开发工具准备
开发工具:idea2017 以上 依赖管理:maven3 以上 jdk:1.8 以上 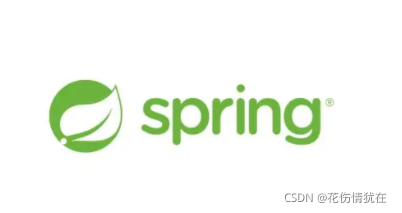
使用Maven创建Spring项目
- 选择Maven
- 勾选Create from archetype
- 选择maven-archetype-quickstart
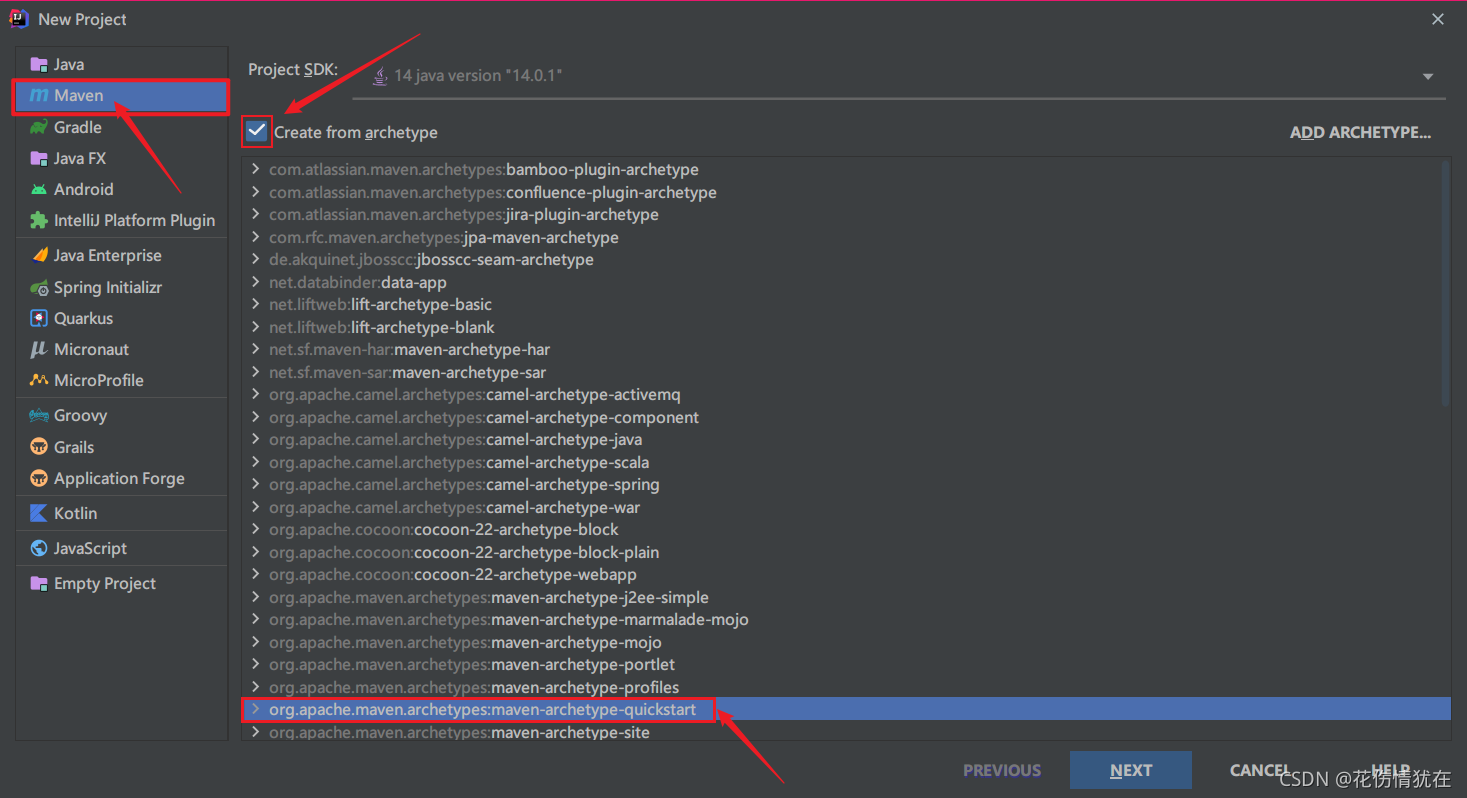 填写项目信息 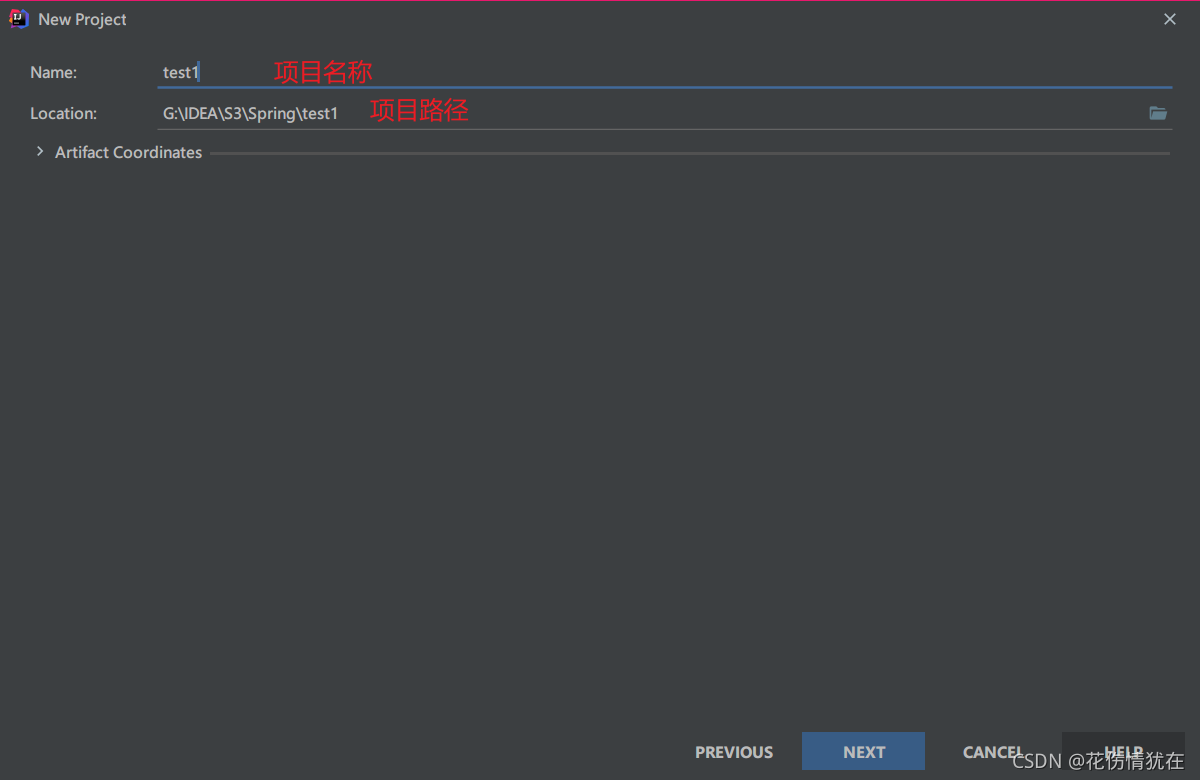 指定maven 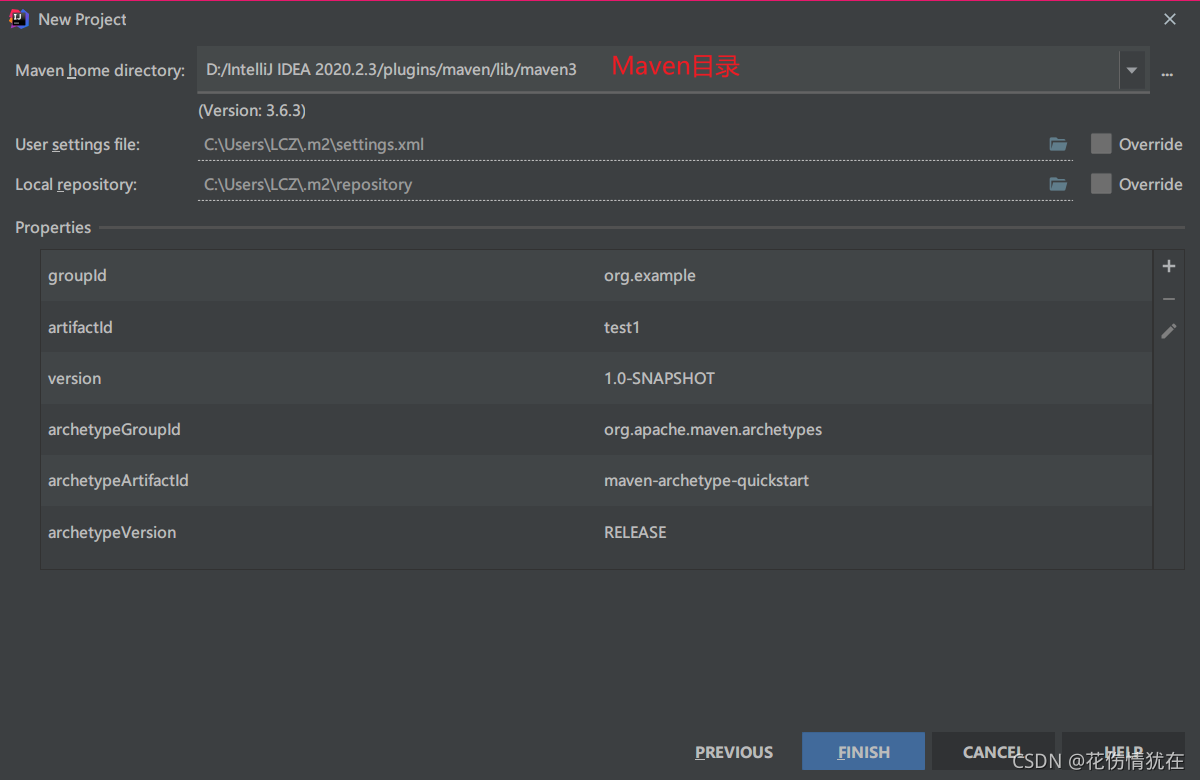 创建成功 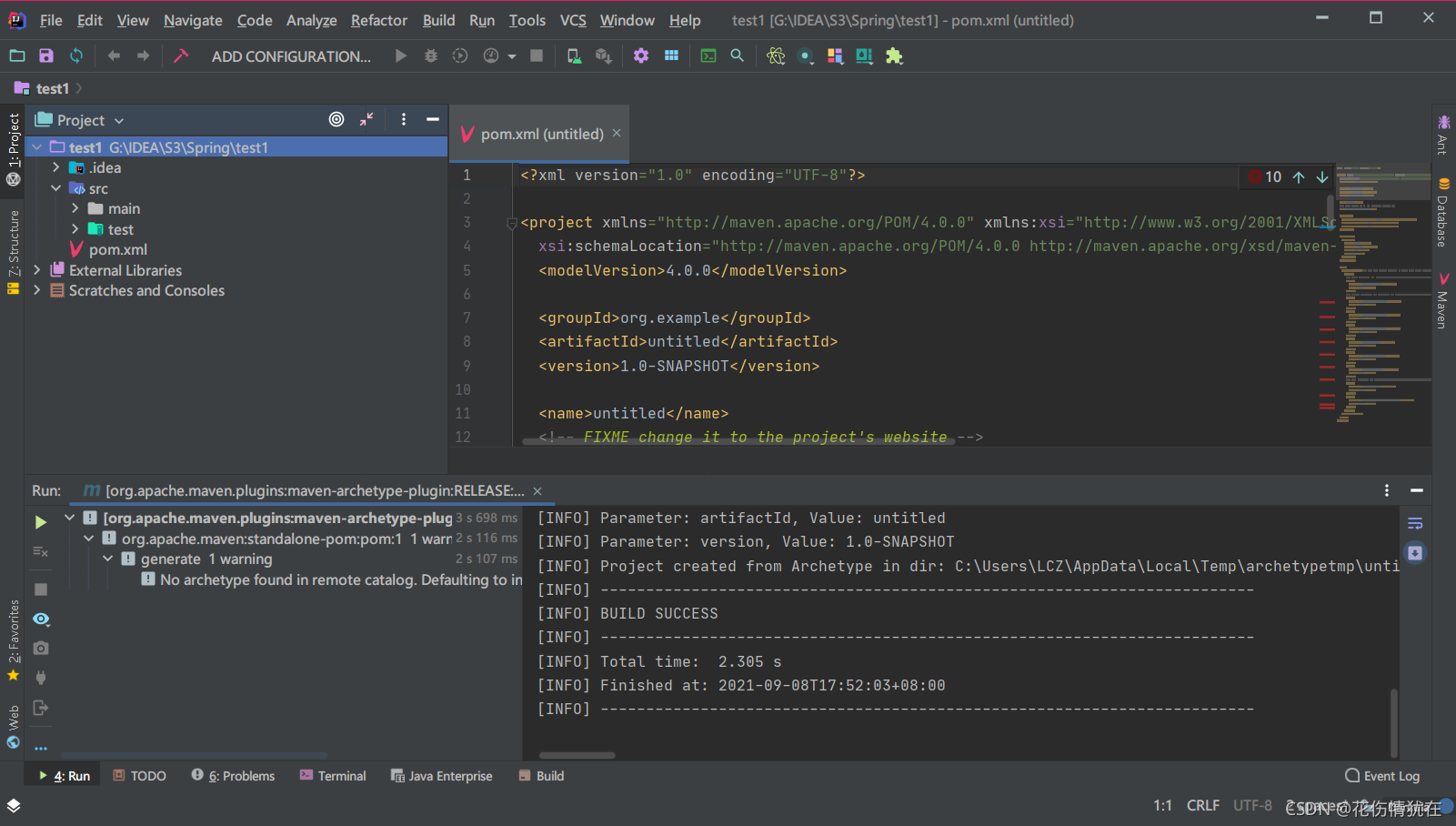
使用Java Enterprise创建Spring项目
默认即可 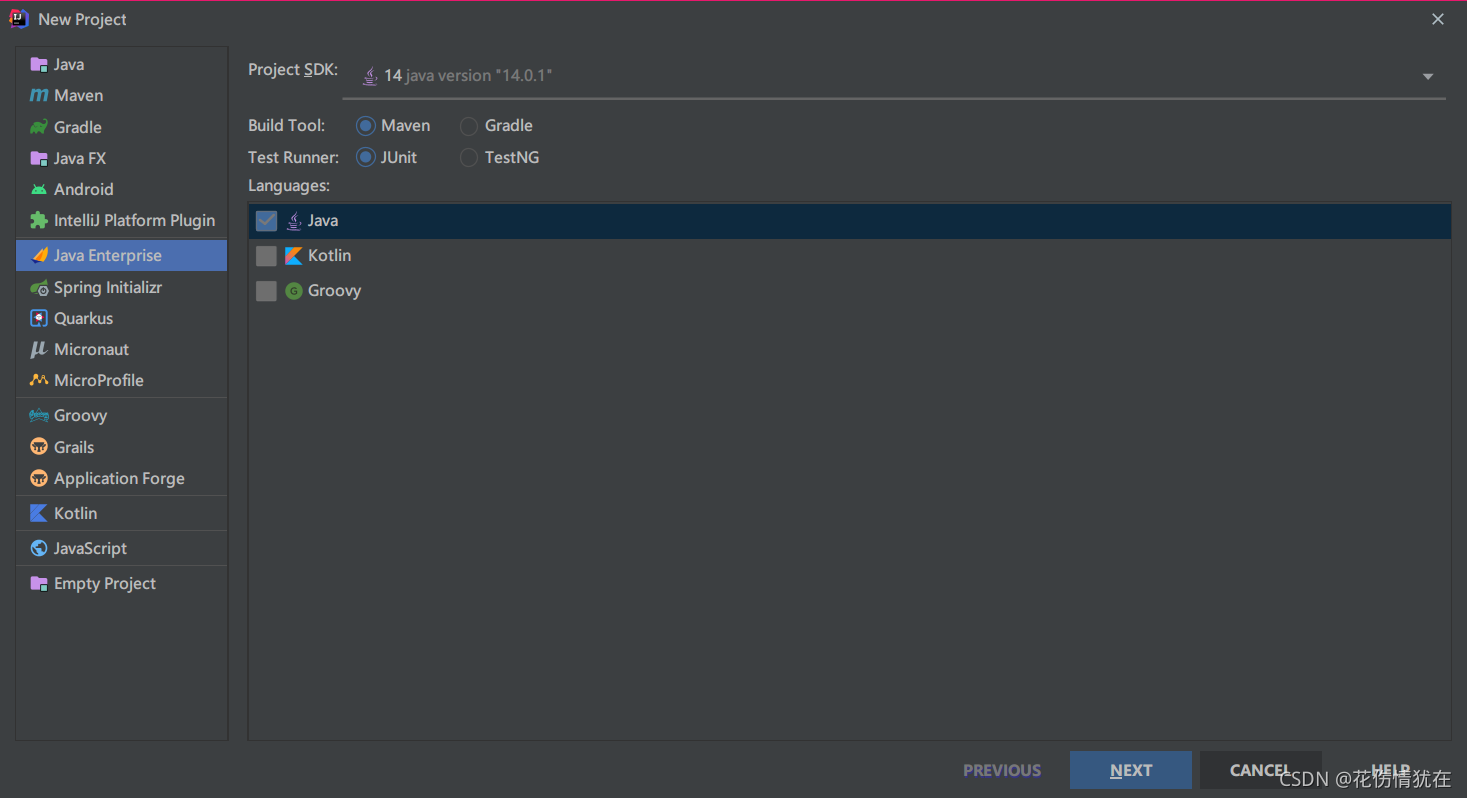 Version一般选择Java EE 8 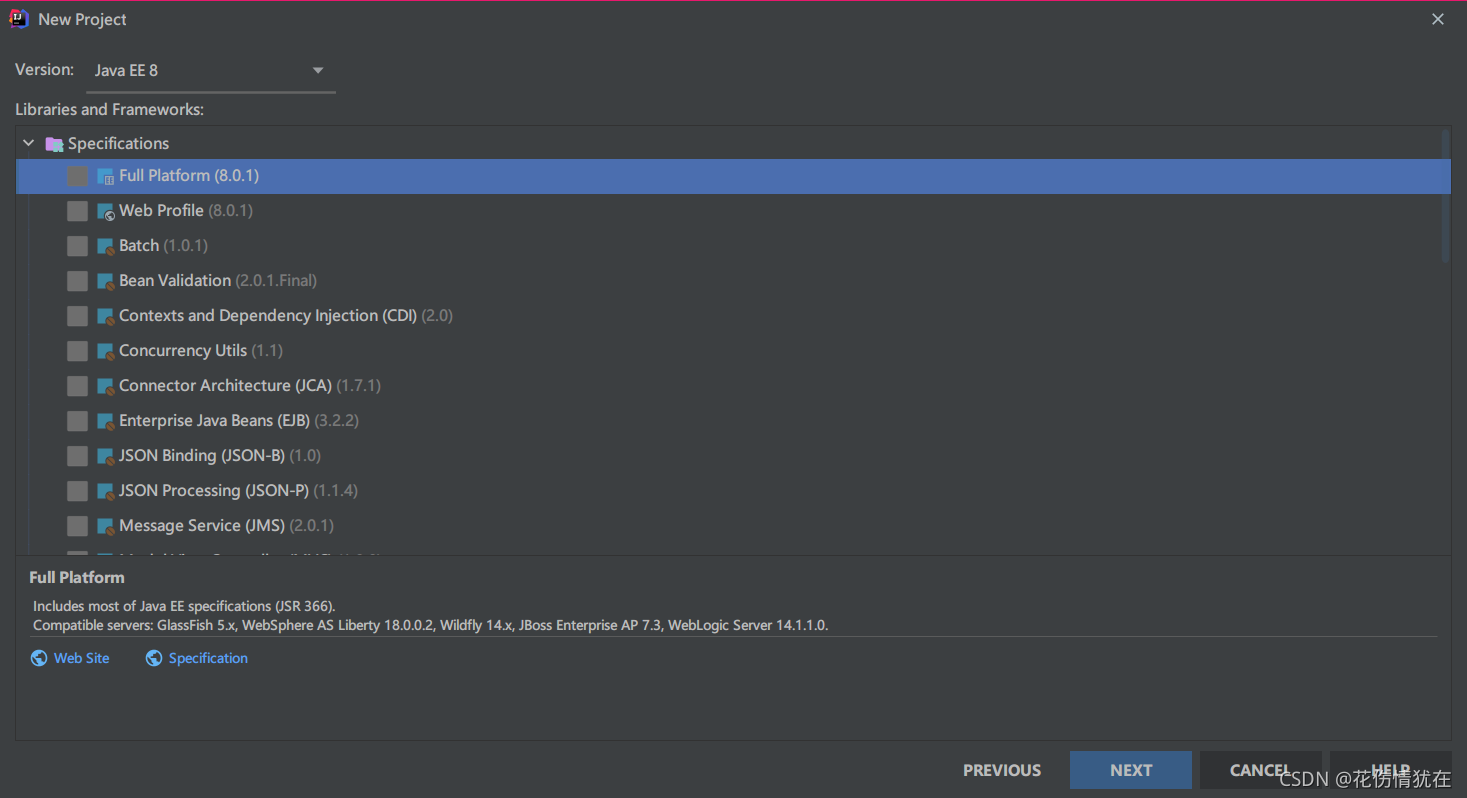 设置项目信息 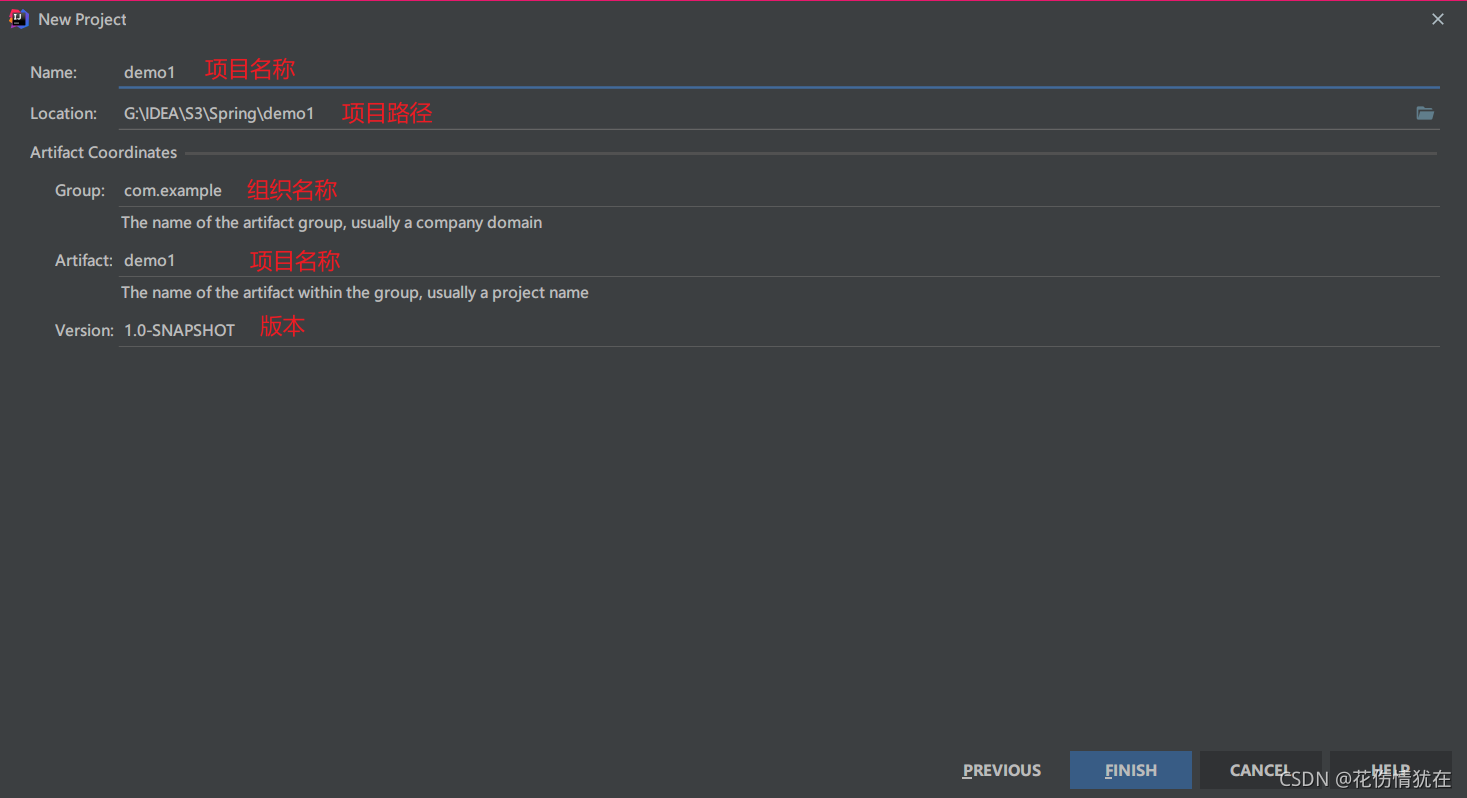 创建成功 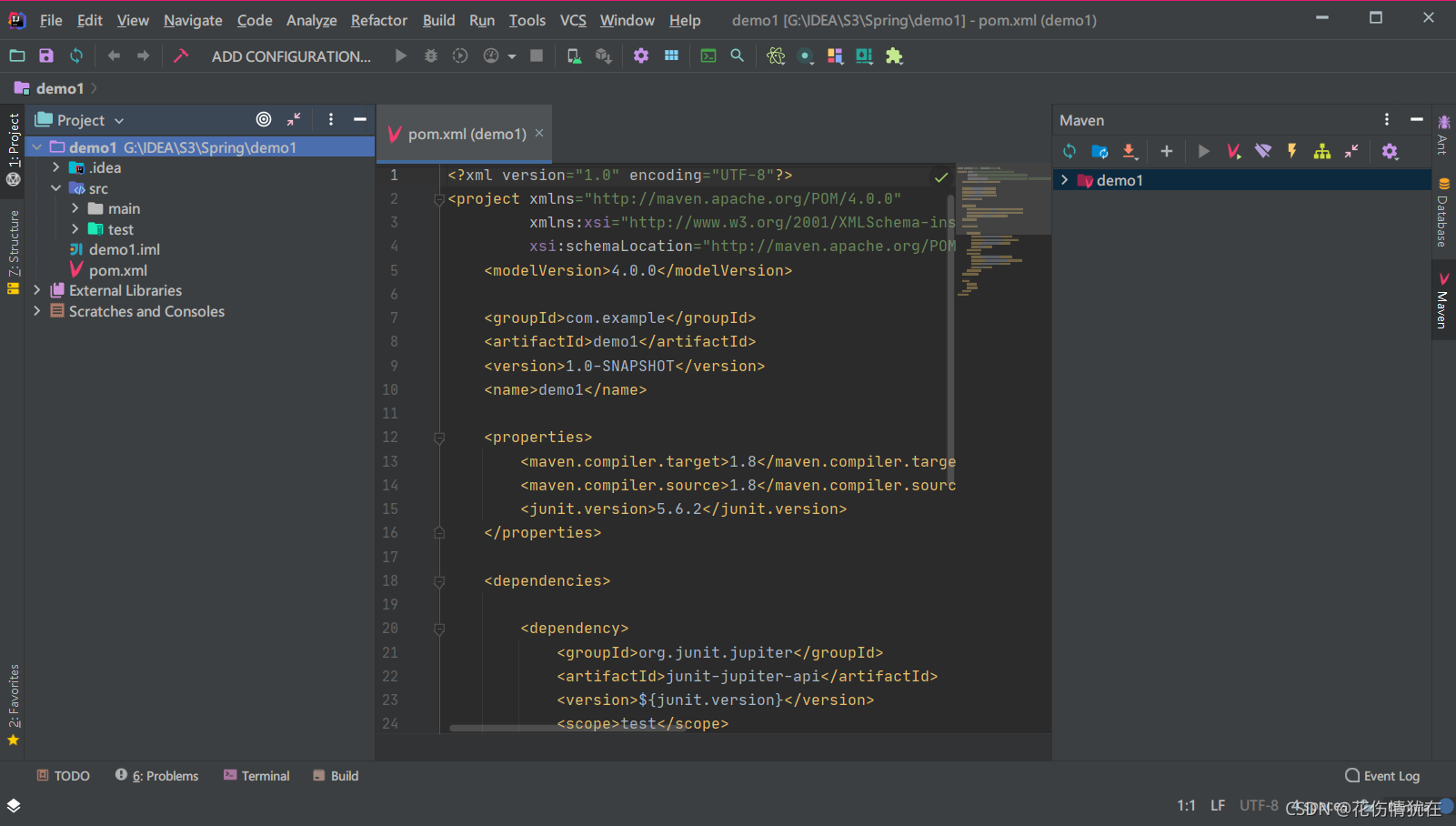
引入 maven 依赖 pom.xml
