第一节 简单的Web应用搭建过程
1.1 Web项目开发需要准备的环境
- jdk:1.8版本以上(一般企业所用jdk版本都为1.8,少数使用11)
- Maven:3.6.3版本以上
- 开发辅助工具:IDEA
1.2 Web项目的开发步骤
1.2.1 Maven工程结构分析
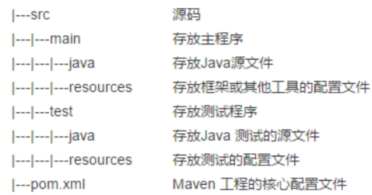 如下图所示: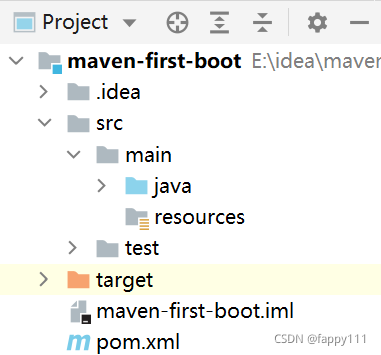
1.2.2第一个Spring Boot应用程序
1.2.2.1 检查环境配置
本节介绍如何开发一个小“Hello World!”,突出Spring Boot的一些关键功能的网络应用程序。我们使用Maven构建这个项目,因为大多数ide都支持它。
Spring.io网站包含许多使用Spring Boot的“入门”指南 可以通过start.spring.io/并从依赖项搜索器中选择“Web”启动器来快捷操作以下步骤。这样做会产生一个新的项目结构,以便可以立即开始编码。有关更多详细信息,请查看start.spring.io用户指南
在我们开始之前,打开一个终端并运行以下命令,以确保机器上安装了有效的java和maven版本:  
1.2.2.2 IDEA创建工程与POM
使用Maven构建Web工程项目的相关骨架 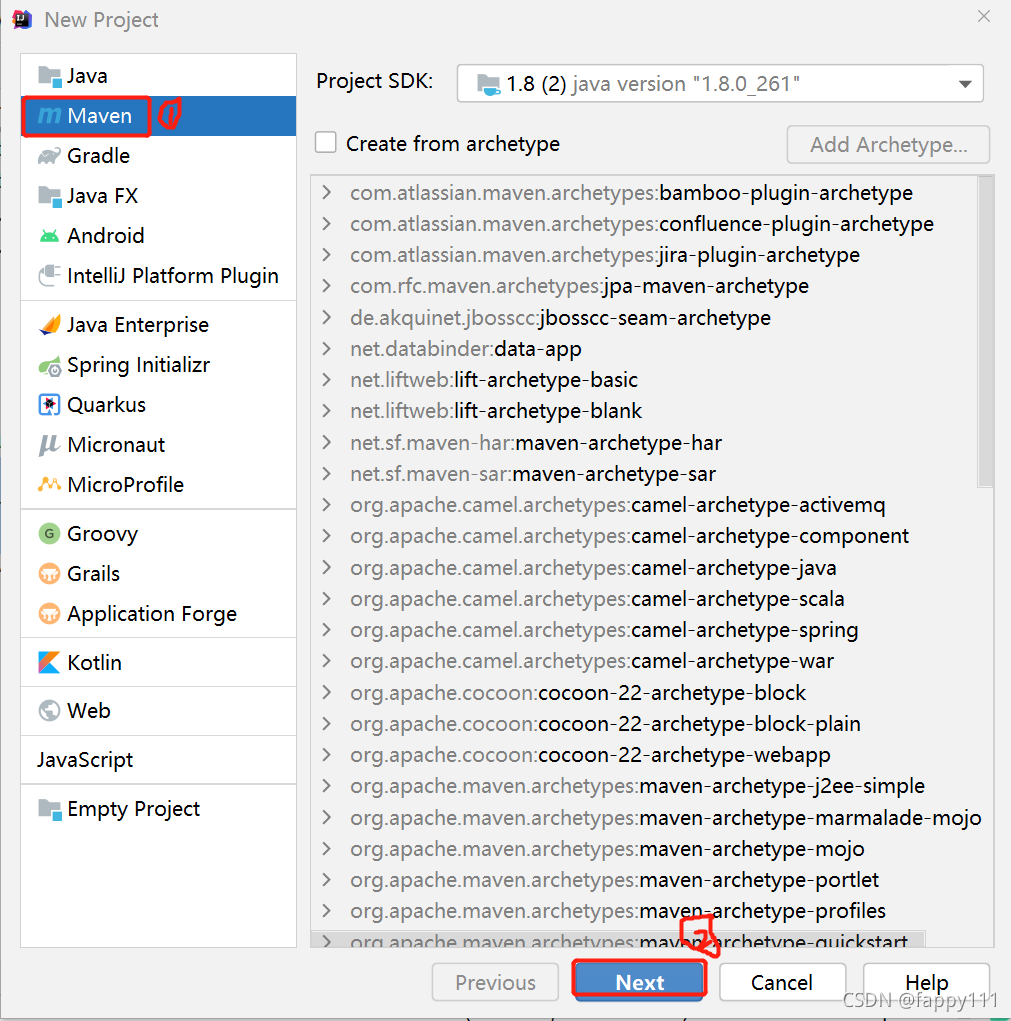 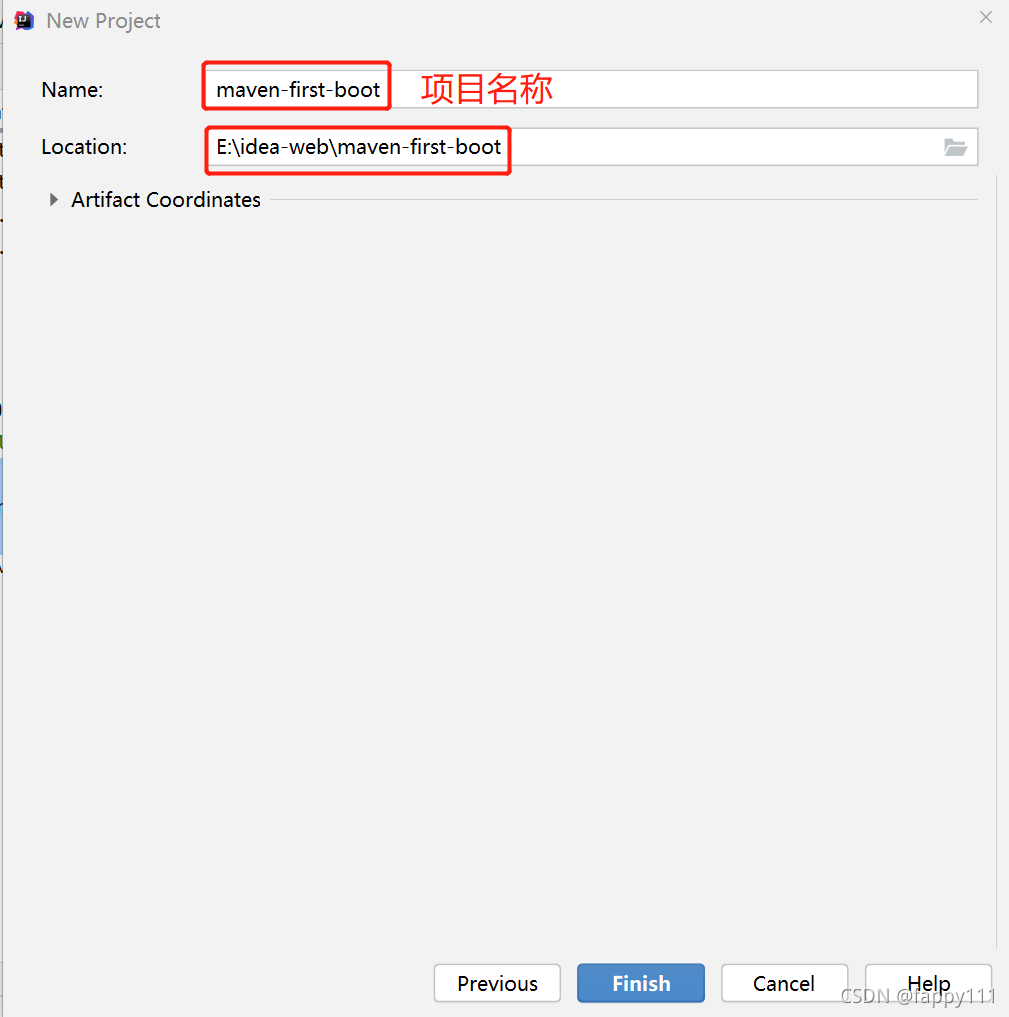 我们需要从创建一个Maven pom.xml文件开始。pom.xml是用于构建项目的依据。
1.2.2.3 添加类路径依赖项
如下所示:
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.edu.zut</groupId>
<artifactId>maven-first-boot</artifactId>
