1.for循环
1.1.for的格式
格式:
for(1初始化语句;2条件判断语句;3条件控制语句){
4循环体语句;
}
执行流程:
1 2 4 3 2 4 3 2
1.2循环输出10次广告
package com.itheima01;
public class Demo01 {
public static void main(String[] args) {
for(int i=0; i<10; i++){
System.out.println("秋天不吃西瓜...");
}
for(int i=1; i<=10; i++){
System.out.println("秋天吃桃...");
}
}
}
1.3循环1-5和5~1
package com.itheima01;
public class Demo02 {
public static void main(String[] args) {
for(int i=1; i<=5; i++){
System.out.println(i);
}
for(int i=5; i>=1; i--){
System.out.println(i);
}
}
}
1.4求1-5的和
package com.itheima01;
public class Demo03 {
public static void main(String[] args) {
int sum = 0;
for(int i=1; i<=5; i++){
sum = sum+i;
}
System.out.println(sum);
}
}
1.5求数字的奇偶数
package com.itheima01;
public class Demo04 {
public static void main(String[] args) {
for(int i=0; i<=10; i++){
if(i%2==0){
System.out.println(i+"是偶数");
}else{
System.out.println(i+"是奇数");
}
}
}
}
2.while循环
2.1while的格式
格式:
初始化语句;
while(条件判断语句){
循环体语句;
条件控制语句;
}
2.2输出1-5和5-1
package com.itheima01;
public class Demo05 {
public static void main(String[] args) {
int i = 1;
while(i<=5){
System.out.println(i);
i++;
}
int j = 5;
while(j>=1){
System.out.println(j);
j--;
}
}
}
2.3求1-100的和
package com.itheima01;
public class Demo06 {
public static void main(String[] args) {
int sum = 0;
int i = 1;
while(i<=100){
sum += i;
i++;
}
System.out.println("1到100的和是" + sum);
}
}
2.4珠穆朗玛峰
package com.itheima01;
public class Demo07 {
public static void main(String[] args) {
double zhi = 0.0001;
int shan = 8848;
int count = 0;
while(zhi <= shan) {
zhi = zhi*2;
count++;
}
System.out.println(count);
}
}
3.do…while
3.1do…while的格式
格式:
初始化语句;
do {
循环体语句;
条件控制语句;
} while(条件判断语句);
3.2打印1-5
package com.itheima01;
public class Demo08 {
public static void main(String[] args) {
int i = 1;
do{
System.out.println(i);
i++;
}while (i<=5);
}
}
4.三种循环区别
for:
在明确循环次数的时候建议使用
while:
在不明确循环次数的时候建议使用
在写死循环时候建议使用
while(true){
}
do..while:
没有使用场景...
一:格式不同
-
for循环各部分形成一个整体; -
while循环和do_while循环的初始化语句和循环定义分开; -
while循环和do_while循环的初始化语句和控制条件语句一般都会省略,而for循环一般不省略;
二:初始化语句不同
-
定义位置不同; -
作用域不同: for循环的初始化条件仅限循环内部使用;
while循环和do_while循环的初始化条件可以在循环外部使用;
三:循环体执行次数不同
-
for循环和while循环的循环体语句执行0~n次; -
do_while循环的循环体语句执行1~n次,即至少执行1次;
四:应用场景不同
-
for循环和while循环可以互换,while循环更加简洁; -
do_while循环在循环体语句至少需要执行1次时使用; ———————————————— 原文链接:
5.Debug代码调试
debug的功能就是查看代码的执行流程,看代码中的问题
用法:
1.加断点,哪里不会点哪里
2.右键选择debug运行
3.点F8向下执行
4.点击stop结束程序
5.点断点,去掉断点
6.循环跳转语句
continue:
在循环中,表示跳过某次循环,进入下一次循环
break:
在循环中,表示终止循环,整个当前循环就结束了
注意事项:
1.任何循环都可以使用,for while do.while..
