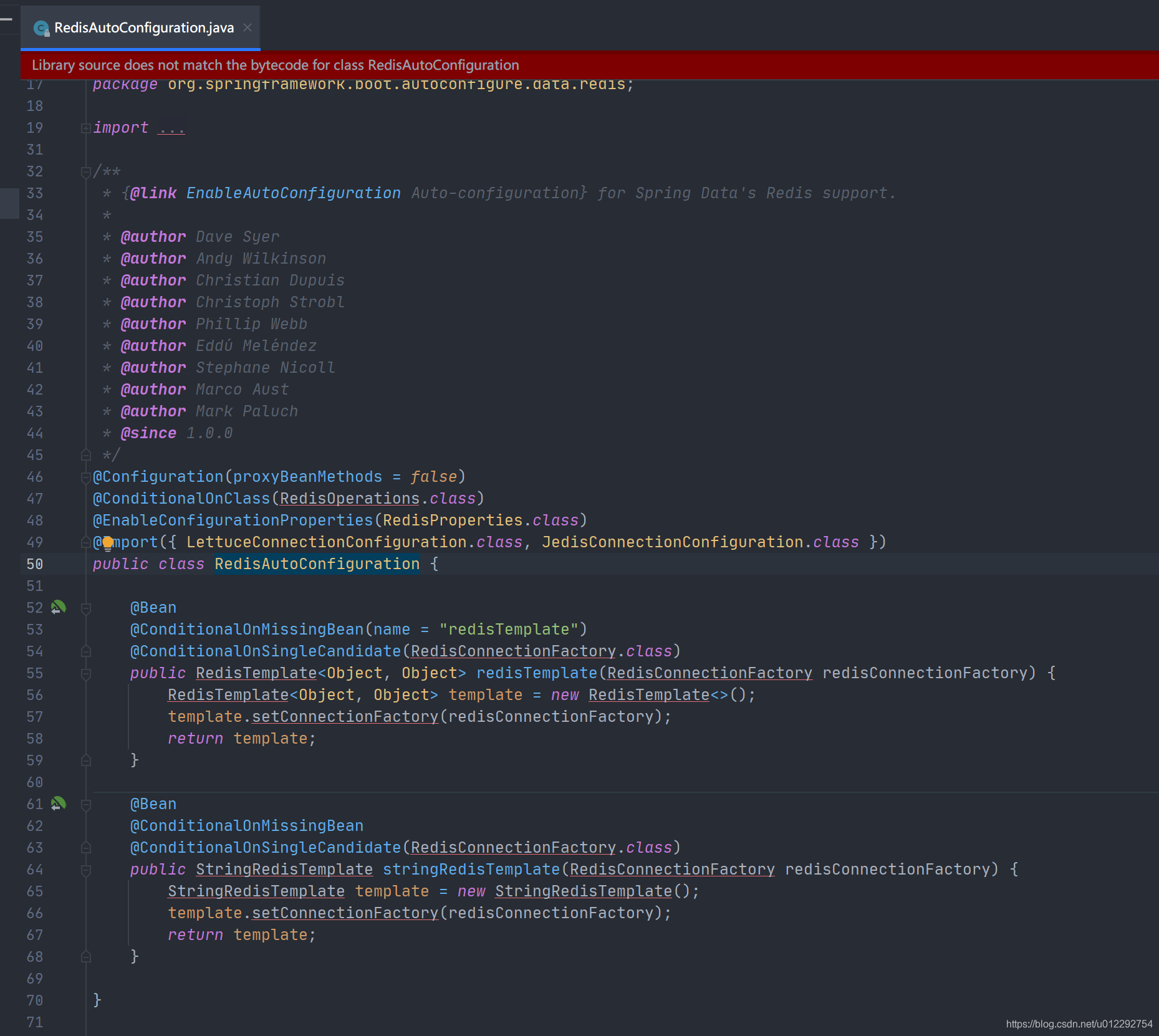 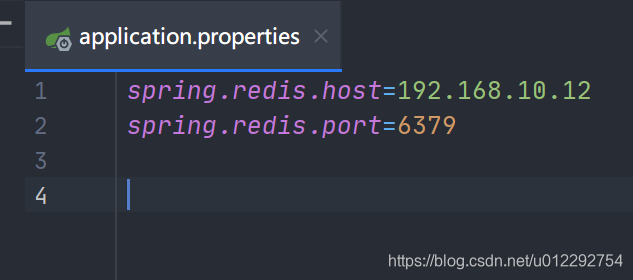 
package com.tzb.cachespringboot;
import com.tzb.cachespringboot.model.User;
import org.junit.jupiter.api.Test;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.data.redis.core.RedisTemplate;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
@SpringBootTest
class CacheSpringbootApplicationTests {
@Autowired
RedisTemplate redisTemplate;
@Autowired
StringRedisTemplate stringRedisTemplate;
@Test
void contextLoads() {
User user = new User();
user.setUsername("Mike");
user.setAddr("Beijing");
ValueOperations ops = redisTemplate.opsForValue();
ops.set("u",user);