<version>1.0-SNAPSHOT</version>
<properties>
<maven.compiler.source>8</maven.compiler.source>
<maven.compiler.target>8</maven.compiler.target>
</properties>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.4.1</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
1.2.2.4编写代码
为了完成我们的应用程序,我们需要创建一个单独的java文件。默认情况下,Maven从src/main/java编译源文件,因此我们需要创建该目录结构,如何添加一个名为src/main/java/MyApplication.java的文件,以包含以下代码:
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.EnableAutoConfiguration;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
@EnableAutoConfiguration
public class MyApplication {
RequestMapping("/hello")
String home() {
return "Hello World!";
}
public static void main(String[] args) {
SpringApplication.run(MyApplication.class, args);
}
}
- @RestController和@RequestMapping注释
MyApplication 类上的第?个注释是 @RestController 。这被称为原型注释。它为阅读代码的?和 Spring 提供了类扮演特定??的提示。在本例中,我们的类是?个web @Controller ,因此Spring在处理传?的web请求时会考虑它。 @RequestMapping 注释提供“路由”信息。它告诉Spring,任何带有 / path的HTTP请求都应该映射到home?法。 @RestController 注释告诉Spring将结果字符串直接呈现给调?者。 @RestController 和 @RequestMapping 注释是Spring MVC注释(它们不是Spring Boot特有的)。
- @EnableAutoConfiguration 注释
第?类级注释是 @EnableAutoConfiguration 。此注释告诉Spring Boot根据我们添加的jar依赖项“猜测”您想要如 何配置Spring。由于 spring-boot-starter-web 添加了Tomcat和Spring MVC,?动配置假设您正在开发?个Web应?程序,并相应地设置Spring。
启动器和?动配置 ?动配置是为了配合“??”?设计的,但这两个概念并没有直接绑定。您可以?由选择起动器以外的罐?依赖项。Spring Boot仍然尽最?努??动配置应?程序。
我们应?程序的最后?部分是 main ?法。 对于应?程序??点,这是?个遵循 Java 约定的标准?法。我们的主要?法通过调? run 来委托 SpringBoot 的 SpringApplication 类。 SpringApplication 引导我们的应?程序,启动 Spring ,然后启动?动配置的 Tomcat Web服务器。我们需要将 MyApplication.class 作为参数传递给 run ?法,以告知 SpringApplication 哪个是主要的 Spring 组件。 args 数组也被传递以公开任何命令?参数。
1.2.2.5 运?项?
