添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-mail</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-actuator</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<optional>true</optional>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-test</artifactId>
<scope>test</scope>
</dependency>
发送者邮箱配置-139邮箱
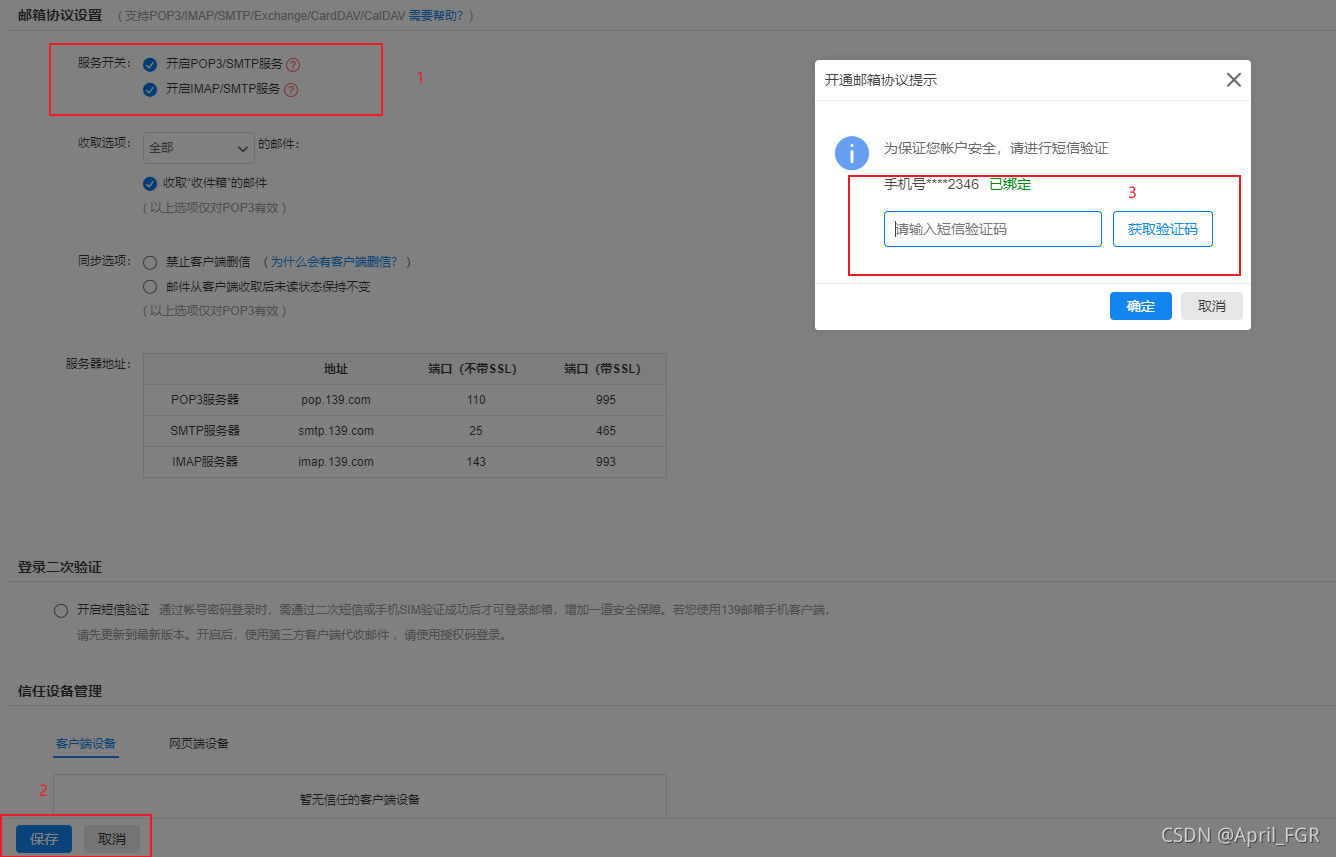 刷新页面后会出现生成授权码的提示 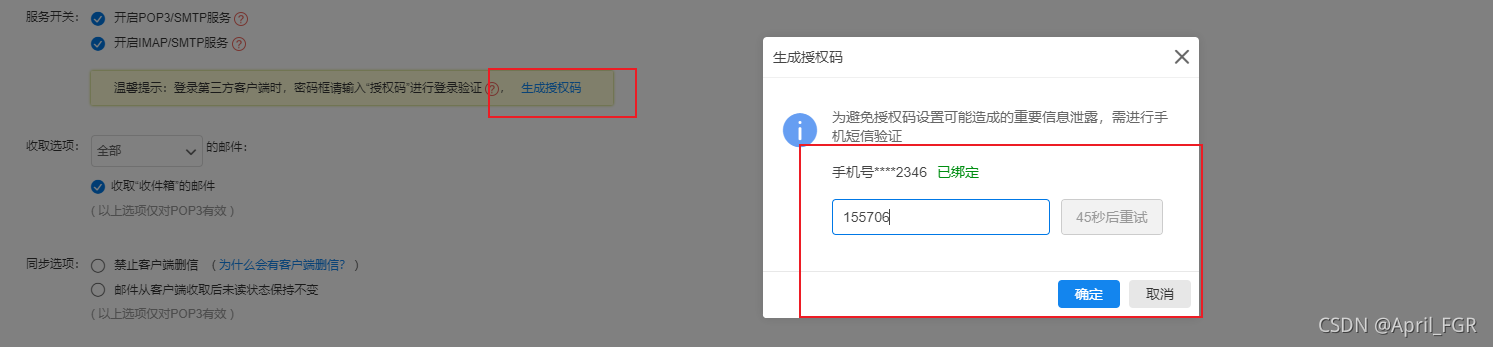
添加配置-139邮箱
server:
port: 9657
spring:
profiles:
active: 139
spring:
mail:
host: smtp.139.com
username: 15178292346@139.com
password: ****
port: 465
default-encoding: UTF-8
properties:
mail:
smtp:
ssl:
enable: true
socketFactoryClass: javax.net.ssl.SSLSocketFactory
debug: true
编写工具类
普通邮件
@Component
public class EmailUtil {
@Autowired
JavaMailSender javaMailSender;
@Value("${spring.mail.username}")
private String sendEmail;
public void sendSimpleMail() {
SimpleMailMessage message = new SimpleMailMessage();
message.setSubject("这是一封测试邮件");
message.setFrom(sendEmail);
message.setTo("*****@163.com");
message.setCc("898365387@qq.com");
message.setBcc("*****@qq.com");
message.setSentDate(new Date());
message.setText("这是测试邮件的正文");
javaMailSender.send(message);
}
}
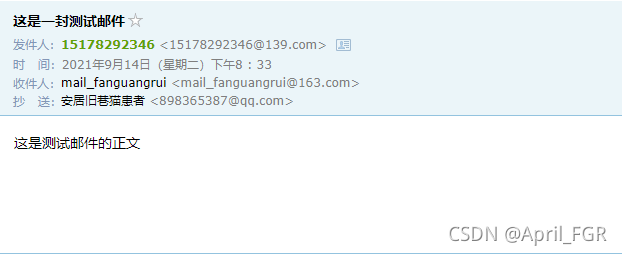
添加附件
public void sendAttachFileMail() {
try {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage,true);
helper.setSubject("这是一封测试邮件");
helper.setFrom(sendEmail);
helper.setTo("mail_fanguangrui@163.com");
helper.setSentDate(new Date());
helper.setText("这是测试邮件的正文");
