Java学习笔记(12)——IO流和网络编程
一、IO流
1 io流概述
1.1 流的分类
- 1.操作数据单位:字节流、字符流
- 2.数据的流向:输入流、输出流
- 3.流的角色:节点流、处理流
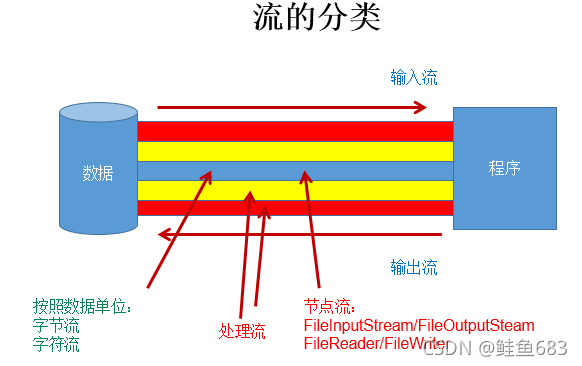 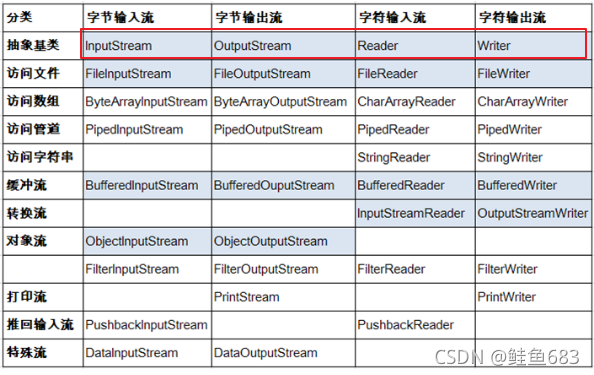 说明:红框对应的是IO流中的4个抽象基类。 蓝框的流需要大家重点关注。
2 File类介绍
2.1.File类的理解
- File类的一个对象,代表一个文件或一个文件目录(俗称:文件夹)
- File类声明在java.io包下
- File类中涉及到关于文件或文件目录的创建、删除、重命名、修改时间、文件大小等方法,并未涉及到写入或读取文件内容的操作。如果需要读取或写入文件内容,必须使用IO流来完成。
- 后续File类的对象常会作为参数传递到流的构造器中,指明读取或写入的"终点".
2.2.File的实例化
常用构造器
- File(String filePath)
- File(String parentPath,String childPath)
- File(File parentFile,String childPath)
路径的分类
- 相对路径:相较于某个路径下,指明的路径。
- 绝对路径:包含盘符在内的文件或文件目录的路径
说明:
- IDEA中:
如果大家开发使用JUnit中的单元测试方法测试,相对路径即为当前Module下。 如果大家使用main()测试,相对路径即为当前的Project下。 - Eclipse中:
不管使用单元测试方法还是使用main()测试,相对路径都是当前的Project下。
路径分隔符
- windows和DOS系统默认使用“\”来表示
- UNIX和URL使用“/”来表示
public void test1(){
File file1 = new File("hello.txt");
File file2 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior\\day08\\hi.txt");
System.out.println(file1);
System.out.println(file2);
File file3 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\","JavaSenior");
System.out.println(file3);
File file4 = new File(file3,"hi.txt");
System.out.println(file4);
}
2.3.File类的常用方法
public String getAbsolutePath():获取绝对路径 public String getPath() :获取路径 public String getName() :获取名称 public String getParent():获取上层文件目录路径。若无,返回null public long length() :获取文件长度(即:字节数)。不能获取目录的长度。 public long lastModified() :获取最后一次的修改时间,毫秒值 public String[] list() :获取指定目录下的所有文件或者文件目录的名称数组 public File[] listFiles() :获取指定目录下的所有文件或者文件目录的File数组
public void test2(){
File file1 = new File("hello.txt");
File file2 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior\\day08\\hi.txt");
System.out.println(file1.getAbsolutePath());
System.out.println(file1.getPath());
System.out.println(file1.getName());
System.out.println(file1.getParent());
System.out.println(file1.length());
System.out.println(new Date(file1.lastModified()));
System.out.println();
System.out.println(file2.getAbsolutePath());
System.out.println(file2.getPath());
System.out.println(file2.getName());
System.out.println(file2.getParent());
System.out.println(file2.length());
System.out.println(file2.lastModified());
}
@Test
public void test3(){
File file = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior");
String[] list = file.list();
for(String s : list){
System.out.println(s);
}
System.out.println();
File[] files = file.listFiles();
for(File f : files){
System.out.println(f);
}
}
@Test
public void test4(){
File file1 = new File("hello.txt");
File file2 = new File("D:\\io\\hi.txt");
boolean renameTo = file2.renameTo(file1);
System.out.println(renameTo);
}
public boolean isDirectory():判断是否是文件目录 public boolean isFile() :判断是否是文件 public boolean exists() :判断是否存在 public boolean canRead() :判断是否可读 public boolean canWrite() :判断是否可写 public boolean isHidden() :判断是否隐藏
@Test
public void test5(){
File file1 = new File("hello.txt");
file1 = new File("hello.txt");
System.out.println(file1.isDirectory());
System.out.println(file1.isFile());
System.out.println(file1.exists());