此时,您的应?程序应该可以?作了。直接运?main?法就可以启动web项?了。
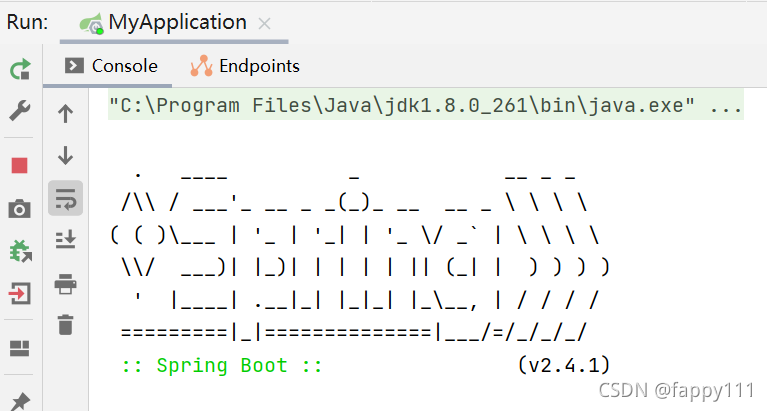 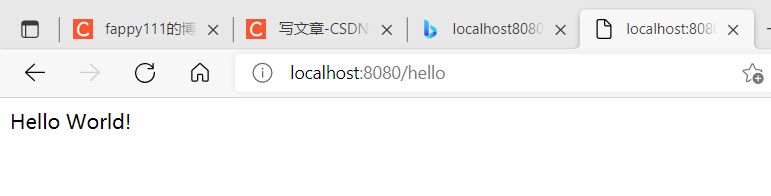
1.2.3 打jar包运行的方法
一、添加依赖 要创建可执?jar,我们需要将spring-boot-maven-plugin添加到pom.xml中。要执?此操作,请在dependencies 部分下?插?以下?: Save your pom.xml and run mvn package from the command line, as follows:
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
</plugin>
</plugins>
</build>
二、 打包 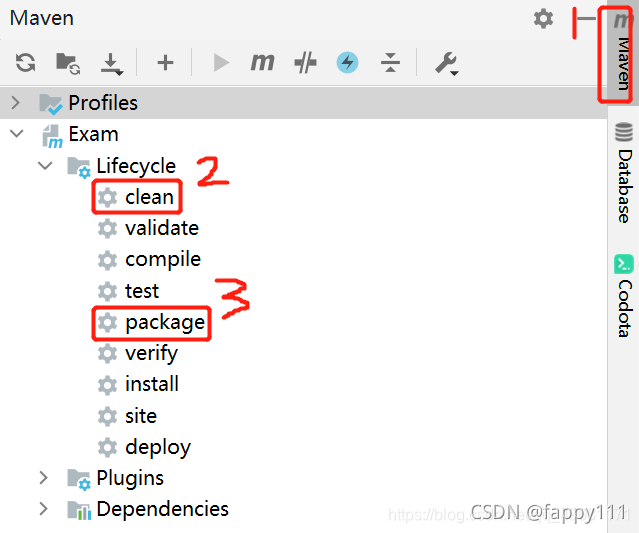
三、 运行 方法一:
C:\Users\开开·的·MagicBook>E:
E:\>cd idea/maven-first-boot/target
E:\idea\maven-first-boot\target>dir
驱动器 E 中的卷是 新加卷
卷的序列号是 C0EC-EAA6
E:\idea\maven-first-boot\target 的目录
2021/09/04 21:12 <DIR> .
2021/09/04 21:12 <DIR> ..
2021/09/04 21:11 <DIR> classes
2021/09/04 21:11 <DIR> generated-sources
2021/09/04 21:11 <DIR> generated-test-sources
2021/09/04 21:11 <DIR> maven-archiver
2021/09/04 21:12 16,997,960 maven-first-boot-1.0-SNAPSHOT.jar
2021/09/04 21:11 2,236 maven-first-boot-1.0-SNAPSHOT.jar.original
2021/09/04 21:11 <DIR> maven-status
2021/09/04 21:11 <DIR> test-classes
2 个文件 17,000,196 字节
8 个目录 106,409,267,200 可用字节
E:\idea\maven-first-boot\target>java -jar maven-first-boot-1.0-SNAPSHOT.jar
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.4.1)
2021-09-04 21:28:13.327 INFO 12604 --- [ main] MyApplication : Starting MyApplication using Java 1.8.0_261 on LAPTOP-MRM1O9TE with PID 12604 (E:\idea\maven-first-boot\target\maven-first-boot-1.0-SNAPSHOT.jar started by 开开·的·MagicBook in E:\idea\maven-first-boot\target)
2021-09-04 21:28:13.330 INFO 12604 --- [ main] MyApplication : No active profile set, falling back to default profiles: default
2021-09-04 21:28:14.342 INFO 12604 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2021-09-04 21:28:14.354 INFO 12604 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2021-09-04 21:28:14.355 INFO 12604 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.41]
2021-09-04 21:28:14.417 INFO 12604 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2021-09-04 21:28:14.417 INFO 12604 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 1045 ms
2021-09-04 21:28:14.595 INFO 12604 --- [ main] o.s.s.concurrent.ThreadPoolTaskExecutor : Initializing ExecutorService 'applicationTaskExecutor'
2021-09-04 21:28:14.771 INFO 12604 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2021-09-04 21:28:14.783 INFO 12604 --- [ main] MyApplication : Started MyApplication in 1.848 seconds (JVM running for 2.245)
2021-09-04 21:28:23.234 INFO 12604 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2021-09-04 21:28:23.234 INFO 12604 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2021-09-04 21:28:23.237 INFO 12604 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 2 ms
方法二:
E:\idea\maven-first-boot>mvn spring-boot:run
[INFO] Scanning for projects...
