SpringStudy01-HelloSpring
这里本人使用的是maven,所以必须导入maven依赖 下面图是该例子包的结构 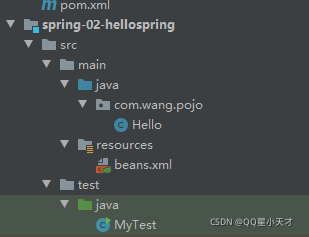
创建一个HelloSpring类
public class Hello {
private String str;
public String getStr() {
return str;
}
public void setStr(String str) {
this.str = str;
}
@Override
public String toString() {
return "Hello{" +
"str='" + str + '\'' +
'}';
}
}
编写beans.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="hello" class="com.wang.pojo.Hello">
<property name="str" value="Spring"/>
</bean>
</beans>
编写测试类
public class MyTest {
public static void main(String[] args){
ApplicationContext context=new ClassPathXmlApplicationContext("beans.xml");
Hello hello=(Hello)context.getBean("hello");
System.out.println(hello.toString());
}
}
=========================================================
SpringStudy02-ioc01(引用ref)
包的结构 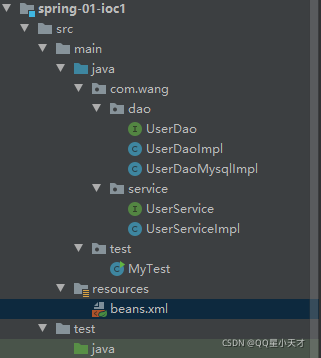 dao层
public interface UserDao {
void getuser();
}
public class UserDaoImpl implements UserDao {
public void getuser() {
System.out.println("该我表演了!");
}
}
public void getuser() {
System.out.println("改你表演了!");
}
}
service层
public interface UserService {
void getuser();
}
public class UserServiceImpl implements UserService{
private UserDao userdao;
public void setUserdao(UserDao userdao) {
this.userdao = userdao;
}
public void getuser() {
userdao.getuser();
}
}
beans.xml文件
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="DaoIml" class="com.wang.dao.UserDaoImpl"/>
<bean id="MysqlIml" class="com.wang.dao.UserDaoMysqlImpl"/>
<bean id="ServiceIml" class="com.wang.service.UserServiceImpl">
<property name="userdao" ref="DaoIml"></property>
</bean>
<bean id="ServiceIml02" class="com.wang.service.UserServiceImpl">
<property name="userdao" ref="MysqlIml"></property>
</bean>
</beans>
测试类
public class MyTest {
public static void main(String[] args){
ApplicationContext context=new ClassPathXmlApplicationContext("beans.xml");
UserServiceImpl userService=(UserServiceImpl)context.getBean("ServiceIml02");
userService.getuser();
UserService userService1=new UserServiceImpl();
((UserServiceImpl)userService1).setUserdao(new UserDaoImpl());
userService1.getuser();
}
}
注意点 UserServiceImpl类中的userdao类型是UserDao接口,否则类型不一致会导致赋值失败
========================================================
SpringStudy03-构造器注入(有参构造)
public class User1 {
private String name1;
private int age;
private String sex;
public User1() {
}
public User1(String name1, int age, String sex) {
this.name1 = name1;
this.age = age;
this.sex = sex;
}
public String getName1() {
return name1;
}
public void setName1(String name1) {
this.name1 = name1;
}
public int getAge() {
return age;
}
public void setAge(int age) {
this.age = age;
}
public String getSex() {
return sex;
}
public void setSex(String sex) {
this.sex = sex;
}
@Override
public String toString() {
return "User1{" +
"name1='" + name1 + '\'' +
", age=" + age +
", sex='" + sex + '\'' +
'}';
}
}
- 在beans.xml注册bean
1.通过属性类型赋值
<bean id="user1" class="com.wang.pojo.User1">
<constructor-arg type="java.lang.String" value="wdawd"></constructor-arg>
<constructor-arg type="int" value="34"></constructor-arg>
<constructor-arg type="java.lang.String" value="男"></constructor-arg>
