面向对象
定义
Java是一门纯面相对象的语言,在面相对象的世界里,一切皆为对象。面相对象是解决问题的一种思想,主要依靠对象之间的交互完成一件事情。
面向对象的优点
由于继承、封装、多态的特性,自然设计出高内聚、低耦合的系统结构,使得系统更灵活、更容易扩展,而且成本较低。
类
定义
类主要是用来对一个实体(对象)来进行描述的,主要描述该实体(对象)具有哪些属性(外观尺寸等),哪些工功能。 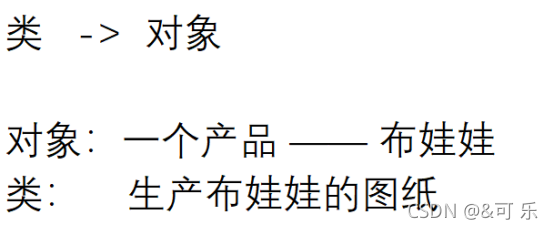
格式
在java中定义类时需要用到class关键字 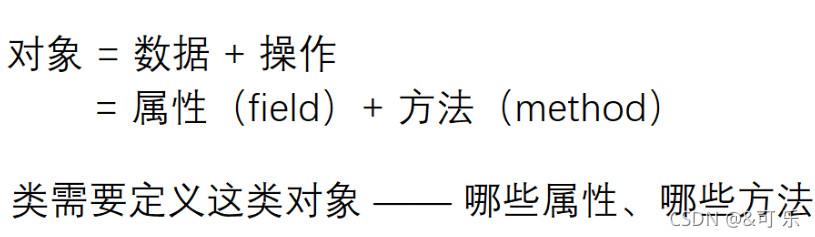 类中包含的内容称为类的成员。
class ClassName{
field;
method;
}
成员属性在类的内部,方法的外部 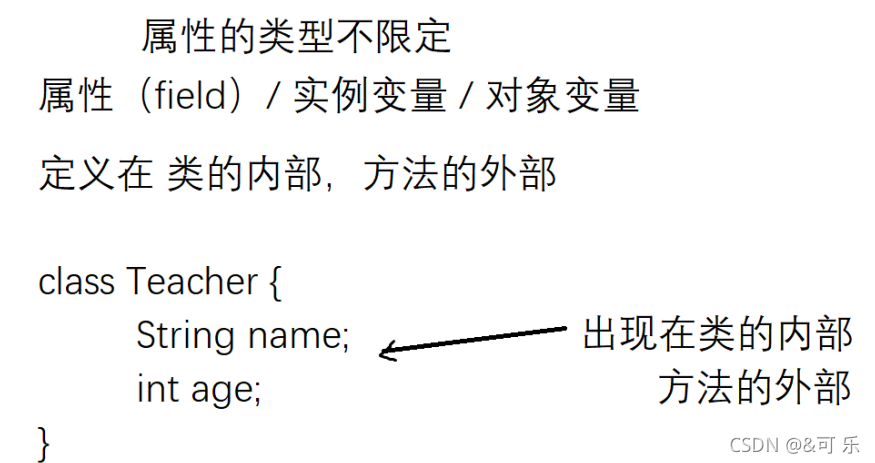 类中包含的内容称为类的成员。 变量信息主要是对类进行描述的,称之为类的成员属性或者类成员变量。 方法主要说明类具有哪些功能,称为类的成员方法。 举例:
class Person {
public int age;
public String name;
public String sex;
public void eat() {
System.out.println("吃饭!");
}
public void sleep() {
System.out.println("睡觉!");
