最邻近内插(nearest neighbor interpolation) 是计算最简单的内插方法。在该算法中,每一个输出值都是该点最近的原始数据样本值,这种内插方法又称点位移算法。最邻近内插通过像素的复制可以实现影像的放大,通过抽取可实现影像缩小。但最邻近内插容易产生影像的块状效应。此外,该方法的偏移误差最大可达到0.5个像素。 下面用数字矩阵显示最邻近内插方法。 借助工具:Excel、Java编程
读取Excel表中数字到二维数组
package Digital.Photogrammetrry;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ExcelToArray {
public double array1[][];
public ExcelToArray(String filePath) {
try {
FileInputStream fip = new FileInputStream(filePath);
XSSFWorkbook wb = new XSSFWorkbook(fip);
XSSFSheet sheet = wb.getSheetAt(0);
{
int rows = sheet.getPhysicalNumberOfRows();
int columns = sheet.getRow(0).getPhysicalNumberOfCells();
array1 = new double[rows][columns];
for (int i = 0; i < rows; i++) {
XSSFRow row = sheet.getRow(i);
for (int j = 0; j < columns; j++) {
array1[i][j] = row.getCell(j).getNumericCellValue();
}
}
}
wb.close();
fip.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
将二维数组写入到表格
package Digital.Photogrammetrry;
import java.io.FileNotFoundException;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.xssf.usermodel.XSSFRow;
import org.apache.poi.xssf.usermodel.XSSFSheet;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
public class ArrayToExcel {
public ArrayToExcel(double array[][], String filePath) {
try {
FileOutputStream fop = new FileOutputStream(filePath);
XSSFWorkbook wb = new XSSFWorkbook();
XSSFSheet sheet = wb.createSheet("Result");
{
for (int i = 0; i < array.length; i++) {
XSSFRow row = sheet.createRow(i);
for (int j = 0; j < array[i].length; j++) {
row.createCell(j).setCellValue(array[i][j]);
}
}
}
wb.write(fop);
wb.close();
fop.close();
} catch (FileNotFoundException e) {
e.printStackTrace();
} catch (IOException e) {
e.printStackTrace();
}
}
}
最邻近内插算子(二维数组)
package Digital.Photogrammetrry;
public class Nearest_Neighbor_Interpolation {
private double array[][];
private double[][] getArray() {
return array;
}
private void setArray(double[][] array, int w, int h) {
float rows = array.length;
float columns = array[0].length;
this.array = new double[w][h];
for (int i = 0; i < w; i++) {
int a = (int) ((float) (i + 1) / ((float) w / rows) + 0.5f) - 1;
for (int j = 0; j < h; j++) {
int b = (int) ((float) (j + 1) / ((float) h / columns) + 0.5f) - 1;
this.array[i][j] = array[a][b];
}
}
}
public static void main(String[] args) {
ExcelToArray eta = new ExcelToArray("src/Digital/Photogrammetrry/table.xlsx");
Nearest_Neighbor_Interpolation neighbor_Interpolation = new Nearest_Neighbor_Interpolation();
neighbor_Interpolation.setArray(eta.array1, 4, 6);
new ArrayToExcel(neighbor_Interpolation.getArray(), "D:\\ceshi.xlsx");
}
}
结果
处理前: 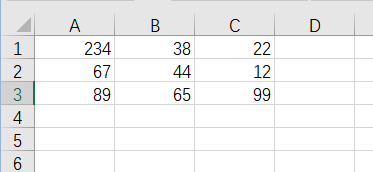 处理后(3×3)-----> (4×6): 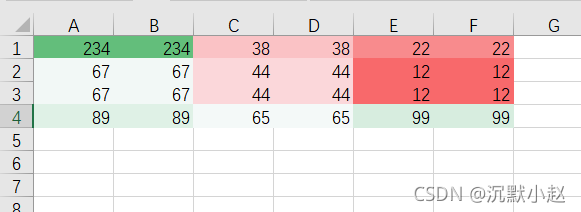 (3×3)-----> (2×2): 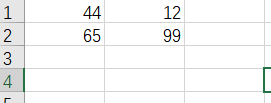
(3×3)-----> (6×6): 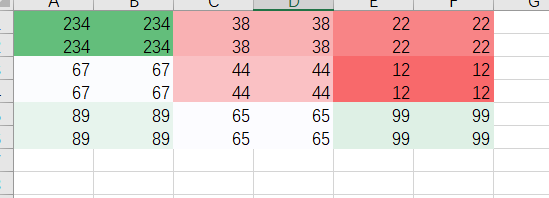
|