打开pom文件添加依赖
<dependency>
<groupId>log4j</groupId>
<artifactId>log4j</artifactId>
<version>1.2.17</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-context</artifactId>
<version>5.0.3.RELEASE</version>
</dependency>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
刷新 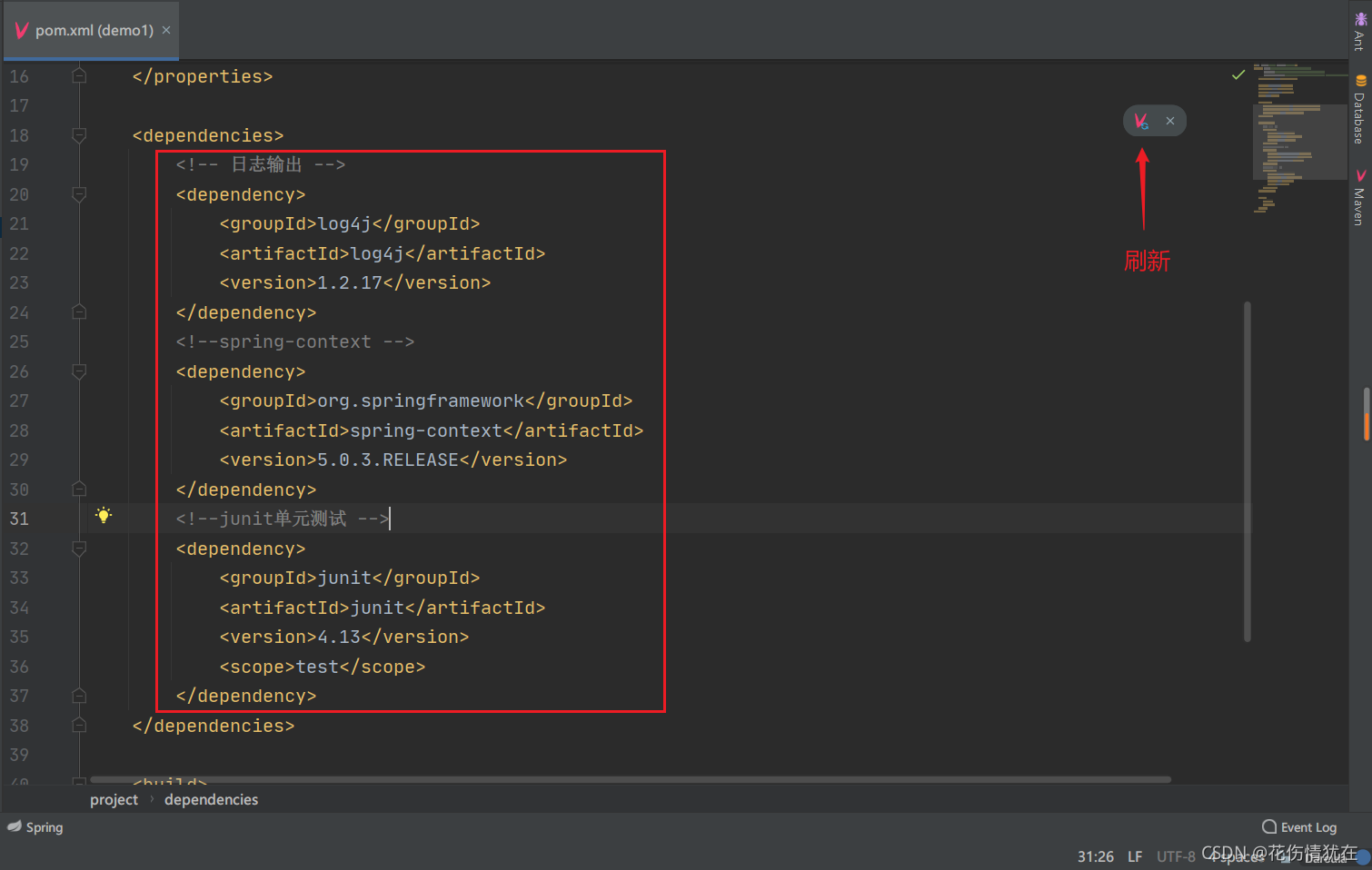 检查依赖 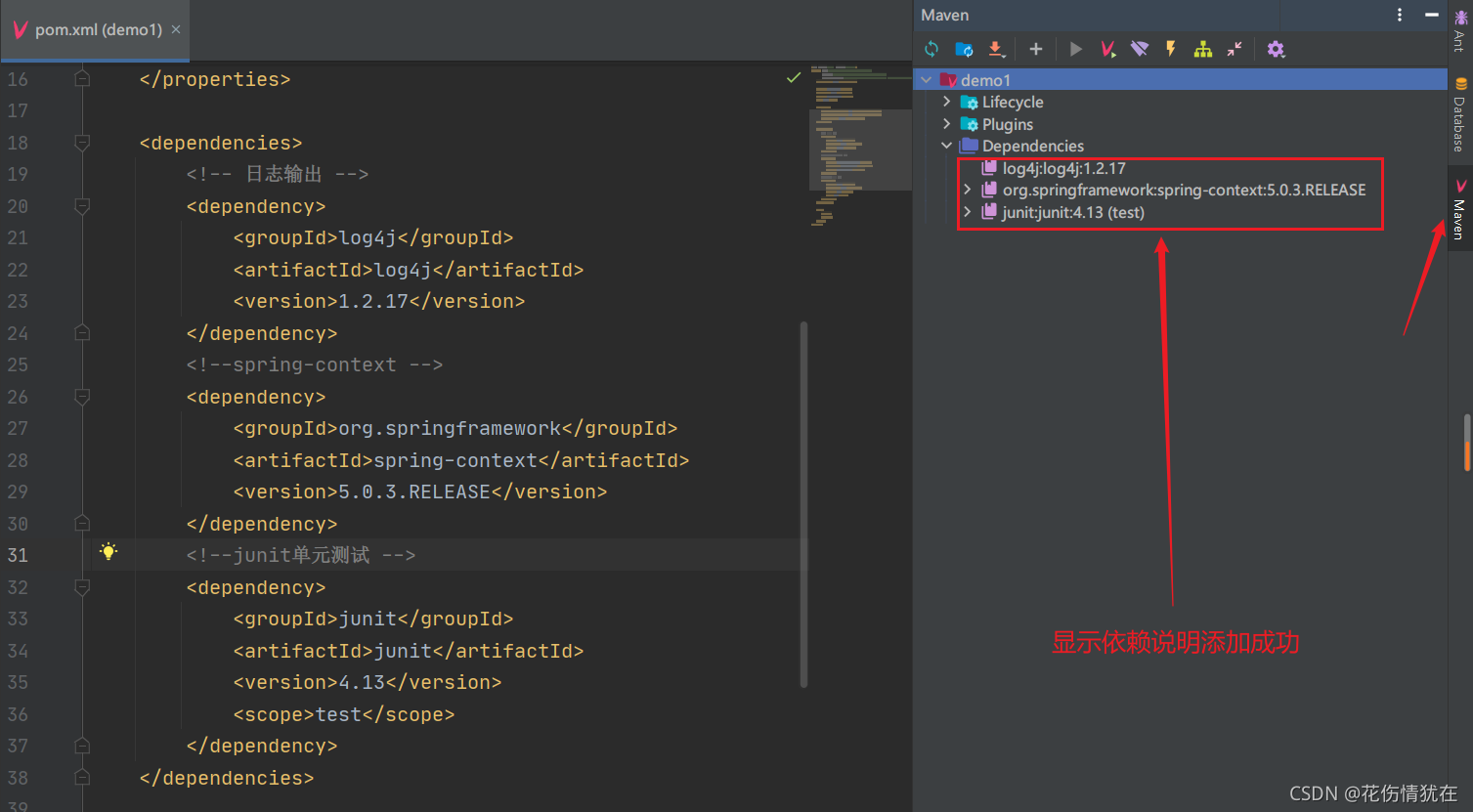
定义接口与实体类
接口
public interface SomeService {
void doSome();
}
实现类
public class SomeServiceImpl implements SomeService {
public SomeServiceImpl() {
System.out.println("SomeServiceImpl无参数构造方法");
}
@Override
public void doSome() {