}
}
类名注意采用大驼峰定义:在小驼峰的基础上把第一个单词的首字母也大写了。 小驼峰法:当一个变量名由多个单词构成的时候, 除了第一个单词之外, 其他单词首字母都大写。
类的实例化
用类类型创建对象的过程,称为类的实例化,在java中才用new关键字,配合类名来实例化对象; 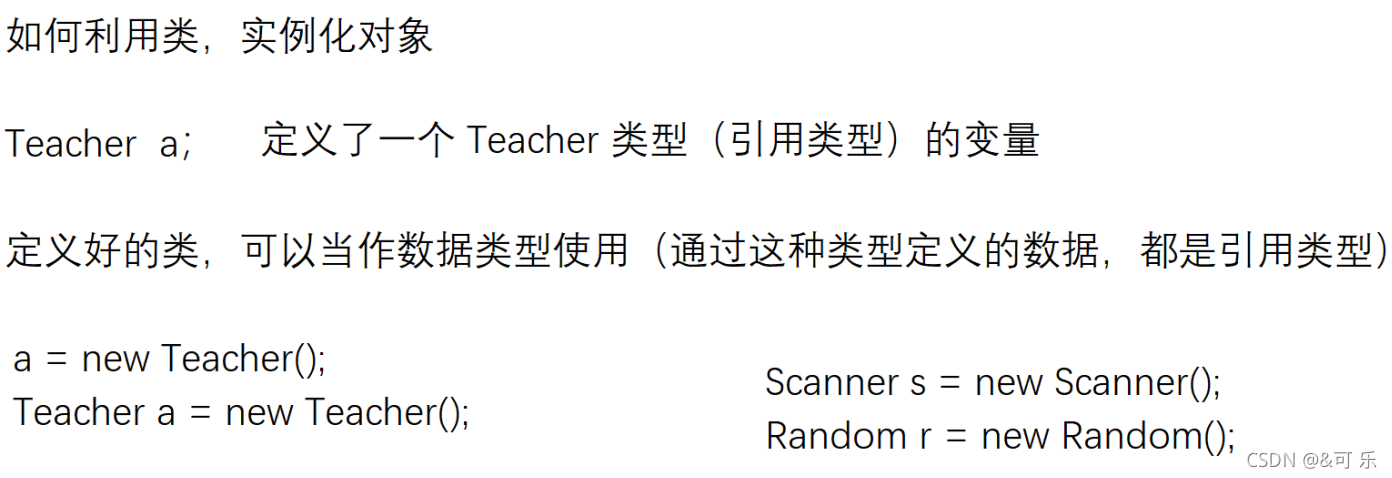 举例:
class Person {
public int age;
public String name;
public String sex;
public void eat() {
System.out.println("吃饭!");
}
public void sleep() {
System.out.println("睡觉!");
}
}
public class Main{
public static void main(String[] args) {
Person person = new Person();
person.eat();
person.sleep();
Person person2 = new Person();
Person person3 = new Person();
}
}
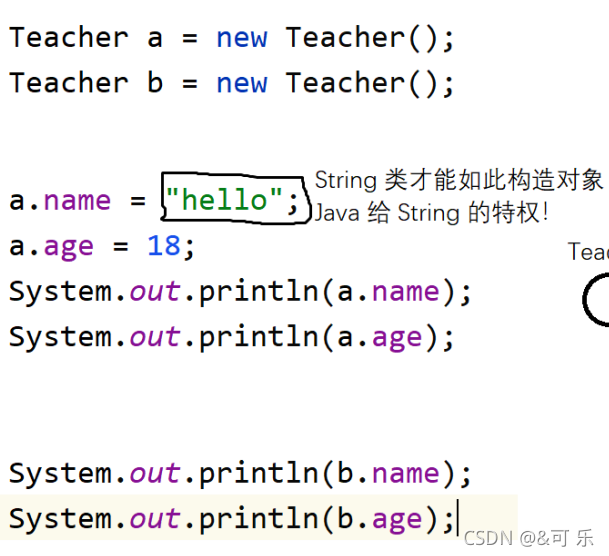
注意:new 关键字用于创建一个对象的实例. 使用 . 来访问对象中的属性和方法. 同一个类可以创建多个实例.
