1.修改springboot的banner
在springboot的资源目录下创建一个banner.txt文件,如图 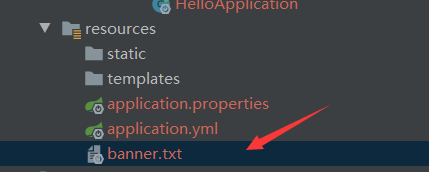 然后在txt文件中放入你的banner…… 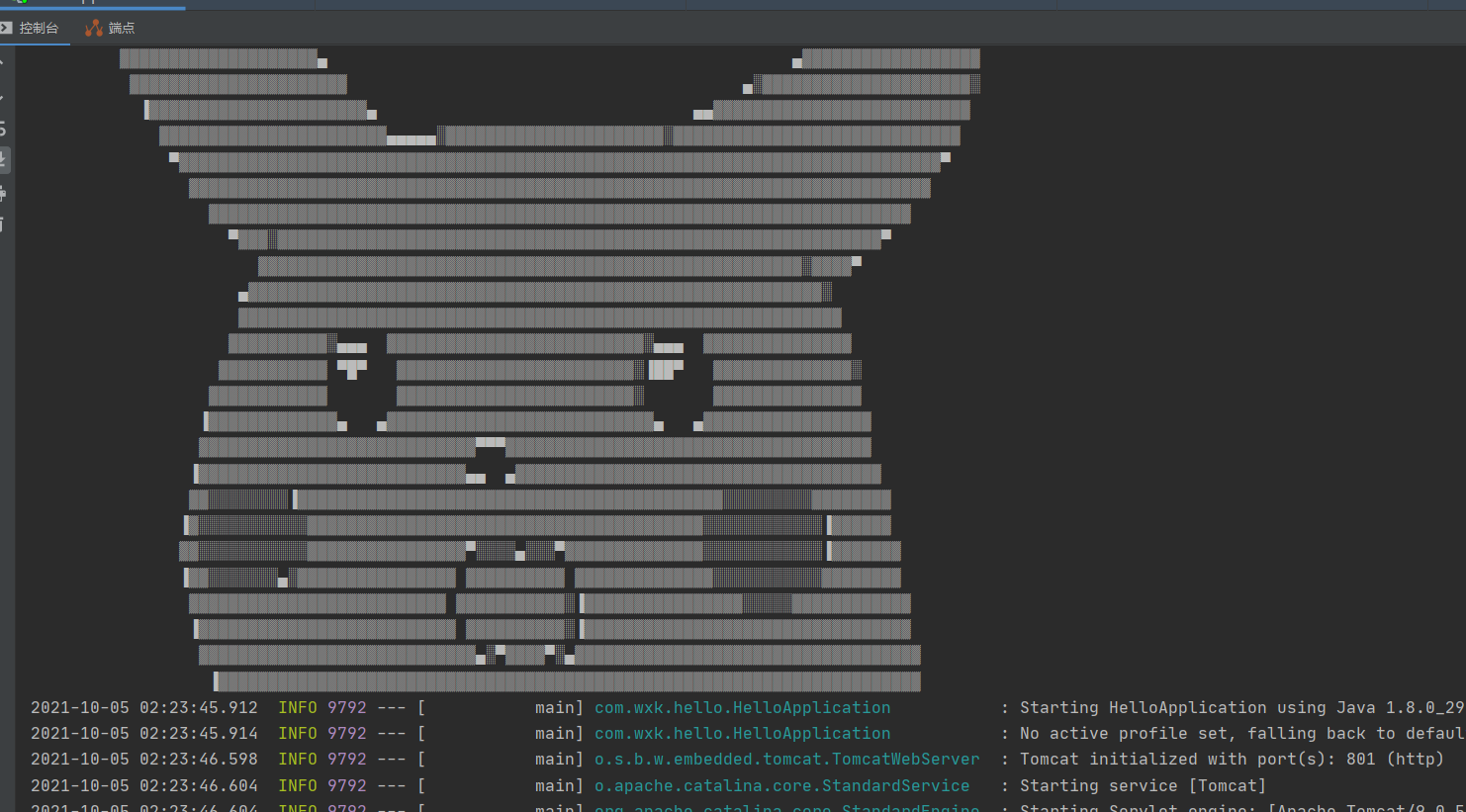
2.springboot自动装配原理
springboot自动装配原理 需要配合原码使用
- springboot在启动的时候,从类路径下/META-INF/spring.factories获取指定的值;
- 将这些自动配置的类导入容器,自动配置就会生效,帮我们进行自动配置;
- 以前我们需要自动配置的东西,现在springboot帮我们做了;
- 整合javaEE,解决方案和自动配置的东西都在spring-boot-autoconfigure这个包下;
- 它会把所有需要导入的组件,以类名的方式返回,这些组件就会被添加到容器;
- 容器中也会存在非常多的xxxAutoConfiguration的文件(@Bean),就是这些类给容器中导入了这个场景需要的所有逐渐;并自动配置;
- 有了自动配置类,免去了我们手动编写配置文件的工作!