System.out.println("====业务方法doSome()===");
}
}
创建 Spring 配置文件
在 src/main/resources/ 目录现创建一个 xml 文件,文件名可以随意,但 Spring 建议的名称为 applicationContext.xml 。
注意:spring 配置中需要加入约束文件才能正常使用,约束文件是 xsd 扩展名。
IDEA工具默认提供Spring模板 选中resources包>New>XML Confiquration File >Spring Config 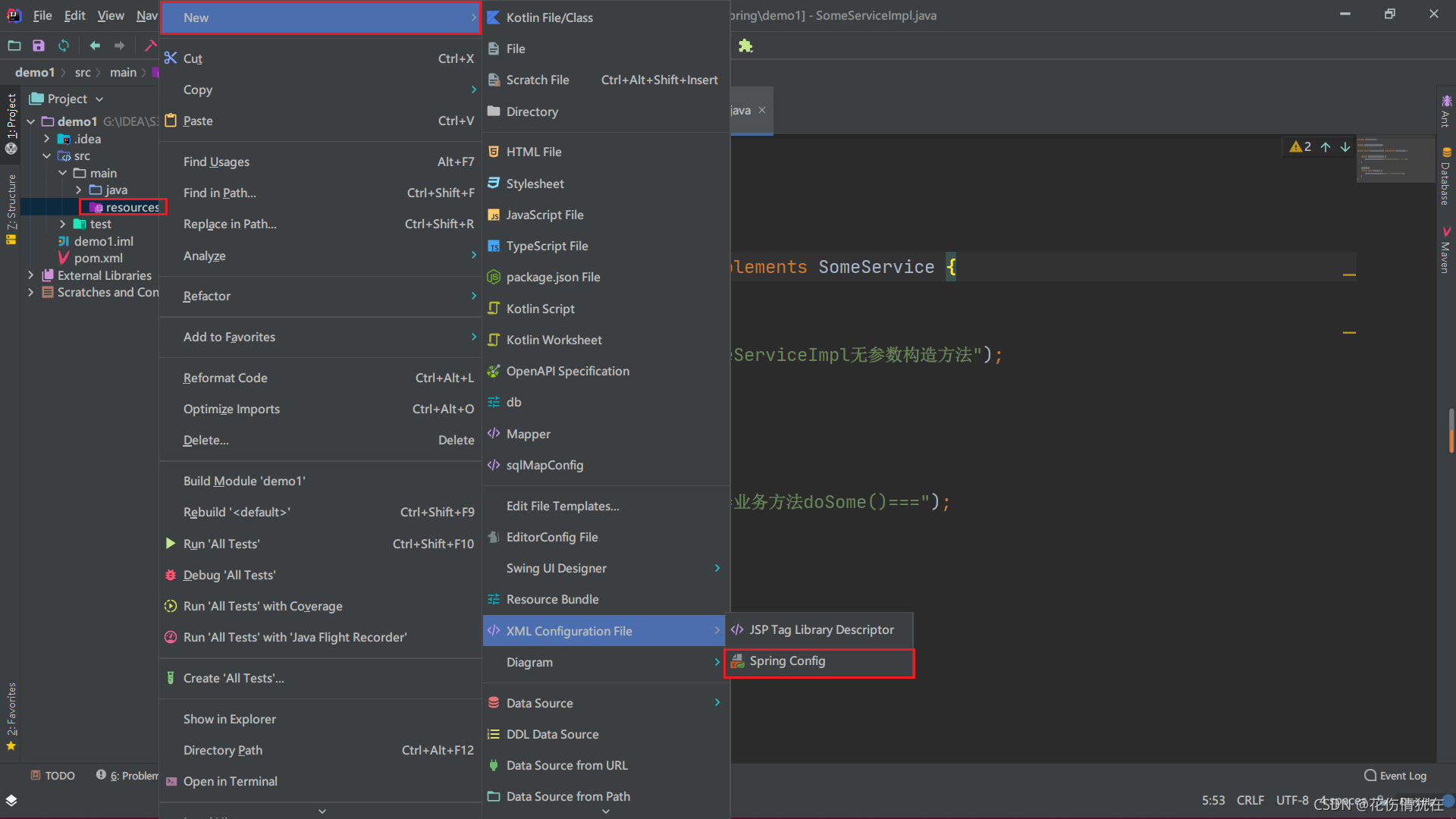 创建成功 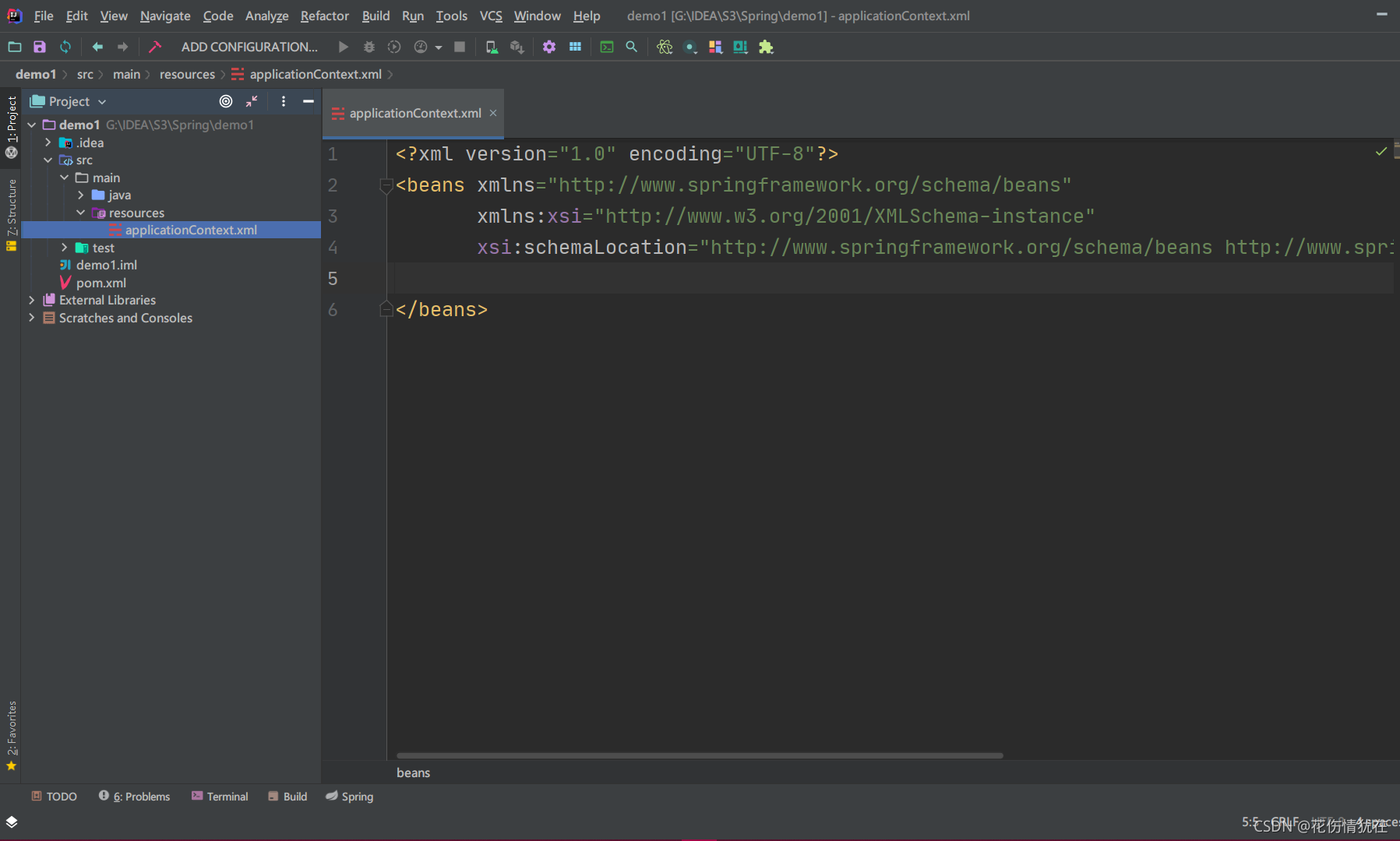 默认模板说明
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
</beans>
spring标准的配置文件:
1)根标签是 beans
2) beans 后面的是约束文件说明
3)beans里面是bean声明。
4)什么是bean: bean就是java对象, spring容器管理的java对象,叫做bean
注册bean对象
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="someService" class="service.Impl.SomeServiceImpl"></bean>
