BeanFactory是SpringBean容器的根接口,定义了Bean工厂的最基本的特性,生成的类都是有此接口的实现类来管理的。
FactoryBean 的本质也是一个Bean,用于生成普通的Bean,初始化时,会把这个接口的Bean给取出来,通过其中getObject() 方法来生成我们想要的bean。
在spring的xml配置文件中可以通过以下方式来创建实例:
public class User {}
public class UserFactory {
public User getUser() {
return new User();
}
}
<bean id="userFactory" class="com.demo.entity.UserFactory"/>
<bean id="user" factory-bean="userFactory" factory-method="getUser" scope="singleton"/>
此种创建bean实例的方式并不是真正通过FactoryBean来创建的,因为这里是通过userFactory实例(factory-bean)中的getUser方法(factory-method) 来创建出来的user实例。
通过实现FactotyBean接口来创建User实例:
public class UserFactoryBean implements FactoryBean<User> {
@Override
public User getObject() throws Exception {
return new User();
}
@Override
public Class<User> getObjectType() {
return User.class;
}
}
载入IOC容器:
<bean id="userFactoryBean" class="com.demo.entity.UserFactoryBean"/>
调用测试,发现是能够通过FactoryBean来创建Bean的
public static void test1() {
String xmlPath = "classpath:spring/spring-beans.xml";
ApplicationContext context = new FileSystemXmlApplicationContext(xmlPath);
User user = (User) context.getBean("userFactoryBean");
System.out.printf("factoryBean创建的实例:%s",user);
}
如果要获取FactoryBean,而不是实现的getObject() 方法所返回的实例,那么就需要使用BeanFactory接口中的:
public interface BeanFactory {
String FACTORY_BEAN_PREFIX = "&";
}
在 FactoryBean 的实现类名前加个&作用符,用于获取factoryBean而不是获取其中getObject() 创建的bean。
public static void test1() {
String xmlPath = "classpath:spring/spring-beans.xml";
ApplicationContext context = new FileSystemXmlApplicationContext(xmlPath);
UserFactoryBean userFactoryBean = (UserFactoryBean) context.getBean("&userFactoryBean");
System.out.printf("factoryBean===> %s", userFactoryBean);
