利用CryptoAPI加解密编程接口写一个简单的加解密程序
菜狗依旧觉得不简单,还是侵删~
了解CryptoAPI编程(crypto_api_doc.pdf),尝试用CryptoAPI或OpenSSL或其它加解密编程接口写一个简单的加解密程序(完成本地文件的加密解密,参考SFT的本地文件加解密功能,要有界面),开发工具不限。
开发环境:IDEA + Java1.8 + crypto库 + exe4j(将jar打包成可执行文件)
缺陷:打包好的exe文件,直接运行,会有中文乱码的问题出现,但是在IDEA中直接运行时不存在问题,不晓得是为什么。
运行界面(主要):
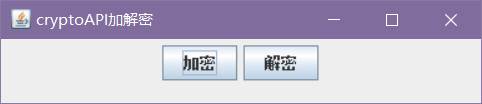
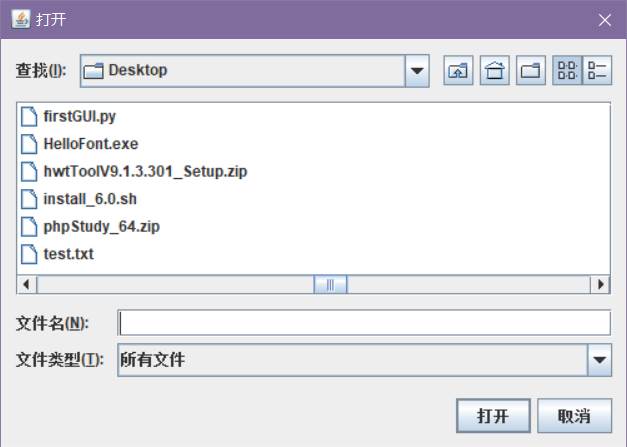
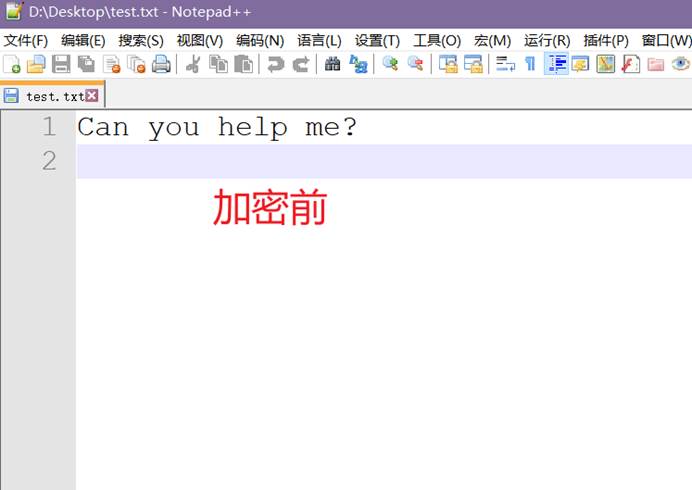
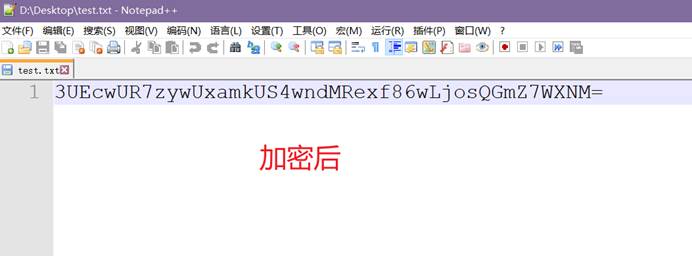
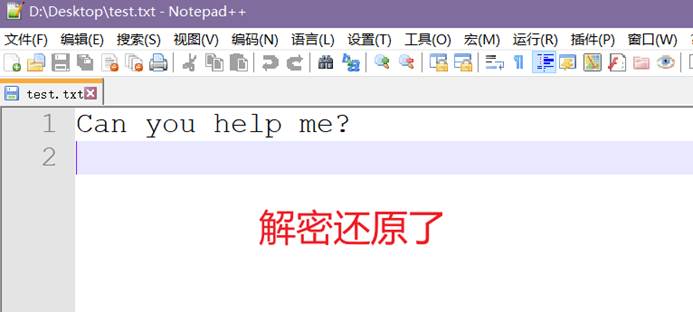
主要代码:
import javax.crypto.spec.*;
import javax.swing.*;
import java.awt.event.*;
import javax.crypto.*;
import java.io.*;
import java.security.*;
import java.util.*;
class cryptoAPI {
private JButton button1;
private JButton button2;
private JPanel panel1;
public static String key;
public static String iv;
public static void main(String[] args) {
key = generateAESKey();
iv = generateAESIv();
JFrame frame = new JFrame("cryptoAPI加解密");
frame.setSize(400,90);
frame.setLocationRelativeTo(null);
frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
JPanel panel1 = new JPanel();
panel1.setSize(400,90);
JButton button1 = new JButton("加密");
button1.addActionListener(new encryptListener());
JButton button2 = new JButton("解密");
button2.addActionListener(new decryptListener());
panel1.add(button1);
panel1.add(button2);
frame.setContentPane(panel1);
frame.setVisible(true);
}
static class encryptListener implements ActionListener{
JFrame frame;
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("加密");
JFileChooser chooser = new JFileChooser(".");
chooser.showOpenDialog(frame);
String filePath = chooser.getSelectedFile().getAbsolutePath();
System.out.println(filePath);
File file = new File(filePath);
FileReader fileReader = null;
try {
fileReader = new FileReader(file);
} catch (FileNotFoundException fileNotFoundException) {
fileNotFoundException.printStackTrace();
}
BufferedReader br = new BufferedReader(fileReader);
StringBuilder sb = new StringBuilder();
String temp = "";
while (true) {
try {
if (!((temp = br.readLine()) != null)) break;
} catch (IOException ioException) {
ioException.printStackTrace();
}
sb.append(temp + "\n");
}
try {
br.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
String context = sb.toString();
String encryptContext = null;
try {
encryptContext = encryptAES(context.getBytes(),key,iv);
} catch (NoSuchPaddingException noSuchPaddingException) {
noSuchPaddingException.printStackTrace();
} catch (NoSuchAlgorithmException noSuchAlgorithmException) {
noSuchAlgorithmException.printStackTrace();
} catch (InvalidKeyException invalidKeyException) {