System.out.println(file1.canRead());
System.out.println(file1.canWrite());
System.out.println(file1.isHidden());
System.out.println();
File file2 = new File("d:\\io");
file2 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior");
System.out.println(file2.isDirectory());
System.out.println(file2.isFile());
System.out.println(file2.exists());
System.out.println(file2.canRead());
System.out.println(file2.canWrite());
System.out.println(file2.isHidden());
}
创建硬盘中对应的文件或文件目录 public boolean createNewFile() :创建文件。若文件存在,则不创建,返回false public boolean mkdir() :创建文件目录。如果此文件目录存在,就不创建了。如果此文件目录的上层目录不存在,也不创建。 public boolean mkdirs() :创建文件目录。如果此文件目录存在,就不创建了。如果上层文件目录不存在,一并创建
删除磁盘中的文件或文件目录 public boolean delete():删除文件或者文件夹 删除注意事项:Java中的删除不走回收站。
@Test
public void test6() throws IOException {
File file1 = new File("hi1.txt");
if(!file1.exists()){
file1.createNewFile();
System.out.println("创建成功");
}else{
file1.delete();
System.out.println("删除成功");
}
}
@Test
public void test7(){
File file1 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior");
boolean mkdir = file1.mkdir();
if(mkdir){
System.out.println("创建成功1");
}
File file2 = new File("E:\\3 编程学习资料\\尚硅谷java\\4_代码\\第2部分:Java高级编程\\JavaSenior");
boolean mkdir1 = file2.mkdirs();
if(mkdir1){
System.out.println("创建成功2");
}
File file3 = new File("D:\\io\\io1\\io4");
file3 = new File("D:\\io\\io1");
System.out.println(file3.delete());
}
}
3 节点流(文件流)
- 对于文本文件(.txt,.java,.c,.cpp),使用字符流处理
- 对于非文本文件(.jpg,.mp3,.mp4,.avi,.doc,.ppt,…),使用字节流处理
3.1 FileReader
文件内容读入程序中,并输出到控制台
说明点:
- read()的理解:返回读入的一个字符。如果达到文件末尾,返回-1
- 异常的处理:为了保证流资源一定可以执行关闭操作。需要使用try-catch-finally处理
- 读入的文件一定要存在,否则就会报FileNotFoundException。
步骤:
- 实例化File类的对象,指明要操作的文件
- 提供具体的流
- 数据的读入
- 流的关闭操作
public void testFileReader(){
FileReader fr = null;
try {
File file = new File("hello.txt");
fr = new FileReader(file);
int data;
while((data = fr.read()) != -1){
System.out.print((char)data);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fr != null){
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileReader1() {
FileReader fr = null;
try {
File file = new File("hello.txt");
fr = new FileReader(file);
char[] cbuf = new char[5];
int len;
while((len = fr.read(cbuf)) != -1){
String str = new String(cbuf,0,len);
System.out.print(str);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fr != null){
try {
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
3.2 FileWriter
从内存中写出数据到硬盘的文件里。
说明:
- 输出操作,对应的File可以不存在的。并不会报异常
- File对应的硬盘中的文件如果不存在,在输出的过程中,会自动创建此文件。
File对应的硬盘中的文件如果存在: 如果流使用的构造器是:FileWriter(file,false) / FileWriter(file):对原有文件的覆盖 如果流使用的构造器是:FileWriter(file,true):不会对原有文件覆盖,而是在原有文件基础上追加内容
public void testFileWriter() {
FileWriter fw = null;
try {
File file = new File("hello1.txt");
fw = new FileWriter(file,true);
fw.write("I have a dream!11111111111\n");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fw != null){
try {
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testFileReaderFileWriter() {
FileReader fr = null;
FileWriter fw = null;
try {
