1、Spring
1.1 简介
Spring理念:使现有的技术更加容易使用,本身是一个大杂烩,整合了现有的技术框架! SSH:Struct2 + Spring + Hibernate! SSM:SpringMVC + Spring + Mybatis! 中文文档
<!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<!-- https://mvnrepository.com/artifact/org.springframework/spring-webmvc -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
1.2 优点
Spring是一个开源的免费的框架(容器)! Spring是一个轻量级的、非入侵式的框架! 控制反转(IOC),面向切面编程(AOP)! 支持事务的处理,对框架整合的支持! 总结一句话:Spring就是一个轻量级的控制反转(IOC)和面向切面编程(AOP)的框架!
1.3 组成
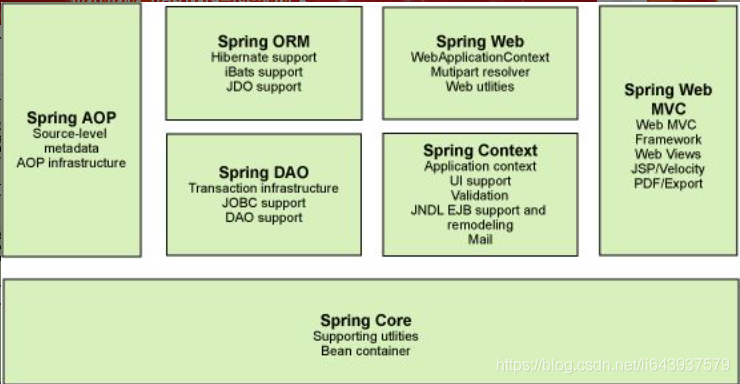
2.IOC
2.1 IOC组成理论推导
原来的实现方式 1.UserDao接口
public interface UserDao {
void getUser();
}
2.UserDaoImpl实现类
public class UserDaoImpl implements UserDao{
public void getUser() {
System.out.println("默认获取用户的数据");
}
}
3.UserService业务接口
public interface UserService {
void getUser();
}
4.UserServiceImpl实现类
public class UserServiceImpl implements UserService{
private UserDao userDao = new UserDaoImpl();
public void getUser() {
userDao.getUser();
}
}
如果想要改变,就需要每次更改UserDao 若将UesrDao使用Set接口实现
public class UserServiceImpl implements UserService{
private UserDao userDao;
public void setUserDao(UserDao userDao) {
this.userDao = userDao;
}
public void getUser() {
userDao.getUser();
}
}
- 之前,程序通过new主动创建对象!控制权在程序猿手上
- 使用set注入后,程序不再具有主动性,而是变成了被动的接受对象!
- 这种思想,从本质上解决了问题,程序员不用再去管理对象的创建了,降低了耦合性
2.2 IOC本质
控制反转IOC(Inversion of Control),是一种设计思想,DI(依赖注入)是实现IOC的一种方法, 也有人认为DI只是IOC的另一种说法。没有IOC的程序中,我们使用面向对象编程,对象的创建与对象间的依赖关系完全硬编码在程序中,对象的创建由程序自己控制,控制反转后将对象的创建转移给第三方,个人认为所谓的控制反转就是:获得依赖的方式反转了。
采用XML方式配置Bean的时候,Bean的定义信息是和实现分离的,而采用注解的方式可以把两者合为一体,Bean的定义信息直接以注解的形式定义在实现类中,从而达到了零配置的目的。
控制反转是一种通过描述(xml或注解)并通过第三方去生产或获取特定对象的方式。在spring中实现控制反转的是IOC容器,其实现方法是依赖注入(Dependency Injection,DI)
xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
// 类型 变量名=new 类型();
// id相对于变量名,class相对于类型
<bean id="hello" class="com.meng.dao.Hello">
// ref引用spring配置中的值
// value 具体的值,基本数据类型数据
<property name="name" value="张三"/>
</bean>
<bean id="userDaoMysql" class="com.meng.dao.userDaoMysqlImpl"></bean>
<bean id="userDaoImpl" class="com.meng.dao.userDaoImpl"></bean>
<bean id="userServiceImpl" class="com.meng.service.userServiceImpl">
<property name="userDao" ref="userDaoMysql"></property>
</bean>
</beans>
实体类
package com.yang.entity;
public class Hello {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Hello{" +
"name='" + name + '\'' +
'}';
}
}
测试
import com.yang.entity.Hello;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
public static void main(String[] args) {
//获取spring的上下文对象
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
//我们的对象现在都在spring中管理,我们要使用,直接去里面取出来
Hello hello = (Hello) context.getBean("hello");
System.out.println(hello.toString());
}
}
发现,已经不需要手动new对象,对象是在xml文件中配置。或者通俗来讲,不需要改底层代码,而xml文件不算底层代码。
控制反转
控制: 谁来控制对象的创建,传统应用程序的对象是由程序本身控制创建的,使用Spring后,对象是由Spring来创建的 反转: 程序本身不创建对象,而变成被动的接收对象。 依赖注入: 就是利用set方法来进行注入的 IOC是一种编程思想,由主动的编程变为被动的接收,所谓的IOC,即对象由Spring来创建,管理,装配
2.3 IOC创建对象的方式
- 默认使用无参构造创建对象
- 使用有参构造创建对象的三种方式
public class User {
private String name;
private int age;
public User(String name, int age) {this.name = name;this.age = age;}