invalidKeyException.printStackTrace();
} catch (BadPaddingException badPaddingException) {
badPaddingException.printStackTrace();
} catch (IllegalBlockSizeException illegalBlockSizeException) {
illegalBlockSizeException.printStackTrace();
} catch (InvalidAlgorithmParameterException invalidAlgorithmParameterException) {
invalidAlgorithmParameterException.printStackTrace();
}
System.out.println("原文:"+context);
try {
if(!file.exists()) {
file.createNewFile();
}
FileWriter fileWriter =new FileWriter(file);
fileWriter.write("");
fileWriter.flush();
fileWriter.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
System.out.println("清空成功");
System.out.println("密文:"+encryptContext);
try {
if(!file.exists()) {
file.createNewFile();
}
FileWriter fileWriter =new FileWriter(file);
fileWriter.write(encryptContext);
fileWriter.flush();
fileWriter.close();
} catch (IOException ioException) {
ioException.printStackTrace();
}
}
}
static class decryptListener implements ActionListener{
JFrame frame;
@Override
public void actionPerformed(ActionEvent e) {
System.out.println("解密");
JFileChooser chooser = new JFileChooser(".");
chooser.showOpenDialog(frame);
String filePath = chooser.getSelectedFile().getAbsolutePath();
System.out.println(filePath);
File file = new File(filePath);
FileReader fileReader = null;
try {
fileReader = new FileReader(file);
} catch (FileNotFoundException fileNotFoundException) {
fileNotFoundException.printStackTrace();
}
BufferedReader br = new BufferedReader(fileReader);
StringBuilder sb = new StringBuilder();
String temp = "";
while (true) {
try {
if (!((temp = br.readLine()) != null)) break;
} catch (IOException exception) {
exception.printStackTrace();
}
sb.append(temp);
}
try {
br.close();
} catch (IOException exception) {
exception.printStackTrace();
}
String encryptContext = sb.toString();
String decryptContext = null;
try {
decryptContext = decryptAES(encryptContext.getBytes(),key,iv);
} catch (NoSuchPaddingException noSuchPaddingException) {
noSuchPaddingException.printStackTrace();
} catch (NoSuchAlgorithmException noSuchAlgorithmException) {
noSuchAlgorithmException.printStackTrace();
} catch (InvalidKeyException invalidKeyException) {
invalidKeyException.printStackTrace();
} catch (BadPaddingException badPaddingException) {
badPaddingException.printStackTrace();
} catch (IllegalBlockSizeException illegalBlockSizeException) {
illegalBlockSizeException.printStackTrace();
} catch (InvalidAlgorithmParameterException invalidAlgorithmParameterException) {
invalidAlgorithmParameterException.printStackTrace();
