一、通过ServletAPI获取请求参数
- 将HttpServletRequest作为控制器方法的形参,此时HttpServletRequest类型的参数表示封装了当前请求的请求报文的对象(会将DispatcherServlet中所获得的表示当前请求的Request对象赋值给这个参数)。
案例: 还是用我上篇博客的web模块为例 index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<a th:href="@{/testRM(username='mike',password=123456)}">跳转到target.html</a><br>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import javax.servlet.http.HttpServletRequest;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(HttpServletRequest request){
String username = request.getParameter("username");
String password = request.getParameter("password");
System.out.println("username:"+username+",password:"+password);
return "target";
}
}
运行Tomcat: 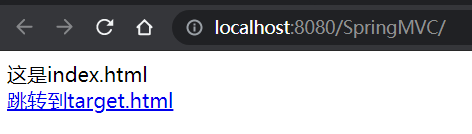  IDEA输出: 
二、通过控制器方法的形参获取请求参数
- 在控制器方法的形参位置,设置和请求参数同名的形参,当浏览器发送请求,匹配到请求映射时,在DispatcherServlet中就会将请求参数赋值给相应的形参。
- 若请求所传输的请求参数中有多个同名的请求参数(如复选框),此时可以在控制器方法的形参中设置字符串数组或者字符串类型的形参接收此请求参数。
- 若使用字符串数组类型的形参,此参数的数组中包含了每一个数据。
- 若使用字符串类型的形参,此参数的值为每个数据中间使用逗号拼接的结果。
案例1: index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<a th:href="@{/testRM(username='mike',password=123456)}">跳转到target.html</a><br>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(String username,String password){
System.out.println("username:"+username+",password:"+password);
return "target";
}
}
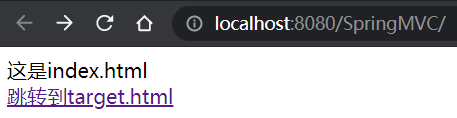  IDEA输出:  案例2: 有复选框的表单 index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<form th:action="@{/testRM}" method="get">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
爱好:<input type="checkbox" name="hobby" value="Java">Java
<input type="checkbox" name="hobby" value="Go">Go
<input type="checkbox" name="hobby" value="Python">Python<br>
<input type="submit">
</form>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(String username,String password,String hobby){
System.out.println("username:"+username+",password:"+password+",hobby:"+hobby);
return "target";
}
}
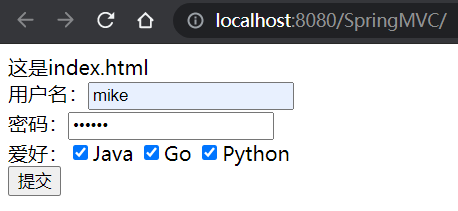  IDEA输出:  可以发现使用字符串形式形参时,结果为每个数据中间使用逗号拼接的结果。 我们也可以使用字符串数组形式的形参:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import java.util.Arrays;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(String username,String password,String[] hobby){
