1.编写测试类
- 本次测试数组, List, Map, Set的注入
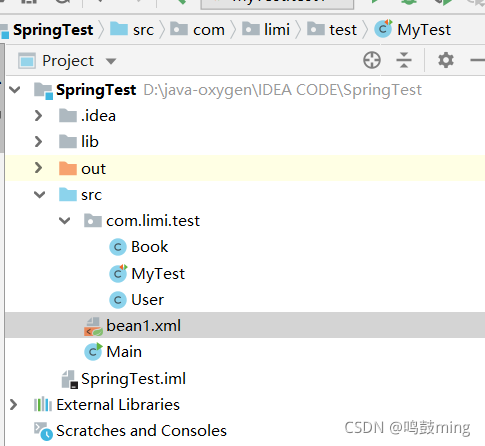 Book类
package com.limi.test;
public class Book {
private String name;
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
@Override
public String toString() {
return "Book{" +
"name='" + name + '\'' +
'}';
}
}
User类
package com.limi.test;
import java.util.List;
import java.util.Map;
import java.util.Set;
public class User {
private Integer[] numArray;
private List<String> hobyList;
private Map<String, String> friendMap;
private Set<Integer> numSet;
private List<Book> bookList;
public Integer[] getNumArray() {
return numArray;
}
public void setNumArray(Integer[] numArray) {
this.numArray = numArray;
}
public List<String> getHobyList() {
return hobyList;
}
public void setHobyList(List<String> hobyList) {
this.hobyList = hobyList;
}
public Map<String, String> getFriendMap() {
return friendMap;
}
public void setFriendMap(Map<String, String> friendMap) {
this.friendMap = friendMap;
}
public Set<Integer> getNumSet() {
return numSet;
}
public void setNumSet(Set<Integer> numSet) {
this.numSet = numSet;
}
public void setBookList(List<Book> bookList) {
this.bookList = bookList;
}
public User() {
}
public void say(){
System.out.println("\n array:");
for (Integer n: numArray) {
System.out.print(n+" ");
}
System.out.println("\n hobyList:"+hobyList.toString());
System.out.println("\n friendMap:"+friendMap.toString());
System.out.println("\n numSet:"+numSet.toString());
System.out.println(("\n bookList"+bookList.toString()));
}
}
测试类
package com.limi.test;
import org.junit.Test;
import org.springframework.context.ApplicationContext;
import org.springframework.context.support.ClassPathXmlApplicationContext;
public class MyTest {
@Test
public void test1(){
ApplicationContext context = new ClassPathXmlApplicationContext("bean1.xml");
User user = context.getBean("User", User.class);
user.say();
}
}
2.编写xml文件为bean注入集合属性
bean1.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd">
<bean id="User" class="com.limi.test.User">
<property name="numArray">
<array>
<value>111</value>
<value>222</value>
<value>333</value>
</array>
</property>
<property name="hobyList">
<list>
<value>篮球</value>
<value>足球</value>
</list>
</property>
<property name="friendMap">
<map>
<entry key="name" value="andy"></entry>
<entry key="tel" value="123456"></entry>
</map>
</property>
<property name="numSet">
<set>
<value>1</value>
<value>2</value>
<value>3</value>
</set>
</property>
<property name="bookList">
<list>
<bean id="book1" class="com.limi.test.Book">
<property name="name" value="English"></property>
</bean>
<ref bean="book2"></ref>
</list>
</property>
</bean>
<bean id="book2" class="com.limi.test.Book">
<property name="name" value="Math"></property>
</bean>
</beans>
测试结果 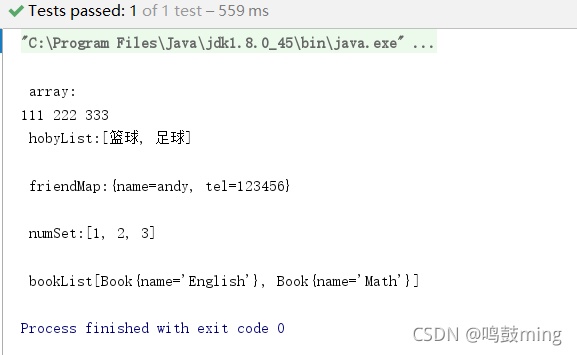
3.使用util命名空间注入List集合的注入
和此前说的p命名空间类似, 这里也是需要改一下xml. 灰色部分就是新添加的  使用方法 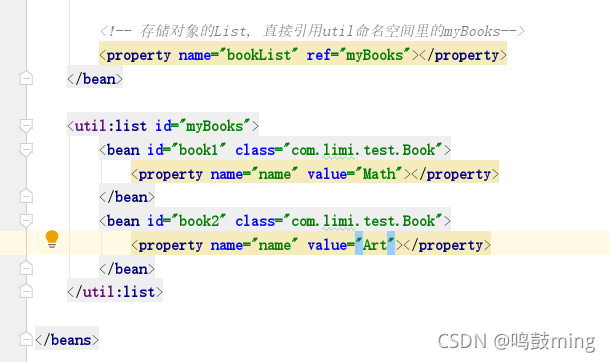
改动后的bean1.xml
<?xml version="1.0" encoding="UTF-8"?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:util="http://www.springframework.org/schema/util"
xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/util http://www.springframework.org/schema/util/spring-util.xsd">
<bean id="User" class="com.limi.test.User">
<property name="numArray">
<array>
<value>111</value>
<value>222</value>
<value>333</value>
</array>
</property>
<property name="hobyList">
<list>
<value>篮球</value>
<value>足球</value>
</list>
</property>
<property name="friendMap">
<map>
<entry key="name" value="andy"></entry>
<entry key="tel" value="123456"></entry>
</map>
</property>
<property name="numSet">
<set>
<value>1</value>
<value>2</value>
<value>3</value>
</set>
</property>
<property name="bookList" ref="myBooks"></property>
</bean>
<util:list id="myBooks">
<bean id="book1" class="com.limi.test.Book">
<property name="name" value="Math"></property>
</bean>
<bean id="book2" class="com.limi.test.Book">
<property name="name" value="Art"></property>
</bean>
</util:list>
</beans>
测试结果 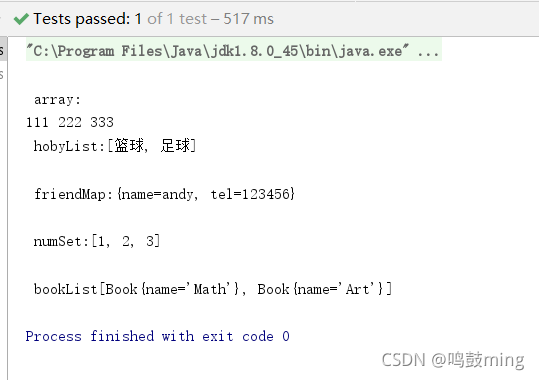
|