public String getName() {return name;}
public void setName(String name) {this.name = name;}
public int getAge() {return age;}
public void setAge(int age) {this.age = age;}
}
- 下标赋值 Constructor argument index
<bean id="user" class="com.yang.entity.User">
<constructor-arg index="0" value="张三"/>
<constructor-arg index="1" value="18"/>
</bean>
- 变量类型赋值 Constructor argument type matching
<bean id="user1" class="com.yang.entity.User">
<constructor-arg type="int" value="18"/>
<constructor-arg type="java.lang.String" value="张三"/>
</bean>
- 变量名称赋值 Constructor argument name
<bean id="user2" class="com.yang.entity.User">
<constructor-arg name="name" value="张三"/>
<constructor-arg name="age" value="18"/>
</bean>
在获取spring的上下文对象( new ClassPathXmlApplicationContext(“beans.xml”); )时,spring容器中的所有的对象就已经被创建了
3.Spring的基础配置
3.1 别名
<alias name="user" alias="userAlias"/>
3.2 Bean的配置
<bean id="user1" class="com.yang.entity.User" name="test test1, test2; test3">
<constructor-arg type="int" value="18"/>
<constructor-arg type="java.lang.String" value="张三"/>
</bean>
3.3 import
import,一般用于团队开发使用,他可以将多个配置文件,导入合并为1个 假设,现在项目中又多个人开发,这三个人负责不同的类开发,不同的类需要注册在不同的bean中,我们可以用import将所有人的beans.xml合并为一个总的! 在总的xml合并,使用总的xml
<import resource="applicationContext1.xml"/>
<import resource="applicationContext2.xml"/>
4.DI依赖注入
4.1 构造器注入
4.2 set方式注入 【重点】
依赖注入 依赖:bean对象的创建依赖于容器 注入:bean对象中的所有属性,由容器来注入
【环境搭建】 1.复杂类型
package com.yang.entity;
public class Address {
private String address;
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "Address{" +
"address='" + address + '\'' +
'}';
}
}
2.真实测试对象
package com.yang.entity;
import java.util.*;
public class Student {
private String name;
private Address address;
private String [] books;
private List<String> hobbies;
private Map<String, String> card;
private Set<String> games;
private String wife;
private Properties info;
}
3.applicationContext.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="address" class="com.meng.pojo.Address">
<property name="address" value="广西"></property>
</bean>
<bean id="student" class="com.meng.pojo.Stundet">
<property name="name" value="李四"></property>
<property name="address" ref="address"></property>
<property name="books">
<array>
<value>红楼梦</value>
<value>三国演义</value>
</array>
</property>
<property name="hobbies">
<list>
<value>游戏</value>
<value>听歌</value>
</list>
</property>
<property name="card">
<map>
<entry key="手机号" value="123123"></entry>
<entry key="银行卡" value="124563322341"></entry>
</map>
</property>
<property name="games">
<set>
<value>lol</value>
<value>dnf</value>
</set>
</property>
<property name="wife">
<null></null>
</property>
<property name="info">
<props>
<prop key="userName">root</prop>
<prop key="pwd">12314</prop>
</props>
</property>
</bean>
</beans>
4.测试类输出
4.3 p、c 标签注入
p标签注入,须在beans中引入 xmlns:p="http://www.springframework.org/schema/p"
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="p_test" class="com.yang.entity.Student" p:name="yang"/>
c标签注入,需在实体中增加有参构造方法,并引入 xmlns:c="http://www.springframework.org/schema/c"