System.out.println("username:"+username+",password:"+password+",hobby:"+ Arrays.toString(hobby));
return "target";
}
}
 这个方法和前篇博客的占位符的方法有点类似,但是那个方法只能获取请求参数的value值不能获取name。
三、@RequestParam
假如我们使用上面的方法,但我们地址栏请求参数的名字是user_name,而我们控制器方法里的形参是username怎么办呢?这就要用到@RequestParam注解:
- @RequestParam是将请求参数和控制器方法的形参创建映射关系
- @RequestParam注解一共有三个属性:
- value:指定为形参赋值的请求参数的参数名
- required:设置是否必须传输此请求参数,默认值为true。
若设置为true时,则当前请求必须传输value所指定的请求参数,若没有传输该请求参数,且没有设置defaultValue属性,则页面报错400。若设置为false,则当前请求不是必须传输value所指定的请求参数,若没有传输,则注解所标识的形参的值为null。 - defaultValue:不管required属性值为true或false,当value所指定的请求参数没有传输或传输的值为
"" (即空串)时,则使用默认值为形参赋值。
案例: index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<a th:href="@{/testRM(user_name='mike',password=123456)}">跳转到target.html</a>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RequestParam;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(
@RequestParam(value = "user_name",required = true,defaultValue = "admin") String username,
String password){
System.out.println("username:"+username+",password:"+password);
return "target";
}
}
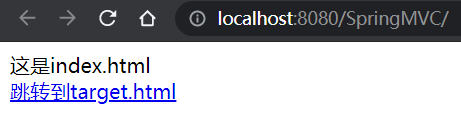  IDEA输出:  如果我们将请求地址里user_name的值改成空串:  那么就会使用它设置的defaultValue默认值admin为形参赋值: 
四、@RequestHeader
- @RequestHeader是将请求头信息和控制器方法的形参创建映射关系
- @RequestHeader注解一共有三个属性:value、required、defaultValue,用法同@RequestParam
案例: index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<a th:href="@{/testRM(username='mike',password=123456)}">跳转到target.html</a>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestHeader;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(String username, String password, @RequestHeader(value = "User-Agent") String userAgent){
System.out.println("username:"+username+",password:"+password+",请求头User-Agent信息:"+userAgent);
return "target";
}
}
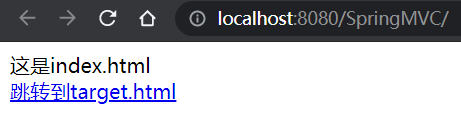 
五、@CookieValue
- @CookieValue是将cookie数据和控制器方法的形参创建映射关系
- @CookieValue注解一共有三个属性:value、required、defaultValue,用法同@RequestParam
案例: index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<a th:href="@{/testRM(username='mike',password=123456)}">跳转到target.html</a>
</body>
</html>
controller:
