本文的配置:idea+Tomcat9.0+MySQL8.0
基本思路:通过java连接数据库,然后对其中的数据进行增删查改;
1. 后端:
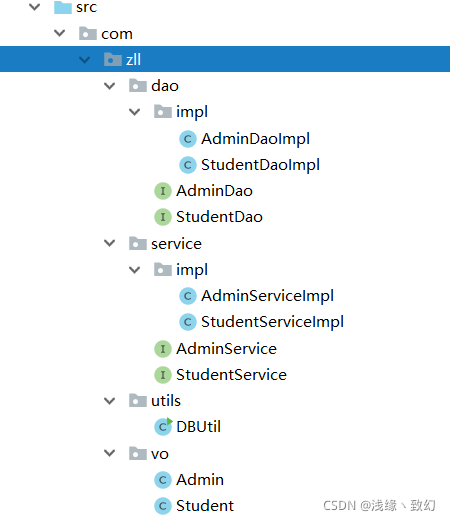
1.1.?util? 数据库的连接
注意要去更换对应的数据库连接的用户名和密码,还有建立的数据库名字也需要更换,不然找不到数据库;更改之后可以对数据库连接进行测试。
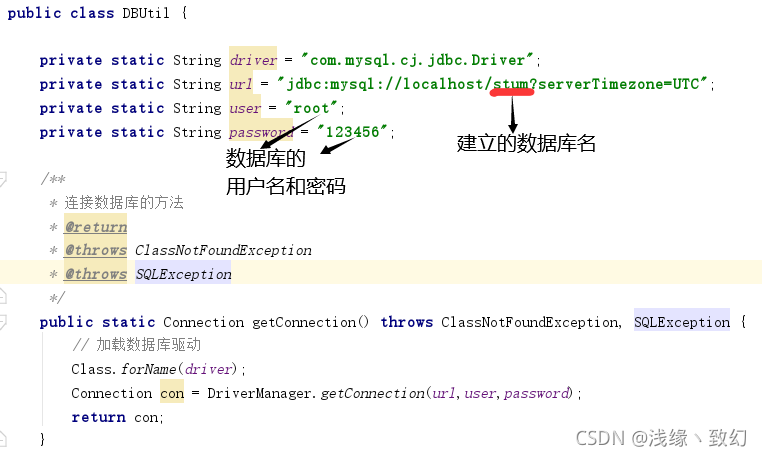
1.2. VO层是对管理员admin和学生student的set和get方法的定义;
1.3.?服务层service:以学生student为例,在studentservice接口中定义了增删查改的方法;并在studentserviceImpl中实现;注意本文中仅是依据id来对数据库的数据实现查询、修改和删除的,所以我将id设置为只可读的属性;
1.4. dao层:具体实现增删查改的功能
2.?Tomcat(服务器)的配置:依据你的电脑Tomcat版本进行配置
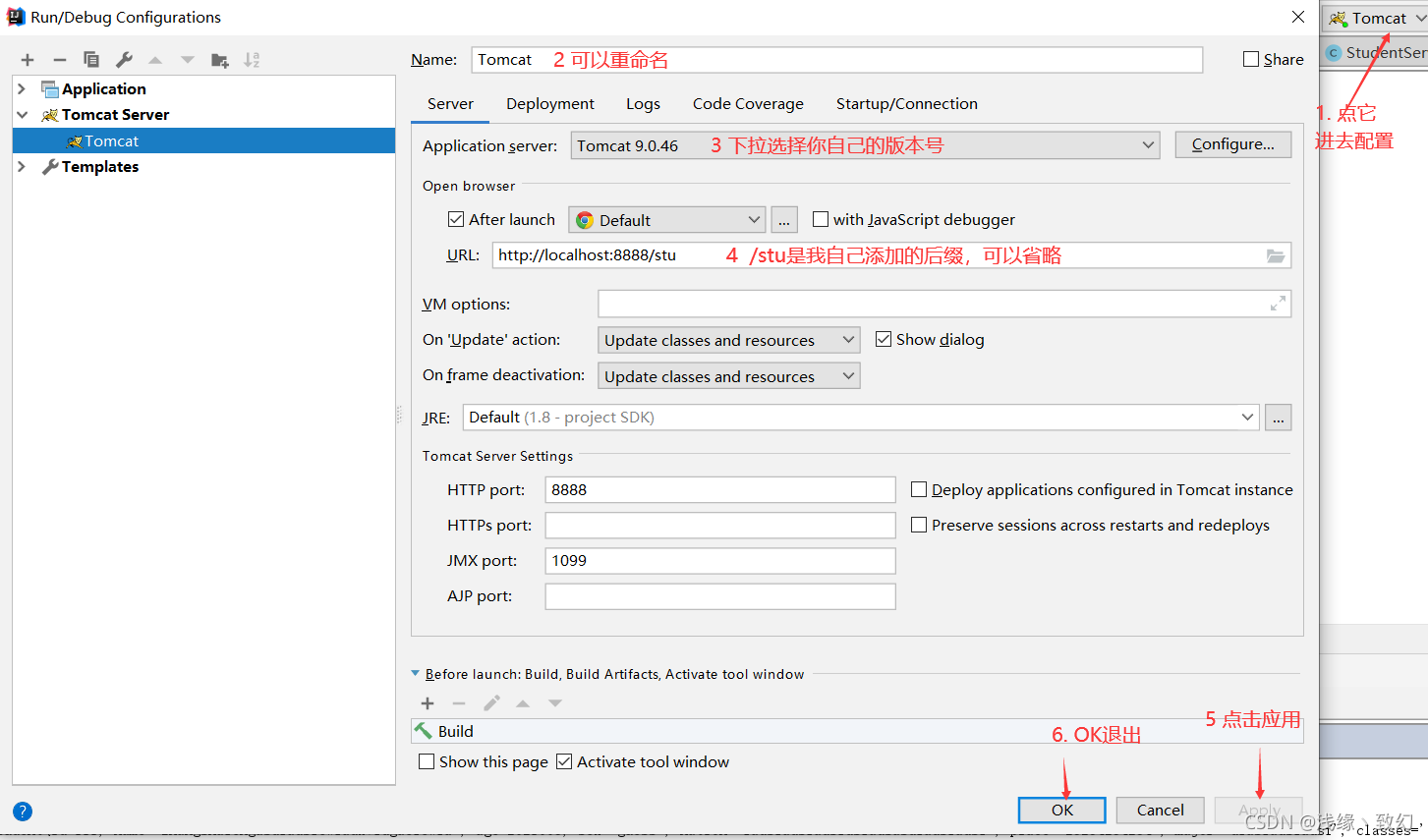
?3.?前端页面:
3.1?登录界面:
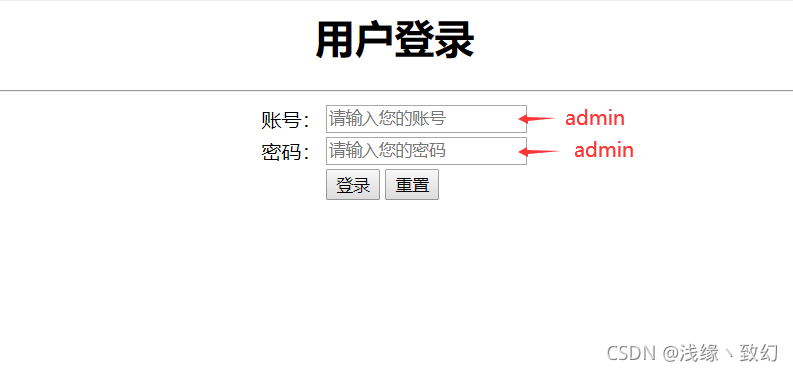
?3.2?主页面:
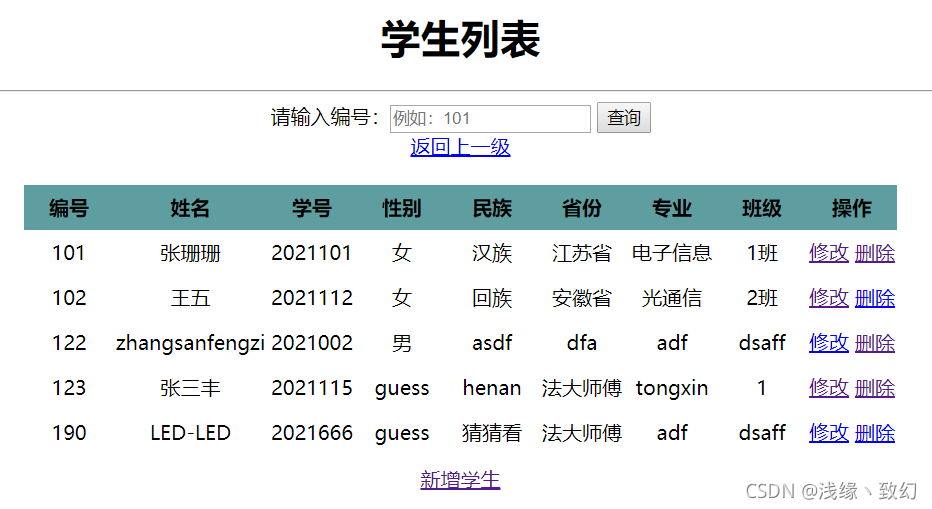
?3.3?添加页面:
对添加页面进行表单校验、对添加的姓名、编号等追加了条件限制;
例如:编号限制为100--199;姓名不能为空;学号限制为7位;
注意:这里说明一下为什么要添加限制,因为编号是int类型的,当你添加的内容为字符串时,会产生HTTP:500的报错;
?
报错页面:
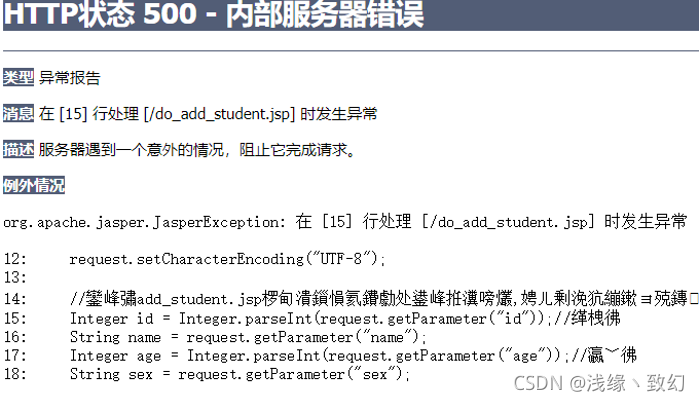
?
?
?
?add_student.jsp:
<%@ page contentType="text/html;charset=UTF-8" language="java" %>
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>新增学生</title>
<style type="text/css">
h1{
text-align: center;
}
div{
text-align: center;