File srcFile = new File("hello.txt");
File destFile = new File("hello2.txt");
fr = new FileReader(srcFile);
fw = new FileWriter(destFile);
char[] cbuf = new char[5];
int len;
while((len = fr.read(cbuf)) != -1){
fw.write(cbuf,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
try {
if(fw != null)
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
try {
if(fr != null)
fr.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
3.3 FileInputStream和FileOutputStream
示例: 实现对图片的复制操作
@Test
public void testFileInputOutputStream() {
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcFile = new File("爱情与友情.jpg");
File destFile = new File("爱情与友情5.jpg");
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
byte[] buffer = new byte[5];
int len;
while((len = fis.read(buffer)) != -1){
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
指定路径下文件的复制
public void copyFile(String srcPath,String destPath){
FileInputStream fis = null;
FileOutputStream fos = null;
try {
File srcFile = new File(srcPath);
File destFile = new File(destPath);
fis = new FileInputStream(srcFile);
fos = new FileOutputStream(destFile);
byte[] buffer = new byte[1024];
int len;
while((len = fis.read(buffer)) != -1){
fos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fis != null){
try {
fis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testCopyFile(){
long start = System.currentTimeMillis();
String srcPath = "C:\\Users\\Administrator\\Desktop\\01-视频.avi";
String destPath = "C:\\Users\\Administrator\\Desktop\\02-视频.avi";
copyFile(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));
}
4.处理流之一缓冲流:
具体:
- BufferedInputStream
- BufferedOutputStream
- BufferedReader
- BufferedWriter
作用: 提供流的读取、写入的速度 提高读写速度的原因:内部提供了一个缓冲区
处理流,就是“套接”在已有的流的基础上。
@Test
public void BufferedStreamTest() throws FileNotFoundException {
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File("爱情与友情.jpg");
File destFile = new File("爱情与友情6.jpg");
FileInputStream fis = new FileInputStream((srcFile));
FileOutputStream fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[10];
int len;
while((len = bis.read(buffer)) != -1){
bos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
public void copyFileWithBuffered(String srcPath,String destPath){
BufferedInputStream bis = null;
BufferedOutputStream bos = null;
try {
File srcFile = new File(srcPath);
File destFile = new File(destPath);
FileInputStream fis = new FileInputStream((srcFile));
FileOutputStream fos = new FileOutputStream(destFile);
bis = new BufferedInputStream(fis);
bos = new BufferedOutputStream(fos);
byte[] buffer = new byte[1024];
int len;
while((len = bis.read(buffer)) != -1){
bos.write(buffer,0,len);
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bos != null){
try {
bos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(bis != null){
try {
bis.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testCopyFileWithBuffered(){
long start = System.currentTimeMillis();
String srcPath = "C:\\Users\\Administrator\\Desktop\\01-视频.avi";
String destPath = "C:\\Users\\Administrator\\Desktop\\03-视频.avi";