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:c="http://www.springframework.org/schema/c"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="c_test" class="com.yang.entity.Address" c:address="DL"/>
4.4 Bean的作用域
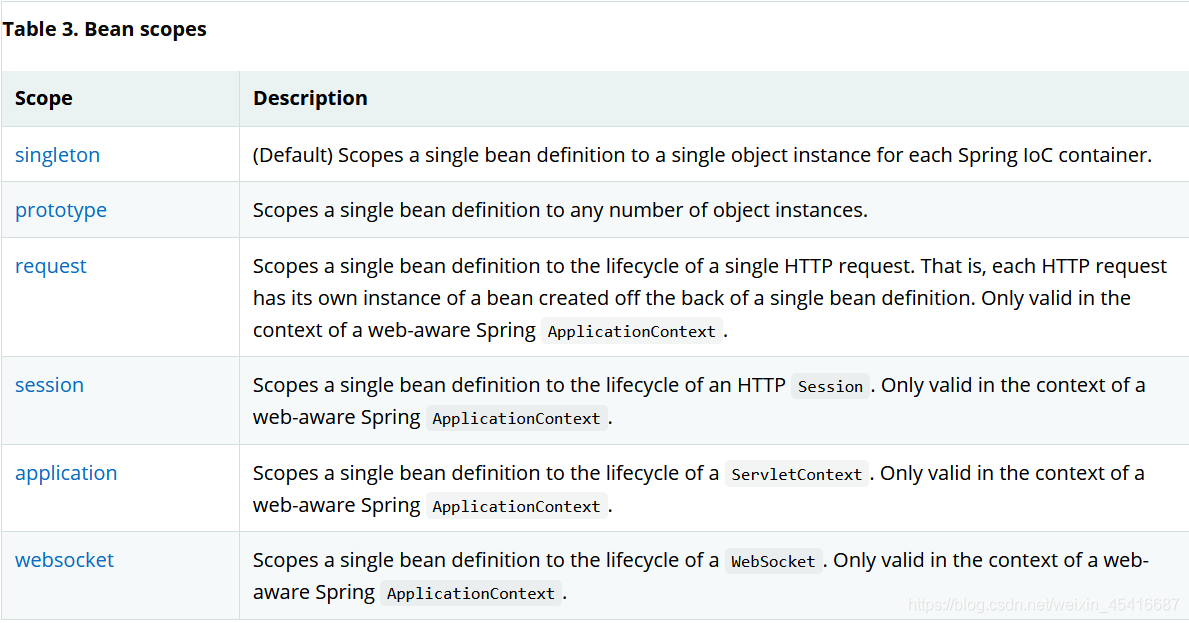
1.单例模式(Spring默认机制)
<bean id="accountService" class="com.something.DefaultAccountService" scope="singleton"/>
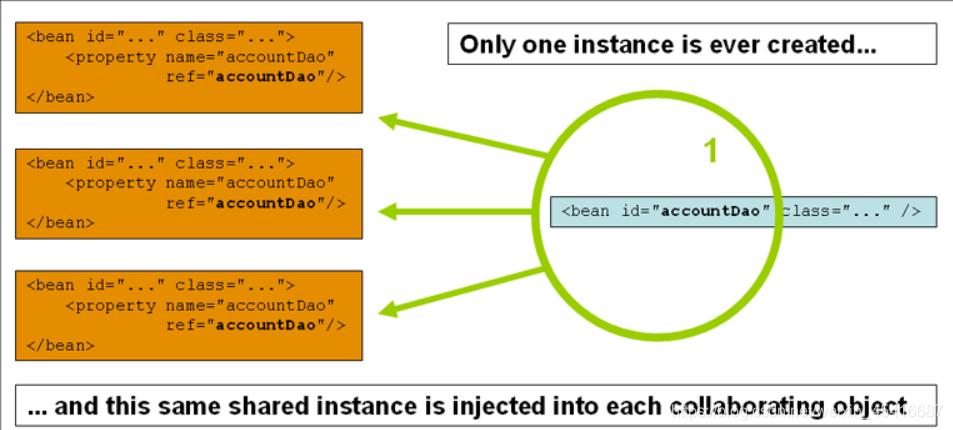
2.原型模式:每次从容器中get对象时,都重新创建
<bean id="accountService" class="com.something.DefaultAccountService" scope="prototype"/>
3.其余的request、session、application、websocket这些只能在web开发中使用
5 Bean的自动装配
- 自动装配是spring满足bean依赖的一种方式
- Spring会在上下文中自动寻找,并自动给bean装配属性
在Spring中由三种装配方式
- 在xml中显式配置
- 在java中显式配置
- 隐式的自动装配bean
5.1 byName与byType自动装配
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="cat" class="com.meng.pojo.Cat"/>
<bean id="dog" class="com.meng.pojo.Dog"/>
<bean id="people" class="com.meng.pojo.People" autowire="byName">
<property name="name" value="yang"/>
</bean>
<bean id="people1" class="com.meng.pojo.People" autowire="byType">
<property name="name" value="yang"/>
</bean>
</beans>
5.2 使用注解实现自动装配
jdk1.5支持的注解 Spring2.5支持的注解 使用注解须知: 1.导入约束:context约束
xmlns:context=“http://www.springframework.org/schema/context”
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
2.配置注解的支持context:annotation-config
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd">
<context:annotation-config/>
</beans>
5.3 @Autowired与@Resource
@Autowired 直接在属性上使用即可!也可以在set方式上使用 使用Autowired可以不用编写set方法了,前提是你这个自动装配的属性在IOC(Spring)容器中存在,且符合名字byName
拓展:
@Nullable 字段标记了这个注解,说明这个字段可以为null;@Nullable 字段标记了这个注解,说明这个字段可以为null;
public class People {
@Autowired(required = false)
private Cat cat;
@Autowired
private Dog dog;
private String name;
如果@Autowired自动装配的环境比较复杂,自动装配无法通过一个注解【@Autowired】完成的时候,我们可以使用@Qualifier(value = “xxx”)去配置@Autowired的使用,指定一个唯一的bean对象注入!
public class People {
@Autowired(required = false)
private Cat cat;
@Autowired
@Qualifier(value = "xxx")
private Dog dog;
private String name;
}
@Resource注解,不指定name值,先去判断byName和byType,有一个能注入即成功
public class People {
@Resource(name = "xxxx")
private Cat cat;
}
小结:@Resource和@Autowired的区别
都是用来自动装配的,都可以放在属性字段上 @Autowired通过byType的方式实现,而且必须要求这个对象存在! @Resource默认通过byName的方式实现,如果找不到名字,则通过byType实现!如果两个都找不到的情况下,就报错! 执行顺序不同:@Autowired通过byType的方式实现。@Resource默认通过byName的方式实现。
5.4 使用注解开发
在Spring4之后,要使用注解开发,必须保证导入aop的包
使用注解需要导入context约束,增加注解的支持!
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
https://www.springframework.org/schema/context/spring-context.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd">
<context:annotation-config/>
<context:component-scan base-package="com.meng.pojo"/>
</beans>
1. bean注入使用@Componet注解
@Component
public class User {
String name;
}
2. 属性注入使用@Value注解
//@Component 等价于<bean id="user" class="com.meng.pojo.User"/>
@Component
public class User {
String name;
//@Value("yang") 相当于<property name="name" value="yang"/>
@Value("yang")
public void setName(String name) {
this.name = name;
}
}
- 衍生注解
@Componet有几个衍生注解,我们在web开发中,会按照mvc三层架构分层!