类和对象的说明
类是用来组织方法的组织单元 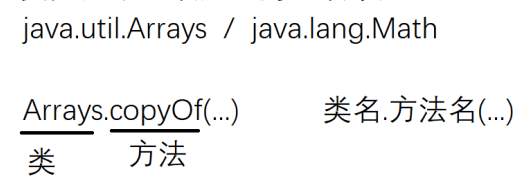 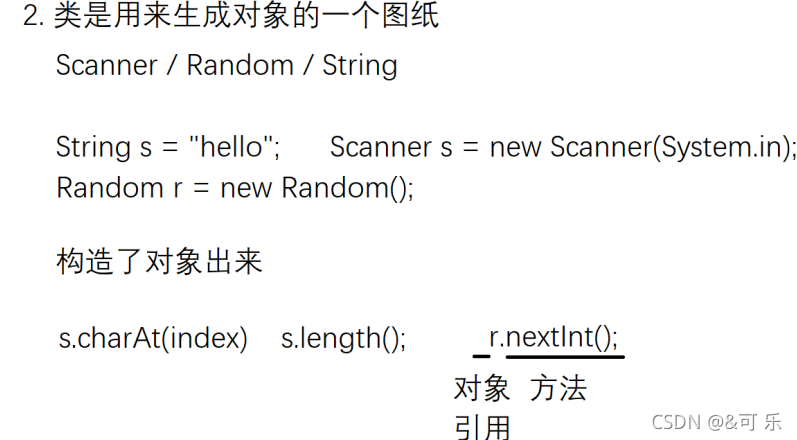
类是一种自定义的类型,可以用来定义变量,但是在java中用类定义出来的变量我们成为对象。 一个类可以实例化出多个对象,实例化出的对象 占用实际的物理空间,存储类成员变量。 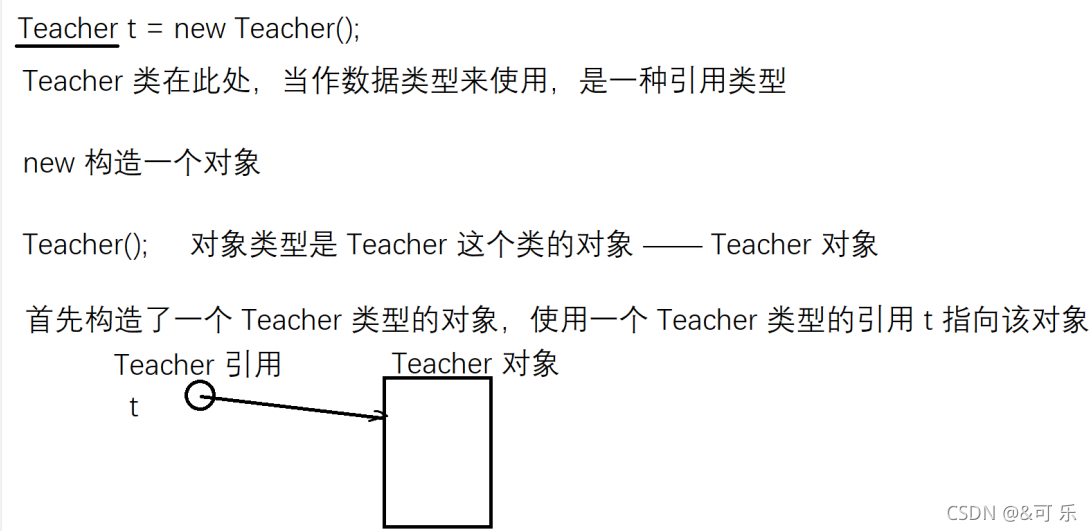
类的成员
类的成员可以包含以下:字段、方法、代码块、内部类和接口等。
字段/属性/成员变量
在类中, 但是方法外部定义的变量. 这样的变量我们称为 “字段” 或 “属性” 或 “成员变量” 举例:
class Person {
public String name;
public int age;
}
class Test {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name);
System.out.println(person.age);
}
}
null
0
认识null
null 在 Java 中为 “空引用”, 表示不引用任何对象. 类似于 C 语言中的空指针. 如果对 null 进行 . 操作就 会引发异常。 空指针异常了
class Person {
public String name;
public int age; }
class Test {
public static void main(String[] args) {
Person person = new Person();
System.out.println(person.name.length());
}
}
Exception in thread "main" java.lang.NullPointerException