User u = (User) ops.get("u");
System.out.println("u = " + u);
}
@Test
void test() {
ValueOperations<String, String> ops = stringRedisTemplate.opsForValue();
ops.set("age","25");
String age = ops.get("age");
System.out.println("age = " + age);
}
}
 
1.1 Session共享
参考
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-redis</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.session</groupId>
<artifactId>spring-session-data-redis</artifactId>
</dependency>
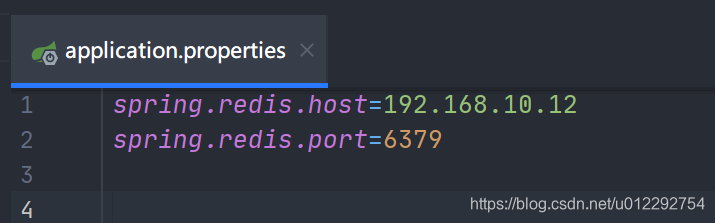
@RestController
public class HelloController {
@Value("${server.port}")
Integer port;
@GetMapping("/set")
public String set(HttpSession session){
session.setAttribute("Mike", "UK");
return String.valueOf(port);
}
@GetMapping("/get")
public String get(HttpSession session){
String s = (String)session.getAttribute("Mike");
return s +" : "+ port;
}
}
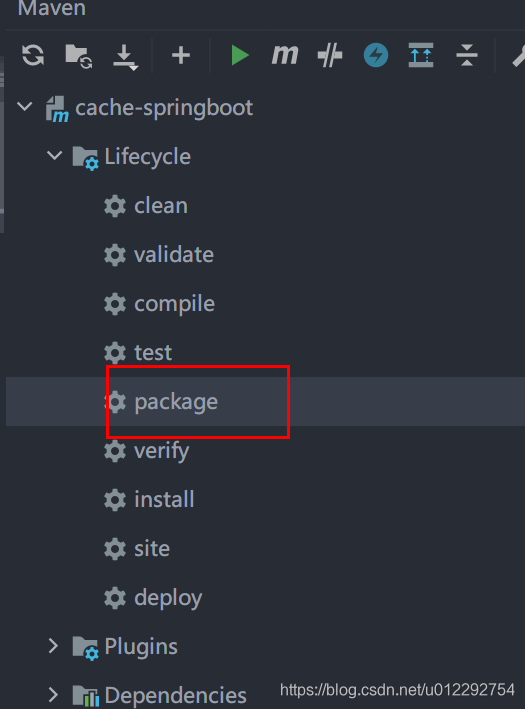 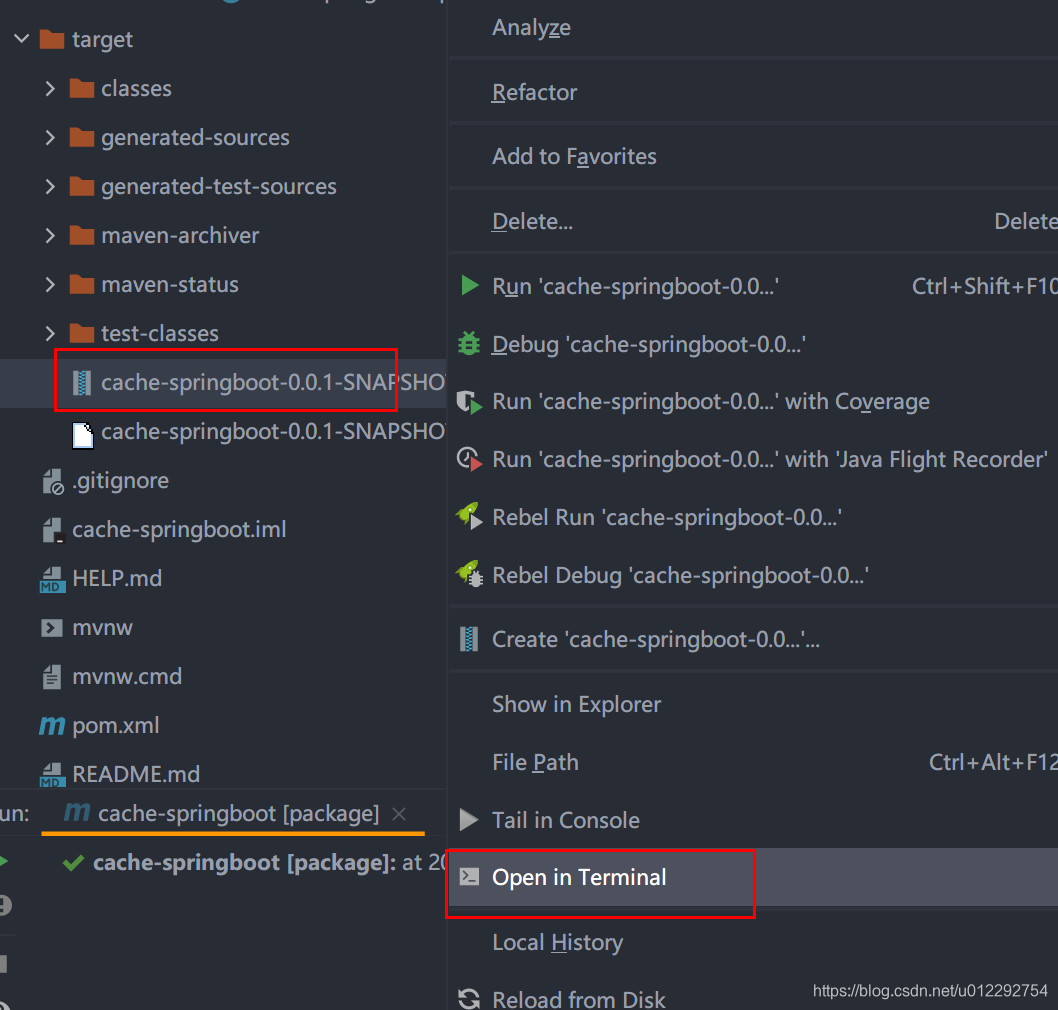  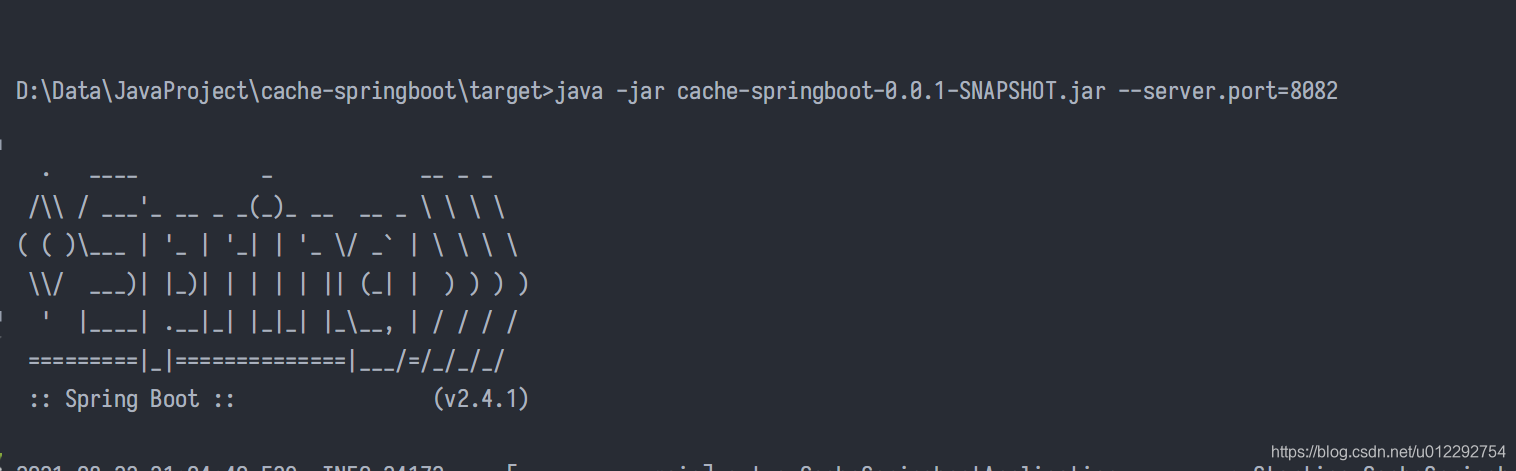
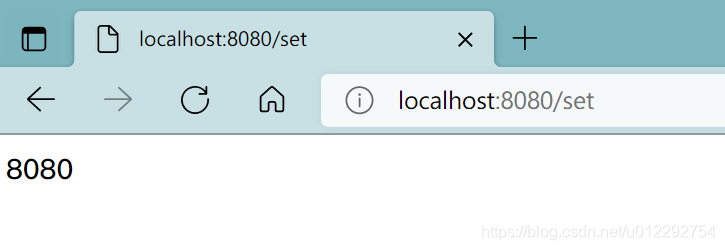 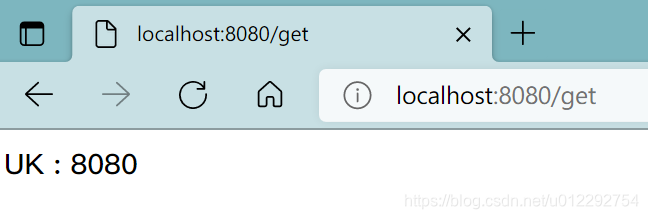 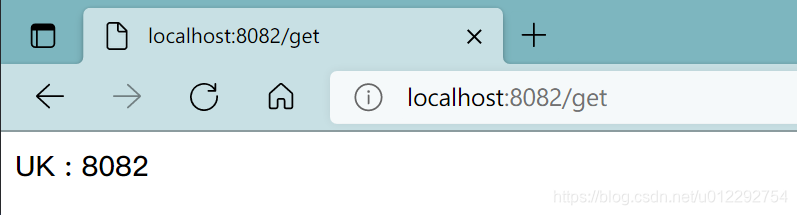
1.2 redis处理接口幂等性
package com.tzb.cachespringboot.token;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.data.redis.core.StringRedisTemplate;
import org.springframework.data.redis.core.ValueOperations;