- dao层 【@Repository】
- service层 【@Service】
- controller层 【@Controller】
这四个注解功能都是一样的,都是代表将某个类注册到Spring中,装配Bean
4.自动装配
@Autowired 自动装配通过类型、名字
如果Autowired不能唯一自动装配上属性,则需要通过@Qualifier(value="xxx")
@Nullable 字段标记了这个注解,说明这个字段可以为null
@Resource 自动装配通过名字,类型
-
作用域 @Scope(“singleton”)单例 -
小结 XML 与 注解
xml更加万能,适用于任何场合!维护简单方便 注解不是自己类使用不了, 维护相对复杂 XML 与 注解最佳实践 xml用来管理bean 注解只负责完成属性的注入 我们在使用过程中,只需要注意一个问题:必须让注解生效,就需要开启注解的支持
5.5 使用java的方式配置Spring
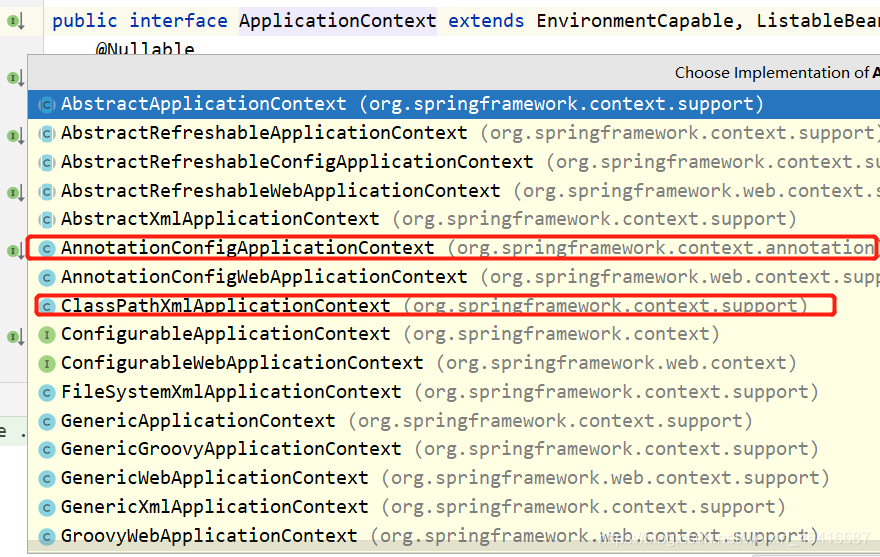
实体类
package com.meng.pojo;
import org.springframework.beans.factory.annotation.Value;
import org.springframework.stereotype.Component;
@Component
public class User {
private String name;
public String getName() {
System.out.println(name);
return name;
}
@Value("李四")
public void setName(String name) {
this.name = name;
}
}
配置类
import com.meng.pojo.User;
import org.springframework.beans.factory.annotation.Configurable;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Import;
@Configurable
@ComponentScan("com.meng.entity")
@Import(com.meng.pojo.User.class)
public class MengConfig {
@Bean
public User getUser() {
return new User();
}
}
测试类
ApplicationContext applicationContext = new ClassPathXmlApplicationContext("beans.xml");
User user = applicationContext.getBean("user", User.class);
user.getName();
AnnotationConfigApplicationContext context =
new AnnotationConfigApplicationContext(MengConfig.class);
User user1 = (User) context.getBean("getUser");
user1.getName();
System.out.println(user == user1);
**注意:**在Component中(@Component标注的类,包括@Service,@Repository, @Controller)使用@Bean注解和在@Configuration中使用是不同的。在@Component类中使用方法或字段时不会使用CGLIB增强(及不使用代理类:调用任何方法,使用任何变量,拿到的是原始对象,后面会有例子解释)。而在@Configuration类中使用方法或字段时则使用CGLIB创造协作对象(及使用代理:拿到的是代理对象);当调用@Bean注解的方法时它不是普通的Java语义,而是从容器中拿到由Spring生命周期管理、被Spring代理甚至依赖于其他Bean的对象引用。在@Component中调用@Bean注解的方法和字段则是普通的Java语义,不经过CGLIB处理
6 代理模式
6.1静态代理

角色分析:
抽象角色(租房事件):一般会使用接口或者抽象类来解决 真实角色(房东):被代理的角色 代理角色(中介):代理真实角色,代理真实角色后,我们一般会做一些附属操作 客户:访问代理对象的人 代码步骤:
接口 真实角色 代理角色 客户端访问代理角色
代理模式的好处:
可以使真实角色的操作更加存粹!不用去关注一些公共的业务 公共交给了代理角色,实现了业务的分工 公共业务发生扩展的时候,方便集中管理 缺点:
一个真实角色就会产生一个代理角色,代码量会翻倍 开发效率变低
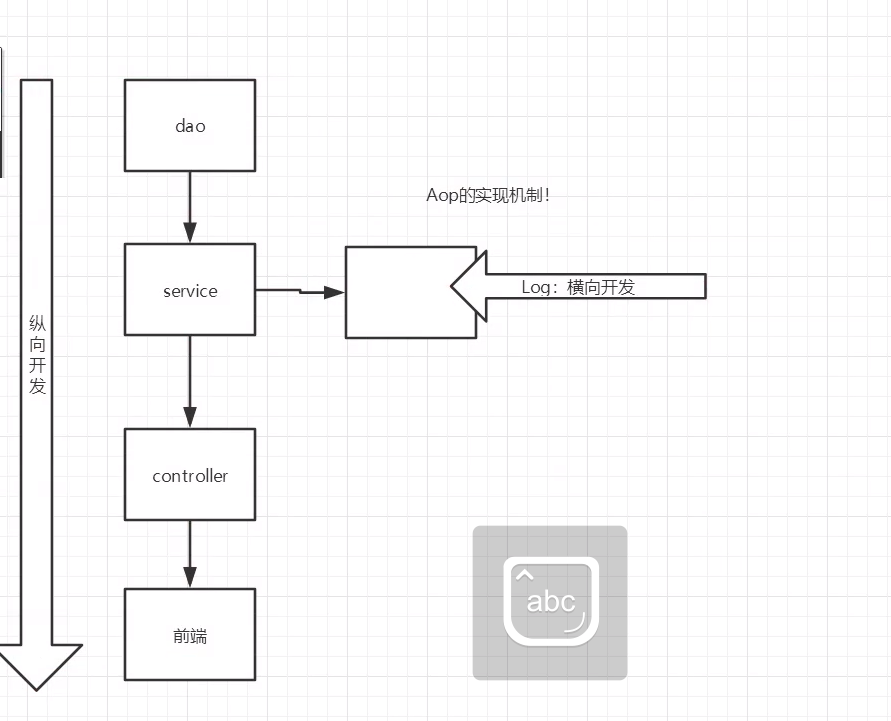
6.2 动态代理
动态代理和静态代理角色一样 动态代理的代理类是动态生成的,不是我们直接写好的 动态代理分为两大类:基于接口的动态代理,基于类的动态代理 基于接口:JDK动态代理 基于类: cglib java字节码实现: javasist 需要了解两个类:Proxy:代理 InvocationHandler:调用处理程序
Proxy
java.lang.reflect.Proxy Proxy:这是一个静态类,类里边有方法得到代理类
动态代理类 (以下简称为代理类 )是一个实现在类创建时在运行时指定的接口列表的类,具有如下所述的行为。 代理接口是由代理类实现的接口。 代理实例是代理类的一个实例。 每个代理实例都有一个关联的调用处理程序对象,它实现了接口InvocationHandler 。 通过其代理接口之一的代理实例上的方法调用将被分派到实例调用处理程序的invoke方法,传递代理实例, java.lang.reflect.Method被调用方法的java.lang.reflect.Method对象以及包含参数的类型Object Object的数组。 调用处理程序适当地处理编码方法调用,并且返回的结果将作为方法在代理实例上调用的结果返回。
代理类具有以下属性:
代理类是公共的,最终的,而不是抽象的,如果所有代理接口都是公共的。 如果任何代理接口是非公开的,代理类是非公开的,最终的,而不是抽象的 。 代理类的不合格名称未指定。 然而,以字符串"$Proxy"开头的类名空间应该保留给代理类。 一个代理类扩展了java.lang.reflect.Proxy 。 代理类完全按照相同的顺序实现其创建时指定的接口。 如果一个代理类实现一个非公共接口,那么它将被定义在与该接口相同的包中。 否则,代理类的包也是未指定的。 请注意,程序包密封不会阻止在运行时在特定程序包中成功定义代理类,并且类也不会由同一类加载器定义,并且与特定签名者具有相同的包。 由于代理类实现了在其创建时指定的所有接口, getInterfaces在其类对象上调用getInterfaces将返回一个包含相同列表接口的数组(按其创建时指定的顺序),在其类对象上调用getMethods将返回一个数组的方法对象,其中包括这些接口中的所有方法,并调用getMethod将在代理接口中找到可以预期的方法。 Proxy.isProxyClass方法将返回true,如果它通过代理类 - 由Proxy.getProxyClass返回的类或由Proxy.newProxyInstance返回的对象的类 - 否则为false。 所述java.security.ProtectionDomain代理类的是相同由引导类装载程序装载系统类,如java.lang.Object ,因为是由受信任的系统代码生成代理类的代码。 此保护域通常将被授予java.security.AllPermission 。 每个代理类有一个公共构造一个参数,该接口的实现InvocationHandler ,设置调用处理程序的代理实例。 而不必使用反射API来访问公共构造函数,也可以通过调用Proxy.newProxyInstance方法来创建代理实例,该方法将调用Proxy.getProxyClass的操作与调用处理程序一起调用构造函数。
InvocationHandler
InvocationHandler是由代理实例的调用处理程序实现的接口 。 每个代理实例都有一个关联的调用处理程序。 当在代理实例上调用方法时,方法调用将被编码并分派到其调用处理程序的invoke方法。 invoke(Object proxy, 方法 method, Object[] args) 处理代理实例上的方法调用并返回结果。
编写实例
接口
public interface UserServiceInterface {
public void add();
public void delete();
public void update();
public void select();
}
接口实现类
public class UserServiceImpl implements UserServiceInterface {
@Override
public void add() {
System.out.println("增加一个用户");
}
@Override
public void delete() {
System.out.println("删除一个用户");
}
@Override
public void update() {
System.out.println("修改一个用户");
}
@Override
public void select() {
System.out.println("查询一个用户");
}
}
创建代理工具类
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
public class ProxyInvocationHandler implements InvocationHandler {
private Object target;
public void setTarget (Object target) {
this.target = target;
}
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
log(method.getName());
Object invoke = method.invoke(target, args);
return invoke;
}
public Object getProxy () {
return Proxy.newProxyInstance(this.getClass().getClassLoader(),
target.getClass().getInterfaces(), this);
}
public void log (String msg) {
System.out.println("执行了" + msg + "方法");
}
}
测试类
public class Client {
public static void main(String[] args) {
UserServiceImpl userService = new UserServiceImpl();
ProxyInvocationHandler proxyInvocationHandler = new ProxyInvocationHandler();
proxyInvocationHandler.setTarget(userService);
UserServiceInterface proxy = (UserServiceInterface)proxyInvocationHandler.getProxy();
proxy.add();
}
}
7.AOP
7.1 什么是AOP
AOP(Aspect Oriented Programming)意为:面向切面编程,通过预编译方式和运行期动态代理实现程序功能的统一维护的一种技术,AOP是OOP的延续,是软件开发中的一个热点,也是Spring框架中的一个重要内容,是函数式编程的一种衍生泛型。利用AOP可以对业务逻辑的各个部分进行隔离,从而使得业务逻辑各部分之间的耦合度降低,提高程序的可重用性,同时提高了开发的效率。 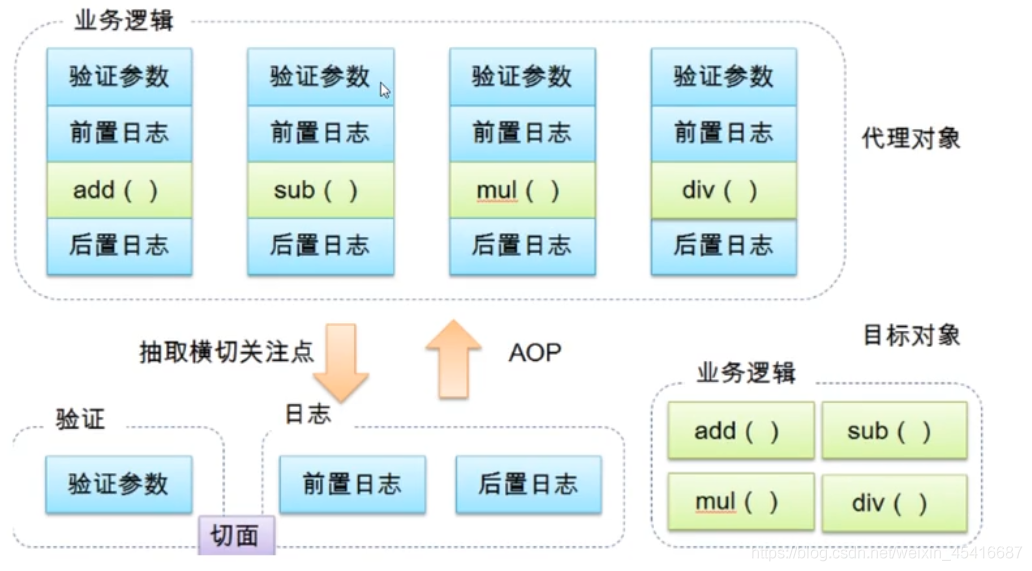
7.2 Aop在Spring中的作用
提供生命事务:允许用户自定义切面
横切关注点:跨越应用程序多个模块的方法或功能。即与我们的业务逻辑无关的,但是我们需要关注的部分,就是横切关注点。如日志,安全,缓存,事务等等。。。 切面(ASPECT):横切关注点 被模块化的特殊对象。即 它是一个类 通知(Advice):切面必须要完成的工作,即 他是类中的一个方法 目标(target):被通知的对象 代理(Proxy):向目标对象应用通知之后创建的对象 切入点(PointCut):切面通知 执行的"地点"的定义 连接点(jointPoint):与切入点匹配的执行点 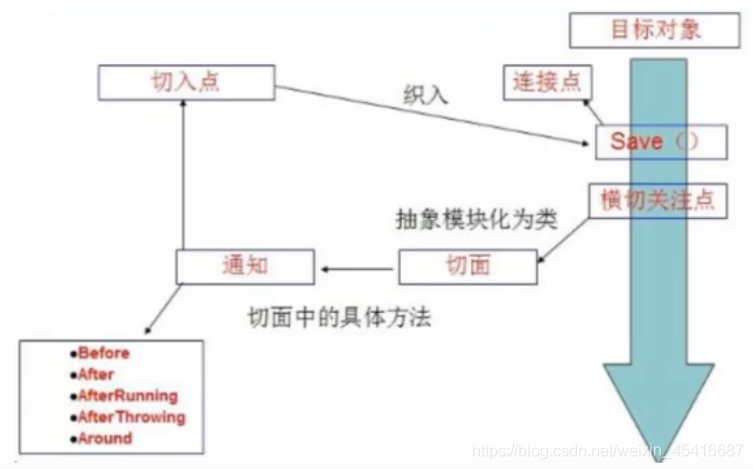
SpringAop中,通过Advice定义横切逻辑,Spring中支持的5种类型的Advice

7.3 使用Spring实现Aop
【重点】使用AOP织入,需要依赖包
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.4</version>
</dependency>
方式一:使用Spring的API接口 在执行UserService实现类的所有方法时,增加日志功能
UserServer接口
public interface UserService {
public void add();
public void update();
public void delete();
