客户信息管理系统——Java
该系统没有涉及文件及数据库,适合刚接触java的新手进行练习。模拟实现基于文本界面的客户软件管理系统。能够实现基本增删改查操作以及类的使用
- 文件排布如下图:
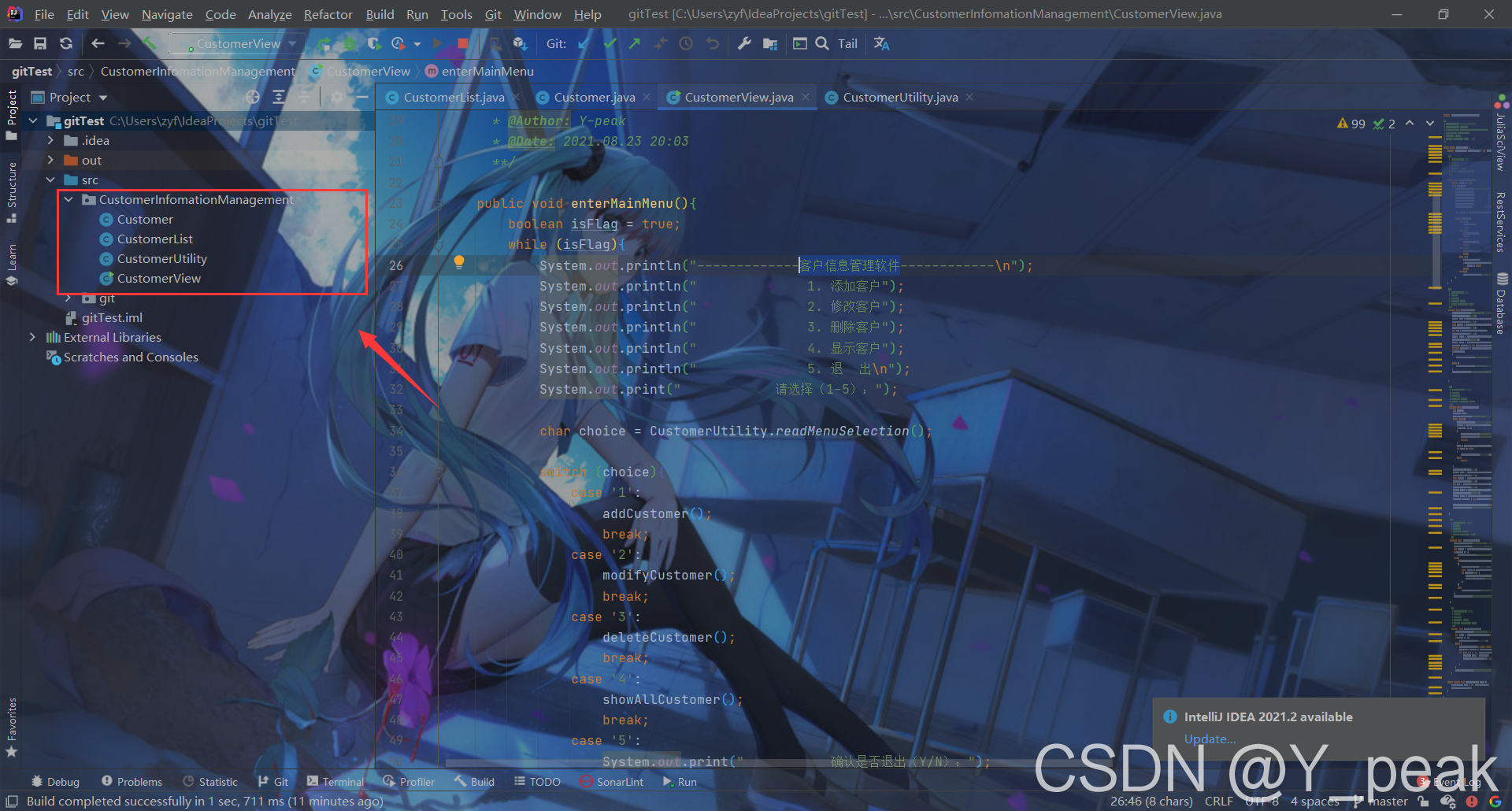
源代码
package CustomerInfomationManagement;
import java.util.Scanner;
public class CustomerUtility {
private static Scanner scanner = new Scanner(System.in);
public static char readMenuSelection(){
char c;
while (true){
String str = readKeyBoard(1,false);
c = str.charAt(0);
if(c > '5' || c < '1'){
System.out.print(" 输入错误,请重新输入:");
}else break;
}
return c;
}
public static char readChar(){
String str = readKeyBoard(1,false);
return str.charAt(0);
}
public static char readChar(char defaultValue){
String str = readKeyBoard(1,true);
return (str.length()==0)?defaultValue:str.charAt(0);
}
public static int readInt(){
int n;
while (true){
String str = readKeyBoard(2,false);
try {
n = Integer.parseInt(str);
if (n>=0)
break;
else
System.out.print(" 输入错误,请重新输入:");
}catch (NumberFormatException e)
{
System.out.print(" 输入错误,请重新输入:");
}
}
return n;
}
public static int readInt(int defaultValue){
int n;
while (true)
{
String str = readKeyBoard(2, true);
if(str.length() == 0)
return defaultValue;
try {
