java实现html转pdf,使用freeMarker替换
最近公司有个需求,需要将一个html转化为pdf,不仅仅是单个html,而是一个模板,里面的值是能够替换的,废话不多说,直接上代码。
1.准备模板 说到模板,怎么能少了freeMarker,我就是利用freeMarker模板替换生成html的。 这个是模板内容,可根据自己的需求替换,注意此模板是.ftl格式。 注意:body里需要加入style="font-family: SimSun;"防止生成的pdf中文乱码
<body style="font-family: SimSun;">
<p style="text-align:center;font-size: 25px;letter-spacing:15px"><strong>${affairBasicInfo.name}</strong></p>
<p style="text-align:center;font-size: 25px;letter-spacing:15px"><strong>准考证</strong></p>
<table>
<tr>
<th>准考证编号</th>
<td colspan="3">${student.ticketNo}</td>
<td rowspan="5">照片</td>
</tr>
<tr>
<th>考生姓名</th>
<td>${student.studentUserName}</td>
<th>所在班级</th>
<td>${student.className}</td>
</tr>
<tr>
<th>学校/校区</th>
<td>${affairBasicInfo.schoolAndCampusName}</td>
<th>学年/学期</th>
<td>${affairBasicInfo.schoolYear}</td>
</tr>
<tr>
<th>考试类型</th>
<td>${affairBasicInfo.examTypeTxt}</td>
<th>考场名称</th>
<td>${student.roomName}</td>
</tr>
<tr>
<th>考场编号</th>
<td>${student.roomNo}</td>
<th>座位号</th>
<td>${student.seatNo}</td>
</tr>
<tr>
<th>序号</th>
<th>考试科目</th>
<th colspan="2">考试日期</th>
<th>考试时间</th>
</tr>
<#list subjectList as subject>
<tr>
<td>${subject.count}</td>
<td>${subject.subjectName}</td>
<td colspan="2">${subject.subjectExamDateTxt}</td>
<td>${subject.subjectExamStartTime}-${subject.subjectExamEndTime}</td>
</tr>
</#list>
<tr>
<td colspan="5">
${affairBasicInfo.remark}
</td>
</tr>
</table>
</body>
2、将模板内容替换并生成html文件 其中的第一个map集合就是你要替换的内容,ftl是你的模板文件, 最后一个就是你要生成html的文件存放在的位置
private String convert(Map<String, Object> context, String ftl, File dest) {
Writer pageJsOut = null;
try {
FileUtil.mkParentDirs(dest);
pageJsOut = new BufferedWriter(new OutputStreamWriter(new FileOutputStream(dest), "UTF-8"));
Template pageJsTemplate = freemarkerConfiguration.getTemplate(ftl);
pageJsTemplate.process(context, pageJsOut);
return dest.getAbsolutePath();
} catch (Exception e) {
throw new RuntimeException(e);
} finally {
if (pageJsOut != null) {
try {
pageJsOut.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
}
Map<String, Object> context = new HashMap<>();
context.put("affairBasicInfo", affairBasicInfo);
context.put("student", student);
context.put("subjectList", subjectList);
String tempHtmlPath = uploadDir + affairBasicInfo.getName() + "_html" + File.separator + affairBasicInfo.getGradeTxt() + File.separator + student.getClassName() + File.separator + student.getStudentUserName() + ".html";
String tempPdfPath = uploadDir + affairBasicInfo.getName() + "_pdf" + File.separator + affairBasicInfo.getGradeTxt() + File.separator + student.getClassName() + File.separator + student.getStudentUserName() + ".pdf";
this.convert(context, "gen/ticket.ftl", new File(tempHtmlPath));
其中的 freemarkerConfiguration是一个配置,导入bean
@Autowired
@Qualifier("freeMarkerCfg")
private Configuration freemarkerConfiguration;
xml中相关代码
<bean id="freeMarkerCfg" class="org.springframework.ui.freemarker.FreeMarkerConfigurationFactoryBean">
<property name="defaultEncoding" value="UTF-8" />
<property name="templateLoaderPaths">
<list>
<value>classpath:ftl/</value>
</list>
</property>
</bean>
<bean id="freemarkEngine" class="com.test.xx.util.FreemarkEngine">
<property name="configuration" ref="freeMarkerCfg"/>
</bean>
3、下面就是大家关心的html转pdf了 3.1、导入依赖
<dependency>
<groupId>org.xhtmlrenderer</groupId>
<artifactId>core-renderer</artifactId>
<version>R8</version>
<scope>compile</scope>
</dependency>
*3.2、生成pdf 根据上面生成的html,我们将它转化为pdf。这里传的第一个参数就是之前生成的html文件,后面是生成的pdf文件位置(自己定义)。
public static void html2pdf(String htmlFile, String pdfFile) throws Exception {
String url = new File(htmlFile).toURI().toURL().toString();
System.out.println(url);
File file = new File(pdfFile);
if (!file.getParentFile().exists()) {
file.getParentFile().mkdirs();
file.createNewFile();
}
OutputStream os = new FileOutputStream(file);
ITextRenderer renderer = new ITextRenderer();
renderer.setDocument(url);
ITextFontResolver fontResolver = renderer.getFontResolver();
if ("linux".equals(getCurrentOperatingSystem())) {
fontResolver.addFont("/usr/share/fonts/chiness/simsun.ttc", BaseFont.IDENTITY_H, BaseFont.EMBEDDED);
} else {
fontResolver.addFont("c:/Windows/Fonts/simsun.ttc", BaseFont.IDENTITY_H, BaseFont.NOT_EMBEDDED);
}
renderer.layout();
renderer.createPDF(os);
os.close();
System.out.println("create pdf done!!");
}
public static String getCurrentOperatingSystem() {
String os = System.getProperty("os.name").toLowerCase();
System.out.println("---------当前操作系统是-----------" + os);
return os;
}
看看效果 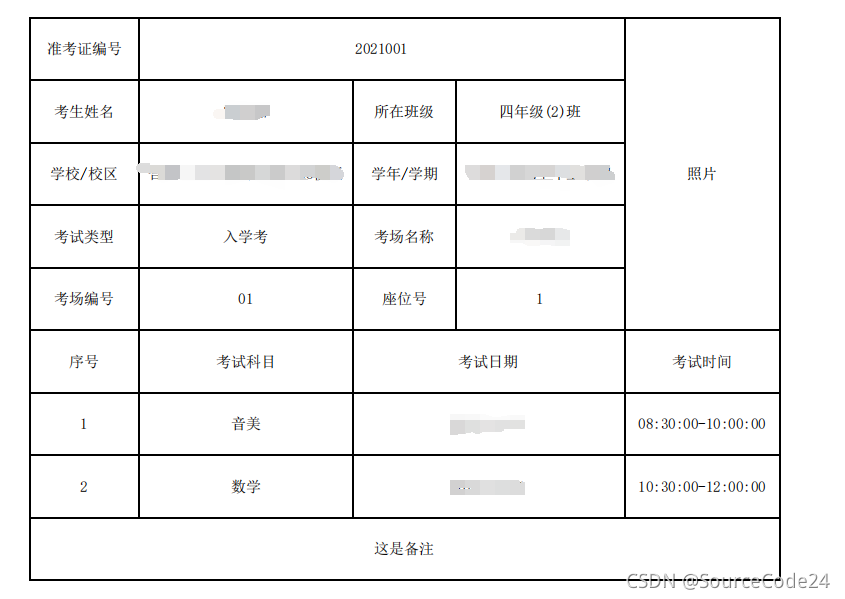
|