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.CookieValue;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(String username, String password, @CookieValue("Idea-ec12eb5b") String cookie){
System.out.println("username:"+username+",password:"+password+",cookie信息:"+cookie);
return "target";
}
}
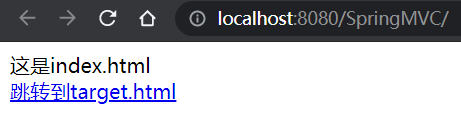  IDEA输出: 
六、通过POJO获取请求参数
- 可以在控制器方法的形参位置设置一个实体类类型的形参,此时若浏览器传输的请求参数的所有参数名都和实体类中的属性名一致,那么请求参数就会为此实体类的对象的属性赋值。
案例: 实体类User:
public class User {
private String username;
private String password;
private Integer age;
private String sex;
private String email;
public User() {
}
public User(String username, String password, Integer age, String sex, String email) {
this.username = username;
this.password = password;
this.age = age;
this.sex = sex;
this.email = email;
}
@Override
public String toString() {
return "User{" +
"username='" + username + '\'' +
", password='" + password + '\'' +
", age=" + age +
", sex='" + sex + '\'' +
", email='" + email + '\'' +
'}';
}
public String getUsername() {
return username;
}
public void setUsername(String username) {
this.username = username;
}
public String getPassword() {
return password;
}
public void setPassword(String password) {
this.password = password;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<form th:action="@{/testRM}" method="post">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
性别:<input type="radio" name="sex" value="男">男<input type="radio" name="sex" value="女">女<br>
年龄:<input type="text" name="age"><br>
邮箱:<input type="text" name="email"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
controller:
import com.fox.mvc.pojo.User;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(User user){
System.out.println(user);
return "target";
}
}
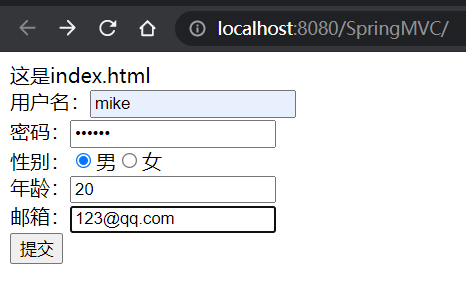  IDEA输出: 
七、解决获取请求参数的乱码问题
在上例中,IDEA输出可能会出现乱码问题。form表单是get方式和post方式出现了乱码的解决方法也不同:
(一)解决get方式的乱码问题
找到你电脑上Tomcat的安装地址,在conf目录下有个server.xml,用记事本打开并做修改: 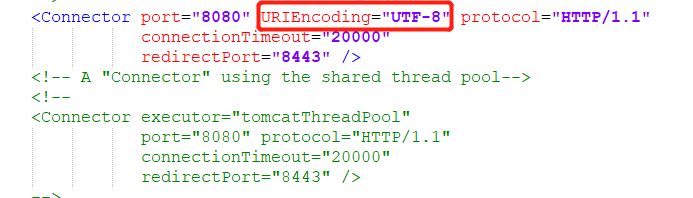 修改了以后在IDEA重启Tomcat即可。
(二)解决post方式的乱码问题