</beans>
- bean标签:用于定义一个实例对象。一个实例对应一个 bean 元素。
- id:该属性是 Bean 实例的唯一标识,程序通过 id 属性访问 Bean,Bean
与 Bean 间的依赖关系也是通过 id 属性关联的。 - class:指定该 Bean 所属的类,注意这里只能是类,不能是接口。
定义测试类
测试类定义在根目录下的test 包下的java 包里面
这里我们创建一个test1(名称任意)测试类 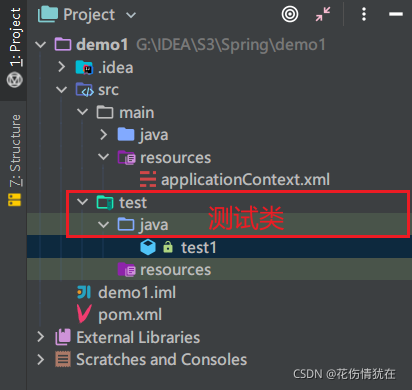
spring代理SomeServiceImpl对象
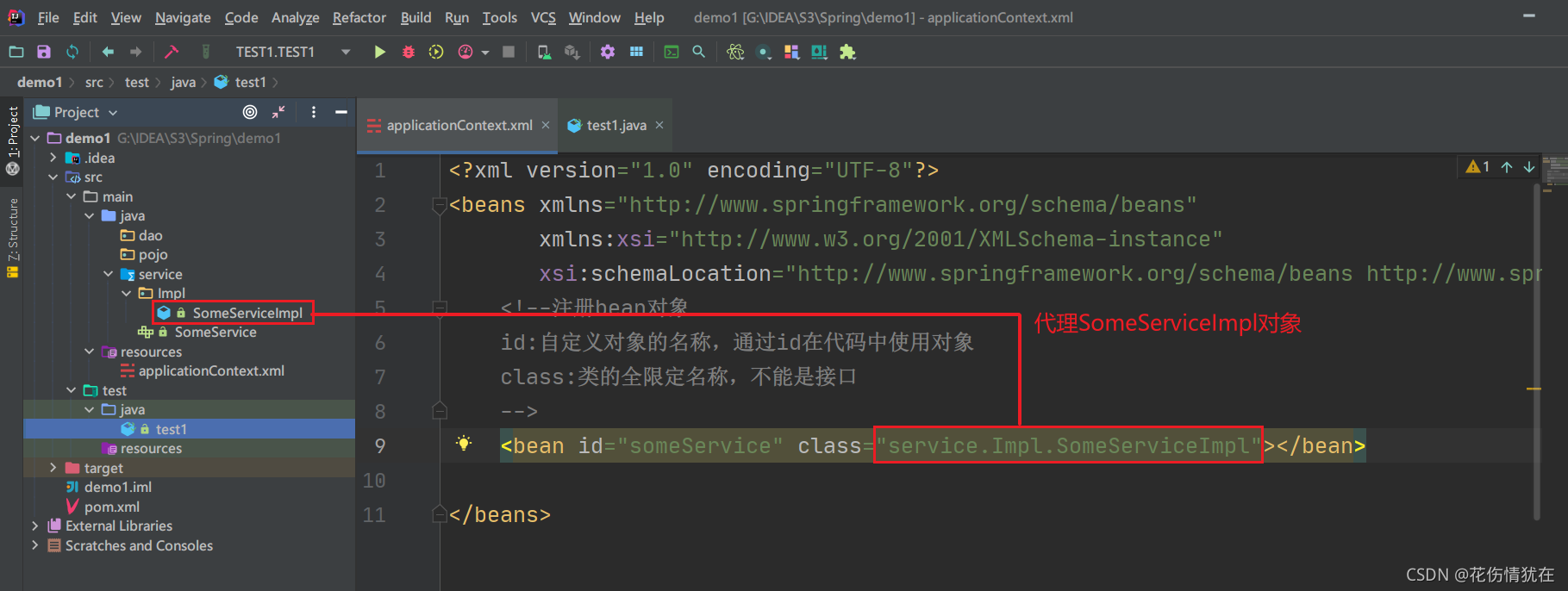
测试spring容器中代理someServiceImpl对象
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import service.SomeService;
public class test1 {
@Test
public void test1(){
String resources = "applicationContext.xml";