n = Integer.parseInt(str);
if (n>=0)
break;
else
System.out.print(" 输入错误,请重新输入:");
}catch (NumberFormatException e)
{
System.out.print(" 输入错误,请重新输入:");
}
}
return n;
}
public static String readString(int limit){
return readKeyBoard(limit,false);
}
public static String readString(int limit,String defaultValue){
String str = readKeyBoard(limit,true);
return str.length() == 0 ? defaultValue:str;
}
public static char readConfirmSelection(){
char c;
while (true){
String str = readKeyBoard(1,false).toUpperCase();
c = str.charAt(0);
if(c == 'Y' || c == 'N')
break;
System.out.print(" 输入错误,请重新输入:");
}
return c;
}
public static String readKeyBoard(int n, boolean b){
String str="";
while (scanner.hasNextLine()){
str = scanner.nextLine();
int len = str.length();
if (len == 0){
if (b)
return str;
continue;
}else if(len>n){
System.out.print(" 输入错误,请重新输入:");
continue;
}
break;
}
return str;
}
}
package CustomerInfomationManagement;
public class Customer {
private String name;
private char gender;
private int age;
private String phone;
private String email;
public Customer() {
}
public Customer(String name, char gender, int age, String phone, String email) {
this.name = name;
this.gender = gender;
this.age = age;
this.phone = phone;
this.email = email;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public char getGender() {
return gender;
}
public void setGender(char gender) {
this.gender = gender;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getPhone() {
return phone;
}
public void setPhone(String phone) {
this.phone = phone;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
}
package CustomerInfomationManagement;
public class CustomerList {
private Customer[] customers;
private int total;
private int maxTotal;
public CustomerList(int totalCustomer) {
this.customers = new Customer[totalCustomer];
this.maxTotal = totalCustomer;
this.total = 0;
}
public boolean addCustomer(Customer customer){
if(total>=maxTotal)
return false;
customers[total++] = customer;
return true;
}
public boolean replaceCustomer(int index,Customer cust){
if(index>= maxTotal)
return false;
customers[index] = cust;
return true;
}
public boolean deleteCustomer(int index){
if (index<0 || index>=total)
return false;
for (int i = 0; i < total - 1; i++) {
customers[i] = customers[i+1];
