可以不让搞,但是不允许你不会
java环境配置
- jdk版本,jdk1.8+;
- IDE环境,ideas;
- 依赖包,web3;
- 区块链网路,开发网,Ganache;
基础概念
区块链网络: 提供区块链交易的网络,测试网和正式网由以太坊提供,开发网络可以本地安装,ganache,Hardhat是两个可以本地安装的区块链服务器,ganache是本教程使用的开发区块链服务器。 web3:与区块链进行交互的网络协议,web3目前有python,nodejs,java,typeScript等语言实现 gas:交易手续费,就是我们所说的(挖矿)费,区块链上的所有操作都由网络带宽存储的成本,这部分费用需要由交易方提供
java环境搭建
jdk1.8
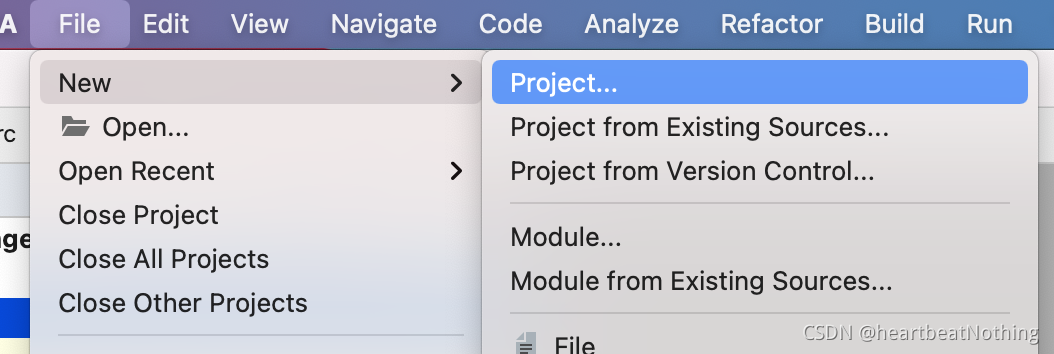 ideas创建java项目引导
file—>New—>Project
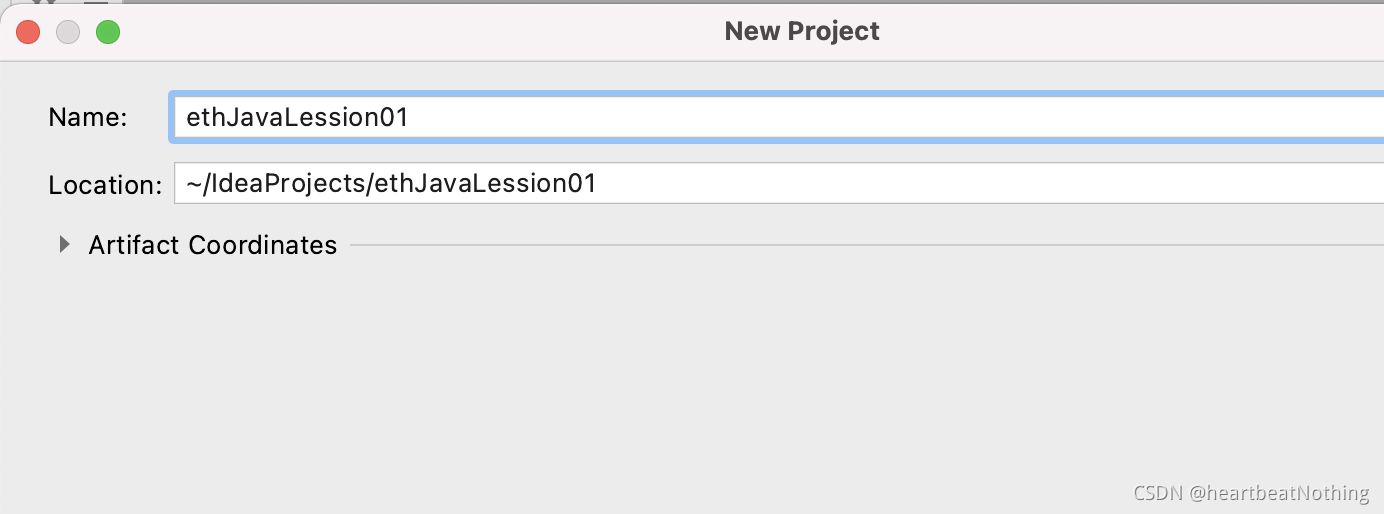 ideas创建java项目,命名 ethJavaLession01
maven包引用
<dependency>
<groupId>org.web3j</groupId>