import org.springframework.stereotype.Service;
import java.util.concurrent.TimeUnit;
@Service
public class RedisService {
@Autowired
StringRedisTemplate stringRedisTemplate;
public boolean setExpire(String key,String value,Long expireTime){
boolean result = false;
try {
ValueOperations<String, String> ops = stringRedisTemplate.opsForValue();
ops.set(key,value);
stringRedisTemplate.expire(key, expireTime, TimeUnit.SECONDS);
result = true;
} catch (Exception e) {
e.printStackTrace();
}
return result;
}
public boolean exists(String key){
return stringRedisTemplate.hasKey(key);
}
public boolean remove(String key){
if(exists(key)){
return stringRedisTemplate.delete(key);
}
return false;
}
}
@Service
public class TokenService {
@Autowired
RedisService redisService;
public String createToken(){
String uuid = UUID.randomUUID().toString();
redisService.setExpire(uuid, uuid, 10000L);
return uuid;
}
public boolean checkToken(HttpServletRequest request) throws Exception {
String token = request.getHeader("token");
if(StringUtils.isEmpty(token)){
token = request.getParameter("token");
if(StringUtils.isEmpty(token)){
throw new Exception("token不存在");
}
}
if(!redisService.exists(token)){
throw new Exception("token不存在");
}
boolean remove = redisService.remove(token);
if(!remove){