at Test.main(Test.java:9)
类中方法的使用
举例:
class Person {
public int age = 18;
public String name = "张三";
public void show() {
System.out.println("我叫" + name + ", 今年" + age + "岁");
}
}
class Test {
public static void main(String[] args) {
Person person = new Person();
person.show();
}
}
我叫张三, 今年18岁
构造方法支持重载. 规则和普通方法的重载一致 这样的 show 方法是和 person 实例相关联的. 如果创建了其他实例, 那么 show 的行为就会发生变化
Person person2 = new Person();
person2.name = "李四";
person2.age = 20;
person2.show()
我叫李四, 今年20岁
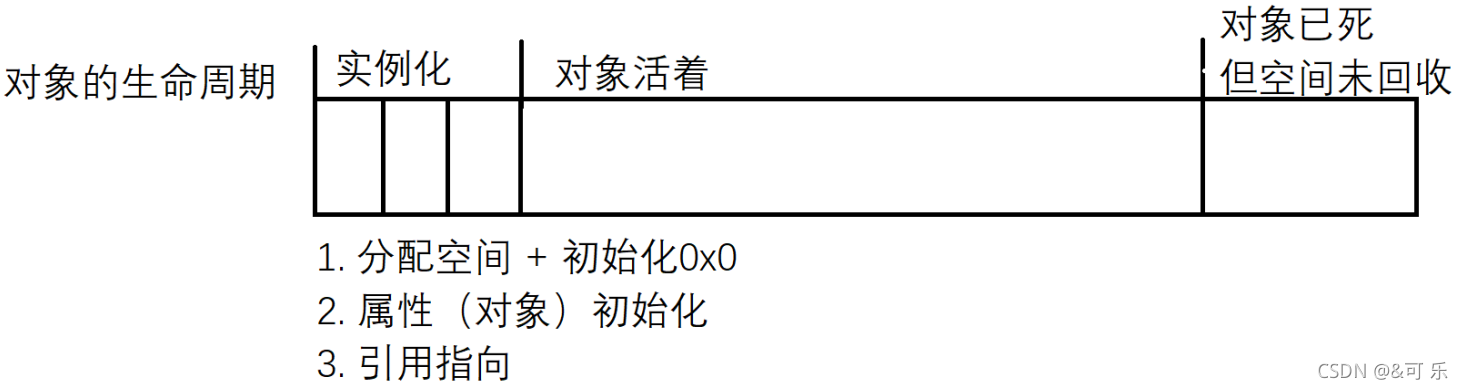
static 关键字
修饰属性,Java静态属性和类相关, 和具体的实例无关. 换句话说, 同一个类的不同实例共用同一个静态属性. 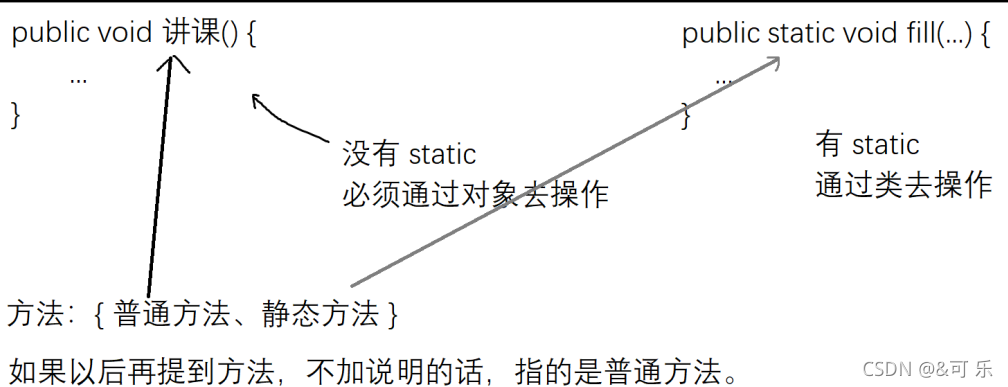 举例: count被static所修饰,所有类共享。且不属于对象,访问方式为:类名 . 属性。
class TestDemo{
public int a;
public static int count;
}
public class Main{
public static void main(String[] args) {