public void select();
}
UserServer实现类
public class UserServiceImpl implements UserService{
public void add() {
System.out.println("增加了一个用户");
}
public void update() {
System.out.println("更新了一个用户");
}
public void delete() {
System.out.println("删除了一个用户");
}
public void select() {
System.out.println("检索了一个用户");
}
}
Log类
import org.springframework.aop.AfterReturningAdvice;
import org.springframework.aop.MethodBeforeAdvice;
import java.lang.reflect.Method;
public class Log implements MethodBeforeAdvice, AfterReturningAdvice {
public void before(Method method, Object[] args, Object target) throws Throwable {
System.out.println(target.getClass().getName() + "的" + method.getName() + "被执行了");
}
public void afterReturning(Object returnValue, Method method, Object[] args, Object target) throws Throwable {
System.out.println("执行了" + method.getName() + "方法,返回值为" + returnValue);
}
}
配置文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="userService" class="com.meng.service.UserServiceImpl"/>
<bean id="log" class="com.meng.pojo.Log"/>
<aop:config>
<aop:pointcut id="pointcut" expression="execution(* com.meng.service.UserServiceImpl.*(..))"/>
<aop:advisor advice-ref="log" pointcut-ref="pointcut"/>
</aop:config>
</beans>
测试类
ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml");
UserServiceInterface userService = context.getBean("userService", UserServiceInterface.class);
userService.add();
}
方式二:自定义来实现AOP【主要是切面定义】
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="userService" class="com.meng.service.UserServiceImpl"/>
<bean id="log" class="com.meng.log.Log"/>
<bean id="diy" class="com.meng.diy.DiyPointCut"/>
<aop:config>
<aop:aspect ref="diy">
<aop:pointcut id="point" expression="execution(* com.meng.service.UserServiceImpl.*(..))"/>
<aop:before method="beforeMethod" pointcut-ref="point"/>
</aop:aspect>
</aop:config>
</beans>
自定义类
public class DiyPointCut {
public void beforeMethod () {
System.out.println("方法执行之前");
}
}
方式三:使用注解实现AOP XML文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd">
<bean id="userService" class="com.meng.service.UserServiceImpl"/>
<aop:aspectj-autoproxy proxy-target-class="false"/>
<bean id="annotationPointCut" class="com.meng.diy.AnnotationPointcut"/>
<beans/>
@Aspect
public class AnnotationPointcut {
@Before("execution(* com.yang.service.UserServiceImpl.*(..))")
public void before () {
System.out.println("====方法执行前====");
}
@After("execution(* com.yang.service.UserServiceImpl.*(..))")
public void after () {
System.out.println("====方法执行后====");
}
@Around("execution(* com.yang.service.UserServiceImpl.*(..))")
public void around (ProceedingJoinPoint pjp) throws Throwable {
System.out.println("环绕前");
Signature signature = pjp.getSignature();
System.out.println("signature" + signature);
Object proceed = pjp.proceed();
System.out.println("环绕后");
}
}

8.整合Mybatis
8.1整合Mybatis方式一
步骤:
- 导入相关jar包
junit mybatis mysql数据库 spring相关的 aop织入 mybatis-spring
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<parent>
<artifactId>spring-study</artifactId>