}
#before{
text-align: center;
}
</style>
</head>
<body>
<%-- 头部 --%>
<jsp:include page="top.jsp"/>
<h1>新增学生</h1>
<hr/>
<div id="before">
<a href="javascript: window.history.go(-1)">返回上一级</a>
</div>
</br>
<form action="do_add_student.jsp" method="post" name="addForm">
<div>
<tr>
<label>编号:</label>
<input type="text" oninput="this.value=this.value.replace(/[^0-9]+/,'')" name="id" id="id" placeholder="例如:1xx" autofocus="autofocus">
</tr>
</div>
<div>
<tr>
<label>姓名:</label></td>
<input type="text" name="name" placeholder="例如:张三">
</tr>
</div>
<div>
<tr>
<label>学号:</label>
<input type="text" oninput="this.value=this.value.replace(/[^0-9]+/,'')" name="age" id="age" placeholder="例如:2021xxx">
</tr>
</div>
<div>
<tr>
<label>性别:</label>
<input type="text" name="sex" placeholder="例如:男/女">
</tr>
</div>
<div>
<tr>
<label>民族:</label>
<input type="text" name="nation" placeholder="例如:汉族">
</tr>
</div>
<div>
<tr>
<label>省份:</label>
<input type="text" name="place" placeholder="例如:安徽省">
</tr>
</div>
<div>
<tr>
<label>专业:</label>