TestDemo t1 = new TestDemo();
t1.a++;
TestDemo.count++;
System.out.println(t1.a);
System.out.println(TestDemo.count);
System.out.println("============");
TestDemo t2 = new TestDemo();
t2.a++;
TestDemo.count++;
System.out.println(t2.a);
System.out.println(TestDemo.count);
}
}
如果在任何方法上应用 static 关键字,此方法称为静态方法 1、静态方法属于类,而不属于类的对象。 2、可以直接调用静态方法,而无需创建类的实例。 3、静态方法可以访问静态数据成员,并可以更改静态数据成员的值。 举例:
class TestDemo{
public int a;
public static int count;
public static void change() {
count = 100;
}
}
public class Main{
public static void main(String[] args) {
TestDemo.change();
System.out.println(TestDemo.count);
}
}
注意: 静态方法和实例无关, 而是和类相关. 因此这导致了两个情况: 1、静态方法不能直接使用非静态数据成员或调用非静态方法(非静态数据成员和方法都是和实例相关 的). 2、this和super两个关键字不能在静态上下文中使用(this 是当前实例的引用, super是当前实例父类实 例的引用, 也是和当前实例相关). 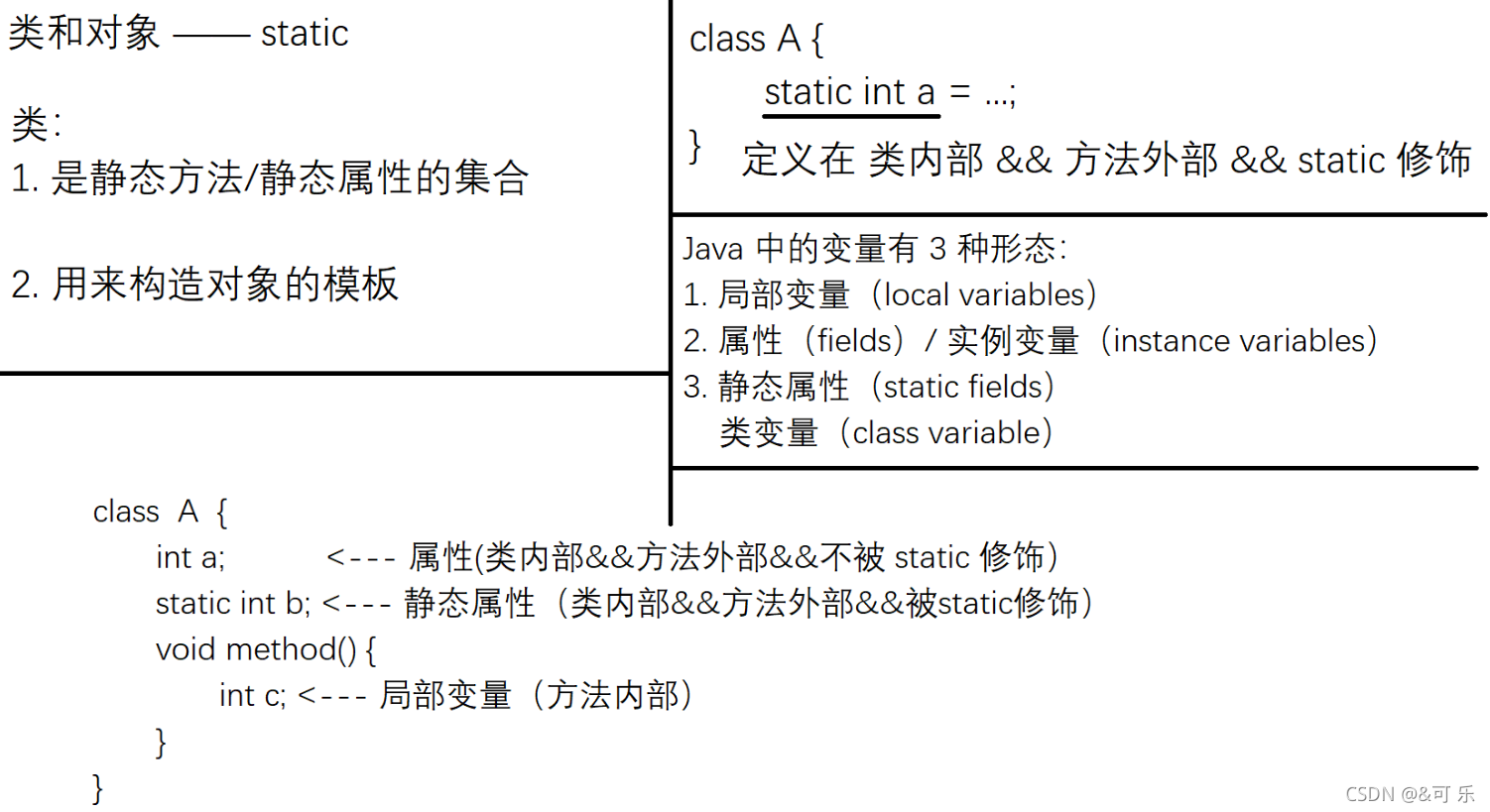