3.yml语法以及ConfigurationProperties注入
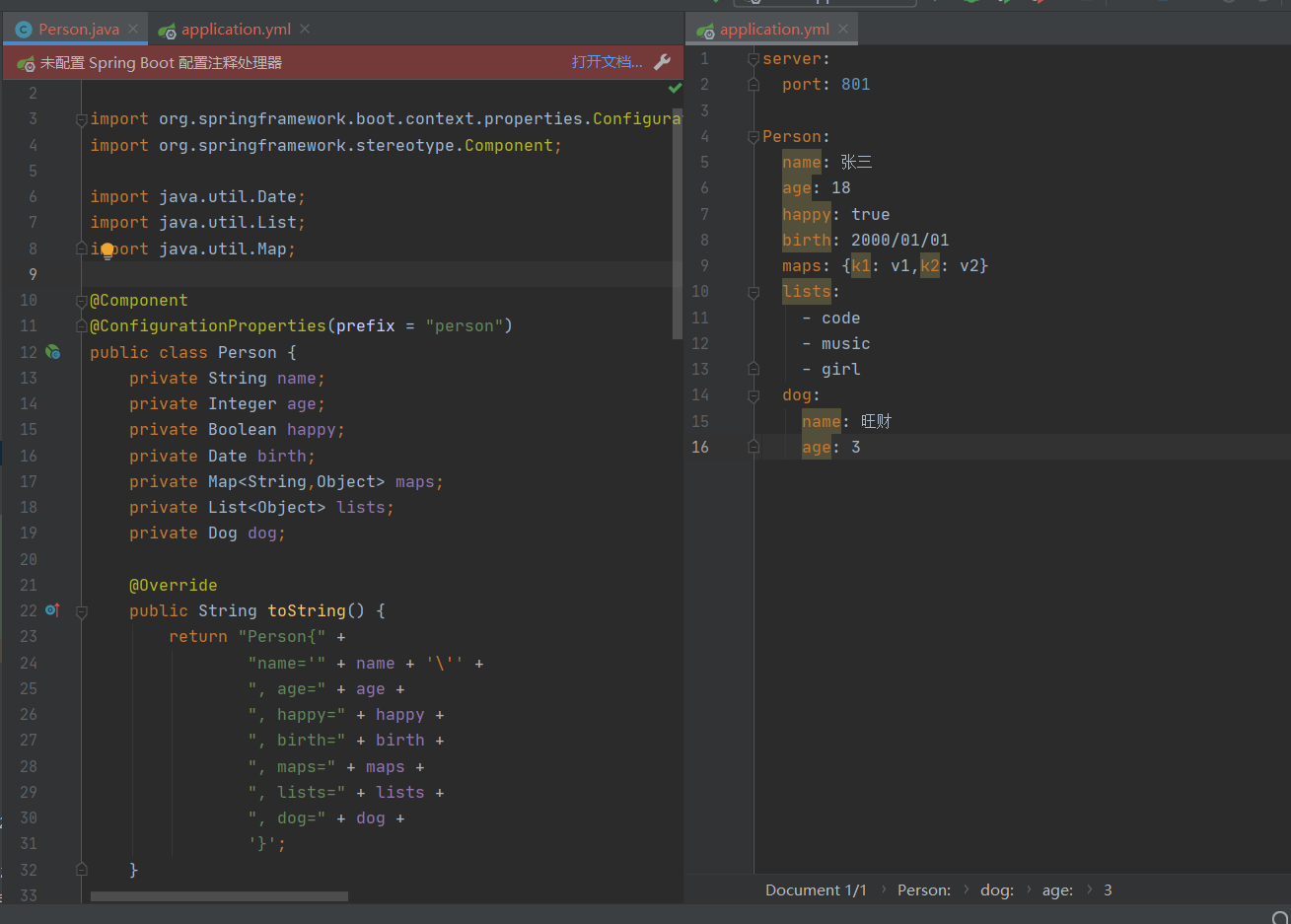 在springboot中,@ConfigurationProperties(prefix = "person") 可以通过prefix和yml中的相同前缀的属性绑定,实现配置注入
那么yml文件或者说properties文件是如何与自动装配的属性挂钩的? 在springboot中,自动装配的类都有一个xxxAutoConfiguration 的类,在该类中,一般会有一个@EnableConfigurationProperties 的注解,这个注解会引入一个Properties 类,该类由@ConfigurationProperties 注解,因此,该类中的属性就是我们在yml文件或是properties文件中关联的值。
如果说想要知道哪些自动配置类生效了哪些没生效,也可以在yml中设置debug=true来进行查看
4.JSR-303校验
在springboot中,可以使用@Validated 注解来进行JSR-303的校验 具体到需要校验的属性可以在属性上使用相应的注解进行校验,注解如下 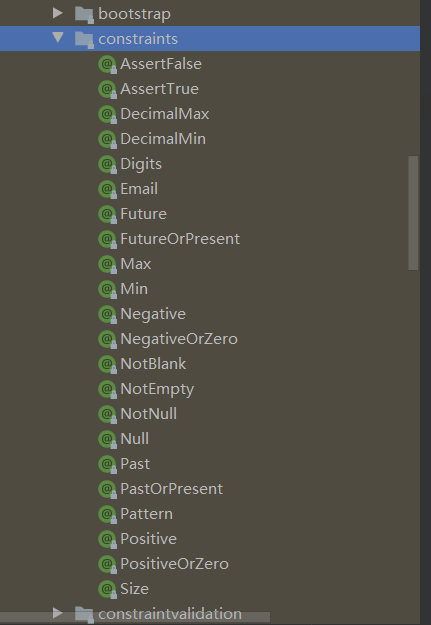
5.yml中进行多环境配置
在实际开发中,可能有很多套环境,例如默认环境、开发环境、测试环境等 在yml中,可以通过--- 的方式在同一个yml文件中拆分出不同环境,如下:
server:
port: 801
spring:
profiles:
active: dev
---
server:
port: 802
spring:
profiles: dev
---
server:
port: 803
spring:
profiles: test
需要切换环境,只需要在默认环境中使用spring.profiles.active 配置即可
6.静态资源处理
- 在springboot中,可以通过以下方式访问静态资源
- webjars 具体映射到页面
localhost:8080/webjars - public,static,/**,resources
localhost:8080/ - 优先级resources>static>public
7.数据库操作
- JDBC的使用
这个只需要在yml文件中进行配置即可
spring:
datasource:
username: xxxx
password: xxxx
url: jdbc:mysql://localhost:3306/xxxx?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&
driver-class-name: com.mysql.cj.jdbc.Driver
- DRUID数据源的使用
要使用德鲁伊的数据源,只需要在jdbc的数据源的基础上增加一个type 配置
spring:
datasource:
username: root
password: q1111111111
url: jdbc:mysql://localhost:3306/novel?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&
driver-class-name: com.mysql.cj.jdbc.Driver
type: com.alibaba.druid.pool.DruidDataSource
当然,在此之前,需要先导入德鲁伊的依赖
<dependency>
<groupId>com.alibaba</groupId>
<artifactId>druid</artifactId>
<version>1.2.8</version>
</dependency>
更多的德鲁伊的相关配置以及德鲁伊后台监控的使用
德鲁伊的部分参数配置
server:
port: 801
spring:
datasource:
username: root
password: q1111111111
url: jdbc:mysql://localhost:3306/novel?serverTimezone=UTC&useUnicode=true&characterEncoding=utf-8&
driver-class-name: com.mysql.cj.jdbc.Driver
type: com.alibaba.druid.pool.DruidDataSource
