Mybatis 开发方式
方式一:注解
1.创建数据库信息
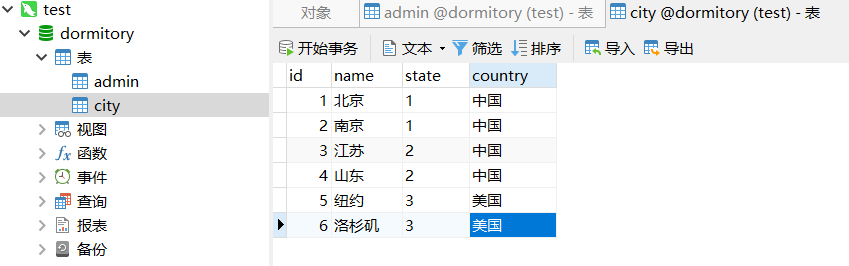
SET NAMES utf8mb4;
SET FOREIGN_KEY_CHECKS = 0;
DROP TABLE IF EXISTS `city`;
CREATE TABLE `city` (
`id` int(11) NOT NULL AUTO_INCREMENT,
`name` varchar(30) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL,
`state` varchar(30) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL,
`country` varchar(30) CHARACTER SET utf8 COLLATE utf8_general_ci NULL DEFAULT NULL,
PRIMARY KEY (`id`) USING BTREE
) ENGINE = InnoDB CHARACTER SET = utf8 COLLATE = utf8_general_ci ROW_FORMAT = Dynamic;
INSERT INTO `city` VALUES (1, '北京', '1', '中国');
INSERT INTO `city` VALUES (2, '南京', '1', '中国');
INSERT INTO `city` VALUES (3, '江苏', '2', '中国');
INSERT INTO `city` VALUES (4, '山东', '2', '中国');
INSERT INTO `city` VALUES (5, '纽约', '3', '美国');
INSERT INTO `city` VALUES (6, '洛杉矶', '3', '美国');
SET FOREIGN_KEY_CHECKS = 1;
返回顶部
2.封装bean
package com.zyx.core.admin.bean;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
@Data
@ToString
@NoArgsConstructor
@AllArgsConstructor
public class City {
private Integer id;
private String name;
private String state;
private String country;
}
返回顶部
3.创建CityMapper
- 我们创建一个查询的方法,可以发现报错:没有对应的mapper.xml匹配,这里我们将采用注解的形式进行。
我们直接使用@Select注解进行查询:@Select("select * from city where id=#{id}") 其中的id就会从方法的参数进行匹配。
package com.zyx.core.admin.mapper;
import com.zyx.core.admin.bean.City;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
@Mapper
public interface CityMapper {
@Select("select * from city where id=#{id}")
public City getById(Integer id);
}
返回顶部
4.创建CityService
package com.zyx.core.admin.service;
import com.zyx.core.admin.bean.City;
import com.zyx.core.admin.mapper.CityMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class CityService {
@Autowired
CityMapper cityMapper;
public City getById(Integer id){
City city = cityMapper.getById(id);
return city;
}
}
返回顶部
5.测试
package com.zyx.core.admin.test;
import com.zyx.core.admin.bean.City;
import com.zyx.core.admin.service.AdminService;
import com.zyx.core.admin.service.CityService;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import java.util.List;
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
public class MybatisTest {
@Autowired
CityService cityService;
@Test
public void getById() {