}
Spring的设计主要分为两个路线:
- 以BeanFactory接口为主的简单容器
- 以ApplicationContext接口为主的高级核心容器,也就是我们广泛使用的容器
接口的定义均符合单一职责。 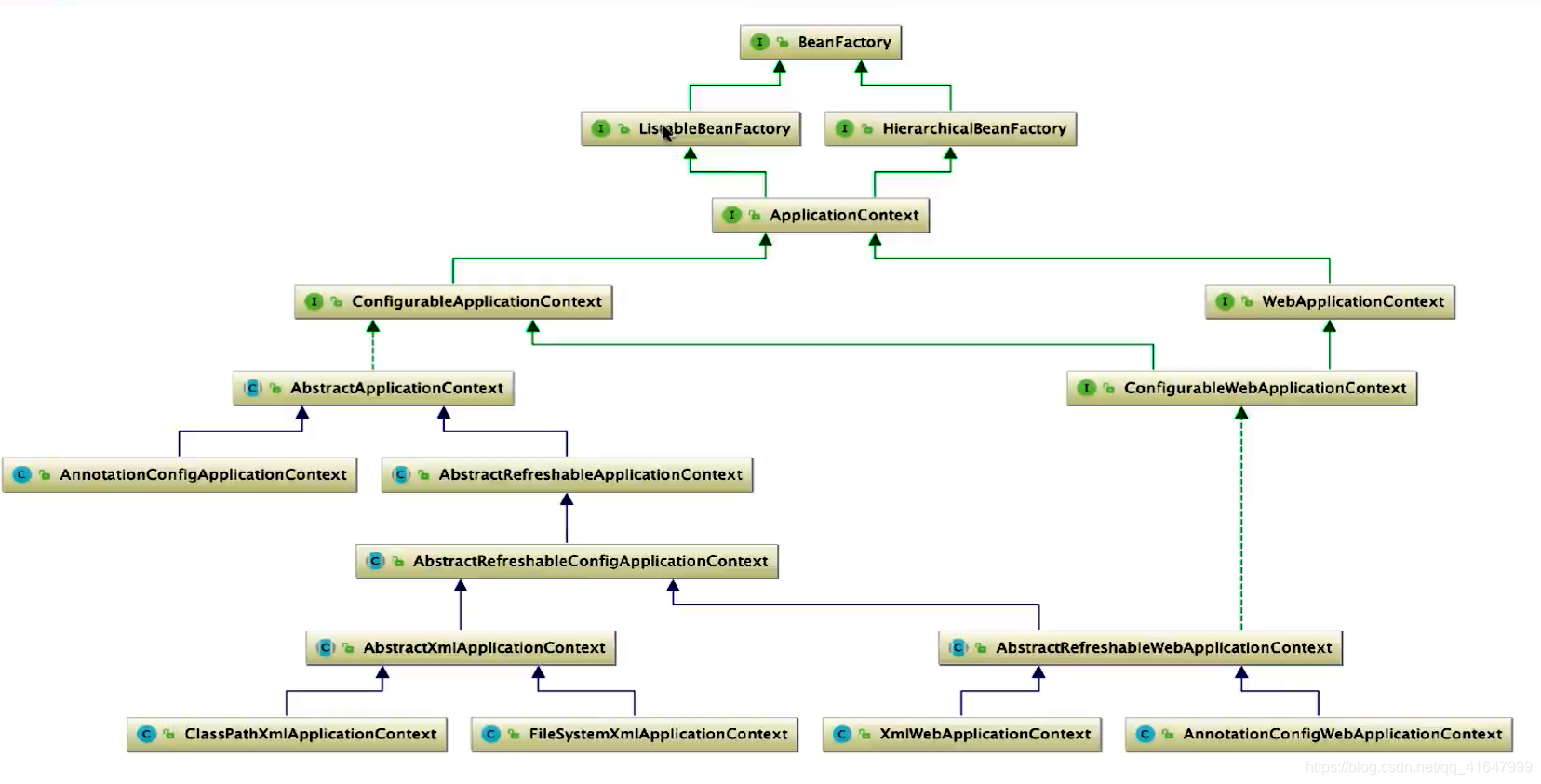 ListAbleBeanFactory 接口可以批量的获取Bean相关信息,例如经常使用的方法 String[ ] getBeanDefinitionNames() 就是来自该接口的定义。
组件扫描与自动装配
组件扫描:自动发现应用容器中需要创建的Bean
自动装配:自动满足Bean之间的依赖
BeanFactory 的子类如下: 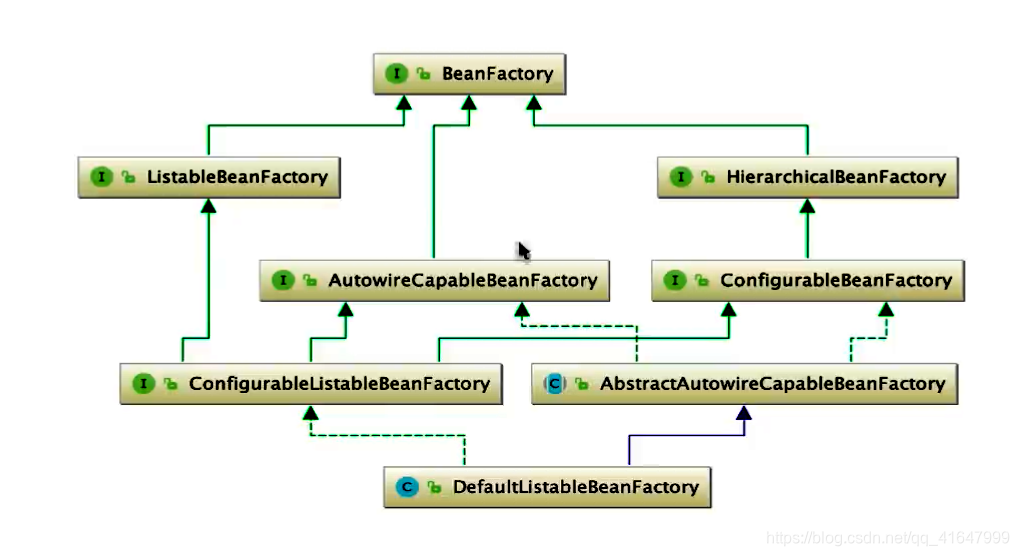
AutowireCapableBeanFactory
该接口定义了用于填充那些不受Spring容器控制的类。
public interface AutowireCapableBeanFactory extends BeanFactory { ... }
装配的方法主要是其实现类中 AbstractAutowireCapableBeanFactory 的 autowireBean(Object) 方法中调用的 populateBean() 方法:
public abstract class AbstractAutowireCapableBeanFactory extends AbstractBeanFactory
implements AutowireCapableBeanFactory {
@Override
public void autowireBean(Object existingBean) {
RootBeanDefinition bd = new RootBeanDefinition(ClassUtils.getUserClass(existingBean));
bd.setScope(BeanDefinition.SCOPE_PROTOTYPE);
bd.allowCaching = ClassUtils.isCacheSafe(bd.getBeanClass(), getBeanClassLoader());
BeanWrapper bw = new BeanWrapperImpl(existingBean);
initBeanWrapper(bw);
populateBean(bd.getBeanClass().getName(), bd, bw);
}
}
在代码中,不建议直接使用 AutowireCapableBeanFactory 接口,建议使用 @Autowired 装配。
ConfigurableListableBeanFactory
该接口继承了BeanFactory体系的其它接口,包含了BeanFactory体系的所有方法。
public interface ConfigurableListableBeanFactory
extends ListableBeanFactory, AutowireCapableBeanFactory, ConfigurableBeanFactory {...}
DefaultListableBeanFactory
该类是真正的第一个可以独立运行的IoC容器。
public class DefaultListableBeanFactory extends AbstractAutowireCapableBeanFactory
implements ConfigurableListableBeanFactory, BeanDefinitionRegistry, Serializable {...}
|