(一)编写线程池的配置文件
@Configuration
public class MyThreadpool {
@Bean
public ThreadPoolExecutor threadPoolExecutor(ThreadPoolConfigProperties pool){
return new ThreadPoolExecutor(pool.getCoreSize(),pool.getMaxSize(),pool.getKeepAliveTime(), TimeUnit.SECONDS,
new LinkedBlockingDeque<>(1000),
Executors.defaultThreadFactory(),
new ThreadPoolExecutor.AbortPolicy());
}
}
(二)自定义编写线程池参数配置,方便配置文件进行更改
@ConfigurationProperties(prefix = "zzc.thread")
@Component
public class ThreadPoolConfigProperties {
private Integer coreSize;
private Integer maxSize;
private Integer keepAliveTime;
private Integer dequeSize;
public ThreadPoolConfigProperties() {
}
public ThreadPoolConfigProperties(Integer coreSize, Integer maxSize, Integer keepAliveTime,Integer dequeSize) {
this.coreSize = coreSize;
this.maxSize = maxSize;
this.keepAliveTime = keepAliveTime;
this.dequeSize=dequeSize;
}
public Integer getCoreSize() {
return coreSize;
}
public void setCoreSize(Integer coreSize) {
this.coreSize = coreSize;
}
public Integer getMaxSize() {
return maxSize;
}
public void setMaxSize(Integer maxSize) {
this.maxSize = maxSize;
}
public Integer getKeepAliveTime() {
return keepAliveTime;
}
public void setKeepAliveTime(Integer keepAliveTime) {
this.keepAliveTime = keepAliveTime;
}
public Integer getDequeSize() {
return dequeSize;
}
public void setDequeSize(Integer dequeSize) {
this.dequeSize = dequeSize;
}
}
(三)配置文件引入 可以在配置文件中自定义线程池参数
zzc.thread.coreSize=20
zzc.thread.maxSize=20
zzc.thread.keepAliveTime=20
zzc.thread.dequeSize=1000
(四)引入项目中
这里就用测试类中,换在业务中也是一样的逻辑
@SpringBootTest
class ThreadpoolApplicationTests {
@Autowired
ThreadPoolExecutor executor;
@Test
public void tesetCompletableFuture() throws ExecutionException, InterruptedException {
CompletableFuture<String> infoFuture = CompletableFuture.supplyAsync(() -> {
String s = "基本信息获取";
return s;
}, executor);
CompletableFuture<Void> saleFuture = infoFuture.thenAcceptAsync((s) -> {
String ss = s + "::销售属性组合";
},executor);
CompletableFuture<Void> descFuture =infoFuture.thenAcceptAsync((s)->{
String ss= "商品介绍";
},executor);
CompletableFuture<Void> parameFuture = infoFuture.thenAcceptAsync((s)->{
String ss= "规格参数";
},executor);
CompletableFuture<Void> imageFuture = CompletableFuture.runAsync(() -> {
String ss = "获取图片";
}, executor);
CompletableFuture.allOf(infoFuture,saleFuture,descFuture,parameFuture,imageFuture).get();
}
}
异步编排的应用场景可以参考电商详情页,采用异步的方式先查出基本信息,然后根据基本信息采用异步分方式分别获取销售属性组合、商品介绍、规格参数,获取图片的任务不依赖于基本信息,故可以新开一个异步任务去处理。最后等所有异步任务完成,调用allOf进行组合 参考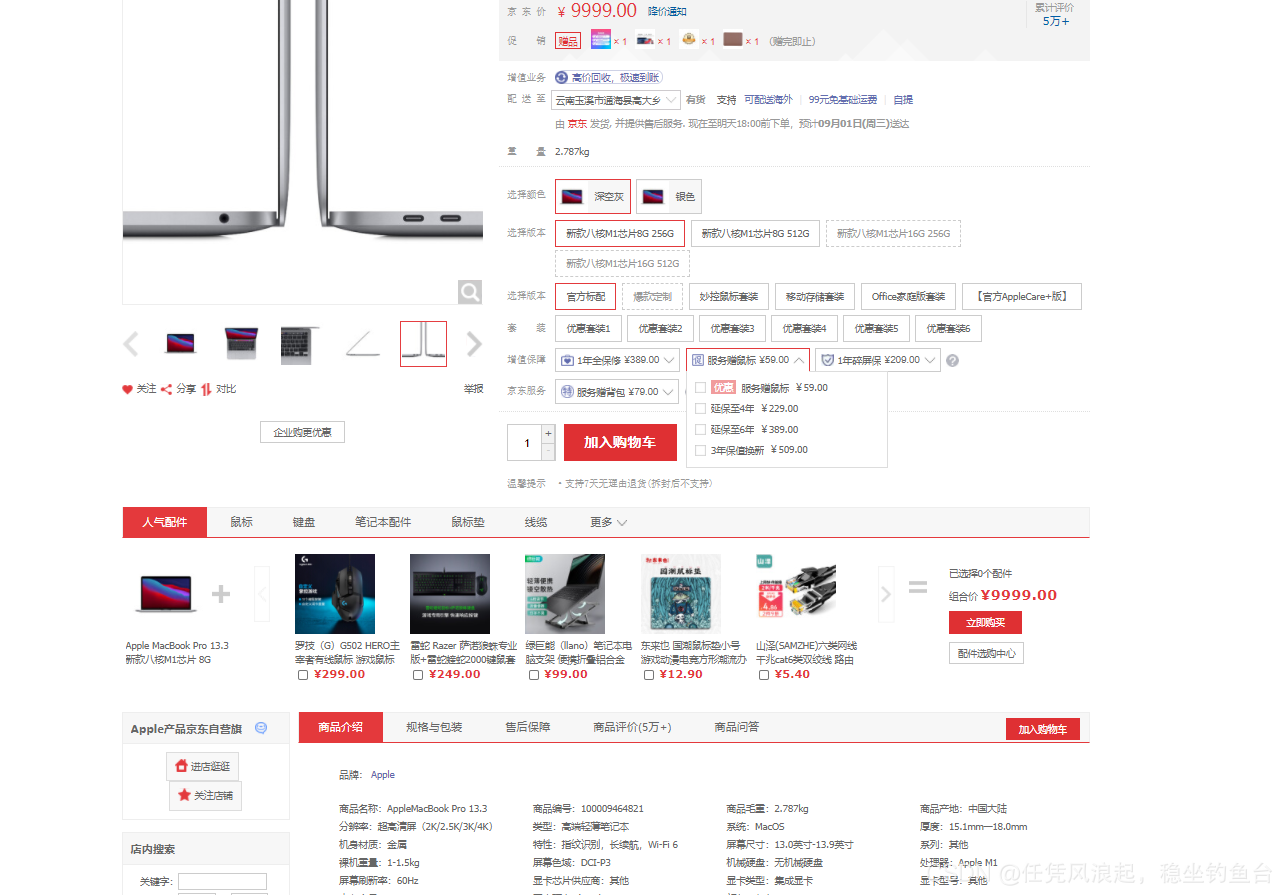
|