ApplicationContext applicationContext = new ClassPathXmlApplicationContext(resources);
SomeService someService = (SomeService)applicationContext.getBean("someService");
someService.doSome();
}
}
成功运行spring代理的someServiceImpl对象
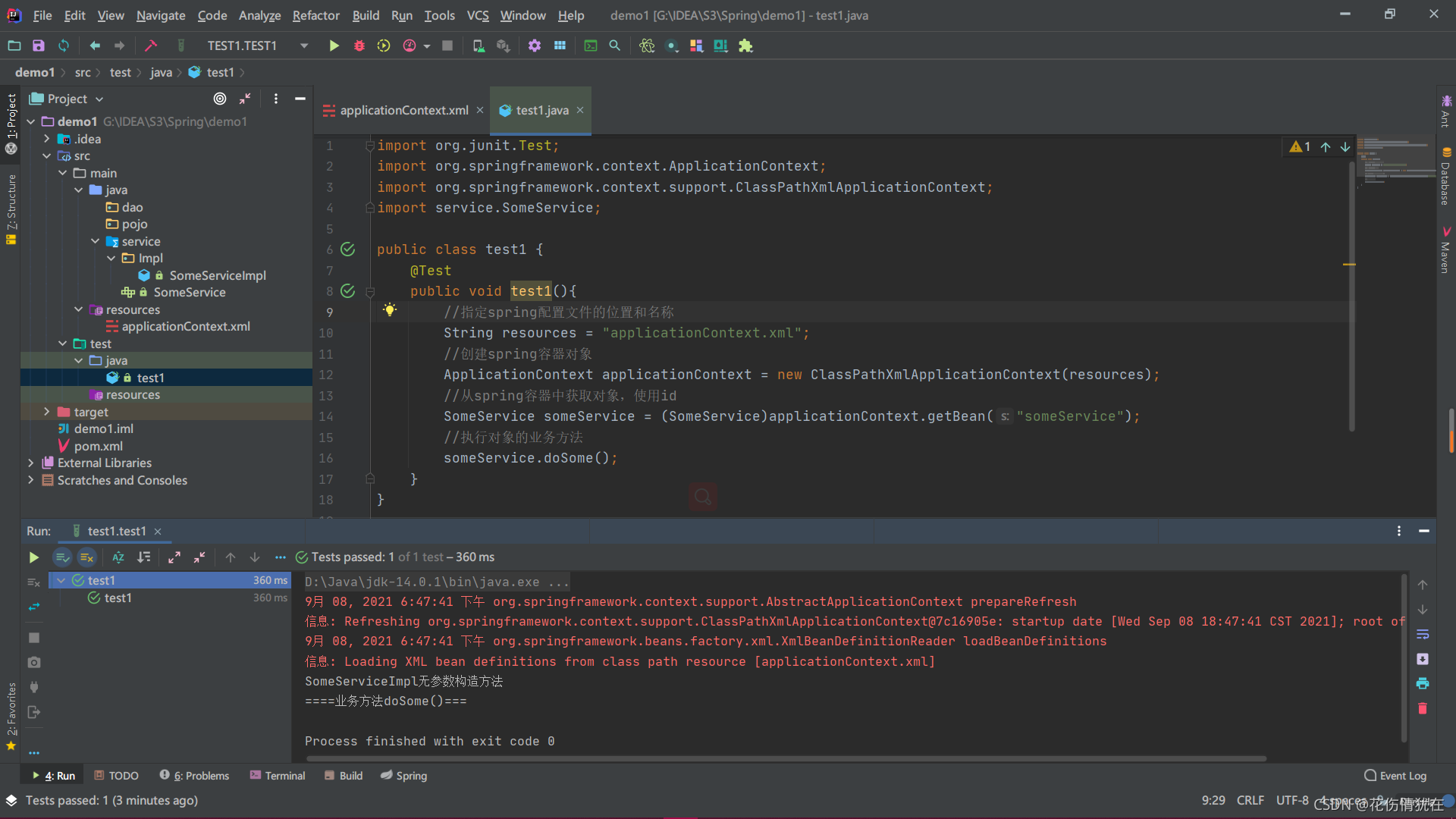
使用 spring 创建非自定义类对象
简单来说就是spring可以创建自定义的对象,也可以创建非自定义的对象。
上面spring代理创建的对象就是我们自定义的对象,我们可以通过spring代理非自定义的对象,例如java下的日期类(Date):
spring 配置文件加入 java.util.Date 定义:
<bean id="myDate" class="java.util.Date" />
如图 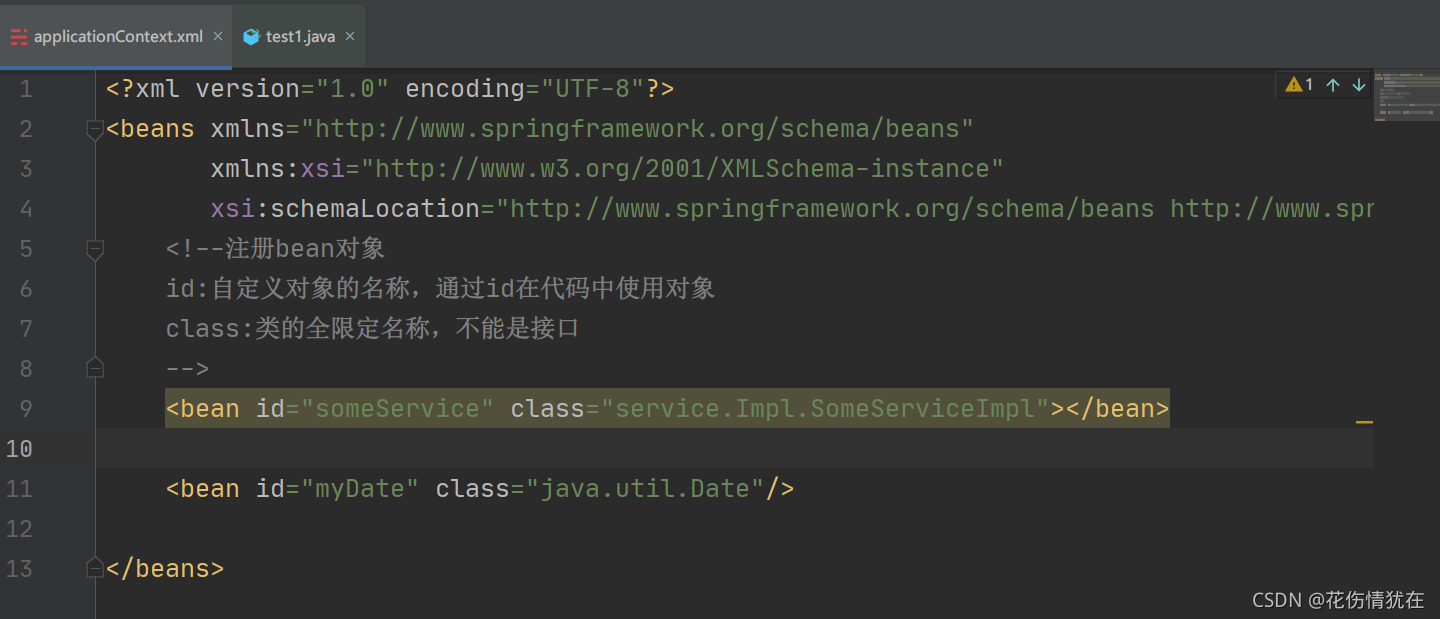 test1 测试类中: 调用 getBean(“myDate”); 获取日期类对象。 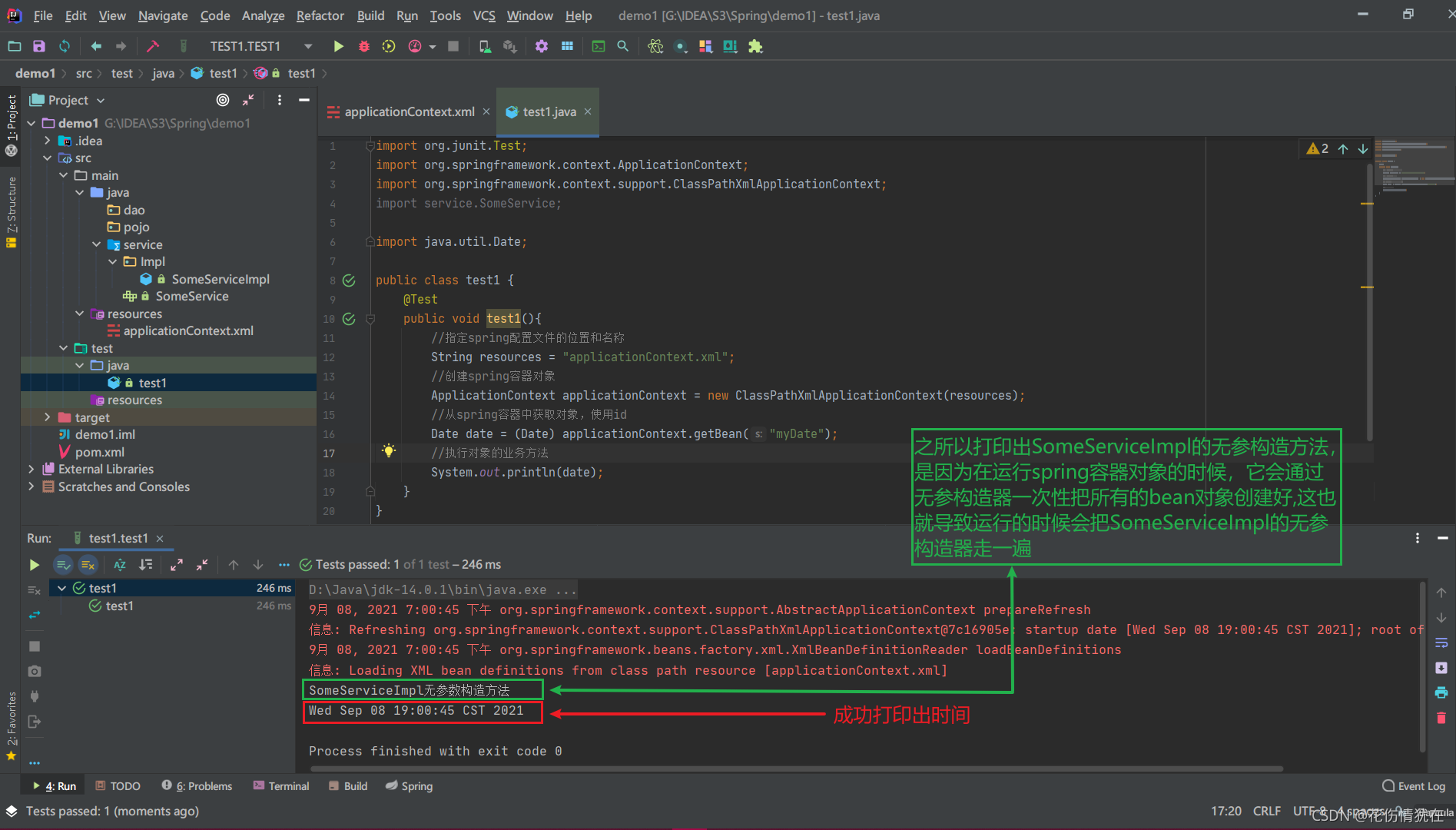
容器接口和实现类
ApplicationContext 接口(容器)