<artifactId>core</artifactId>
<version>5.0.0</version>
</dependency>
<dependency>
<groupId>org.apache.commons</groupId>
<artifactId>commons-lang3</artifactId>
<version>3.12.0</version>
</dependency>
<repositories>
<repository>
<id>central</id>
<name>central</name>
<url>https://maven.aliyun.com/repository/central</url>
<releases>
<enabled>true</enabled>
</releases>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
<repository>
<id>jcenter</id>
<name>jcenter</name>
<url>http://jcenter.bintray.com/</url>
<releases>
<enabled>true</enabled>
</releases>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
<repository>
<id>maven-net-cn</id>
<name>Maven China Mirror</name>
<url>https://dl.bintray.com/ethereum/maven</url>
<releases>
<enabled>true</enabled>
</releases>
<snapshots>
<enabled>false</enabled>
</snapshots>
</repository>
</repositories>
java代码
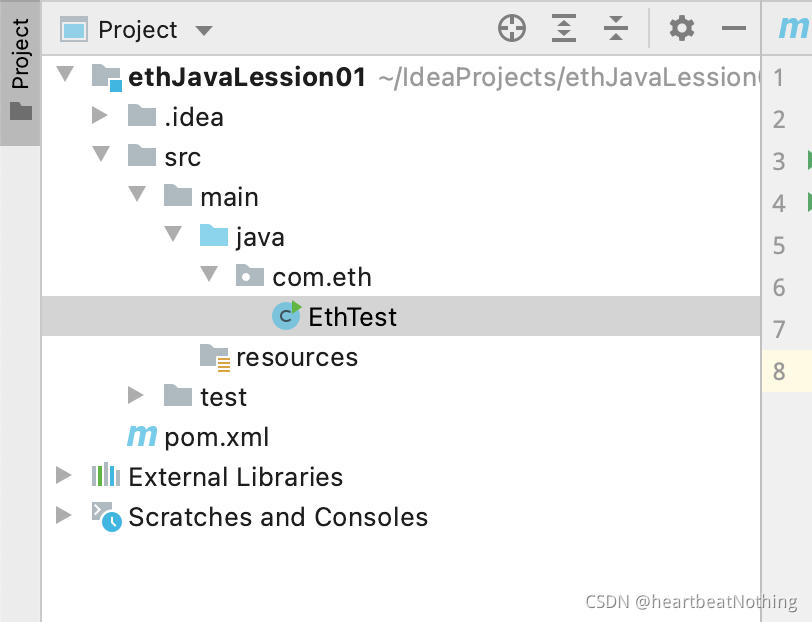
package com.eth;
import org.web3j.protocol.Web3j;
import org.web3j.protocol.core.methods.response.EthBlockNumber;