</bean>
- 通过下标进行赋值(从0开始)
<bean id="user1" class="com.wang.pojo.User1">
<constructor-arg index="0" value="wdwda"></constructor-arg>
<constructor-arg index="1" value="34"></constructor-arg>
<constructor-arg index="2" value="nan"></constructor-arg>
</bean>
- 通过参数名赋值
<bean id="user1" class="com.wang.pojo.User1">
<constructor-arg name="name1" value="adawd"></constructor-arg>
<constructor-arg name="age" value="34"></constructor-arg>
<constructor-arg name="sex" value="nan"></constructor-arg>
</bean>
=========================================================
SpringStudy04-di注入
首先创建一个Student类,该类中包含各种属性,让我们看看各种属性注入的方法
public class Student {
private String name;
private Address address;
private String[] books;
private List<String> hobbys;
private Map<String,String> card;
private Set<String> games;
private String wife;
private Properties info;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Address getAddress() {
return address;
}
public void setAddress(Address address) {
this.address = address;
}
public String[] getBooks() {
return books;
}
public void setBooks(String[] books) {
this.books = books;
}
public List<String> getHobbys() {
return hobbys;
}
public void setHobbys(List<String> hobbys) {
this.hobbys = hobbys;
}
public Map<String, String> getCard() {
return card;
}
public void setCard(Map<String, String> card) {
this.card = card;
}
public Set<String> getGames() {
return games;
}
public void setGames(Set<String> games) {
this.games = games;
}
public String getWife() {
return wife;
}
public void setWife(String wife) {
this.wife = wife;
}
public Properties getInfo() {
return info;
}
public void setInfo(Properties info) {
this.info = info;
}
@Override
public String toString() {
return "Student{" +
"name='" + name + '\'' +
", address=" + address.toString() +
", books=" + Arrays.toString(books) +
", hobbys=" + hobbys +
", card=" + card +
", games=" + games +
", wife='" + wife + '\'' +
", info=" + info +
'}';
}
}
其中的Address类
public class Address {
private String address;
public String getAddress() {
return address;
}
public void setAddress(String address) {
this.address = address;
}
@Override
public String toString() {
return "Address{" +
"address='" + address + '\'' +
'}';
}
}
下面进行bean注册
<bean id="address" class="com.wang.pojo.Address">
</bean>
<bean id="student" class="com.wang.pojo.Student">
<property name="name" value="we"></property>
<property name="address" ref="address"></property>
<property name="books">
<array>
<value>红楼梦</value>
<value>西游记</value>
<value>三国演义</value>
<value>水浒传</value>
</array>
</property>
<property name="hobbys">
<list>
<value>打游戏</value>
<value>学习</value>
<value>看书</value>
</list>
</property>
<property name="card">
<map>
<entry key="身份证" value="4141414141414141414"></entry>
<entry key="学号" value="20210903"></entry>
</map>
</property>
<property name="games">
<set>
<value>王者荣耀</value>
<value>和平精英</value>
</set>
</property>
<property name="wife">
<null></null>
</property>
<property name="info">
<props>
<prop key="学号">20210903</prop>
<prop key="性别">男</prop>
<prop key="姓名">we</prop>
</props>
</property>
</bean>
注意map注入!!!!!!!!
