一、SpringBoot2 基础入门
1.1. 什么是 SpringBoot
Spring Boot makes it easy to create stand-alone, production-grade Spring based Applications that you can “just run”.
能快速的创建一个生产级别的 Spring 应用。
1.2. SpringBoot 优点
-
Create stand-alone Spring applications
-
Embed Tomcat, Jetty or Undertow directly (no need to deploy WAR files)
-
Provide opinionated ‘starter’ dependencies to simplify your build configuration
-
Automatically configure Spring and 3rd party libraries whenever possible
-
Provide production-ready features such as metrics, health checks, and externalized configuration
-
Absolutely no code generation and no requirement for XML configuration
1.3. SpringBoot 缺点
1.4. SpringBoot(2.5.5) 入门
1.4.1. 系统要求
- Java 8 以上 并且兼容 Java 17
- Spring Framework 5.3.10
- Maven 3.5 +
1.4.2. 配置 Maven
修改 Maven 的 settings.xml :
<mirrors>
<mirror>
<id>nexus-aliyun</id>
<mirrorOf>central</mirrorOf>
<name>Nexus aliyun.</name>
<url>http://maven.aliyun.com/nexus/content/groups/public</url>
</mirror>
</mirrors>
<profiles>
<profile>
<id>jdk-1.8</id>
<activation>
<activeByDefault>true</activeByDefault>
<jdk>1.8</jdk>
</activation>
<properties>
<maven.compiler.source>1.8</maven.compiler.source>
<maven.compiler.target>1.8</maven.compiler.target>
<maven.compiler.compilerVersion>1.8</maven.compiler.compilerVersion>
</properties>
</profile>
</profiles>
1.4.3. 创建 Hello World
需求:浏览器发送 /Hello,响应 Hello Spring Boot 2
1.4.3.1. 创建 Maven 工程并且引入依赖
pom.xml
<?xml version="1.0" encoding="UTF-8"?>
<project xmlns="http://maven.apache.org/POM/4.0.0"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd">
<modelVersion>4.0.0</modelVersion>
<groupId>cn.com.springboot.demo</groupId>
<artifactId>boot-01-helloworld</artifactId>
<version>1.0-SNAPSHOT</version>
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.5</version>
</parent>
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
</project>
1.4.3.2. 创建主程序
dir:cn.com.springboot.demo.MainApp.java
package cn.com.springboot.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApp {
public static void main(String[] args) {
SpringApplication.run(MainApp.class, args);
}
}
1.4.3.3. 创建业务类
dir:cn.com.springboot.demo.controller.HelloController.java
package cn.com.springboot.demo.controller;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class HelloController {
@RequestMapping("/hello")
public String handler() {
return "Hello Spring Boot";
}
}
1.4.3.4. 测试
直接运行主程序。
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.5.5)
