1、安装 Tomcat

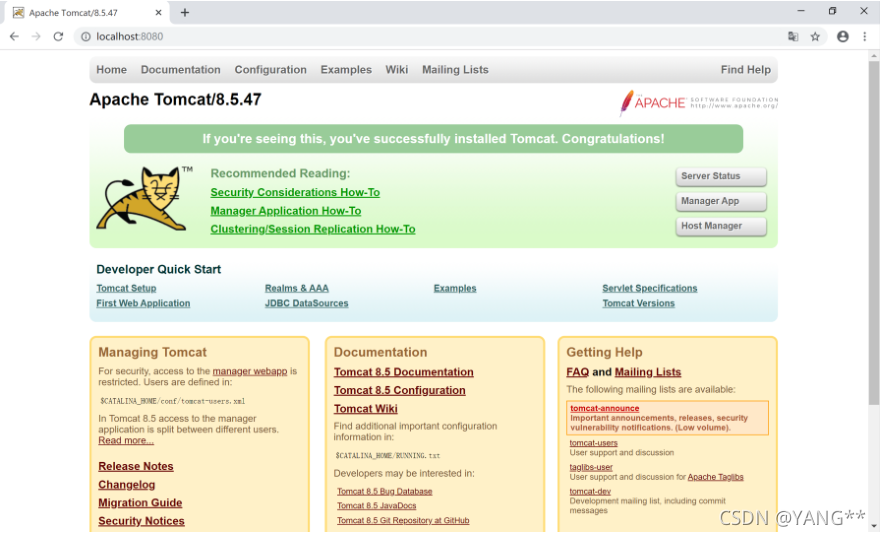
2、Servlet 的使用方式
2.1、Tomcat 环境下使用注解方式
2.1.1、添加注解依赖
<dependencies>
? ?<dependency>
? ? ? ?<groupId>javax.servlet</groupId>
? ? ? <artifactId>javax.servlet-api</artifactId>
? ? ? <version>4.0.1</version>
? ? ? <scope>provided</scope>
? ?</dependency>
</dependencies>
2.1.2、添加 tomcat 插件
<build>
? ?<plugins>
? ? ? ?<plugin>
? ? ? ? ? ?<groupId>org.apache.tomcat.maven</groupId>
? ? ? ? <artifactId>tomcat7-maven-plugin</artifactId>
? ? ? ? <version>2.2</version>
? ? ? ? <configuration>
? ? ? ? ? ? <uriEncoding>UTF-8</uriEncoding>
? ? ? ? ? ? <!-- 访问路径 -->
? ? ? ? ? ? <path>/</path>
? ? ? ? ? ? <!--
? ? ? ? ? ? ? ? 也可以通过命令指定访问端口
? ? ? ? ? ? ? ? mvn tomcat7:run -D maven.tomcat.port=8080
? ? ? ? ? ? -->
? ? ? ? ? ? <port>8080</port>
? ? ? ? ? ? <!-- 是否开启热部署 -->
? ? ? ? ? ? <contextReloadable>false</contextReloadable>
? ? ? ? </configuration>
? ? </plugin>
? ?</plugins>
</build>
2.1.3、测试代码:
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
?
@WebServlet(urlPatterns = "/first")
public class FirstServlet extends HttpServlet {
? ?@Override
? ?protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
? ? ? ?super.service(req, resp);
? ? ? ?System.out.println("Hello Servelt!");
? }
}
2.1.4、访问 http://localhost:8080/first

2.2、jetty 环境下使用注解方式
2.2.1、添加 jetty 插件
<plugin>
? ?<groupId>org.eclipse.jetty</groupId>
? ?<artifactId>jetty-maven-plugin</artifactId>
? ?<version>9.4.43.v20210629</version>
? ?<configuration>
? ? ? ?<!-- 访问路径 -->
? ? ? ?<webAppConfig>
? ? ? ? ? ?<contextPath>/</contextPath>
? ? ? ?</webAppConfig>
? ? ? ?<httpConnector>
? ? ? ? ? ?<!--
? ? ? ? ? ? ? ?也可以通过命令指定访问端口
? ? ? ? ? ? ? ?mvn jetty:run -D jetty.port=8080
? ? ? ? ? ?-->
? ? ? ? ? ?<port>8080</port>
? ? ? ?</httpConnector>
? ? ? ?<!-- 扫描进行热部署的间隔时间,0:不进行热部署 -->
? ? ? ?<scanIntervalSeconds>0</scanIntervalSeconds>
? ?</configuration>
</plugin>
2.2.2、替换 web.xml 文件内容
<?xml version="1.0" encoding="UTF-8"?>
<web-app xmlns="http://xmlns.jcp.org/xml/ns/javaee"
? ? ? ? xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
? ? ? ? xsi:schemaLocation="http://xmlns.jcp.org/xml/ns/javaee
? ? ? ? http://xmlns.jcp.org/xml/ns/javaee/web-app_4_0.xsd"
? ? ? ? version="4.0">
</web-app>
2.2.3、访问 http://localhost:8080/first
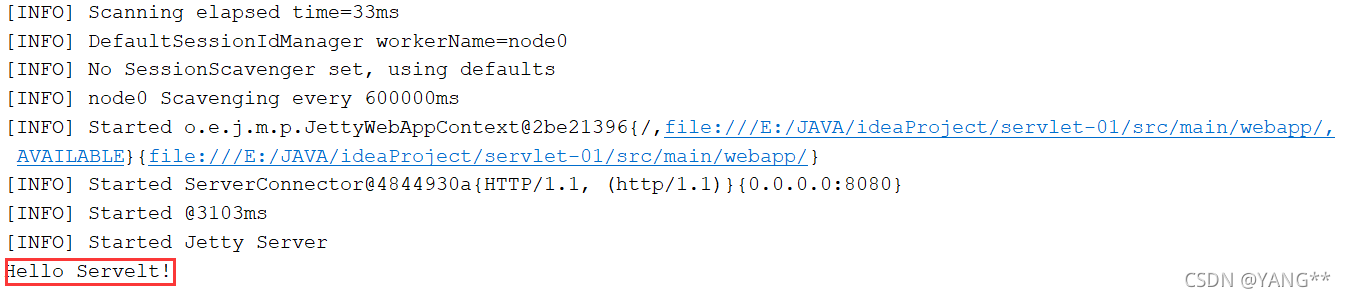
2.3、Servlet 的 xml 方式使用
2.3.1、修改 web.xml 文件内容
<servlet>
? ?<servlet-name>secondServlet</servlet-name>
? ?<servlet-class>com.yjxxt.servlet.SecondServlet</servlet-class>
</servlet>
<servlet-mapping>
? ?<servlet-name>secondServlet</servlet-name>
? ?<url-pattern>/second</url-pattern>
</servlet-mapping>
2.3.2、测试代码:
import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
?
public class SecondServlet extends HttpServlet {
? ?@Override
? ?protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
? ? ? ?super.service(req, resp);
? ? ? ?System.out.println("SecondServlet");
? }
}
2.3.3、访问 http://localhost:8080/second
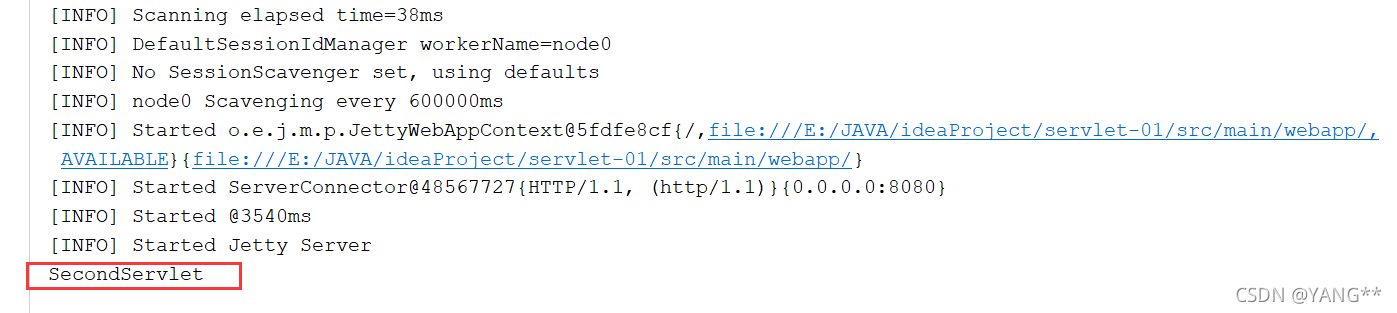
?
3、Servlet 的生命周期
????????Servlet 的生命周期,简单的概括这就分为四步:servlet 类加载 --> 初始化(init())--> 处理业务 --> 销毁(destroy())
4、Request 对象
4.1、Request 对象常用方法
package com.yjxxt.servlet;
?
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException;
?
@WebServlet(urlPatterns = "/Servlet")
public class Servlet extends HttpServlet {
? ?@Override
? ?protected void service(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
? ? ? ?super.service(req, resp);
?
? ? ? ?// 一个完整的请求地址,到 ? 结束
? ? ? ?StringBuffer url = req.getRequestURL();
? ? ? ?System.out.println(url.toString());
? ? ? ?// 获取请求的部分 URL(从站点名开始,到 ? 前面结束)
? ? ? ?String uri = req.getRequestURI();
? ? ? ?System.out.println(uri);
? ? ? ?// 获取请求地址中的参数
? ? ? ?String queryString = req.getQueryString();
? ? ? ?System.out.println(queryString);
? ? ? ?// 获取请求方式
? ? ? ?String method = req.getMethod();
? ? ? ?System.out.println(method);
? ? ? ?// 获取 HTTP 版本号
? ? ? ?String protocol = req.getProtocol();
? ? ? ?System.out.println(protocol);
? ? ? ?// 获取站点名
? ? ? ?String contextPath = req.getContextPath();
? ? ? ?System.out.println(contextPath);
? }
}
|