copyFileWithBuffered(srcPath,destPath);
long end = System.currentTimeMillis();
System.out.println("复制操作花费的时间为:" + (end - start));
}
@Test
public void testBufferedReaderBufferedWriter(){
BufferedReader br = null;
BufferedWriter bw = null;
try {
br = new BufferedReader(new FileReader(new File("dbcp.txt")));
bw = new BufferedWriter(new FileWriter(new File("dbcp1.txt")));
String data;
while((data = br.readLine()) != null){
bw.write(data);
bw.newLine();
}
} catch (IOException e) {
e.printStackTrace();
} finally {
if(bw != null){
try {
bw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(br != null){
try {
br.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
4 处理流之二:转换流
转换流:属于字符流
- InputStreamReader:将一个字节的输入流转换为字符的输入流
- OutputStreamWriter:将一个字符的输出流转换为字节的输出流
作用:
- 提供字节流与字符流之间的转换
- 解码:字节、字节数组 —>字符数组、字符串
- 编码:字符数组、字符串 —> 字节、字节数组
字符集
- ASCII:美国标准信息交换码。 用一个字节的7位可以表示。
- ISO8859-1:拉丁码表。欧洲码表用一个字节的8位表示。
- GB2312:中国的中文编码表。最多两个字节编码所有字符
- GBK:中国的中文编码表升级,融合了更多的中文文字符号。最多两个字节编码
- Unicode:国际标准码,融合了目前人类使用的所有字符。为每个字符分配唯一的字符码。所有的文字都用两个字节来表示。
- UTF-8:变长的编码方式,可用1-4个字节来表示一个字符。
public class InputStreamReaderTest {
@Test
public void test1() throws IOException {
FileInputStream fis = new FileInputStream("dbcp.txt");
InputStreamReader isr = new InputStreamReader(fis,"UTF-8");
char[] cbuf = new char[20];
int len;
while((len = isr.read(cbuf)) != -1){
String str = new String(cbuf,0,len);
System.out.print(str);
}
isr.close();
}
@Test
public void test2() throws Exception {
File file1 = new File("dbcp.txt");
File file2 = new File("dbcp_gbk.txt");
FileInputStream fis = new FileInputStream(file1);
FileOutputStream fos = new FileOutputStream(file2);
InputStreamReader isr = new InputStreamReader(fis,"utf-8");
OutputStreamWriter osw = new OutputStreamWriter(fos,"gbk");
char[] cbuf = new char[20];
int len;
while((len = isr.read(cbuf)) != -1){
osw.write(cbuf,0,len);
}
isr.close();
osw.close();
}
}
5 处理流之对象流
ObjectInputStream 和 ObjectOutputStream
作用:
- 用于存储和读取基本数据类型数据或对象的处理流。它的强大之处就是可以把Java中的对象写入到数据源中,也能把对象从数据源中还原回来。
要想一个java对象是可序列化的,需要满足相应的要求。
序列化机制:
- 对象序列化机制允许把内存中的Java对象转换成平台无关的二进制流,从而允许把这种二进制流持久地保存在磁盘上,或通过网络将这种二进制流传输到另一个网络节点。 当其它程序获取了这种二进制流,就可以恢复成原来的Java对象。
public class ObjectInputOutputStreamTest {
@Test
public void testObjectOutputStream(){
ObjectOutputStream oos = null;
try {
oos = new ObjectOutputStream(new FileOutputStream("object1.dat"));
oos.writeObject(new String("我爱北京天安门"));
oos.flush();
oos.writeObject(new Person("王铭",23));
oos.flush();
oos.writeObject(new Person("张学良",23,1001,new Account(5000)));
oos.flush();
} catch (IOException e) {
e.printStackTrace();
} finally {
if(oos != null){
try {
oos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
@Test
public void testObjectInputStream(){
ObjectInputStream ois = null;
try {
ois = new ObjectInputStream(new FileInputStream("object1.dat"));
Object obj = ois.readObject();
String str = (String) obj;
Person p = (Person) ois.readObject();
Person p1 = (Person) ois.readObject();
System.out.println(str);
System.out.println(p);
System.out.println(p1);