initialSize: 5
minIdel: 5
maxActive: 20
maxWait: 60000
timeBetweenEvictionRunsMillis: 60000
minEvictableIdleTimeMillis: 300000
validationQuery: SELECT 1 FROM DUAL
testWhileIdle: true
testOnBorrow: false
testOnReturn: false
poolPreparedStatements: true
filters: stat,wall,log4j
maxPoolPreparedStatementPerConnectionSize: 20
useGlobalDataSourceStat: true
connectionProperties: druid.stat.mergeSql=true;druid.stat.slowSqlMillis=500
如果需要使用这些自己配置的参数以及配置德鲁伊的后台,需要自己创建一个配置类
package com.wxk.hello.config;
import com.alibaba.druid.pool.DruidDataSource;
import com.alibaba.druid.support.http.StatViewServlet;
import org.springframework.boot.context.properties.ConfigurationProperties;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.sql.DataSource;
import java.util.HashMap;
@Configuration
public class DruidConfig {
@ConfigurationProperties(prefix = "spring.datasource")
@Bean
public DataSource druidDataSource(){
return new DruidDataSource();
}
@Bean
public ServletRegistrationBean statViewServlet(){
ServletRegistrationBean<StatViewServlet> bean=new ServletRegistrationBean<>(new StatViewServlet(),"/druid/*");
HashMap<String , String> initParameter = new HashMap<>();
initParameter.put("loginUsername","admin");
initParameter.put("loginPassword","123456");
initParameter.put("allow","");
bean.setInitParameters(initParameter);
return bean;
}
}
- mybatis整合及使用
首先在中央仓库找到mybatis的starter依赖,安装好依赖后,在yml文件中进行如下配置mybatis:
mapper-locations: classpath:mybatis/mapper/*.xml
这个配置是你的映射文件的位置,配置好这个后就可以使用了 具体使用:
@Mapper
@Repository
public interface BooksMapper {
List<Book> queryBooks();
}
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.wxk.hello.mapper.BooksMapper">
<select id="queryBooks" resultType="com.wxk.hello.vo.Book">
select * from book
</select>
</mapper>
8.thymeleaf常用约束
xmlns:th=http://www.thymeleaf.org
xmlns:sec=http://www.thymeleaf.org/extras/spring-security
xmlns:shiro=http://www.pollix.at/thymeleaf/shiro
|