<groupId>com.yang</groupId>
<version>1.0-SNAPSHOT</version>
</parent>
<modelVersion>4.0.0</modelVersion>
<artifactId>spring-10-mybatis</artifactId>
<dependencies>
<dependency>
<groupId>junit</groupId>
<artifactId>junit</artifactId>
<version>4.13</version>
<scope>test</scope>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
<version>5.1.47</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.2</version>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.2.0.RELEASE</version>
</dependency>
<!--Spring操作数据库的话,还需要一个spring-jdbc-->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-jdbc</artifactId>
<version>5.3.9</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjweaver</artifactId>
<version>1.9.4</version>
</dependency>
<dependency>
<groupId>org.aspectj</groupId>
<artifactId>aspectjrt</artifactId>
<version>1.8.13</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.2</version>
</dependency>
<dependency>
<groupId>org.projectlombok</groupId>
<artifactId>lombok</artifactId>
<version>1.16.10</version>
</dependency>
</dependencies>
<build>
<resources>
<resource>
<directory>src/main/java</directory>
<includes>
<include>**/*.xml</include>
</includes>
<filtering>true</filtering>
</resource>
</resources>
</build>
</project>
可能存在找不到xml配置文件,需要配置< build >
- 编写配置文件
spring-dao.xml配置数据源DataSource与sqlSession
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="dataSource" class="org.springframework.jdbc.datasource.DriverManagerDataSource">
<property name="driverClassName" value="com.mysql.jdbc.Driver"/>
<property name="url"
value="jdbc:mysql://localhost:3306/mybatis?useSSL=true&useUnicode=false&characterEncoding=utf-8"/>
<property name="username" value="root"/>
<property name="password" value="123456"/>
</bean>
<bean class="org.mybatis.spring.SqlSessionFactoryBean" id="sqlSessionFactory">
<property name="dataSource" ref="dataSource"></property>
<property name="configLocation" value="classpath:mybatis-config.xml"></property>
<property name="mapperLocations" value="classpath:com/meng/dao/*.xml"></property>
</bean>
<bean id="sqlSession" class="org.mybatis.spring.SqlSessionTemplate">
<constructor-arg index="0" ref="sqlSessionFactory"></constructor-arg>
</bean>
</beans>
mybatis-config.xml配置一些mybatis专属配置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
<typeAliases>
<package name="com.meng.pojo"/>
</typeAliases>
</configuration>
applicationContext.xml整合与注册bean等
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd">
<import resource="spring-dao.xml"/>
<bean id="userMapper" class="com.meng.dao.UserMapperImpl">
<property name="sqlSessionTemplate" ref="sqlSession"/>
</bean>
</beans>
- 测试
实体类
package com.meng.pojo;
import lombok.Data;
@Data
public class User {
private int id;
private String name;
private int pwd;
}
mapper接口
package com.meng.dao;
import com.meng.pojo.User;
import java.util.List;
public interface UserMapper {
public List<User> selectUser();
}
mapper实现类
package com.meng.dao;
import com.meng.pojo.User;
import org.mybatis.spring.SqlSessionTemplate;