} catch (IOException e) {
e.printStackTrace();
} catch (ClassNotFoundException e) {
e.printStackTrace();
} finally {
if(ois != null){
try {
ois.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
}
二、 网络编程
1 计算机网络储备知识
一、网络编程中有两个主要的问题: 1.如何准确地定位网络上一台或多台主机;定位主机上的特定的应用 2.找到主机后如何可靠高效地进行数据传输
二、网络编程中的两个要素: 1.对应问题一:IP和端口号 2.对应问题二:提供网络通信协议:TCP/IP参考模型(应用层、传输层、网络层、物理+数据链路层)
三、通信要素一:IP和端口号
-
IP:唯一的标识 Internet 上的计算机(通信实体) -
在Java中使用InetAddress类代表IP -
IP分类:IPv4 和 IPv6 ; 万维网 和 局域网 -
域名: www.baidu.com www.mi.com www.sina.com www.jd.com www.vip.com -
本地回路地址:127.0.0.1 对应着:localhost -
如何实例化InetAddress:两个方法:getByName(String host) 、 getLocalHost() 两个常用方法:getHostName() / getHostAddress() -
端口号:正在计算机上运行的进程。 要求:不同的进程有不同的端口号 范围:被规定为一个 16 位的整数 0~65535。 -
端口号与IP地址的组合得出一个网络套接字:Socket
public static void main(String[] args) {
try {
InetAddress inet1 = InetAddress.getByName("192.168.10.14");
System.out.println(inet1);
System.out.println("****");
InetAddress inet2 = InetAddress.getByName("www.atguigu.com");
System.out.println(inet2);
InetAddress inet3 = InetAddress.getByName("127.0.0.1");
System.out.println(inet3);
System.out.println("****");
InetAddress inet4 = InetAddress.getLocalHost();
System.out.println(inet4);
System.out.println(inet2.getHostName());
System.out.println(inet2.getHostAddress());
} catch (UnknownHostException e) {
e.printStackTrace();
}
}
四、通信要素二:网络通信协议 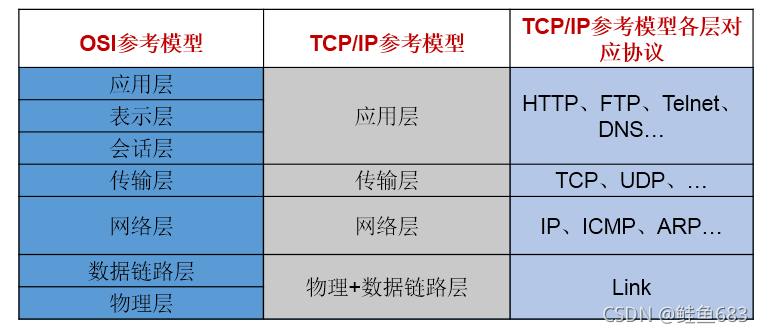 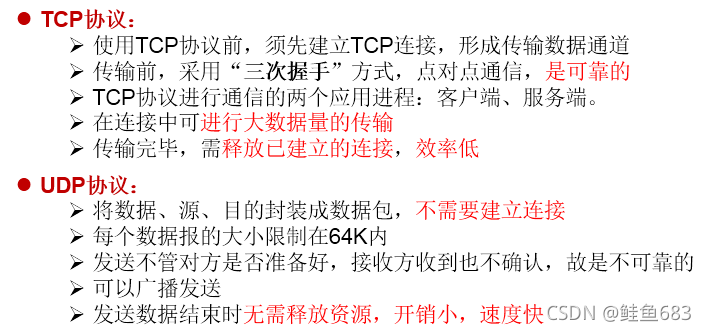 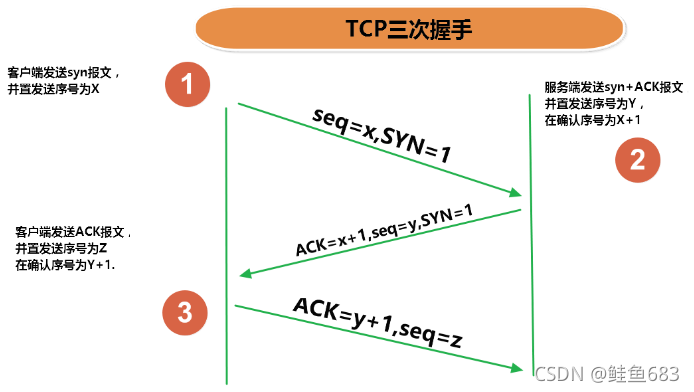 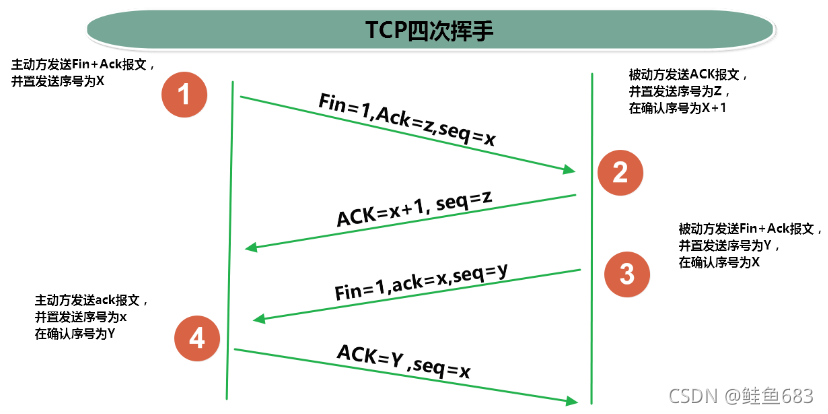
2 实现TCP的网络编程
代码示例1:客户端发送信息给服务端,服务端将数据显示在控制台上 //客户端
@Test
public void client() {
Socket socket = null;
OutputStream os = null;
try {
InetAddress inet = InetAddress.getByName("192.168.14.100");
socket = new Socket(inet,8899);
os = socket.getOutputStream();
os.write("你好,我是客户端mm".getBytes());
} catch (IOException e) {
e.printStackTrace();
} finally {
if(os != null){
try {
os.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
//服务端
@Test
public void server() {
ServerSocket ss = null;
Socket socket = null;
InputStream is = null;
ByteArrayOutputStream baos = null;
try {
ss = new ServerSocket(8899);
socket = ss.accept();
is = socket.getInputStream();
baos = new ByteArrayOutputStream();
byte[] buffer = new byte[5];
int len;
while((len = is.read(buffer)) != -1){
baos.write(buffer,0,len);
}
System.out.println(baos.toString());
System.out.println("收到了来自于:" + socket.getInetAddress().getHostAddress() + "的数据");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(baos != null){
try {
baos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(socket != null){
try {
socket.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(ss != null){
try {
ss.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
实现TCP的网络编程 例题3:从客户端发送文件给服务端,服务端保存到本地。并返回“发送成功”给客户端。 并关闭相应的连接。
public class TCPTest3 {
@Test
public void client() throws IOException {
Socket socket = new Socket(InetAddress.getByName("127.0.0.1"),9090);
OutputStream os = socket.getOutputStream();
FileInputStream fis = new FileInputStream(new File("beauty.jpg"));