2021-09-29 00:18:47.607 INFO 8248 --- [ main] cn.com.springboot.demo.MainApp : Starting MainApp using Java 1.8.0_121 on DESKTOP-LT8H9RE with PID 8248 (F:\IdeaProjects\boot-01-helloworld\target\classes started by wwwjy in F:\IdeaProjects\my-app)
2021-09-29 00:18:47.617 INFO 8248 --- [ main] cn.com.springboot.demo.MainApp : No active profile set, falling back to default profiles: default
2021-09-29 00:18:48.255 INFO 8248 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8080 (http)
2021-09-29 00:18:48.265 INFO 8248 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2021-09-29 00:18:48.265 INFO 8248 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.53]
2021-09-29 00:18:48.328 INFO 8248 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2021-09-29 00:18:48.328 INFO 8248 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 676 ms
2021-09-29 00:18:48.578 INFO 8248 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8080 (http) with context path ''
2021-09-29 00:18:48.586 INFO 8248 --- [ main] cn.com.springboot.demo.MainApp : Started MainApp in 1.252 seconds (JVM running for 1.529)
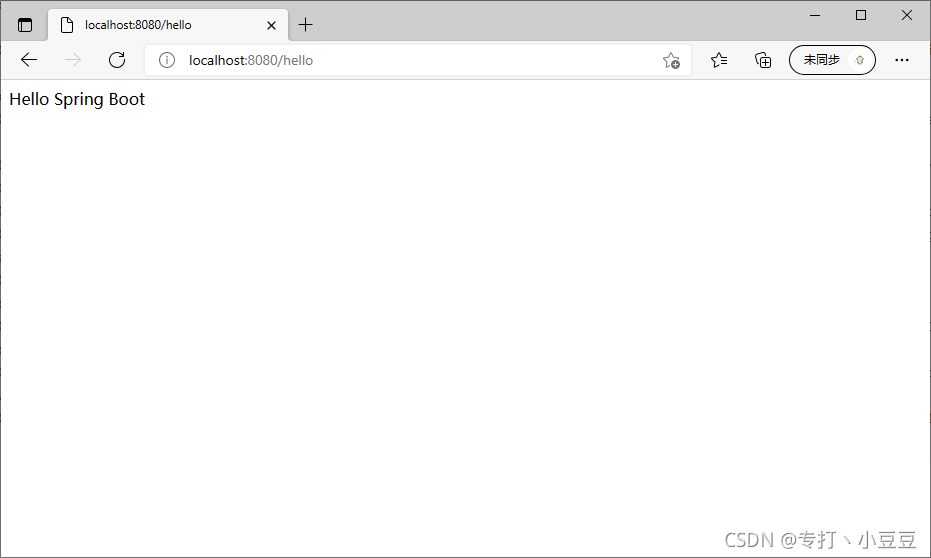
1.4.3.5. 简化配置
dir:resources/application.properties
server.port=8081
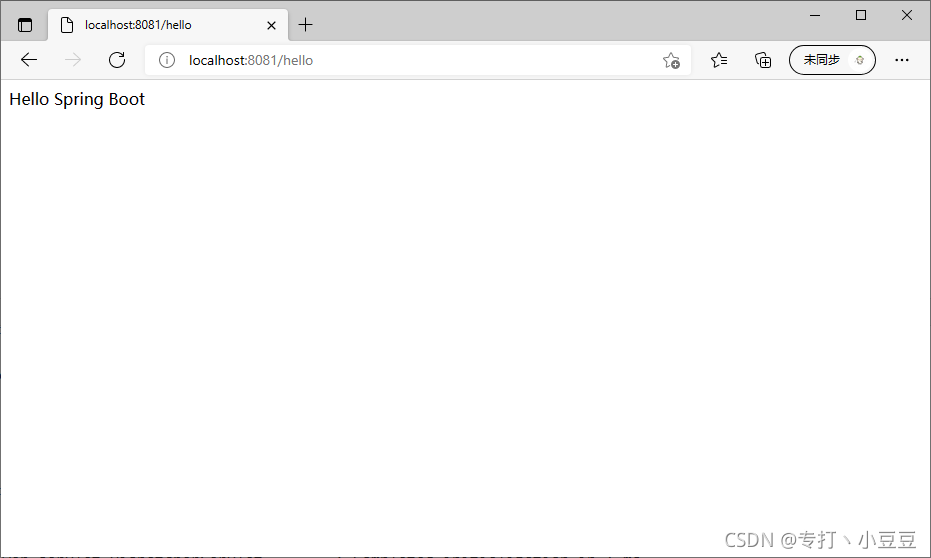
1.4.3.6. 简化部署
生成可执行 jar ,引入以下的依赖:
...
<build>
<plugins>
<plugin>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-maven-plugin</artifactId>
<version>2.5.5</version>
</plugin>
</plugins>
</build>
...
执行以下的命令:
mvn -clean
mvn -package
进入 jar 所在路径,执行以下的命令:
F:\IdeaProjects\boot-01-helloworld\target>java -jar boot-01-helloworld-1.0-SNAPSHOT.jar
. ____ _ __ _ _
/\\ / ___'_ __ _ _(_)_ __ __ _ \ \ \ \
( ( )\___ | '_ | '_| | '_ \/ _` | \ \ \ \
\\/ ___)| |_)| | | | | || (_| | ) ) ) )
' |____| .__|_| |_|_| |_\__, | / / / /
=========|_|==============|___/=/_/_/_/
:: Spring Boot :: (v2.5.5)
2021-09-29 00:25:34.990 INFO 2120 --- [ main] cn.com.springboot.demo.MainApp : Starting MainApp v1.0-SNAPSHOT using Java 1.8.0_121 on DESKTOP-LT8H9RE with PID 2120 (F:\IdeaProjects\boot
-01-helloworld\target\boot-01-helloworld-1.0-SNAPSHOT.jar started by wwwjy in F:\IdeaProjects\boot-01-helloworld\target)
2021-09-29 00:25:34.992 INFO 2120 --- [ main] cn.com.springboot.demo.MainApp : No active profile set, falling back to default profiles: default
2021-09-29 00:25:35.823 INFO 2120 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat initialized with port(s): 8081 (http)
2021-09-29 00:25:35.833 INFO 2120 --- [ main] o.apache.catalina.core.StandardService : Starting service [Tomcat]