import org.web3j.protocol.core.methods.response.EthGasPrice;
import org.web3j.protocol.core.methods.response.Web3ClientVersion;
import org.web3j.protocol.http.HttpService;
import java.io.IOException;
public class EthTest {
public static void main(String[] args) {
System.out.println("Connecting to Ethereum ...");
Web3j web3 = Web3j.build(new HttpService("HTTP://127.0.0.1:7545"));
System.out.println("Successfuly connected to Ethereum");
try {
Web3ClientVersion clientVersion = web3.web3ClientVersion().send();
EthBlockNumber blockNumber = web3.ethBlockNumber().send();
EthGasPrice gasPrice = web3.ethGasPrice().send();
System.out.println("Client version: " + clientVersion.getWeb3ClientVersion());
System.out.println("Block number: " + blockNumber.getBlockNumber());
System.out.println("Gas price: " + gasPrice.getGasPrice());
} catch (IOException ex) {
throw new RuntimeException("Error whilst sending json-rpc requests", ex);
}
}
}
Ganache
Ganache为可以本地启动的开发区块链服务器 ganache官网地址
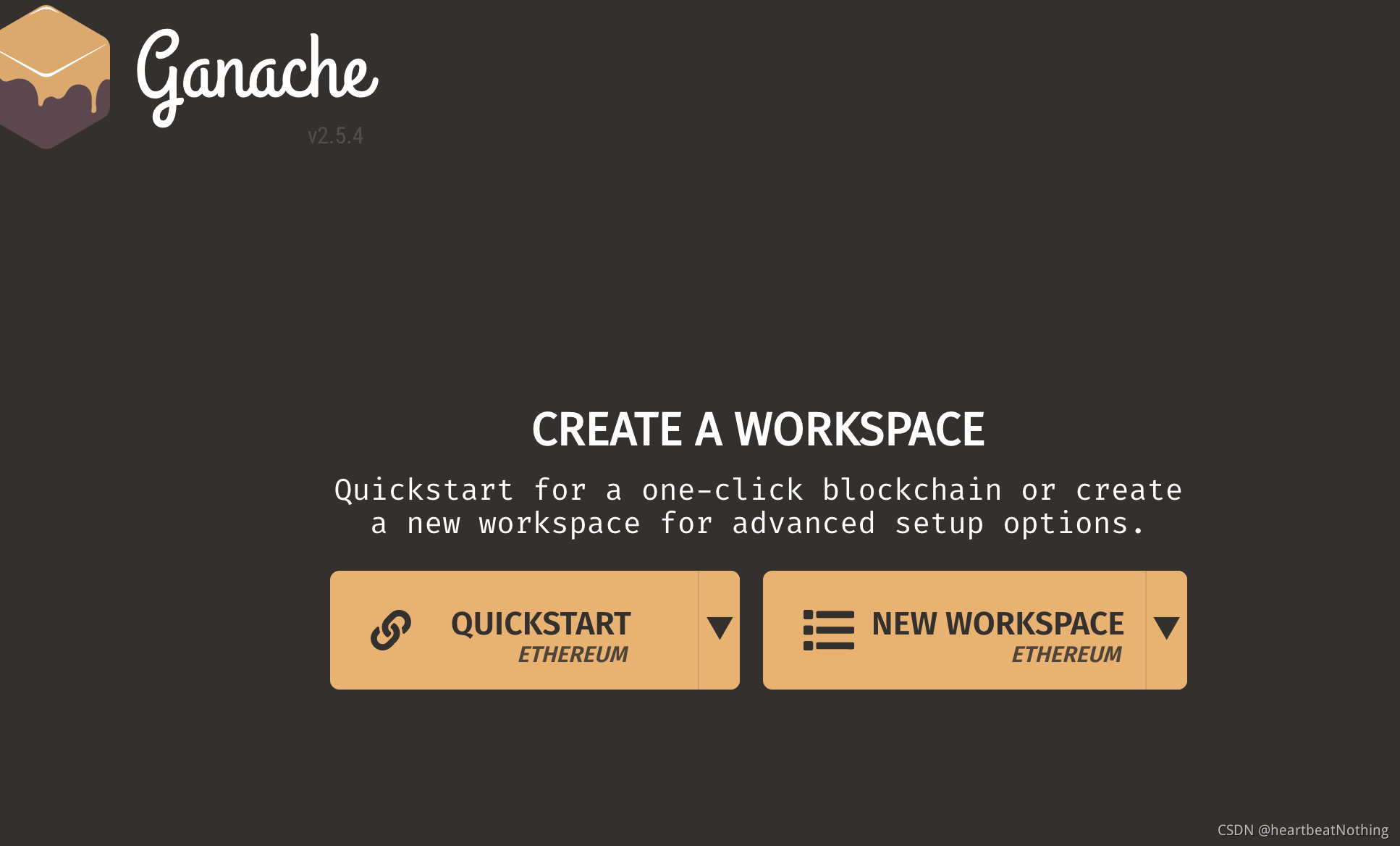 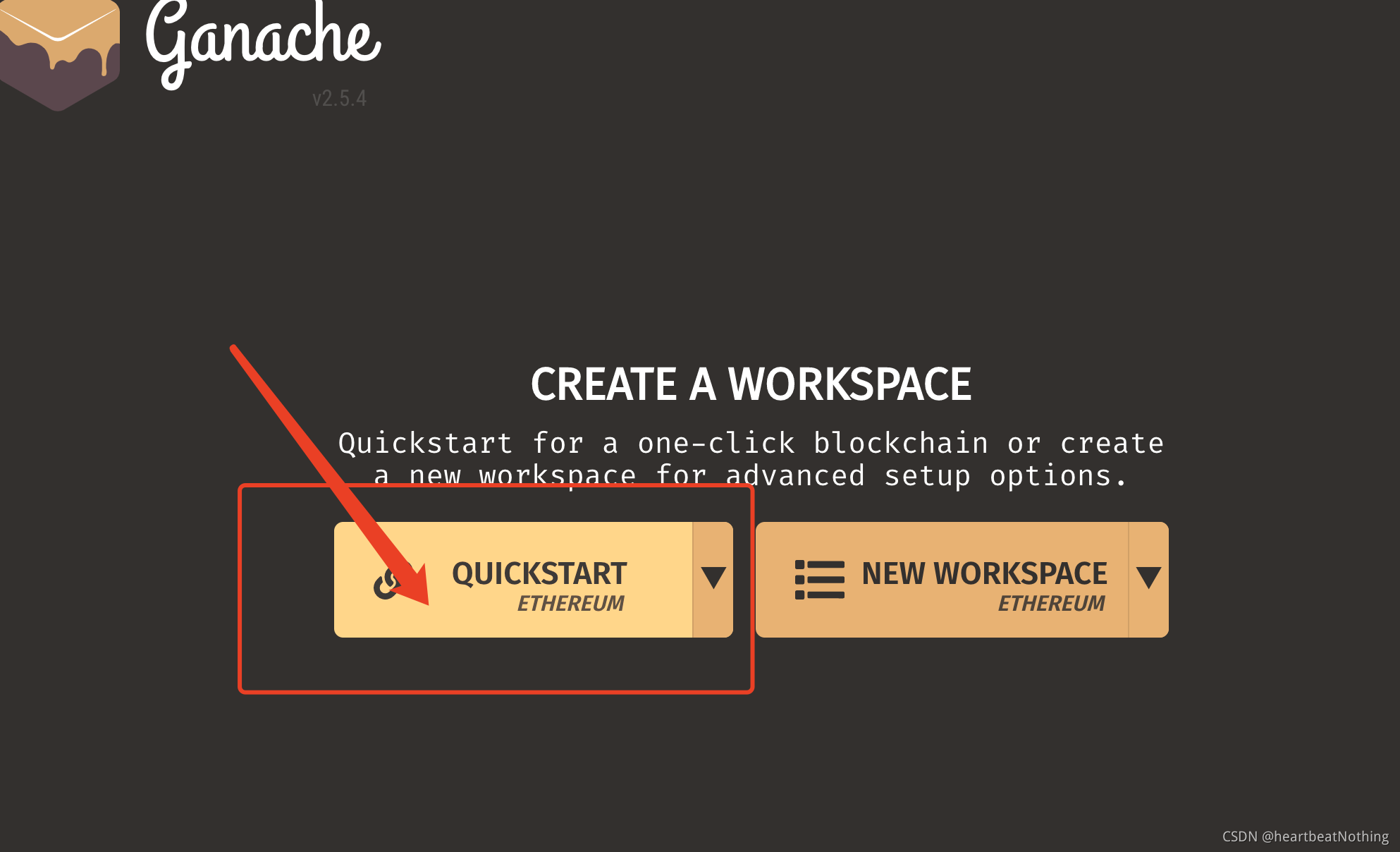 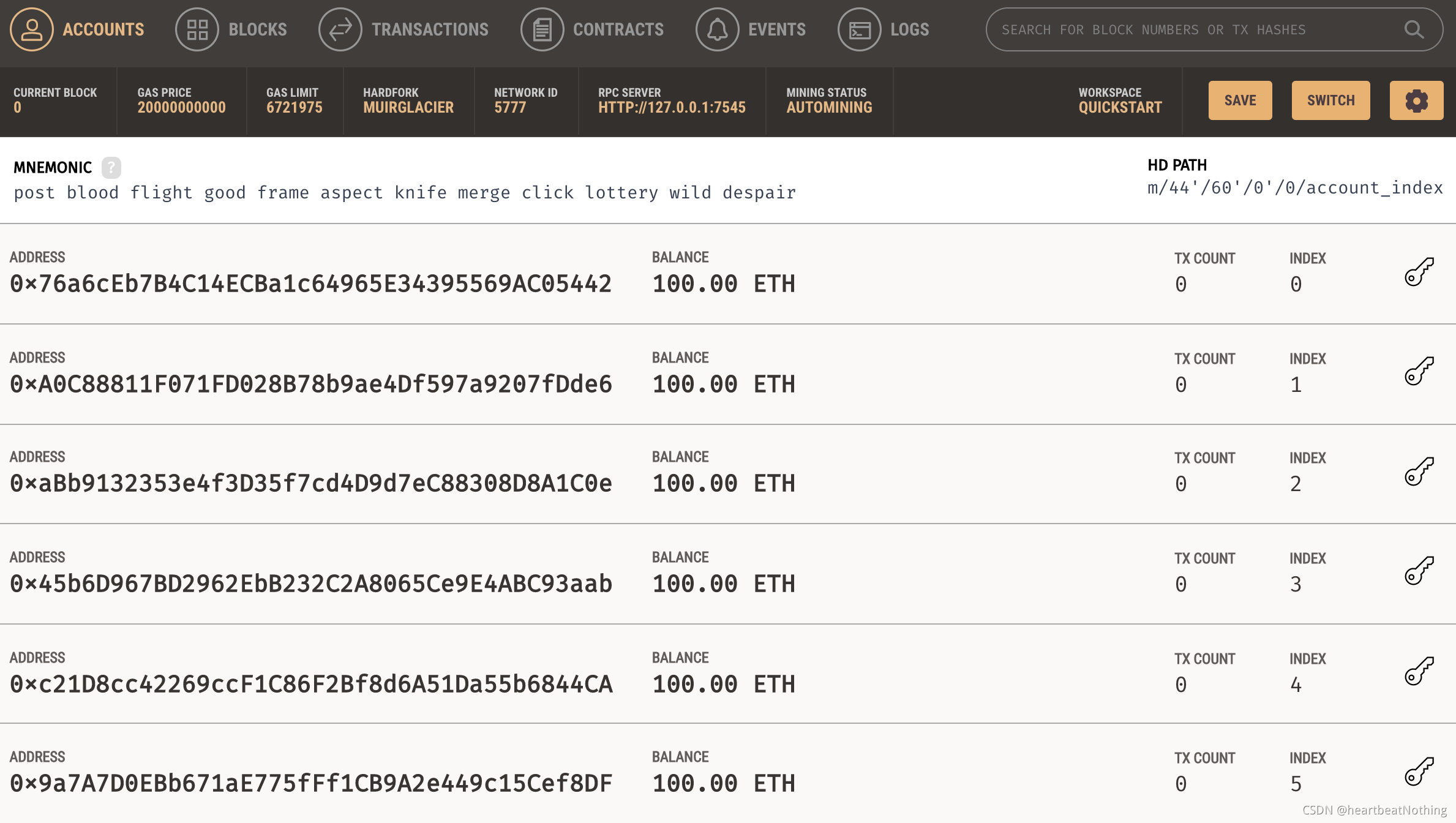 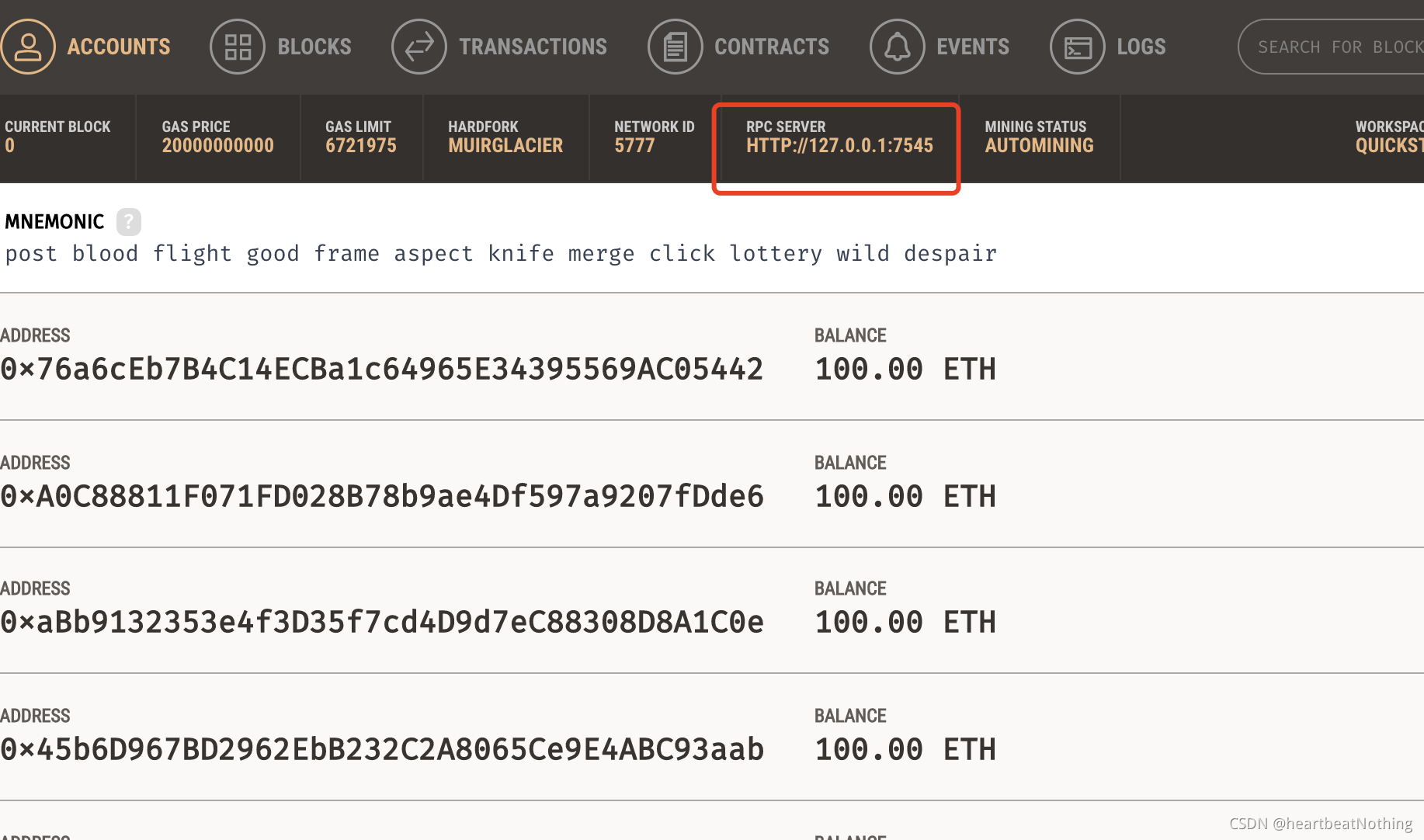
执行结果
Successfuly connected to Ethereum Client version: EthereumJS TestRPC/v2.13.1/ethereum-js Block number: 0 Gas price: 20000000000
代码解释
Web3j web3 = Web3j.build(new HttpService(“HTTP://127.0.0.1:7545”)) 连接区块链服务器
Web3ClientVersion clientVersion = web3.web3ClientVersion().send(); 客户端版本号
EthBlockNumber blockNumber = web3.ethBlockNumber().send(); 获取当前区块链中块个数
EthGasPrice gasPrice = web3.ethGasPrice().send(); 获取gas值
|