Springboot数据访问(整合MyBatis-Plus)实际开发的规范写法
首先写我们的User类:
package com.example.boot.bean;
import lombok.AllArgsConstructor;
import lombok.Data;
import lombok.NoArgsConstructor;
import lombok.ToString;
@Data
@ToString
@AllArgsConstructor
@NoArgsConstructor
public class User {
private Long id;
private String name;
private Integer age;
private String email;
}
然后写我们的mapper继承BaseMapper: 泛型是我们的User类(也就是我们的Bean)。
package com.example.boot.mapper;
import com.baomidou.mybatisplus.core.mapper.BaseMapper;
import com.example.boot.bean.User;
public interface UserMapper extends BaseMapper<User> {
}
然后写我们的Service接口,继承IService,泛型是我们的User类(Bean):
package com.example.boot.service;
import com.baomidou.mybatisplus.extension.service.IService;
import com.example.boot.bean.User;
public interface UserService extends IService<User> {
}
然后写我们的ServiceImpl类,他继承ServiceImpl类,泛型有两个参数,第一个是继承BaseMapper<T>的Mapper接口,第二个是我们的User类(Bean),然后还要实现我们的UserService接口。
package com.example.boot.service.impl;
import com.baomidou.mybatisplus.extension.service.impl.ServiceImpl;
import com.example.boot.bean.User;
import com.example.boot.mapper.UserMapper;
import com.example.boot.service.UserService;
import org.springframework.stereotype.Service;
@Service
public class UserServiceImpl extends ServiceImpl<UserMapper, User> implements UserService {
}
之后在我们的控制器中进行UserService的自动注入和调用。
package com.example.boot.controller;
import com.example.boot.bean.User;
import com.example.boot.service.UserService;
import org.springframework.stereotype.Controller;
import org.springframework.web.bind.annotation.*;
import org.springframework.web.servlet.ModelAndView;
import javax.annotation.Resource;
import java.util.List;
@Controller
public class UserController {
@Resource
UserService userService;
@GetMapping("/user")
public ModelAndView findAll(ModelAndView modelAndView){
List<User> userList = userService.list();
modelAndView.addObject("userList",userList);
modelAndView.setViewName("showUser");
return modelAndView;
}
}
然后我们写一个页面来大概展示一下:
<!DOCTYPE html>
<html lang="en" xmlns:th="http://www.thymeleaf.org">
<head>
<meta charset="UTF-8">
<title>用户展示</title>
</head>
<body>
<table border="1" width="50%" align="center">
<tr>
<th>编号</th>
<th>姓名</th>
<th>年龄</th>
<th>邮箱</th>
</tr>
<tr th:each="user:${userList}">
<td th:text="${user.id}"></td>
<td th:text="${user.name}"></td>
<td th:text="${user.age}"></td>
<td th:text="${user.email}"></td>
</tr>
</table>
</body>
</html>
运行结果: 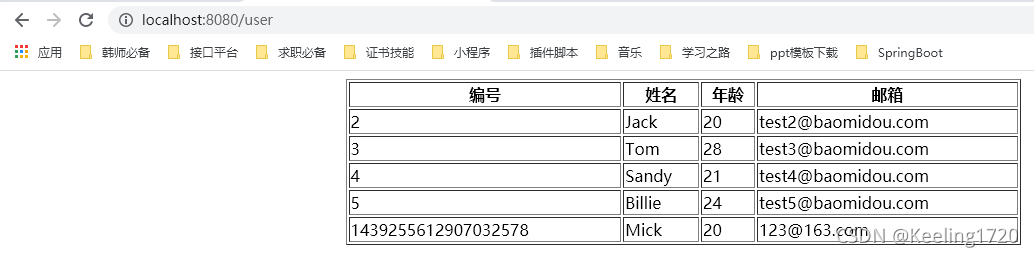
|