2021-09-29 00:25:35.833 INFO 2120 --- [ main] org.apache.catalina.core.StandardEngine : Starting Servlet engine: [Apache Tomcat/9.0.53]
2021-09-29 00:25:35.881 INFO 2120 --- [ main] o.a.c.c.C.[Tomcat].[localhost].[/] : Initializing Spring embedded WebApplicationContext
2021-09-29 00:25:35.881 INFO 2120 --- [ main] w.s.c.ServletWebServerApplicationContext : Root WebApplicationContext: initialization completed in 848 ms
2021-09-29 00:25:36.156 INFO 2120 --- [ main] o.s.b.w.embedded.tomcat.TomcatWebServer : Tomcat started on port(s): 8081 (http) with context path ''
2021-09-29 00:25:36.164 INFO 2120 --- [ main] cn.com.springboot.demo.MainApp : Started MainApp in 1.504 seconds (JVM running for 1.831)
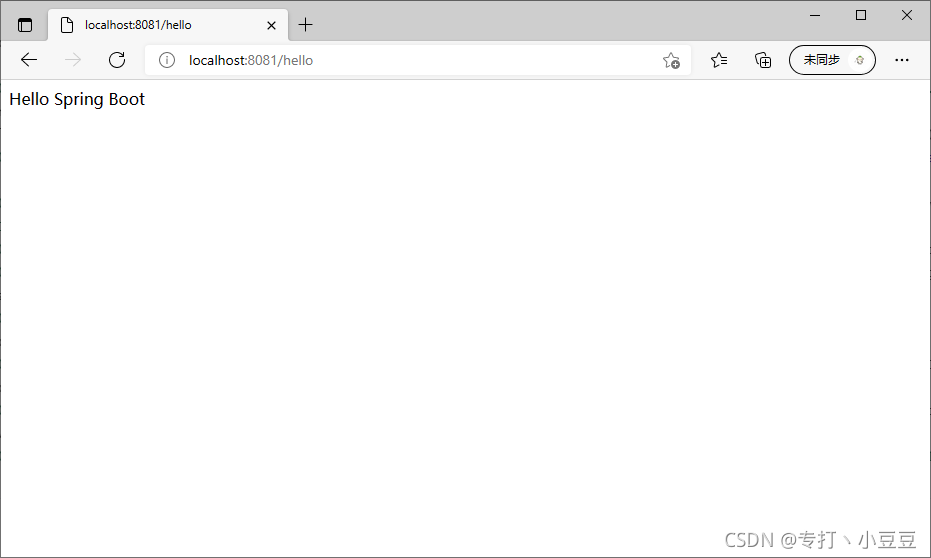
1.5. SpringBoot 特点
1.5.1. 依赖管理
1.5.1.1. 父项目做依赖管理
所有的项目(这里指:用 Maven 构建的项目)的 pom.xml 文件都会引入以下的内容:
...
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-parent</artifactId>
<version>2.5.5</version>
</parent>
...
这将导致下边的所有依赖都不需要指定版本号。如引入 web 开发的依赖:
...
<dependencies>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
</dependencies>
...
进入 spring-boot-starter-parent 中(Ctrl + 左键),发现它还引用了父项目:
...
<parent>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-dependencies</artifactId>
<version>2.5.5</version>
</parent>
...
进入 spring-boot-dependencies 中(Ctrl + 左键),它引入了所有的常规开发所需要的依赖。
1.5.1.2. 场景启动器
- spring-boot-starter-*:是官方定义的 spring-boot 启动器,只要引入对应的 starter ,那么该启动器所对应的所有依赖都会被自动引入
- *-spring-boot-starter:是第三方提供的启动器
- 所有启动器最底层都依赖,如下
...
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.5.5</version>
<scope>compile</scope>
</dependency>
...
1.5.1.3. 版本仲裁
因为在 spring-boot-dependencies 中配置了当前 spring-boot 版本(2.5.5)所支持依赖版本。
如:
...
<properties>
<activemq.version>5.16.3</activemq.version>
<antlr2.version>2.7.7</antlr2.version>
<appengine-sdk.version>1.9.91</appengine-sdk.version>
<artemis.version>2.17.0</artemis.version>
<aspectj.version>1.9.7</aspectj.version>
<assertj.version>3.19.0</assertj.version>
<atomikos.version>4.0.6</atomikos.version>
<awaitility.version>4.0.3</awaitility.version>
<build-helper-maven-plugin.version>3.2.0</build-helper-maven-plugin.version>
<byte-buddy.version>1.10.22</byte-buddy.version>
<caffeine.version>2.9.2</caffeine.version>
<cassandra-driver.version>4.11.3</cassandra-driver.version>
<classmate.version>1.5.1</classmate.version>
<commons-codec.version>1.15</commons-codec.version>
<commons-dbcp2.version>2.8.0</commons-dbcp2.version>
<commons-lang3.version>3.12.0</commons-lang3.version>
<commons-pool.version>1.6</commons-pool.version>
<commons-pool2.version>2.9.0</commons-pool2.version>
...
