一:实体类
package domian;
public class Student {
private Integer id;
private String name;
private Integer age;
public Student() {
}
public Student(Integer id, String name, Integer age) {
this.id = id;
this.name = name;
this.age = age;
}
public Integer getId() {
return id;
}
public void setId(Integer id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getAge() {
return age;
}
public void setAge(Integer age) {
this.age = age;
}
@Override
public String toString() {
return "Student{" +
"id=" + id +
", name='" + name + '\'' +
", age=" + age +
'}';
}
}
二:mapper层
package mapper;
import domian.Student;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface StudentMapper {
List<Student> selectAll();
List<Student> selectnameorage(@Param("p1") String name ,@Param("p2") Integer age);
List<Student> selectnameandage(Student stu);
List<Student> selectageandname(List<Integer> ids);
}
三:sevrver层
package service;
import domian.Student;
import java.util.List;
public interface StudentService {
List<Student> SelectAll();
List<Student> selectnameorage(String name,Integer age);
List<Student> selectnameandage(Student stu);
List<Student> selectageandname(List<Integer> ids);
}
实现类
package service;
import domian.Student;
import mapper.StudentMapper;
import org.apache.ibatis.io.Resources;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.apache.ibatis.session.SqlSessionFactoryBuilder;
import java.io.IOException;
import java.io.InputStream;
import java.util.List;
public class StudentServices implements StudentService {
@Override
public List<Student> SelectAll() {
InputStream is = null;
SqlSession sqlSession = null;
List<Student> list = null;
try {
is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(is);
sqlSession = build.openSession(true);
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
list = mapper.selectAll();
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (sqlSession!=null){
sqlSession.close();
}
}
return list;
}
@Override
public List<Student> selectnameorage(String name, Integer age) {
InputStream is = null;
SqlSession sqlSession = null;
List<Student> list = null;
try {
is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(is);
sqlSession = build.openSession(true);
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
list = mapper.selectnameorage(name, age);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (sqlSession!=null){
sqlSession.close();
}
}
return list;
}
@Override
public List<Student> selectnameandage(Student stu) {
InputStream is = null;
SqlSession sqlSession = null;
List<Student> list = null;
try {
is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(is);
sqlSession = build.openSession(true);
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
list = mapper.selectnameandage(stu);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (sqlSession!=null){
sqlSession.close();
}
}
return list;
}
@Override
public List<Student> selectageandname(List<Integer> ids) {
InputStream is = null;
SqlSession sqlSession = null;
List<Student> list = null;
try {
is = Resources.getResourceAsStream("MyBatisConfig.xml");
SqlSessionFactory build = new SqlSessionFactoryBuilder().build(is);
sqlSession = build.openSession(true);
StudentMapper mapper = sqlSession.getMapper(StudentMapper.class);
list = mapper.selectageandname(ids);
} catch (IOException e) {
e.printStackTrace();
} finally {
if (is!=null){
try {
is.close();
} catch (IOException e) {
e.printStackTrace();
}
}
if (sqlSession!=null){
sqlSession.close();
}
}
return list;
}
}
配置文件:
全局配置
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE configuration PUBLIC "-//mybatis.org//DTD Config 3.0//EN" "http://mybatis.org/dtd/mybatis-3-config.dtd">
<configuration>
//加载数据源的配置
<properties resource="jdbc.properties"/>
//起别名
<typeAliases>
<typeAlias type="domian.Student" alias="student"/>
<!--<package name="com.itheima.bean"/>-->
</typeAliases>
<environments default="mysql">
<environment id="mysql">
<transactionManager type="JDBC"></transactionManager>
<dataSource type="POOLED">
<property name="driver" value="${driver}"/>
<property name="url" value="${url}"/>
<property name="username" value="${username}"/>
<property name="password" value="${password}"/>
</dataSource>
</environment>
</environments>
//加载sql语句的配置文件
<mappers>
<mapper resource="StudentMapper.xml"/>
</mappers>
</configuration>
数据源? jdbc.properties文件
driver=com.mysql.jdbc.Driver
url=jdbc:mysql://127.0.0.1:3306/da1
username=root
password=root
编写sql文件
<?xml version="1.0" encoding="UTF-8" ?>
<!--MyBatis的DTD约束-->
<!DOCTYPE mapper
PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN"
"http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="mapper.StudentMapper">
<select id="selectAll" resultType="student">
select * from student
</select>
<select id="selectnameorage" resultType="student">
select * from student where name = #{p1} or age =#{p2}
</select>
<!--多条件查询-->
<select id="selectnameandage" resultType="student" parameterType="student">
select * from student
<where>
<if test="age!=null">
age=#{age}
</if>
<if test="name!=null">
and name=#{name}
</if>
</where>
</select>
<select id="selectageandname" resultType="student" parameterType="list">
select * from student
<where>
<foreach collection="list" open="id in (" close=")" separator="," item="id">
id=#{id}
</foreach>
</where>
</select>
</mapper>
引入jar包:
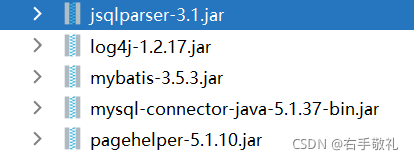
?
|