helper.addAttachment("bg1.png",new File("E:\\springboot\\springboot-plus\\learn-email\\src\\main\\resources\\file\\bg1.png"));
javaMailSender.send(mimeMessage);
} catch (Exception e){
e.printStackTrace();
}
}
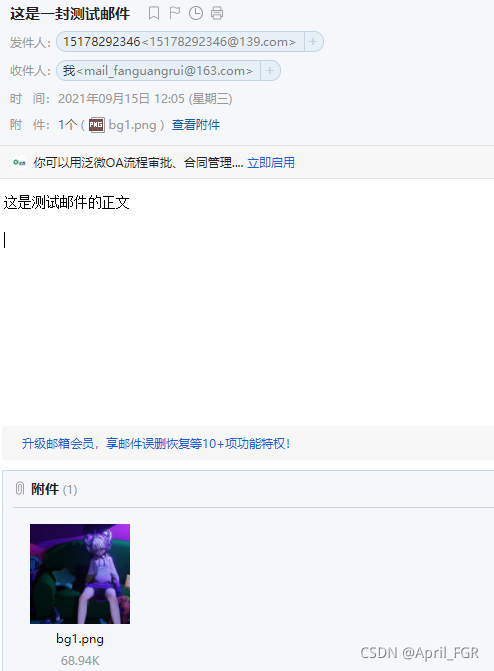
添加图片
public void sendImgResMail() {
try {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true);
helper.setSubject("这是一封测试邮件");
helper.setFrom(sendEmail);
helper.setTo("898365387@qq.com");
helper.setSentDate(new Date());
helper.setText("<p>hello 大家好,这是一封测试邮件,这封邮件包含两种图片,分别如下</p><p>第一张图片:</p><img src='cid:p01'/><p>第二张图片:</p><img src='cid:p02'/>",true);
helper.addInline("p01",new FileSystemResource(new File("E:\\springboot\\springboot-plus\\learn-email\\src\\main\\resources\\file\\bg1.png")));
helper.addInline("p02",new FileSystemResource(new File("E:\\springboot\\springboot-plus\\learn-email\\src\\main\\resources\\file\\bg2.png")));javaMailSender.send(mimeMessage);
} catch (Exception e){
e.printStackTrace();
}
}
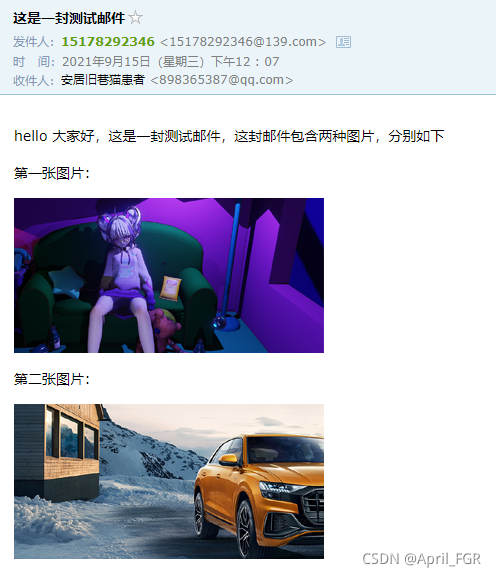
使用thymeleaf模板
添加依赖
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
添加模板 最好放在resources/templates文件夹下
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<base target="_blank" />
<style type="text/css">::-webkit-scrollbar{ display: none; }</style>
<style id="cloudAttachStyle" type="text/css">#divNeteaseBigAttach, #divNeteaseBigAttach_bak{display:none;}</style>
<style id="blockquoteStyle" type="text/css">blockquote{display:none;}</style>
<style type="text/css">
body{font-size:14px;font-family:arial,verdana,sans-serif;line-height:1.666;padding:0;margin:0;overflow:auto;white-space:normal;word-wrap:break-word;min-height:100px}
