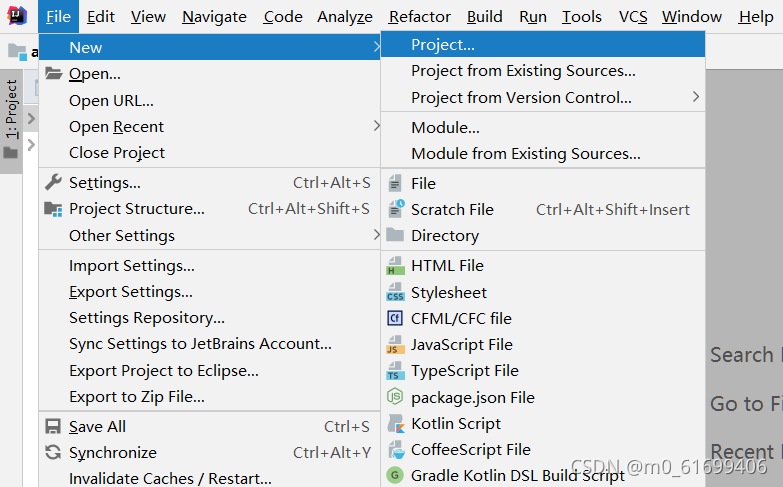
?
?
?
?
?
?
?
?
..在Users类里编写,代码如下
package com.bean; public class Users { ? ? private int id; ? ? private String name; ? ? private String password;
? ? public Users(){
? ? } ? ? public Users(String name,String password){ ? ? ? ? this.name=name; ? ? ? ? this.password=password; ? ? } ? ? public int getId() { ? ? ? ? return id; ? ? } ? ? public void setId(int id){ ? ? ? ? this.id=id; ? ? }
? ? public String getName() { ? ? ? ? return name; ? ? }
? ? public void setName(String name) { ? ? ? ? this.name = name; ? ? }
? ? public String getPassword() { ? ? ? ? return password; ? ? }
? ? public void setPassword(String password) { ? ? ? ? this.password = password; ? ? } } .在UsersDao里编写,代码如下
package com.dao; import com.util.Util; import com.bean.Users;
import java.util.ArrayList; import java.util.HashMap; import java.util.List; import java.util.Map;
public class UsersDao extends Util { ? ? //添加记录 ? ? public void insert(Users user) throws Exception { ? ? ? ? //1.获取连接对象 ? ? ? ? conn(); ? ? ? ? //2.获取一个可以执行sql语句的对象 ? ? ? ? String sql="insert users(name,password) values(?,?)"; ? ? ? ? pstm=conn.prepareStatement(sql); ? ? ? ? pstm.setString(1,user.getName()); ? ? ? ? pstm.setString(2,user.getPassword()); ? ? ? ? //3.执行一条添加的sql ? ? ? ? pstm.execute(); ? ? ? ? System.out.println("添加记录"); ? ? ? ? //4.关闭数据库 ? ? ? ? close(); ? ? } ? ? //删除记录 ? ? public void delete(int id) throws Exception{ ? ? ? ? //1.获取连接对象 ? ? ? ? conn(); ? ? ? ? //2.获取一个可以执行sql语句的对象 ? ? ? ? String sql="delete from users where id=?"; ? ? ? ? pstm=conn.prepareStatement(sql); ? ? ? ? pstm.setInt(1, id); ? ? ? ? //3.执行一条删除的sql ? ? ? ? pstm.execute(); ? ? ? ? System.out.println("删除记录"); ? ? ? ? //4.关闭数据库 ? ? ? ? close(); ? ? } ? ? //修改记录 ? ? public void update(int id,Users user) throws Exception{ ? ? ? ? //1.获取连接对象 ? ? ? ? conn(); ? ? ? ? //2.获取一个可以执行sql语句的对象 ? ? ? ? String sql="update users set name=?,password=? where id=?"; ? ? ? ? pstm=conn.prepareStatement(sql); ? ? ? ? pstm.setString(1, user.getName()); ? ? ? ? pstm.setString(2,user.getPassword()); ? ? ? ? pstm.setInt(3,id); ? ? ? ? //3.执行一条修改的sql ? ? ? ? pstm.executeUpdate(); ? ? ? ? System.out.println("修改记录"); ? ? ? ? //4.关闭数据库 ? ? ? ? close(); ? ? } ? ? //查询全部 ? ? public List<Map> select() throws Exception{ ? ? ? ? //1.获取连接对象 ? ? ? ? conn(); ? ? ? ? //2.获取一个可以执行sql语句的对象 ? ? ? ? String sql="select * from users"; ? ? ? ? pstm=conn.prepareStatement(sql); ? ? ? ? //3.执行一条修改的sql ? ? ? ? rs=pstm.executeQuery(); ? ? ? ? List list=new ArrayList(); ? ? ? ? while(rs.next()){ ? ? ? ? ? ? int id=rs.getInt("id"); ? ? ? ? ? ? String name=rs.getString("name"); ? ? ? ? ? ? String password=rs.getString("password"); ? ? ? ? ? ? Map map=new HashMap(); ? ? ? ? ? ? map.put("id",id); ? ? ? ? ? ? map.put("name",name); ? ? ? ? ? ? map.put("password",password); ? ? ? ? ? ? list.add(map); ? ? ? ? } ? ? ? ? System.out.println("查询全部记录");
? ? ? ? //4.关闭数据库 ? ? ? ? close(); ? ? ? ? return list;
? ? } ? ? public static void main(String[] args) throws Exception { ? ? ? ? //在此处对表进行操作 ? ? ? ? UsersDao db=new UsersDao(); ? ? ? ? Users user=new Users("百里","111"); ? ? ? ? db.insert(user); ? ? ? ? db.delete(7); ? ? ? ? Users user1=new Users("云英","111"); ? ? ? ? db.update(1,user); ? ? ? ? List list=db.select(); ? ? ? ? System.out.println(list); ? ? } }
在Util里编写,代码如下
package com.util; import java.sql.*;
public class Util { ? ? public static String url; ? ? public static String name; ? ? public static String password; ? ? public static Connection conn=null;//连接数据库 ? ? public static Statement stm=null;//执行sql语句 ? ? public static PreparedStatement pstm=null; ? ? public ResultSet rs=null;//结果集 ? ? //连接数据库 ? ? public static Connection conn() throws Exception { ? ? ? ? Class.forName("com.mysql.jdbc.Driver"); ? ? ? ? url="jdbc:mysql://localhost:3306/1919"; ? ? ? ? name="root"; ? ? ? ? password="x5"; ? ? ? ? conn= DriverManager.getConnection(url,name,password); ? ? ? ? System.out.println("连接数据库"); ? ? ? ? return conn; ? ? } ? ? //关闭数据库 ? ? public static void close() throws Exception { ? ? ? ? if(stm!=null){ ? ? ? ? ? ? stm.close(); ? ? ? ? } ? ? ? ? if(pstm!=null){ ? ? ? ? ? ? pstm.close(); ? ? ? ? } ? ? ? ? if(conn!=null){ ? ? ? ? ? ? conn.close(); ? ? ? ? }
? ? ? ? System.out.println("关闭数据库"); ? ? }
} ?
|