[INFO]
[INFO] --------------------< cn.edu.zut:maven-first-boot >---------------------
[INFO] Building maven-first-boot 1.0-SNAPSHOT
[INFO] --------------------------------[ jar ]---------------------------------
[INFO]
[INFO] >>> spring-boot-maven-plugin:2.4.1:run (default-cli) > test-compile @ maven-first-boot >>>
[INFO]
[INFO] --- maven-resources-plugin:3.2.0:resources (default-resources) @ maven-first-boot ---
[INFO] Using 'UTF-8' encoding to copy filtered resources.
[INFO] Using 'UTF-8' encoding to copy filtered properties files.
[INFO] Copying 0 resource
[INFO] Copying 0 resource
[INFO]
[INFO] --- maven-compiler-plugin:3.8.1:compile (default-compile) @ maven-first-boot ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] --- maven-resources-plugin:3.2.0:testResources (default-testResources) @ maven-first-boot ---
[INFO] Using 'UTF-8' encoding to copy filtered resources.
[INFO] Using 'UTF-8' encoding to copy filtered properties files.
[INFO] skip non existing resourceDirectory E:\idea\maven-first-boot\src\test\resources
[INFO]
[INFO] --- maven-compiler-plugin:3.8.1:testCompile (default-testCompile) @ maven-first-boot ---
[INFO] Nothing to compile - all classes are up to date
[INFO]
[INFO] <<< spring-boot-maven-plugin:2.4.1:run (default-cli) < test-compile @ maven-first-boot <<<
[INFO]
[INFO]
[INFO] --- spring-boot-maven-plugin:2.4.1:run (default-cli) @ maven-first-boot ---
[INFO] Attaching agents: []
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.4.1)
2021-09-04 21:32:30.463 INFO 16660 --- [ main] MyApplication : Starting MyApplication using Java 1.8.0_261 on LAPTOP-MRM1O9TE with PID 16660 (E:\idea\maven-first-boot\target\classes started by 开开·的 ·MagicBook in E:\idea\maven-first-boot)
2021-09-04 21:32:30.465 INFO 16660 --- [ main] MyApplication : No active profile set, falling back to default profiles: default
2021-09-04 21:32:31.089 INFO 16660 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2021-09-04 21:32:31.096 INFO 16660 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2021-09-04 21:32:31.097 INFO 16660 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.41]
2021-09-04 21:32:31.142 INFO 16660 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2021-09-04 21:32:31.143 INFO 16660 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 648 ms
2021-09-04 21:32:31.246 INFO 16660 --- [ main] o.s.s.concurrent.ThreadPoolTaskExecutor : Initializing ExecutorService 'applicationTaskExecutor'
2021-09-04 21:32:31.348 INFO 16660 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2021-09-04 21:32:31.355 INFO 16660 --- [ main] MyApplication : Started MyApplication in 1.116 seconds (JVM running for 1.373)
2021-09-04 21:32:40.590 INFO 16660 --- [nio-8080-exec-1] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring DispatcherServlet 'dispatcherServlet'
2021-09-04 21:32:40.591 INFO 16660 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : Initializing Servlet 'dispatcherServlet'
2021-09-04 21:32:40.593 INFO 16660 --- [nio-8080-exec-1] o.s.web.servlet.DispatcherServlet : Completed initialization in 0 ms
|