<input type="text" name="major" placeholder="例如:电子信息">
</tr>
</div>
<div>
<tr>
<label>班级:</label>
<input type="text" name="classes" placeholder="例如:1班">
</tr>
</div>
<br>
<div id="submit">
<tr>
<button type="submit" onclick="return checkForm()">添加</button>
<button type="reset">重置</button>
</tr>
</div>
</form>
<script type="text/javascript">
function checkForm() {
var id = addForm.id.value;
var name = addForm.name.value;
var age = addForm.age.value;
// 编号、学号和姓名不能为空
if (id == "" || id == null || id<100 || id>199) {//编号不能为空,限制编号为3个数字(int类型)100 到 199
alert("请注意编号的格式,例如:101");
addForm.id.focus();
return false;
} else if (name == "" || name == null) {//姓名不能为空
alert("不能为空,请输入姓名");
addForm.name.focus();
return false;
}else if (age == "" || age == null || age.length != 7 ) {//学号不能为空,限制编号为3个数字(先把age当做学号来做)
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}/*else if (age == "" || age == null || age.length != 7) {//性别
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}else if (age == "" || age == null || age.length != 7) {//名族
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}else if (age == "" || age == null || age.length != 7) {//省份
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}else if (age == "" || age == null || age.length != 7) {//专业
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}else if (age == "" || age == null || age.length < 5) {//班级
alert("请注意学号的格式,例如:2021101");
addForm.name.focus();
return false;
}*/
alert('添加成功!');
return true;
}
</script>
<%-- 底部 <jsp:include page="bottom.jsp"/>
onKeyUp="this.value=this.value.replace(/[^\u4e00-\u9fa5]/g,'')" //限制输入为汉字
--%>
</body>
</html>
3.4?修改页面同理与添加页面:
它是在添加的基础上实现的;首先依据编号将学生的信息全部显示在修改页面,然后对除了编号以外的信息进行修改,这里我另外追加了限制,例如姓名中不能出现数字和字符;读者也可以自己添加对应的设置;
注意:这里说下为什么不能修改编号,因为本项目增删查改功能都依据这个编号,如果你修改了,在你返回表单时就会跳转到出错界面,导致修改失败。
3.5?删除页面:
删除页面与修改页面也是相同的道理,都是依据编号进行删除的,这里添加了一个删除提醒(确认你是否要删除的提示);
?
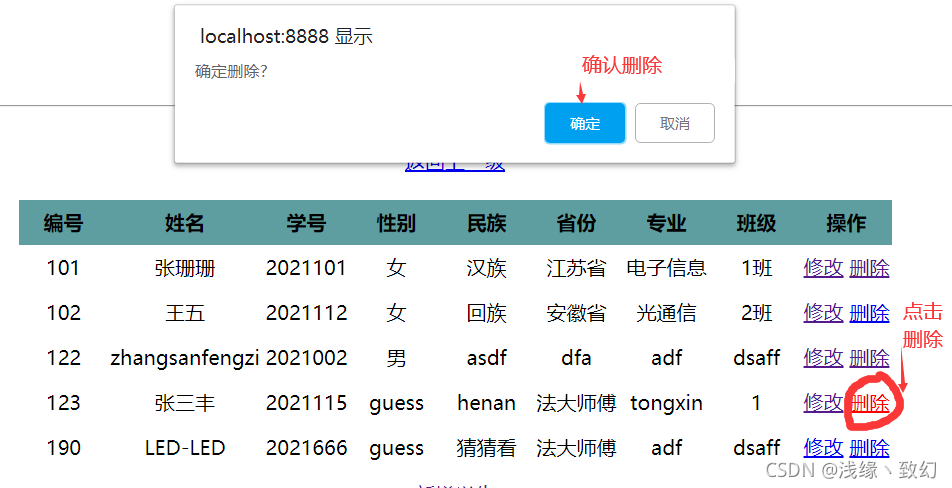
4.?总结:
首先要根据自己的数据库和Tomcat进行对应的配置;以确保项目可以在你的电脑上顺利运行;
对于添加页面、修改页面、删除页面的完善,读者可以有自己的思路进行改动;
需要源码留言或者私聊获取;
本文依据水坚石青学生管理系统改进而来,有不足之处还请指教,不胜感激!
|