=========================================================
SpringStudy05-p命名注入和c命名注入
首先编写User类
public class User {
private String name;
private String age;
public User() {
}
public User(String name, String age) {
this.name = name;
this.age = age;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public String getAge() {
return age;
}
public void setAge(String age) {
this.age = age;
}
@Override
public String toString() {
return "User{" +
"name='" + name + '\'' +
", age='" + age + '\'' +
'}';
}
}
进行p和c命名注入需要在xml文件中beans标签加入 xmlns:p=“http://www.springframework.org/schema/p” xmlns:c="http://www.springframework.org/schema/c" 如下所示
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:p="http://www.springframework.org/schema/p"
xmlns:c="http://www.springframework.org/schema/c"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="user" class="com.wang.pojo.User" p:age="20" p:name="we">
</bean>
<bean id="user2" class="com.wang.pojo.User" c:name="20" c:age="we1"></bean>
</beans>
=========================================================
SpringStudy06-自动装配(Autowired)
创建Cat4,Dog4,People这三个类
public class Cat4 {
public void shout(){
System.out.println("喵~");
}
}
public class Dog4 {
public void shout(){
System.out.println("汪~");
}
}
public class People4 {
private Dog4 dog4;
private Cat4 cat4;
private String name;
public Dog4 getDog4() {
return dog4;
}
public void setDog4(Dog4 dog4) {
this.dog4 = dog4;
}
public Cat4 getCat4() {
return cat4;
}
public void setCat4(Cat4 cat4) {
this.cat4 = cat4;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "People4{" +
"dog4=" + dog4 +
", cat4=" + cat4 +
", name='" + name + '\'' +
'}';
}
}
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<bean id="cat4" class="com.wang.pojo4.Cat4"></bean>
<bean id="dog4" class="com.wang.pojo4.Dog4"></bean>
<bean id="people4" class="com.wang.pojo.People4" autowire="byName">
<property name="name" value="we"></property>
</bean>
<bean id="people4" class="com.wang.pojo.People4" autowire="byType">
<property name="name" value="we"></property>
</bean>
</beans>
=========================================================
SpringStudy07-使用注解进行自动装配(@Autowired @Resource)
@Autowire通过byType方式实现
@Resource默认通过byname方式实现,如果找不到则通过byType,都找不到就报错
1. @Autowire
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:annotation-config></context:annotation-config>
<bean id="dog2" class="com.wang.pojo2.Dog2"></bean>
<bean id="=cat2" class="com.wang.pojo2.Cat2"></bean>
<bean id="dog22" class="com.wang.pojo2.Dog2"></bean>
<bean id="cat22" class="com.wang.pojo2.Cat2"></bean>
<bean id="people2" class="com.wang.pojo2.People2">
<property name="name2" value="we2"></property>
</bean>
</beans>
public class Cat2 {
public void shout(){
System.out.println("喵~");
}
}
public class Dog2 {
public void shout(){
System.out.println("汪~");
}
}
public class People2 {
@Autowired
@Qualifier(value = "dog22")
private Dog2 dog2;
@Autowired
@Qualifier(value = "cat22")
private Cat2 cat2;
private String name2;
public Dog2 getDog2() {
return dog2;
}
public Cat2 getCat2() {
return cat2;
}
public String getName2() {
return name2;
}
public void setName2(String name2) {
this.name2 = name2;
}
@Override
public String toString() {
return "People2{" +
"dog2=" + dog2 +
", cat2=" + cat2 +
", name2='" + name2 + '\'' +
'}';
}
}
2.@Resource
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd">
<context:annotation-config></context:annotation-config>
<bean id="dog3" class="com.wang.pojo3.Dog3"></bean>
<bean id="cat3" class="com.wang.pojo3.Cat3"></bean>
<bean id="people3" class="com.wang.pojo3.People3"></bean>
</beans>
重点是People3类
public class People3 {
@Resource
private Dog3 dog3;
@Resource
private Cat3 cat3;
private String name3;
public Dog3 getDog3() {
return dog3;
}
public void setDog3(Dog3 dog3) {
this.dog3 = dog3;
}
public Cat3 getCat3() {
return cat3;
}
public void setCat3(Cat3 cat3) {
this.cat3 = cat3;
}
public String getName3() {
return name3;
}
public void setName3(String name3) {
this.name3 = name3;
}
@Override
public String toString() {
return "People3{" +
"dog3=" + dog3 +
", cat3=" + cat3 +
", name3='" + name3 + '\'' +
'}';
}
}
注意:@Resource(name="")进行特定指定bean对象
=========================================================
|