<mysql.version>8.0.26</mysql.version>
...
</properties>
...
所以引入的依赖都不需要写版本号,这就是 spring-boot 的自动版本仲裁机制。
如果不想使用 spring-boot 自动仲裁的版本
在当前项目(开发项目中)的 pom.xml 中,指定自己的依赖即可,如:
...
<properties>
<mysql.version>6.0.6</mysql.version>
</properties>
...
- <mysql.version> 一定要和 spring-boot-dependencies 中标签名相同
[外链图片转存失败,源站可能有防盗链机制,建议将图片保存下来直接上传(img-cMFJSSTK-1632929238416)(C:\Users\wwwjy\AppData\Roaming\Typora\typora-user-images\image-20210929230430351.png)]
1.5.2. 自动配置
1.5.2.1. 引入 spring-boot-starter-web
自动配置好了以下的内容:
- Tomcat
- SpringMVC
...
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-tomcat</artifactId>
<version>2.5.5</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-web</artifactId>
<version>5.3.10</version>
<scope>compile</scope>
</dependency>
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>5.3.10</version>
<scope>compile</scope>
</dependency>
...
1.5.2.2. 默认的包结构
主程序所在的包及其子包都会被扫描到。
示例:在主程序所在包外新建 controller
主程序
package cn.com.springboot.demo;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
@SpringBootApplication
public class MainApp {
public static void main(String[] args) {
SpringApplication.run(MainApp.class, args);
}
}
WorldController
package cn.com.springboot;
import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController;
@RestController
public class WorldController {
@RequestMapping("/world")
public String handler() {
return "Hello World.";
}
}
运行结果
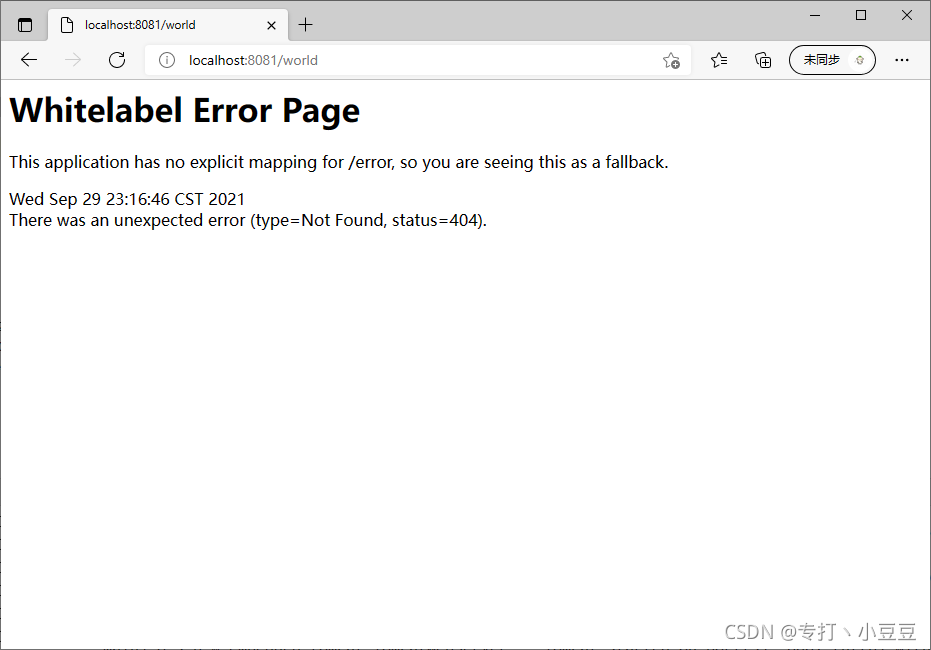
1.5.2.3. 默认配置都拥有默认值
- 所有的配置最终都映射到一个具体的 java 类上
- 配置文件的值都会绑定到对应的类上,这个类会在容器中创建对象
1.5.2.4. 按需加载自动配置项
- 引入哪个启动器,才会启用这个启动器的自动配置
- spring-boot 的所有自动配置都绑在 spring-boot-autoconfigure 包内
|