2.必须用在判断语句中
package com.itheima02;
public class Demo01 {
public static void main(String[] args) {
for(int i=1; i<=100; i++){
if(i%7==0){
continue;
}
System.out.println(i);
}
}
}
7.循环嵌套
package com.itheima02;
public class Demo02 {
public static void main(String[] args) {
for(int i=0; i<24; i++){
for(int j=0; j<60; j++){
System.out.println(i + "小时" + j+"分钟");
}
}
}
}
package com.itheima02;
public class Demo03 {
public static void main(String[] args) {
for(int i=0; i<4; i++){
for(int j=0; j<5; j++) {
System.out.print("*");
}
System.out.println();
}
}
}
8.Random随机数
1.导包
import java.util.Random;
2.创建对象
Random r = new Random();
3.生成随机数(0-9的随机数)
int a = r.nextInt(10);
package com.itheima02;
import java.util.Random;
public class Demo05 {
public static void main(String[] args) {
Random r = new Random();
int a = r.nextInt(10);
System.out.println(a);
int b = r.nextInt(100);
System.out.println(b);
int c = r.nextInt(10) + 1;
int d = r.nextInt(10) + 11;
int e = r.nextInt(21) + 20;
}
}
除此之外: Random 有两个构造方法:
public Random() public Random(long seed) 其实第一个无参构造方法会默认以当前时间作为种子。那么什么是种子呢?
先来看看 Random 的 next() 方法:
protected int next(int bits) { long oldseed, nextseed; AtomicLong seed = this.seed; do { oldseed = seed.get(); nextseed = (oldseed * multiplier + addend) & mask; } while (!seed.compareAndSet(oldseed, nextseed)); return (int)(nextseed >>> (48 - bits)); } seed 就是种子,它的作用就是用于生成一个随机数。
- 两个构造方法有什么不同?
现在看一个例子,对比这两个构造方法有什么不同。
public class RandomDemo {
public static void main(String[] args) {
for(int i = 0; i < 5; i++) {
Random random = new Random();
for(int j = 0; j < 5; j++) {
System.out.print(" " + random.nextInt(10) + ", ");
}
System.out.println("");
}
}
} 以上使用了 Random 无参的构造方法,运行结果如下:
2 0 3 2 5 6 4 1 9 7 9 1 8 3 6 2 5 3 5 6 9 9 9 4 5 可以看出每次的结果都不一样。下面使用 Random 的有参构造方法:
public class RandomDemo {
public static void main(String[] args) {
for(int i = 0; i < 5; i++) {
Random random = new Random(47);
for(int j = 0; j < 5; j++) {
System.out.print( + random.nextInt(10) + " ");
}
System.out.println("");
}
}
} 打印结果如下:
8 5 3 1 1 8 5 3 1 1 8 5 3 1 1 8 5 3 1 1 8 5 3 1 1 这是因为无参的构造方法是以当前时间作为种子,每次的种子都不一样,随机性更强。而有参的构造方法是以固定值作为种子,每次输出的值都是一样的。 ————————————————
原文链接:
9.猜数字小游戏
package com.itheima02;
import java.util.Random;
import java.util.Scanner;
public class Demo06 {
public static void main(String[] args) {
Random r = new Random();
int num = r.nextInt(100)+1;
while (true) {
Scanner sc = new Scanner(System.in);
System.out.println("请输入一个1-100的整数:");
int a = sc.nextInt();
if (a > num) {
System.out.println("你猜大了~");
} else if (a < num) {
System.out.println("你猜小了");
} else {
System.out.println("恭喜猜对了!");
break;
}
}
}
}
RESPECT&LOVE :一起进步&一起成长 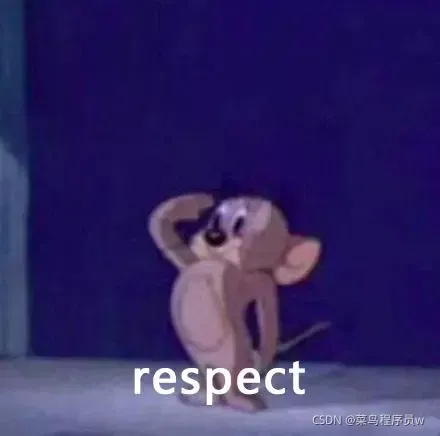
|