用户交互Scanner
用法
java.util.Scanner 类可以用于获取用户的输入 通过类中的next() 、nextLine() 方法获取输入的字符串 在读取前一般需要使用hasNext() 、hasNextLine() 判断是否还有输入的数据
区别
next() 方法:只能读取有效字符,在有效字符之前的空白会自动删除,之后的会作为分隔符或者结束符 nextLine() 方法:以Enter 为结束符,返回输入回车之前的所有字符
代码
package com.zlsaxx.scanner;
import java.util.Scanner;
public class Demo01 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
System.out.println("使用next方式接收:");
if (scanner.hasNext()){
String str = scanner.next();
System.out.println("输入的内容:"+str);
}
scanner.close();
}
}
小练习:和与平均数
求连续输入的数字的和以及他们的平均数
package com.zlsaxx.scanner;
import java.util.Scanner;
public class Demo02 {
public static void main(String[] args) {
Scanner scanner = new Scanner(System.in);
int cnt = 0 ;
double x = 0;
double sum = 0;
while(scanner.hasNextDouble()){
x = scanner.nextDouble();
sum += x;
cnt ++;
}
System.out.println(cnt + "个数的和为" + sum);
System.out.println(cnt + "个数的平均值为" + (sum / cnt));
scanner.close();
}
}
运行框: 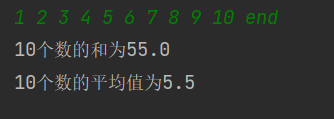
流程结构
顺序结构
按从上到下的顺序进行,是由若干个依次执行的处理步骤,是最基本的算法结构
选择结构
if 选择
一个if语句 至多有一个else语句 ,但是可以有不限个else if语句
public static void T2(){
Scanner scanner = new Scanner(System.in);
System.out.print("输入学生成绩:");
int score = scanner.nextInt();
if(score > 80){
System.out.println("优秀");
}else if(score > 60 && score <= 80){
System.out.println("及格");
}else{
System.out.println("不及格");
}
scanner.close();
}
运行框: 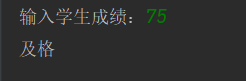
switch 选择
switch语句 中的变量类型可以是:byte 、short 、int 、char case 标签必须是:字符串常量 、字面量 case 可以理解为钥匙,匹配成功就从该语句进入 之后的每条语句都执行
switch(x){
case value1:
break;
case value2:
break;
default:
}
循环结构
while 循环
先判断再执行,只要布尔表达式 为true ,循环就一直执行
while(布尔表达式){
}
do…while 循环
先执行再判断,只要布尔表达式 为true ,循环就一直执行
do{
}while(布尔表达式)
for 循环
for(初始化;布尔表达式;更新){
}
小练习:九九乘法表
要求: 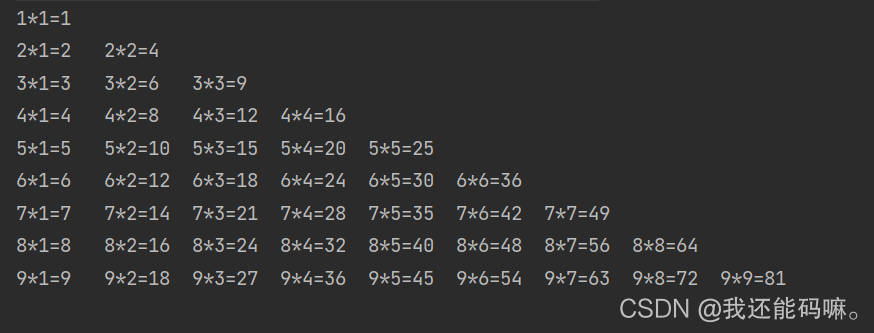 代码:
public static void T3(){
for(int i = 1;i < 10;i ++){
for(int j = 1;j <= i;j ++){
System.out.print(i + "*" + j + "=" + i*j + "\t");
}
System.out.println();
}
}
for 循环 Pro
在Java5 之后引入了一种用于数组或集合的增强型for循环
public static void T4(){
int[] numbers = {10,20,30,40,50};
for(int x:numbers){
System.out.print(x + " ");
}
}
运行框: 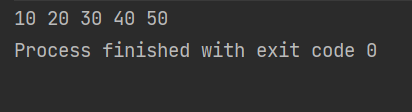
break & continue
介绍
break: 跳出整层循环 continue: 结束本次循环
补充:goto关键字
goto关键字 在语言中并未得到正式的使用,但带标签的break和带标签的continue是它的延伸 暂时还不理解,大概就是通过 outer 标签跳到第一层循环
public static void T5(){
outer:for(int i = 2;i < 100;i ++){
for(int j = 2;j < i / 2;j ++){
if(i % j == 0) {
continue outer;
}
}
System.out.print(i + " ");
}
}
运行框: 
|