} catch (UnsupportedEncodingException unsupportedEncodingException) {
unsupportedEncodingException.printStackTrace();
}
System.out.println("原文:"+encryptContext);
try {
if(!file.exists()) {
file.createNewFile();
}
FileWriter fileWriter =new FileWriter(file);
fileWriter.write("");
fileWriter.flush();
fileWriter.close();
} catch (IOException exception) {
exception.printStackTrace();
}
System.out.println("清空成功");
System.out.println("密文:"+encryptContext);
try {
if(!file.exists()) {
file.createNewFile();
}
FileWriter fileWriter =new FileWriter(file);
fileWriter.write(decryptContext);
fileWriter.flush();
fileWriter.close();
} catch (IOException exception) {
exception.printStackTrace();
}
System.out.println("解密文:"+decryptContext);
}
}
static class ShowDialogLintener extends MouseAdapter {
JFrame frame;
public void ShowDialogLintener() {
}
@Override
public void mouseClicked(MouseEvent arg0) {
super.mouseClicked(arg0);
JFileChooser chooser = new JFileChooser(".");
chooser.showOpenDialog(frame);
String filePath = chooser.getSelectedFile().getAbsolutePath();
System.out.println(filePath);
}
}
private void createUIComponents() {
}
public static String generateAESKey(){
UUID uuid = UUID.randomUUID();
String aesKey = Base64.getEncoder().encodeToString(uuid.toString().getBytes()).substring(2,34);
return aesKey;
}
public static String generateAESIv(){
UUID uuid = UUID.randomUUID();
String iv = Base64.getEncoder().encodeToString(uuid.toString().getBytes()).substring(2,18);
return iv;
}
public static String encryptAES(byte[] content, String secretKeyStr, String iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException, InvalidAlgorithmParameterException {
SecretKeySpec secretKeySpec = new SecretKeySpec(secretKeyStr.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv.getBytes());
cipher.init(Cipher.ENCRYPT_MODE, secretKeySpec, ivParameterSpec);
return Base64.getEncoder().encodeToString(cipher.doFinal(content));
}
public static String decryptAES(byte[] content, String secretKeyStr, String iv) throws NoSuchPaddingException, NoSuchAlgorithmException, InvalidKeyException, BadPaddingException, IllegalBlockSizeException, InvalidAlgorithmParameterException, UnsupportedEncodingException {
byte[] contentDecByBase64 = Base64.getDecoder().decode(content);
SecretKeySpec secretKeySpec = new SecretKeySpec(secretKeyStr.getBytes(), "AES");
Cipher cipher = Cipher.getInstance("AES/CBC/PKCS5Padding");
IvParameterSpec ivParameterSpec = new IvParameterSpec(iv.getBytes());
cipher.init(Cipher.DECRYPT_MODE, secretKeySpec, ivParameterSpec);
return new String(cipher.doFinal(contentDecByBase64), "utf8");
}
}
|