项目开发中,不会使用下面 3 种线程的实现方式,因为上面 3 种线程实现方式无法控制
线程,可能会造成系统资源耗尽,浪费系统资源,造成系统的性能下降。仅做原理理解。
1、继承 Thread 类,实现多线程
@Slf4j
public class ThreadDemo {
public static void main(String[] args) {
ThreadA threadA = new ThreadA();
threadA.run();
}
public static class ThreadA extends Thread{
@Override
public void run() {
log.info("继承 Thread 实现方式.......");
int i = 100/3;
log.info("业务代码执行结果:{}, 线程名称:{}, 线程 ID:{}",i,this.getName(),this.getId());
}
}
}
2、 实现 Runnable 接口,实现多线程
2.1 普通构建方式
@Slf4j
public class ThreadDemo {
public static void main(String[] args) {
Thread02 thread02 = new Thread02();
Thread thread = new Thread(thread02);
thread.start();
}
public static class Thread02 implements Runnable {
@Override
public void run() {
log.info("继承 Thread 实现方式.......");
int i = 100 / 3;
log.info("业务代码执行结果:{}", i);
}
}
}
2.2 匿名内部类的构建方式
匿名内部类使用: 接口名称 对象名=new 接口名称(){//覆盖重写所有的抽象方法}; 作用:内部类能够访问外部内的一切成员变量和方法,包括私有的,而实现接口或继承类做不到。
@Slf4j
public class ThreadDemo {
public static void main(String[] args) {
Runnable runnable = new Runnable() {
@Override
public void run() {
log.info("继承 Thread 实现方式.......");
int i = 100 / 3;
log.info("业务代码执行结果:{}", i);
}
};
Thread thread = new Thread(runnable);
thread.start();
}
}
2.3 lambda 表达式实现
lambda 表达式特点:
- @FunctionalInterface: 此直接表示可以使用 lambda 表达式的编程方式,此注解相当于是一个标识
- 接口只有一个方法(必须满足)-- 即使没有上面注解,也可以使用lambda表达式;程序员会在后台自动识别
- 写作形式: 方法括号(有参写,无参不写)
Runnable 接口只有一个方法,满足条件。
@Slf4j
public class ThreadDemo {
public static void main(String[] args) {
Thread thread = new Thread(()->{
log.info("继承 Thread 实现方式.......");
int i = 100 / 3;
log.info("业务代码执行结果:{}", i);
});
thread.start();
}
}
3 Callable + FutureTask 实现多线程
- jdk1.5 后:添加 callable 接口,实现多线程,相较于 Thread, runnable 接口没有返回值,但是 callable 接口是有返回值。
- 接口参数 public interface Callable :
- 接口说明:
1、具有泛型的接口,只有一个方法 call,call 方法就是多线程执行业务主体; 2、方法执行完毕后有返回值,返回值类型就是制定的泛型类型
疑问:多线程执行必须和 Thread 有关系,callable 接口和 thread 有什么关系呢?? FutureTask 构造函数 有Callable. 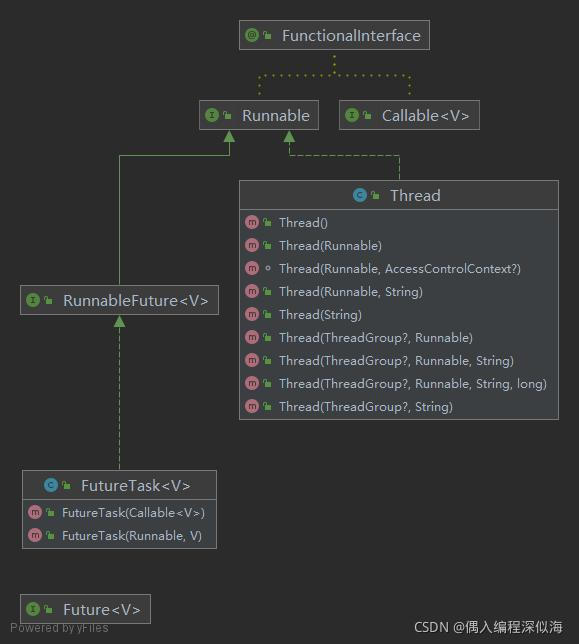
3.1 普通实现方式
@Slf4j
public class ThreadDemo {
public static void main(String[] args) {
Thread03 thread03 = new Thread03();
FutureTask<Integer> task = new FutureTask<Integer>(thread03);
Thread thread = new Thread(task);
thread.start();
try {
Integer integer = task.get();
} catch (InterruptedException e) {
e.printStackTrace();
} catch (ExecutionException e) {
e.printStackTrace();
}
}
public static class Thread03 implements Callable<Integer> {
@Override
public Integer call() throws Exception {
log.info("继承 Thread 实现方式.......");
int i = 100 / 3;
log.info("业务代码执行结果:{}", i);
return i;
}
}
}
3.2 匿名内部类
3.3 lambda 表达式
|