byte[] buffer = new byte[1024];
int len;
while((len = fis.read(buffer)) != -1){
os.write(buffer,0,len);
}
socket.shutdownOutput();
InputStream is = socket.getInputStream();
ByteArrayOutputStream baos = new ByteArrayOutputStream();
byte[] bufferr = new byte[20];
int len1;
while((len1 = is.read(buffer)) != -1){
baos.write(buffer,0,len1);
}
System.out.println(baos.toString());
fis.close();
os.close();
socket.close();
baos.close();
}
@Test
public void server() throws IOException {
ServerSocket ss = new ServerSocket(9090);
Socket socket = ss.accept();
InputStream is = socket.getInputStream();
FileOutputStream fos = new FileOutputStream(new File("beauty6.jpg"));
byte[] buffer = new byte[1024];
int len;
while((len = is.read(buffer)) != -1){
fos.write(buffer,0,len);
}
System.out.println("图片传输完成");
OutputStream os = socket.getOutputStream();
os.write("你好,美女,照片我已收到,非常漂亮!".getBytes());
fos.close();
is.close();
socket.close();
ss.close();
os.close();
}
}
3 UDP协议的网络编程
public class UDPTest {
@Test
public void sender() throws IOException {
DatagramSocket socket = new DatagramSocket();
String str = "我是UDP方式发送的导弹";
byte[] data = str.getBytes();
InetAddress inet = InetAddress.getLocalHost();
DatagramPacket packet = new DatagramPacket(data,0,data.length,inet,9090);
socket.send(packet);
socket.close();
}
@Test
public void receiver() throws IOException {
DatagramSocket socket = new DatagramSocket(9090);
byte[] buffer = new byte[100];
DatagramPacket packet = new DatagramPacket(buffer,0,buffer.length);
socket.receive(packet);
System.out.println(new String(packet.getData(),0,packet.getLength()));
socket.close();
}
}
4 URL
统一资源定位符,对应着互联网的某一资源地址 如何实例化:
- URL url = new URL(“http://localhost:8080/examples/beauty.jpg?username=Tom”);
常用方法: 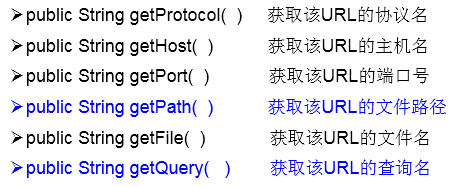
public class URLTest {
public static void main(String[] args) {
try {
URL url = new URL("http://localhost:8080/examples/beauty.jpg?username=Tom");
System.out.println(url.getProtocol());
System.out.println(url.getHost());
System.out.println(url.getPort());
System.out.println(url.getPath());
System.out.println(url.getFile());
System.out.println(url.getQuery());
} catch (MalformedURLException e) {
e.printStackTrace();
}
}
}
可以读取、下载对应的url资源:
public static void main(String[] args) {
HttpURLConnection urlConnection = null;
InputStream is = null;
FileOutputStream fos = null;
try {
URL url = new URL("http://localhost:8080/examples/beauty.jpg");
urlConnection = (HttpURLConnection) url.openConnection();
urlConnection.connect();
is = urlConnection.getInputStream();
fos = new FileOutputStream("day10\\beauty3.jpg");
byte[] buffer = new byte[1024];
int len;
while((len = is.read(buffer)) != -1){
fos.write(buffer,0,len);
}
System.out.println("下载完成");
} catch (IOException e) {
e.printStackTrace();
} finally {
if(is != null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(fos != null){
try {
fos.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if(urlConnection != null){
urlConnection.disconnect();
}
}
}
|