ApplicationContext 用于加载 Spring 的配置文件,在程序中充当“容 器”的角色。其实现类有两个: 使用Ctrl+H可以查看类的继承关系 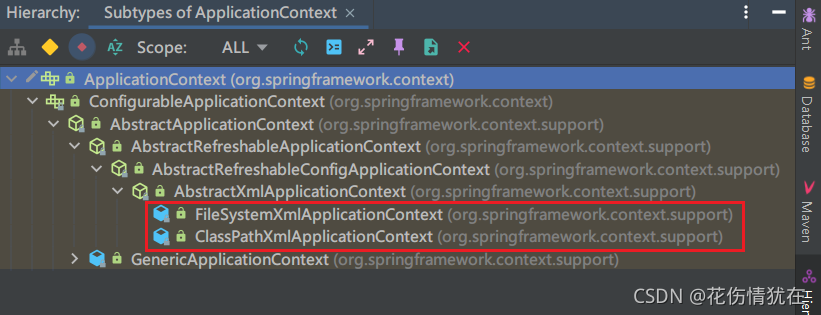 ClassPathXmlApplicationContext和FileSystemXmlApplicationContext的区别
ClassPathXmlApplicationContext[只能读放在web-info/classes目录下的配置文件]
- 默认就是指项目的classpath路径下面
- 如果要使用绝对路径,需要加上file:前缀表示这是绝对路径
使用ClassPathXmlApplicationContext指定spring配置文件位置
默认就是指项目的classpath路径下面
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
加上file表示使用绝对路径指定spring配置文件位置
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("file:G:/IDEA/S3/Spring/demo1/src/main/resources/applicationContext.xml");
FileSystemXmlApplicationContext:
- 默认是项目工作路径,即项目的根目录
- 有file表示的是文件绝对路径
- 如果要使用classpath路径,需要前缀classpath
使用FileSystemXmlApplicationContext指定spring配置文件位置
默认是项目工作路径,即项目的根目录,而我们的配置文件在根目录下的src/main/resources下面
ApplicationContext applicationContext = new FileSystemXmlApplicationContext("src/main/resources/applicationContext.xml");
加上file表示的是文件绝对路径
ApplicationContext applicationContext = new FileSystemXmlApplicationContext("file:G:/IDEA/S3/Spring/demo1/src/main/resources/applicationContext.xml");
使用classpath路径,需要前缀classpath,相当于ClassPathXmlApplicationContext
ApplicationContext applicationContext = new FileSystemXmlApplicationContext("classpath:applicationContext.xml");
配置文件在类路径下
我们的配置文件一般件存放在项目的类路径下,则使用ClassPathXmlApplicationContext 实现类进行加载。 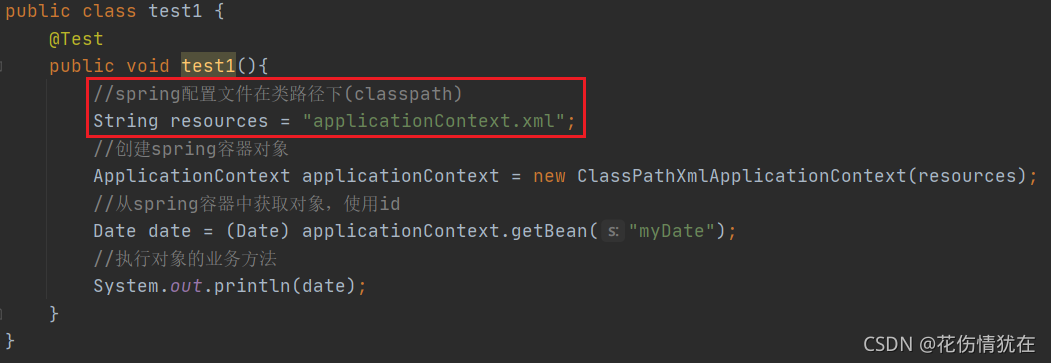 ApplicationContext 容器中对象的装配时机
ApplicationContext 容器,会在容器对象初始化时,将其中的所有对象一次性全部装配好。以后代码中若要使用到这些对象,只需从内存中直接获取即可。执行效率较高。但占用内存。
 代码
public class test1 {
@Test
public void test1(){
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("applicationContext.xml");
}
}
使用 spring 容器创建的 java 对象 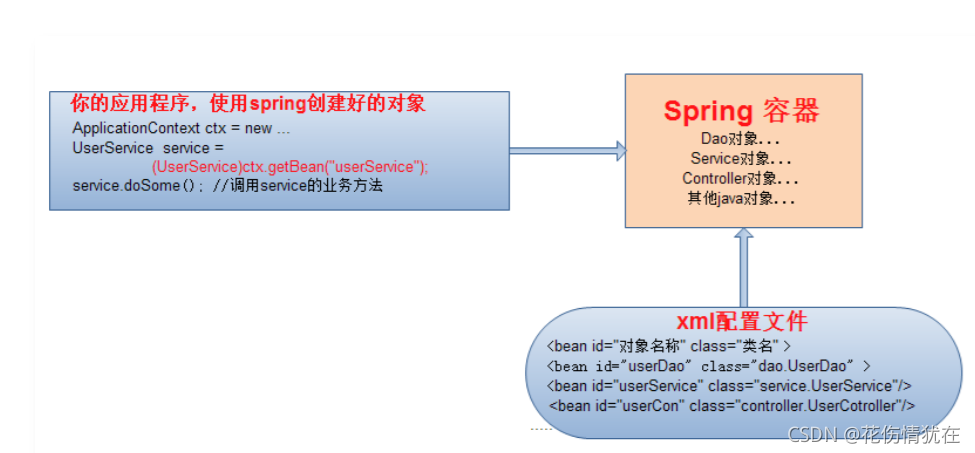
- spring容器在运行的时候会先读取applicationContext.xml配置文件
- spring读取配置文件, 一次创建好所有的java对象, 都放到map中。
- 通过applicationContext.getBean(“bean对象的id”)获取代理对象
|