由于在访问我们自己写的请求控制器的方法前,请求会统一先提交到DispatcherServlet,所以我们在自己的请求控制器方法里修改编码没用。因此我们需要在DispatcherServlet获取请求参数之前就要修改编码。那么我们知道JavaWeb 三大组件分别是:Servlet 程序、Filter 过滤器、Listener 监听器,它们的执行顺序是:Listener 监听器——>Filter 过滤器——>Servlet 程序。那么我们就可以在过滤器阶段修改编码。过滤器也不需要我们自己写,可以使用SpringMVC提供的编码过滤器CharacterEncodingFilter,但是必须在web.xml中进行注册。
注:SpringMVC中处理编码的过滤器一定要配置到其他过滤器之前,否则无效
我们通过查看org.springframework.web.filter.CharacterEncodingFilter的源码: 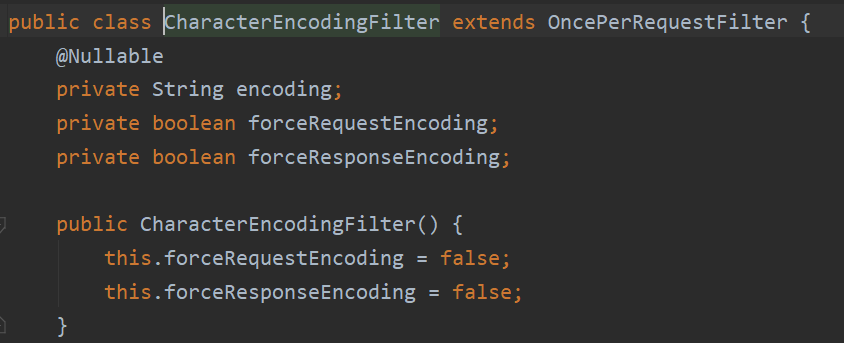 我们发现,它有三个属性:encoding、forceRequestEncoding、forceResponseEncoding,起初forceRequestEncoding和forceResponseEncoding的值都是false。 我们再找到它真正执行过滤的方法doFilterInternal() : 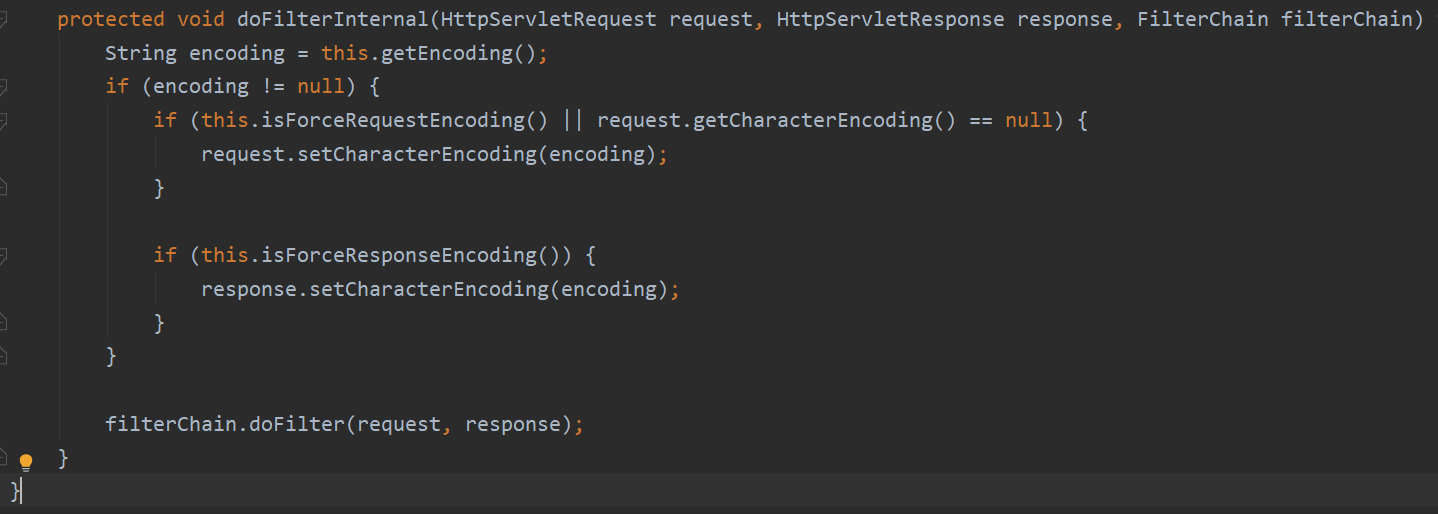 首先this.getEncoding()即它的属性encoding的get方法,一开始encoding是没有值的,而下面的代码,当encoding!=null 才可以执行,因此我们可以在web.xml里给encoding进行注入。接着if (this.isForceRequestEncoding() || request.getCharacterEncoding() == null) 由于是或运算,我们只需要满足一边条件为true即可,左边的方法其实就是判断其属性forceRequestEncoding是否为true,我们知道一开始就是false,那么再看右边的表达式,request.getCharacterEncoding() 确实为空,并没有设置过,因此代码会往下执行request.setCharacterEncoding(encoding); ,那么请求的编码就设置为我们自己注入的encoding属性了。那么此时这个post方式的乱码问题就已经解决了,再说题外话:我想一并解决响应的乱码问题怎么办呢? 那么再往下面的代码看,if(this.idForceResponseEncoding) 也即forceResponseEncoding必须为true才会执行,因此我们只需要在web.xml里给这个属性也进行注入,设置为true即可执行后面的代码response.setCharacterEncoding(encoding); ,从而解决响应的乱码问题。
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
<init-param>
<param-name>forceResponseEncoding</param-name>
<param-value>true</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
index.html:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>首页</title>
</head>
<body>
这是index.html<br>
<form th:action="@{/testRM}" method="post">
用户名:<input type="text" name="username"><br>
密码:<input type="password" name="password"><br>
性别:<input type="radio" name="sex" value="男">男<input type="radio" name="sex" value="女">女<br>
年龄:<input type="text" name="age"><br>
邮箱:<input type="text" name="email"><br>
<input type="submit" value="提交">
</form>
</body>
</html>
controller:
import com.fox.mvc.pojo.User;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.RequestMapping;
@Controller
public class MyController {
@RequestMapping(value = "/testRM")
public String testRequestMapping(User user){
System.out.println(user);
return "target";
}
}
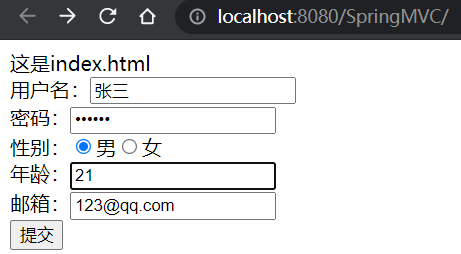 就不会有乱码问题了: 
|