td, input, button, select, body{font-family:Helvetica, 'Microsoft Yahei', verdana}
pre {white-space:pre-wrap;white-space:-moz-pre-wrap;white-space:-pre-wrap;white-space:-o-pre-wrap;word-wrap:break-word;width:95%}
th,td{font-family:arial,verdana,sans-serif;line-height:1.666}
img{ border:0}
header,footer,section,aside,article,nav,hgroup,figure,figcaption{display:block}
blockquote{margin-right:0px}
</style>
</head>
<body tabindex="0" role="listitem">
<table width="700" border="0" align="center" cellspacing="0" style="width:700px;">
<tbody>
<tr>
<td>
<div style="width:700px;margin:0 auto;border-bottom:1px solid #ccc;margin-bottom:30px;">
<table border="0" cellpadding="0" cellspacing="0" width="700" height="39" style="font:12px Tahoma, Arial, 宋体;">
<tbody><tr><td width="210"></td></tr></tbody>
</table>
</div>
<div style="width:680px;padding:0 10px;margin:0 auto;">
<div style="line-height:1.5;font-size:14px;margin-bottom:25px;color:#4d4d4d;">
<strong style="display:block;margin-bottom:15px;">尊敬的用户:<span style="color:#f60;font-size: 16px;"></span>您好!</strong>
<strong style="display:block;margin-bottom:15px;">
您正在进行<span style="color: red">注册\登录</span>操作,请在验证码输入框中输入:<span style="color:#f60;font-size: 24px" th:text="${code}"></span>,以完成操作。
</strong>
</div>
<div style="margin-bottom:30px;">
<small style="display:block;margin-bottom:20px;font-size:12px;">
<p style="color:#747474;">
注意:此操作可能会修改您的密码、登录邮箱或绑定手机。如非本人操作,请及时登录并修改密码以保证帐户安全
<br>(工作人员不会向你索取此验证码,请勿泄漏!)