City city = cityService.getById(1);
System.out.println(city);
}
}
返回顶部
方式二:xml 配置
1.修改CityMapper
package com.zyx.core.admin.mapper;
import com.zyx.core.admin.bean.City;
import org.apache.ibatis.annotations.Mapper;
import org.apache.ibatis.annotations.Select;
@Mapper
public interface CityMapper {
@Select("select * from city where id=#{id}")
public City getById(Integer id);
public void insert(City city);
}
返回顶部
2.创建CityMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.zyx.core.admin.mapper.CityMapper">
<insert id="insert" parameterType="com.zyx.core.admin.bean.City" useGeneratedKeys="true" keyColumn="id">
INSERT INTO city(name, state, country) VALUES(#{name},#{state},#{country})
</insert>
</mapper>
该方式等同于:
@Insert(" INSERT INTO city(name, state, country) VALUES(#{name},#{state},#{country})")
@Options(useGeneratedKeys = true,keyProperty = "id")
public void insert(City city);
- useGeneratedKeys 和 keyProperty 搭配使用,这样可以解决在主键自增的情况下获取主键
返回顶部
3.修改CityService
package com.zyx.core.admin.service;
import com.zyx.core.admin.bean.City;
import com.zyx.core.admin.mapper.CityMapper;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.stereotype.Service;
@Service
public class CityService {
@Autowired
CityMapper cityMapper;
public City getById(Integer id){
City city = cityMapper.getById(id);
return city;
}
public void insert(City city){
cityMapper.insert(city);
}
}
返回顶部
4.测试
package com.zyx.core.admin.test;
import com.zyx.core.admin.bean.City;
import com.zyx.core.admin.service.AdminService;
import com.zyx.core.admin.service.CityService;
import org.junit.Test;
import org.junit.runner.RunWith;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.boot.test.context.SpringBootTest;
import org.springframework.test.context.junit4.SpringJUnit4ClassRunner;
import java.util.List;
@RunWith(SpringJUnit4ClassRunner.class)
@SpringBootTest
public class MybatisTest {
@Autowired
CityService cityService;
@Test
public void getById() {
City city = cityService.getById(1);
System.out.println(city);
}
@Test
public void insert() {
City city = new City();
city.setName("成都");
city.setState("2");
city.setCountry("中国");
cityService.insert(city);
System.out.println(city);
}
}
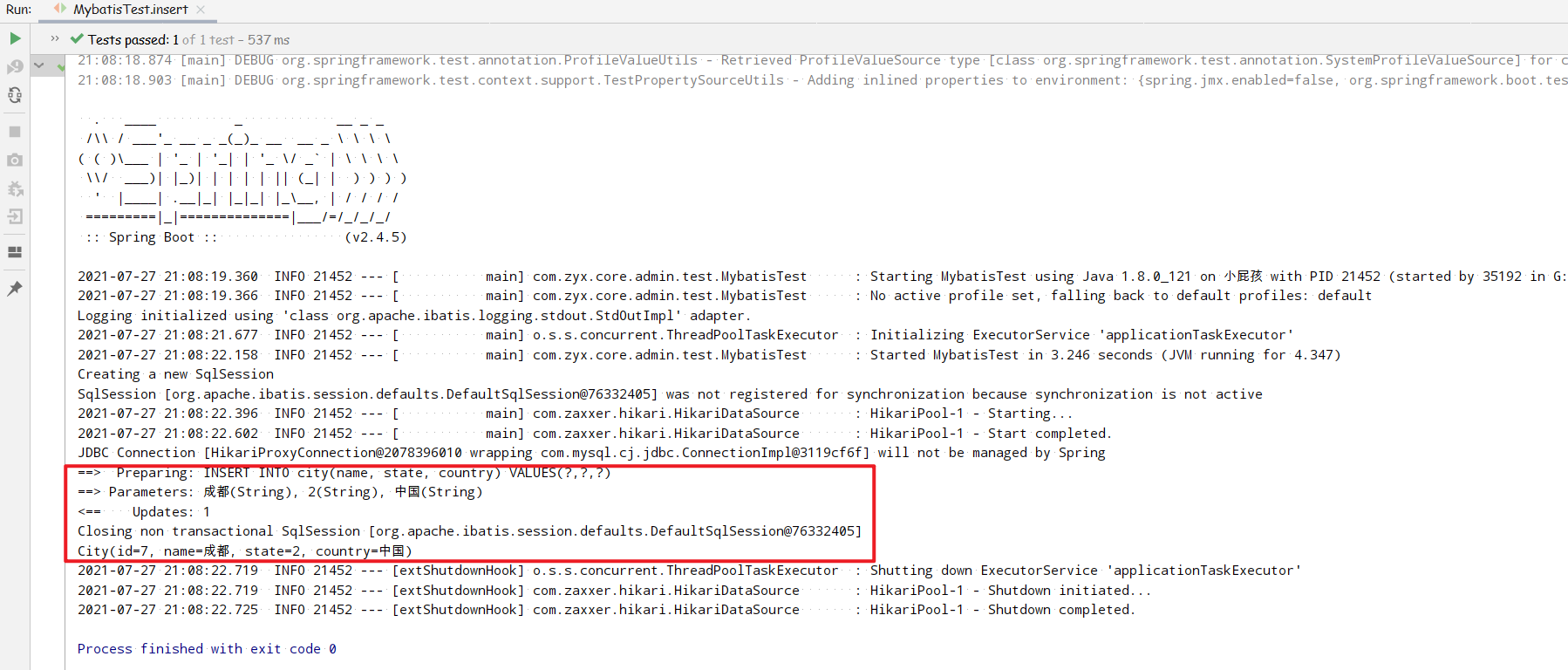
返回顶部
方式三:混合注解和xml
最佳实战:
返回顶部
参考:https://www.bilibili.com/video/BV19K4y1L7MT?p=64
|