}
customers[total - 1] = null;
total--;
return true;
}
public Customer[] gerAllCustomer(){
Customer[] customers1 = new Customer[total];
for (int i = 0; i < total; i++) {
customers1[i] = customers[i];
}
return customers1;
}
public Customer getCustomer(int index){
if(index < 0 || index >= total)
return null;
return customers[index];
}
public int getTotal(){
return total;
}
}
package CustomerInfomationManagement;
public class CustomerView {
private CustomerList customerList = new CustomerList(10);
public void enterMainMenu(){
boolean isFlag = true;
while (isFlag){
System.out.println("-------------客户信息管理软件------------\n");
System.out.println(" 1. 添加客户");
System.out.println(" 2. 修改客户");
System.out.println(" 3. 删除客户");
System.out.println(" 4. 显示客户");
System.out.println(" 5. 退 出\n");
System.out.print(" 请选择(1-5):");
char choice = CustomerUtility.readMenuSelection();
switch (choice){
case '1':
addCustomer();
break;
case '2':
modifyCustomer();
break;
case '3':
deleteCustomer();
break;
case '4':
showAllCustomer();
break;
case '5':
System.out.print(" 确认是否退出(Y/N):");
if(CustomerUtility.readConfirmSelection() == 'Y')
isFlag = false;
break;
default:
System.out.println("怎么1-5都能输入错误");
}
}
}
private void addCustomer(){
System.out.println("------------- 添加客户信息 ------------");
System.out.printf("姓名:");
String name = CustomerUtility.readString(10);
System.out.printf("性别:");
char gender = CustomerUtility.readChar();
System.out.printf("年龄:");
int age = CustomerUtility.readInt();
System.out.printf("电话:");
String phone = CustomerUtility.readString(13);
System.out.printf("Email:");
String email = CustomerUtility.readString(20);
Customer customer = new Customer(name,gender, age, phone, email);
boolean isSuccess = customerList.addCustomer(customer);
if(isSuccess)
{
System.out.println("-------------添加成功-----------------");
}else {
System.out.println("-----------空间已满,添加失败-----------");
}
System.out.println("-------------------------------------");
}
private void modifyCustomer(){
int number;
Customer temp;
System.out.println("-------------修改客户信息----------------");
while (true){
System.out.printf("请选择待修改的客户编号(0退出):");
number = CustomerUtility.readInt();
if(number == 0)
{
System.out.println("------------已退出修改客户信息------------");