</p>
</small>
</div>
</div>
<div style="width:700px;margin:0 auto;">
<div style="padding:10px 10px 0;border-top:1px solid #ccc;color:#747474;margin-bottom:20px;line-height:1.3em;font-size:12px;">
<p>此为系统邮件,请勿回复<br>
请保管好您的邮箱,避免账号被他人盗用
</p>
<p>FGRAPP</p>
</div>
</div>
</td>
</tr>
</tbody>
</table>
</body>
</html>
发送邮件方法
public boolean sendThymeleafMail() {
try {
MimeMessage mimeMessage = javaMailSender.createMimeMessage();
MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true);
mimeMessage.setSubject("【FGRAPP】注册登录验证码");
helper.setFrom(sendEmail);
helper.setTo("898365387@qq.com");
helper.setSentDate(new Date());
Context context = new Context();
context.setVariable("code", "123456");
String process = templateEngine.process("register.html", context);
helper.setText(process,true);
javaMailSender.send(mimeMessage);
return true;
} catch (Exception e){
e.printStackTrace();
return false;
}
}
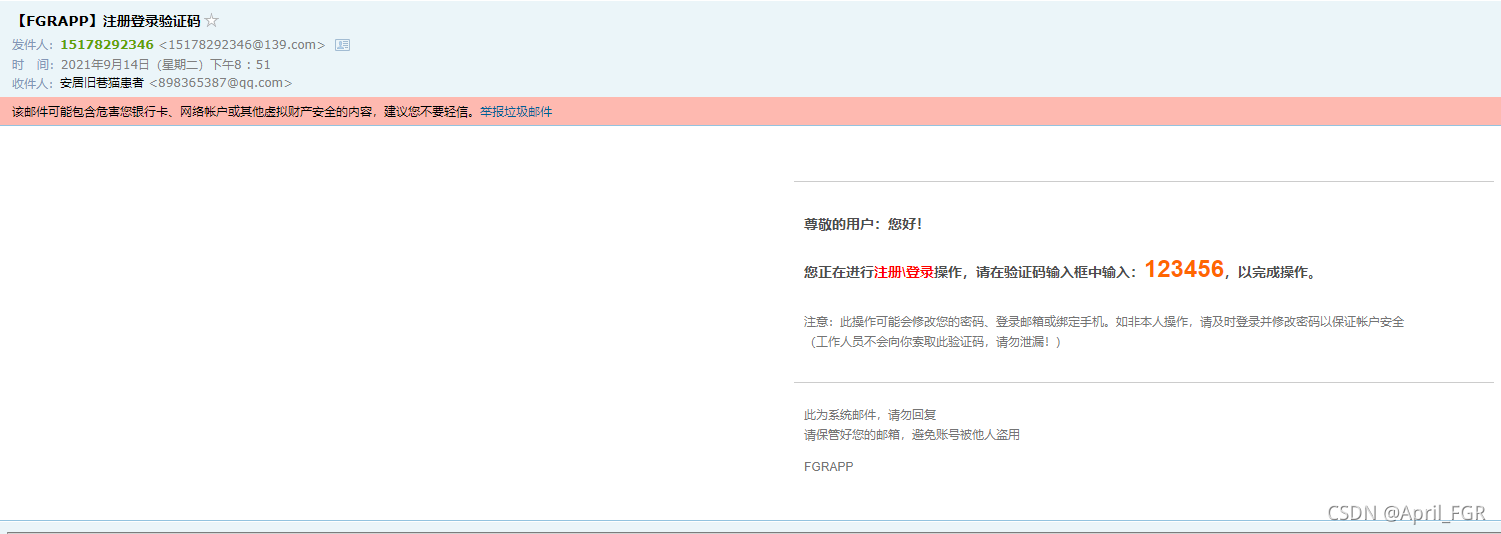
测试代码
@SpringBootTest
@RunWith(SpringRunner.class)
@WebAppConfiguration
public class EmailUtilTest {
@Autowired
private EmailUtil emailUtil;
@Test
public void testSendSimpleMail(){
emailUtil.sendSimpleMail();
}
@Test
public void sendThymeleafMail(){
emailUtil.sendThymeleafMail();
}
@Test
public void sendImgResMail(){
emailUtil.sendImgResMail();
}
@Test
public void sendAttachFileMail(){
emailUtil.sendAttachFileMail();
}
}
网易邮箱配置
spring:
mail:
host: smtp.163.com
username: mail_fanguangrui@163.com
password: ***
port: 465
default-encoding: UTF-8
properties:
mail:
smtp:
ssl:
enable: true
socketFactoryClass: javax.net.ssl.SSLSocketFactory
debug: true
QQ邮箱配置
spring:
mail:
host: smtp.qq.com
username: 898365387@qq.com
password: ***
port: 465
default-encoding: UTF-8
properties:
mail:
smtp:
ssl:
enable: true
socketFactoryClass: javax.net.ssl.SSLSocketFactory
debug: true
总结
各个邮箱的配置区别就在于服务器地址以及不使用ssl时的端口号:
服务商 | 服务器地址 | 端口号(不使用ssl) |
---|
139邮箱 | smtp.139.com | 25 | 网易邮箱 | smtp.163.com | 994 | QQ邮箱 | smtp.qq.com | 587 |
在使用ssl的情况下端口号都是465
项目源码
GitHub地址
|