一、Spring概述
1.1 web 项目开发中的耦合度问题
-
在Servlet中需要调用service中的方法,则需要在Servlet类中通过new关键字创建Service的实例 public interface StudentService{
? ?public List<Student> listStudent();
}
?
public class StudentServiceImpl1 implements StudentService{
? ?public List<Student> listStudent(){
? ? ? ?//查询学生基本信息
? }
}
?
public class StudentServiceImpl2 implements StudentService{
? ?public List<Studen> listStudent(){
? ? ? ?//查询学生成绩信息
? }
}
?
public class StudentListService extends HttpServlet{
? ?//在Servlet中使用new关键字创建StudentServiceImpl1对象,增加了servlet和service的耦合度
? ?private StudentService studentService = new StudentServiceImpl1();
? ?protected void doGet(HttpServletRequest request,HttpServletResponse response){
? ? ? ?studentService.listStudent();
? }
? ?protected void doPost(HttpServletRequest request,HttpServletResponse response){
? ? ? ?doGet(resquest,response);
? }
}
1.2 面向接口编程
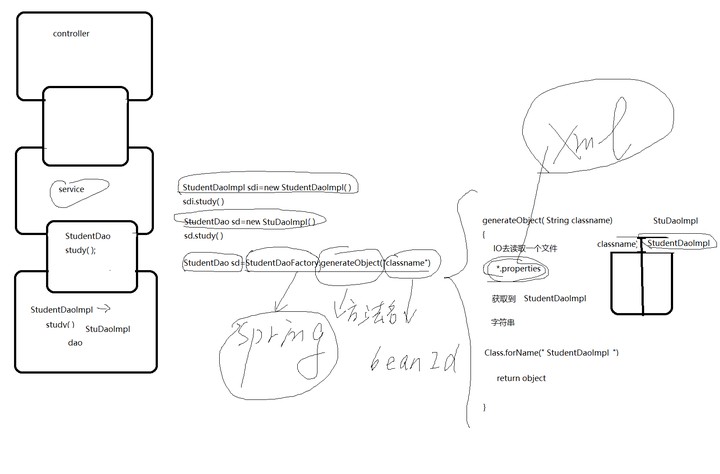
解决方案 :在servlet中定义service接口的对象,不使用new关键字创建实现类对象,在servlet实例化的时候,通过反射动态的给service对象变量赋值
如何实现 :Spring实现
二、Spring IoC
Spring IoC容器组件,可以完成对象的创建、对象的属性赋值、对象管理
2.1 Spring框架部署(IoC)
2.1.1 创建Maven工程
2.1.2 添加SpringIoC依赖
-
core -
beans -
aop -
expression -
context
<dependency>
? ? ? ? ? ?<groupId>org.springframework</groupId>
? ? ? ? ? ?<artifactId>spring-beans</artifactId>
? ? ? ? ? ?<version>5.2.13.RELEASE</version>
? ? ? ?</dependency>
?
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework</groupId>
? ? ? ? ? ?<artifactId>spring-core</artifactId>
? ? ? ? ? ?<version>5.2.13.RELEASE</version>
? ? ? ?</dependency>
?
? ? ? ?<dependency>
? ? ? ? ? ?<groupId>org.springframework</groupId>
? ? ? ? ? ?<artifactId>spring-context</artifactId>
? ? ? ? ? ?<version>5.2.13.RELEASE</version>
? ? ? ?</dependency>
2.1.3 创建Spring配置文件
通过配置文件 “告诉” Spring容器创建什么对象,给对象属性赋什么值
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
? ? ? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
? ? ? xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
?
?
</beans>
2.2 SpringIoC使用
使用SpringIoC组件创建并管理对象
2.2.1 创建一个实体类
package com.whoami.bean;
?
import java.util.Date;
?
/**
* @author whoami
* @date 2021年08月27日 20:56
*/
public class Student {
?
? ?private String stuNum;
? ?private String stuNname;
? ?private int stuAge;
? ?private String stuGender;
? ?private Date entranceTime; //入学时间
? ? ? 、、、Get ?Set ?toString、、、
}
?
2.2.2 在Spring配置文件中配置实体类
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
? ? ? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
? ? ? xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
? ?<!--通过bean将实体类配置给Spring进行管理,id表示实体类的唯一标识-->
? ?<bean id="student" class="com.whoami.bean.Student">
? ? ? ?<property name="stuNum" value="10002"></property>
? ? ? ?<property name="stuNname" value="李四"></property>
? ? ? ?<property name="stuAge" value="20"></property>
? ? ? ?<property name="stuGender" value="女"></property>
? ?</bean>
?
</beans>
?
2.2.4 初始化Spring对象工厂,获取对象
? ? ? ?//1.初始化Spring容器,加载Spring配置文件
? ? ? ?ClassPathXmlApplicationContext context = new ClassPathXmlApplicationContext("applicationContext.xml");
? ? ? ?//通过Spring容器获取Student对象
? ? ? ?Student student1 = (Student) context.getBean("student");
|