System.out.println("--------------------------------------");
return;
}
temp = customerList.getCustomer(number-1);
if (temp == null)
{
System.out.println("无法找到指定客户");
}else
break;
}
System.out.printf("姓名:");
String name = CustomerUtility.readString(10,temp.getName());
System.out.printf("性别:");
char gender = CustomerUtility.readChar(temp.getGender());
System.out.printf("年龄:");
int age = CustomerUtility.readInt(temp.getAge());
System.out.printf("电话:");
String phone = CustomerUtility.readString(13,temp.getPhone());
System.out.printf("Email:");
String email = CustomerUtility.readString(20,temp.getEmail());
Customer customer = new Customer(name,gender, age, phone, email);
customerList.replaceCustomer(number-1,customer);
System.out.println("--------------------------------------");
}
private void deleteCustomer(){
System.out.println("-------------删除客户信息----------------");
System.out.printf("请选择需要删除的客户编号(0退出):");
int number = CustomerUtility.readInt();
if(number == 0)
System.out.println("-----------------已退出----------------");
else{
if(customerList.getCustomer(number-1) != null)
{
System.out.print("确认是否删除(Y/N):");
char choice = CustomerUtility.readConfirmSelection();
if (choice=='Y') {
customerList.deleteCustomer(number - 1);
System.out.println("----------------删除成功----------------");
}
}
else
System.out.println("----------未找到指定编号,删除失败---------");
}
System.out.println("--------------------------------------");
}
private void showAllCustomer(){
System.out.println("------------- 客户信息显示 ------------");
int total = customerList.getTotal();
if(total == 0){
System.out.println("未找到客户记录");
}else {
System.out.println("编号\t\t姓名\t\t性别\t\t年龄\t\t电话\t\t\t邮箱");
Customer[] custNew = customerList.gerAllCustomer();
for (int i = 0; i < custNew.length; i++) {
Customer temp = custNew[i];
System.out.println((i+1) + "\t\t" + temp.getName() + "\t\t" + temp.getGender() + "\t\t" +temp.getAge() + "\t\t" + temp.getPhone() + "\t\t\t" + temp.getEmail());
}
}
System.out.println("-------------------------------------");
}
public static void main(String[] args) {
CustomerView view = new CustomerView();
view.enterMainMenu();
}
}
使用截图
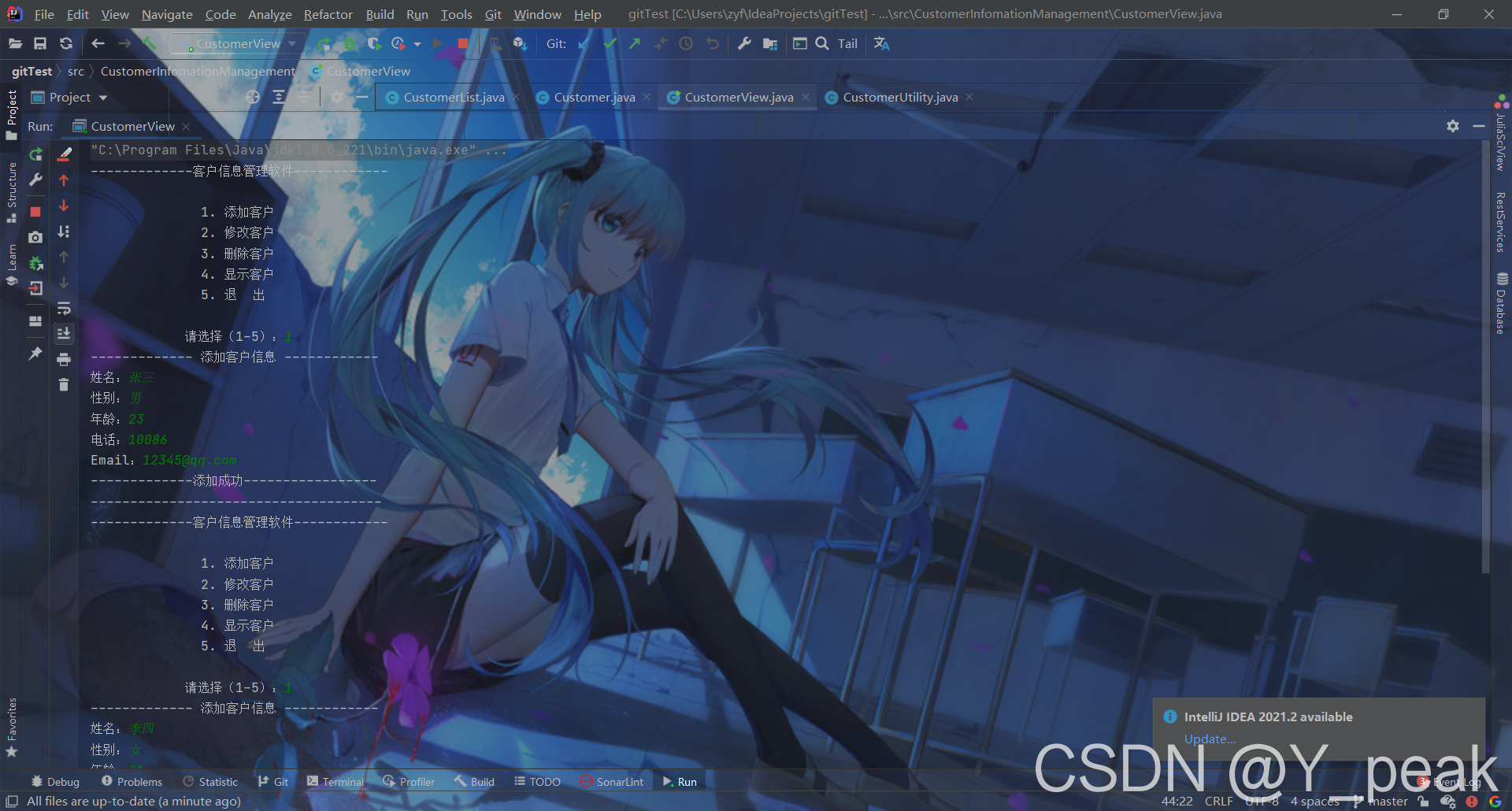
- 查
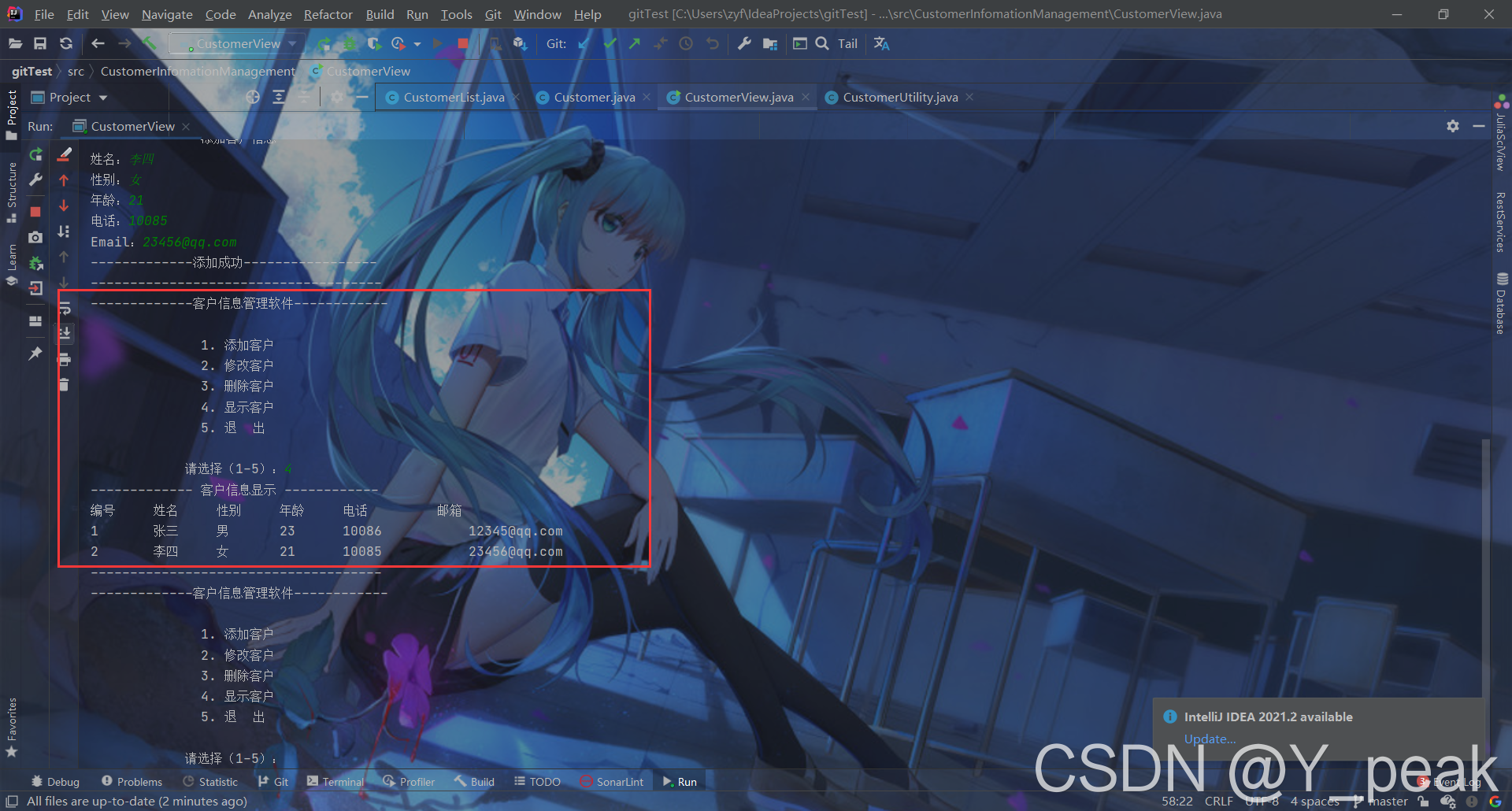 - 改
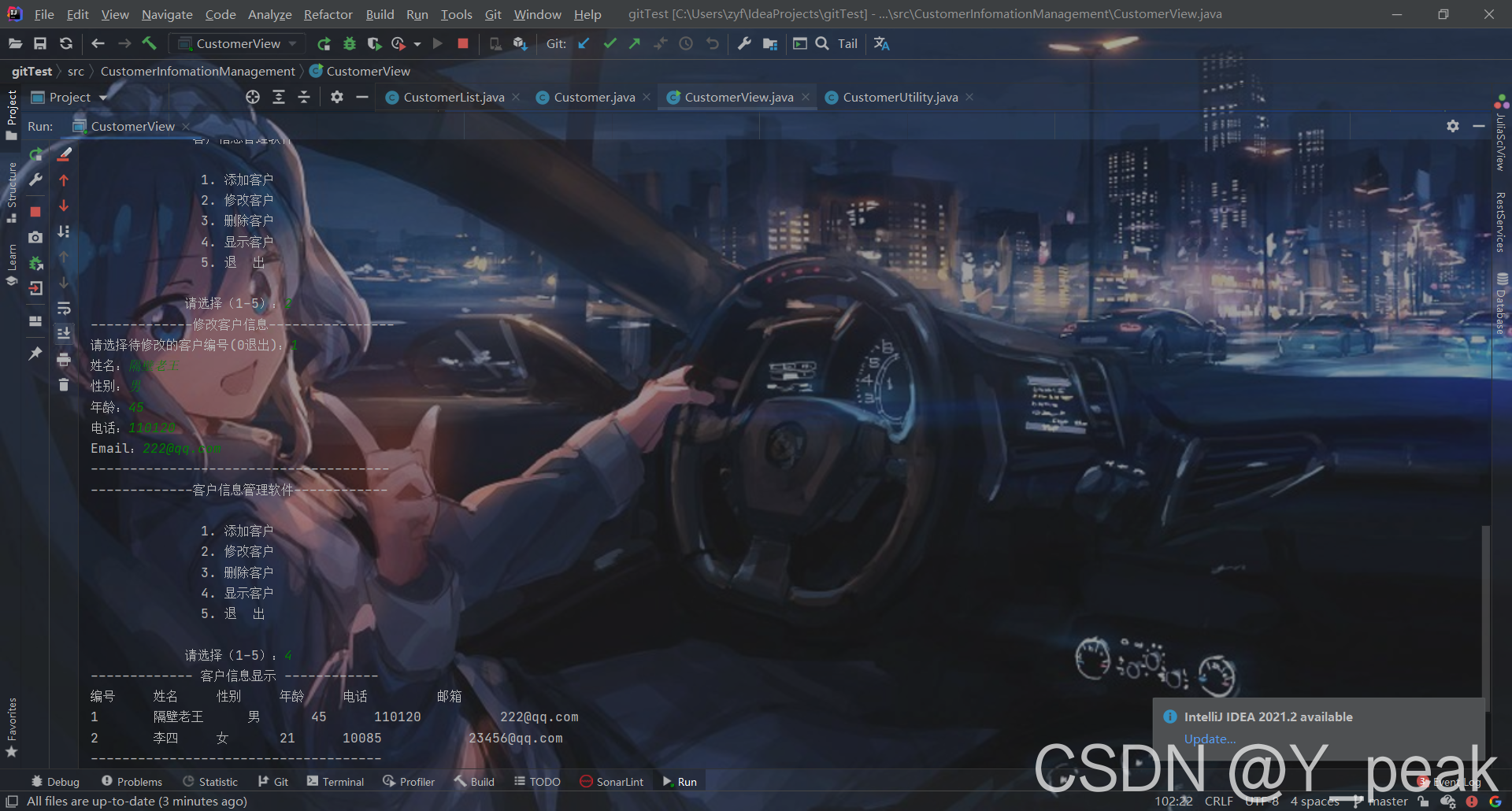 - 删
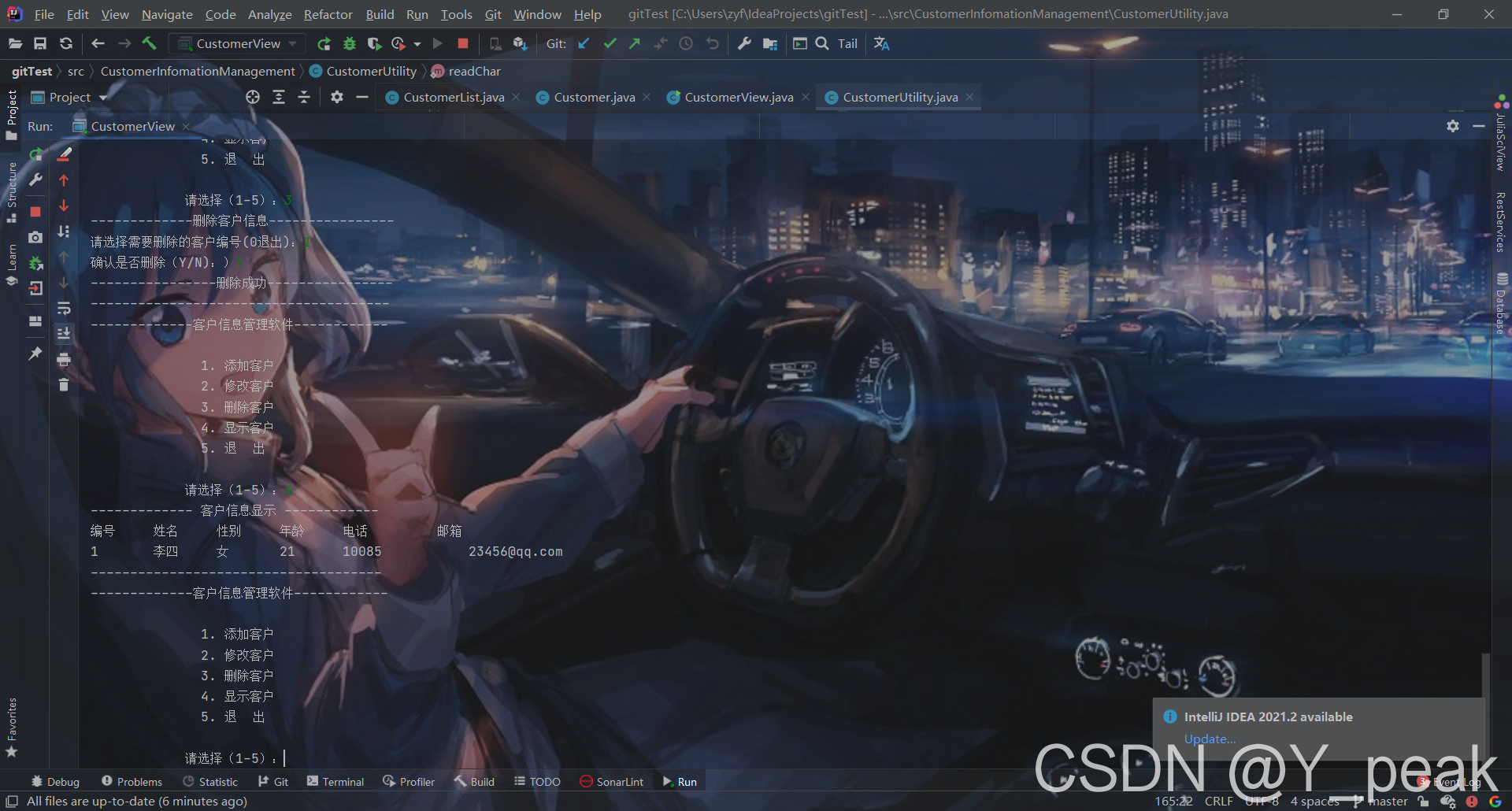
|