import java.util.List;
public class UserMapperImpl implements UserMapper {
private SqlSessionTemplate sqlSessionTemplate;
public void setSqlSessionTemplate(SqlSessionTemplate sqlSessionTemplate) {
this.sqlSessionTemplate = sqlSessionTemplate;
}
@Override
public List<User> selectUser() {
UserMapper mapper = sqlSessionTemplate.getMapper(UserMapper.class);
return mapper.selectUser();
}
}
mapper映射文件
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Config 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.meng.dao.UserMapper">
<select id="selectUser" resultType="user">
select *
from users;
</select>
</mapper>
测试类
import com.meng.dao.UserMapper;
import com.meng.pojo.User;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
import java.io.IOException;
import java.util.List;
public class MyTest {
@Test
public void test() throws IOException {
ApplicationContext classPathXmlApplicationContext = new ClassPathXmlApplicationContext("applicationContext" +
".xml");
UserMapper userMapper = classPathXmlApplicationContext.getBean("userMapper", UserMapper.class);
List<User> userList = userMapper.selectUser();
for (User user : userList) {
System.out.println(user);
}
}
}
8.2整合Mybatis方式二
SqlSessionDaoSupport
SqlSessionDaoSupport 是一个抽象的支持类,用来为你提供 SqlSession。调用 getSqlSession() 方法你会得到一个 SqlSessionTemplate,之后可以用于执行 SQL 方法
public class UserMapperImpl2 extends SqlSessionDaoSupport implements UserMapper {
public List<User> selectUser() {
SqlSession sqlSession = getSqlSession();
UserMapper mapper = sqlSession.getMapper(UserMapper.class);
List<User> users = mapper.selectUser();
return users;
}
}
实际上是整合mybatis一与整合mybatis二是一样的方法,只不过二继承了SqlSessionDaoSupport ,在getSqlSession(),做的也是setSqlSessionTemplate
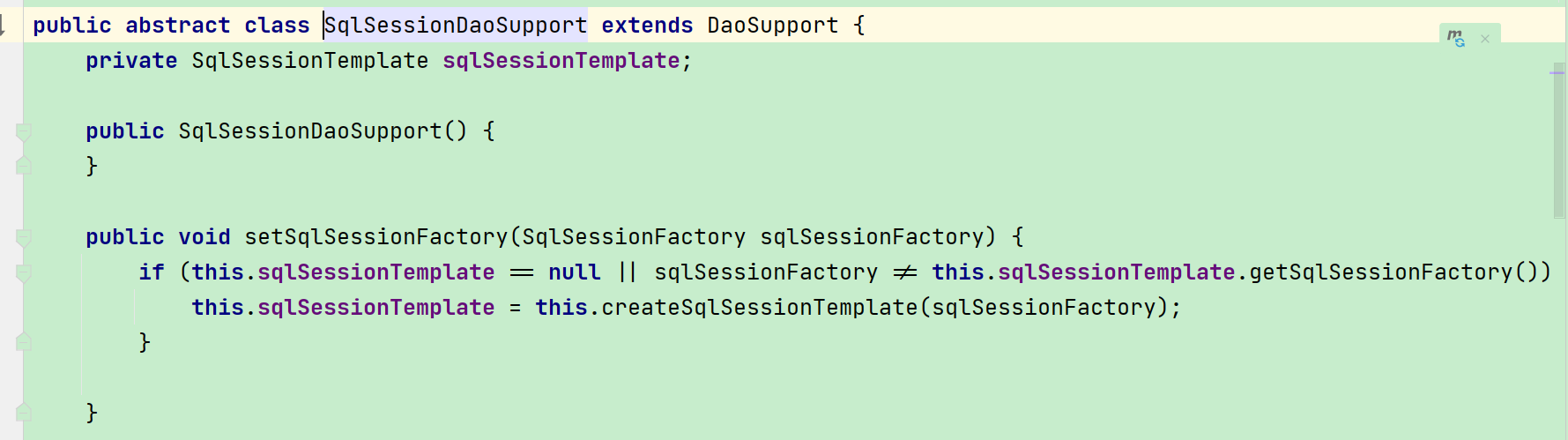
9.Spring配置声明事务注入
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:aop="http://www.springframework.org/schema/aop"
xmlns:tx="http://www.springframework.org/schema/tx"
xsi:schemaLocation="http://www.springframework.org/schema/beans
https://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/aop
https://www.springframework.org/schema/aop/spring-aop.xsd
http://www.springframework.org/schema/tx
http://www.springframework.org/schema/tx/spring-tx.xsd">
<import resource="spring-dao.xml"/>
<bean id="userMapper" class="com.yang.mapper.UserMapperImpl2">
<property name="sqlSessionTemplate" ref="sqlSession"/>
</bean>
<bean id="transactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="dataSource"/>
</bean>
<tx:advice id="txAdvice" transaction-manager="transactionManager">
<tx:attributes>
<tx:method name="add" propagation="REQUIRED"/>
<tx:method name="delete" propagation="REQUIRED"/>
<tx:method name="update" propagation="REQUIRED"/>
<tx:method name="select" read-only="true"/>
<tx:method name="*" propagation="REQUIRED"/>
</tx:attributes>
</tx:advice>
<aop:config>
<aop:pointcut id="txPointCut" expression="execution(* com.yang.mapper.*.*(..))"/>
<aop:advisor advice-ref="txAdvice" pointcut-ref="txPointCut"/>
</aop:config>
</beans>
|