throw new Exception("token不存在");
}
return true;
}
}
注解的解析方式:
- 通过拦截器解析
- 通过aop
1.2.1 拦截器处理
@Component
public class IdempotentInterceptor implements HandlerInterceptor {
@Autowired
TokenService tokenService;
@Override
public boolean preHandle(HttpServletRequest request, HttpServletResponse response, Object handler) throws Exception {
if (!(handler instanceof HandlerMethod)) {
return true;
}
Method method = ((HandlerMethod) handler).getMethod();
AutoIdempotent idempotent = method.getAnnotation(AutoIdempotent.class);
if (idempotent != null) {
try {
return tokenService.checkToken(request);
} catch (Exception e) {
throw e;
}
}
return true;
}
}
@Configuration
public class WebMvcConfig implements WebMvcConfigurer {
@Autowired
IdempotentInterceptor idempotentInterceptor;
@Override
public void addInterceptors(InterceptorRegistry registry) {
registry.addInterceptor(idempotentInterceptor).addPathPatterns("/**");
}
}
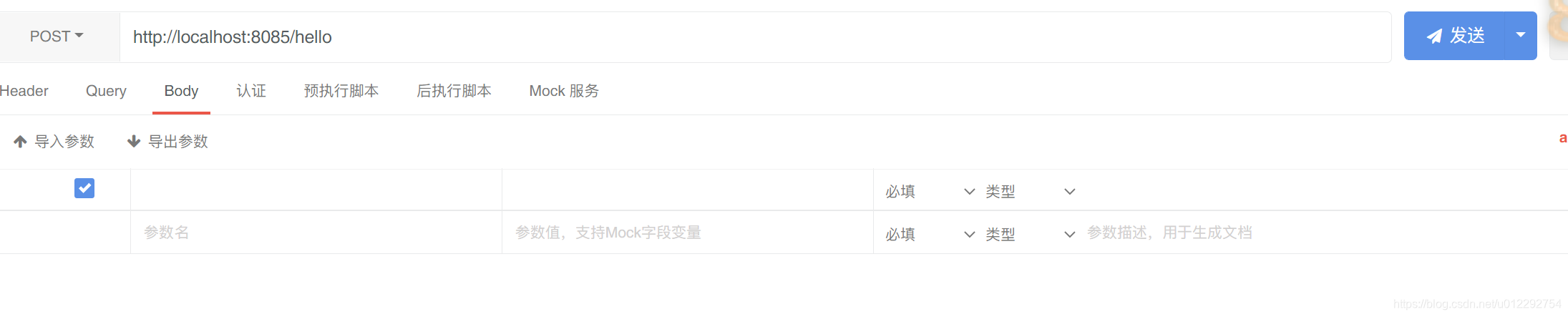 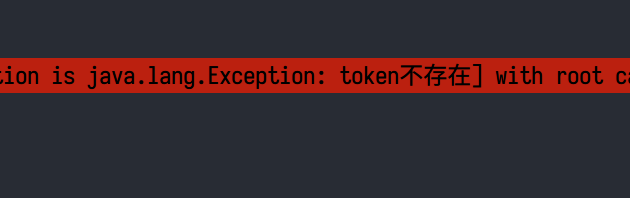 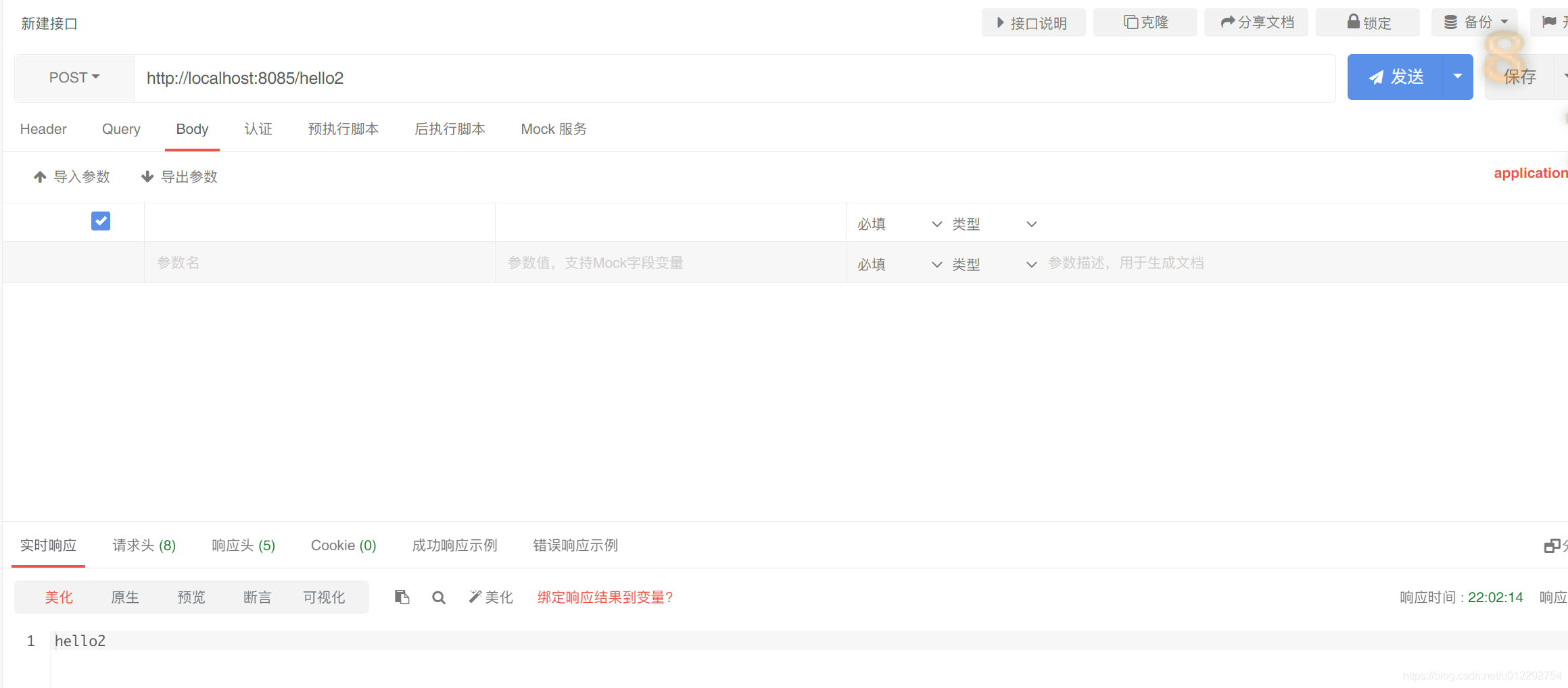 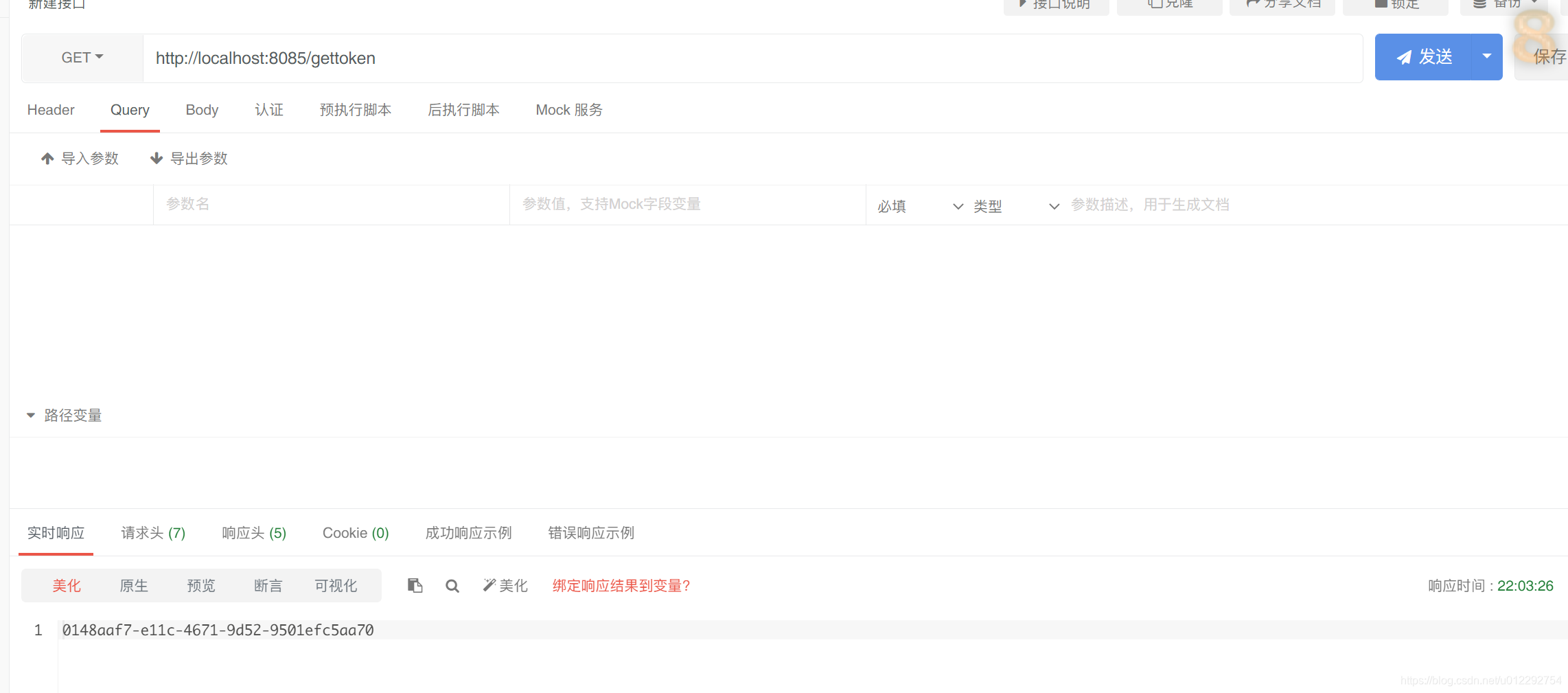 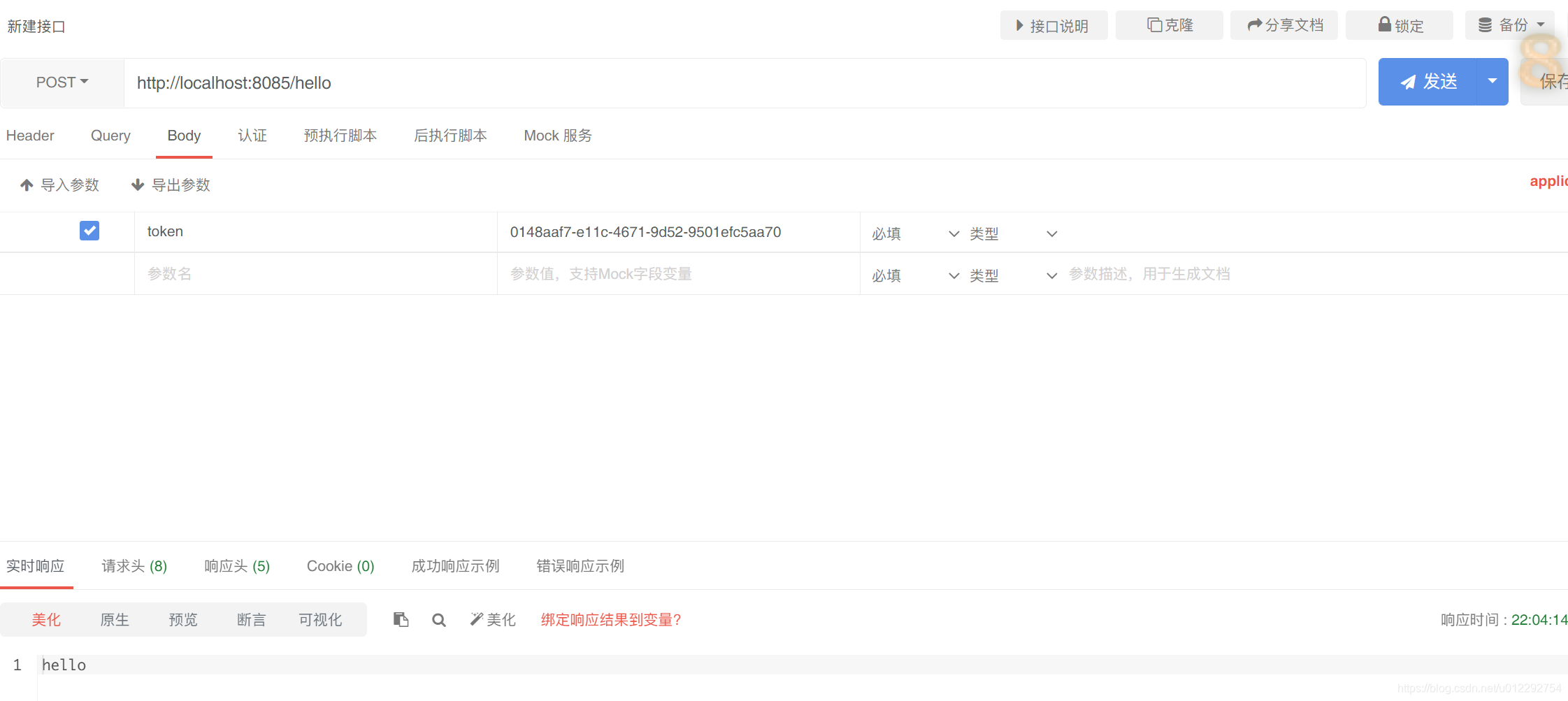
1.2.2 aop处理
import com.tzb.cachespringboot.token.TokenService;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.aspectj.lang.annotation.Pointcut;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Component;
import org.springframework.web.context.request.RequestContextHolder;
import org.springframework.web.context.request.ServletRequestAttributes;
import javax.servlet.http.HttpServletRequest;
@Component
@Aspect
public class IdempotentAspect {
@Autowired
TokenService tokenService;
@Pointcut("@annotation(com.tzb.cachespringboot.anno.AutoIdempotent)")
public void pc1(){
}
@Before("pc1()")
public void before() throws Exception {
HttpServletRequest request = ((ServletRequestAttributes) RequestContextHolder.getRequestAttributes()).getRequest();
try {
tokenService.checkToken(request);
} catch (Exception e) {
throw e;
}
}
}
|