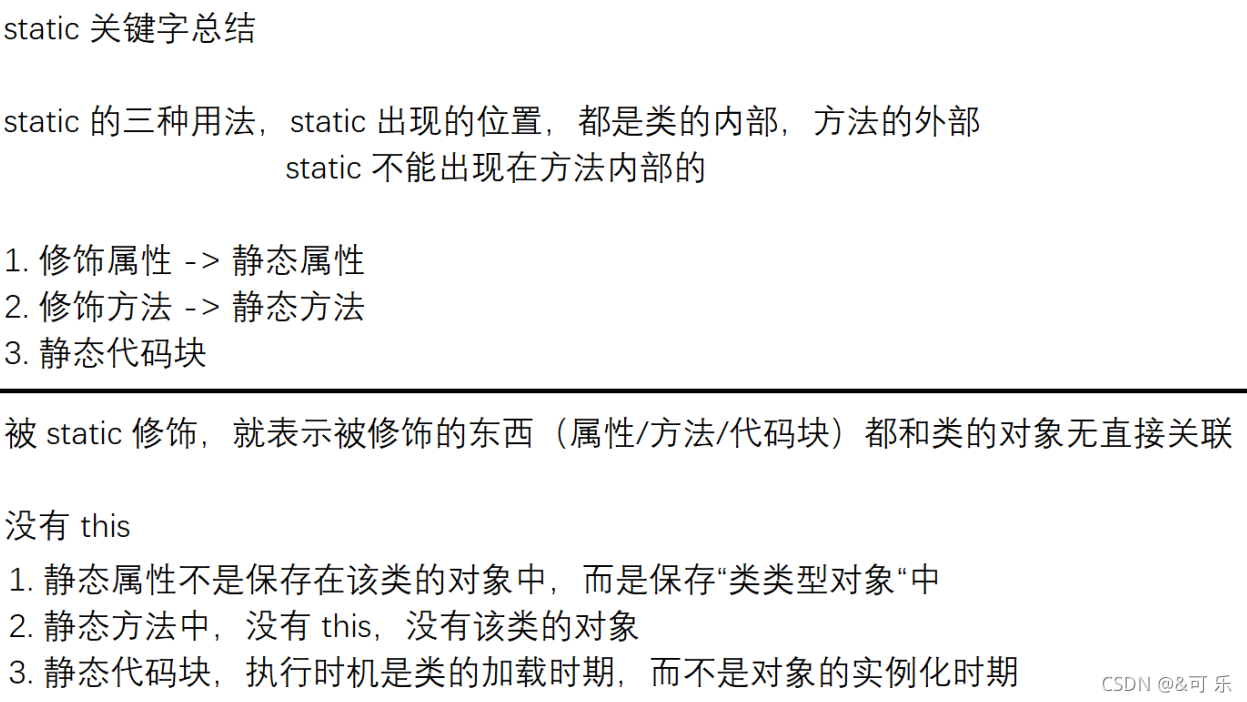  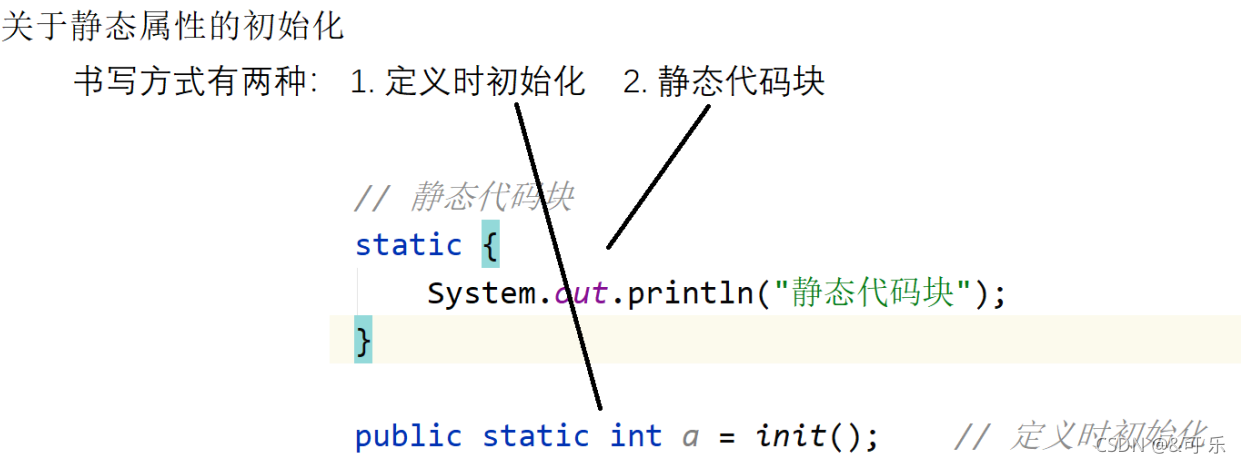 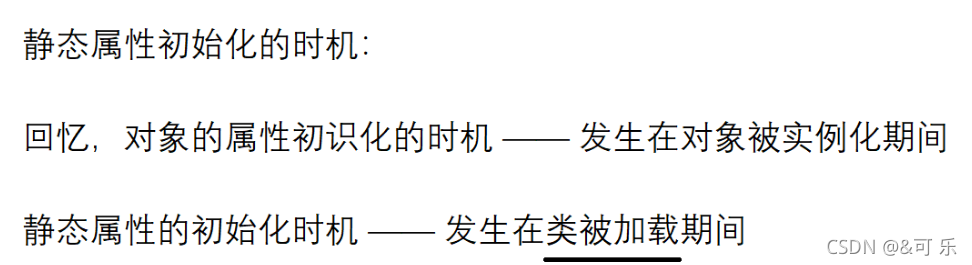
this关键字
this关键字是一个引用,java编译器给每个“成员方法“增加了一个隐藏的引用类型参数,让该引用参数指向当前对象(成员方法运行时调用该成员方法的对象)。 举例:
public class Date {
public int year;
public int month;
public int day;
public void setDay(int year, int month, int day){
this.year = year;
this.month = month;
this.day = day;
}
public void printDate(){
System.out.println(this.year + "/" + this.month + "/" + this.day);
}
}
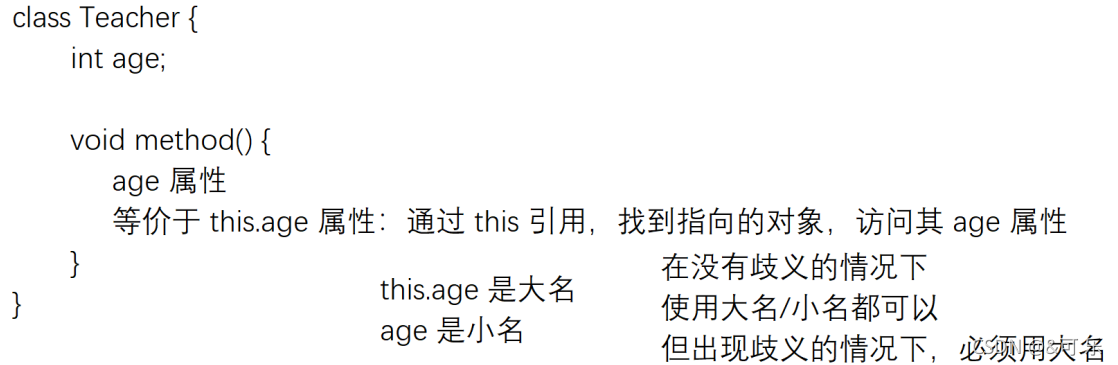
注意:this引用的是调用成员方法的对象 特点: 1.this引用的类型:对应类类型引用,即那个对象调用就是那个对象的引用类型 2. this引用只能在"成员方法中使用" 3. this引用具有final属性,在一个成员方法中,不能再去引用其他的对象 4. this引用是成员方法第一个隐藏的参数,编译器会自动传递,在成员方法执行时,编译器会负责将 调用成员方法对象的引用传递给该成员方法,this引用负责来接收 5. 在成员函数中,所有成员变量的访问,都会被编译器修改成通过this来访问
通过this调用的方法
通过this调用类中的属性
举例:这涉及到构造方法的重载
class Person {
private String name;
private int age;
private String sex;
public Person() {
this.name = "caocao";
this.age = 10;
this.sex = "男";
}
public Person(String name,int age,String sex) {
this.name = name;
this.age = age;
this.sex = sex;
}
public void show(){
System.out.println("name: "+this.name+" age: "+this.age+" sex:
"+this.sex);
}
}
public class Main{
public static void main(String[] args) {
Person p1 = new Person();
的构造函数
p1.show();
Person p2 = new Person("zhangfei",80,"男");
p2.show();
}
}
name: caocao age: 10 sex: 男
name: zhangfei age: 80 sex: 男
通过this调用类中的方法
构造方法调用构造方法
class Person {
private String name;
private int age;
private String sex;
public Person() {
this.name = "caocao";
this.age = 10;
this.sex = "男";
}
public Person(String name,int age,String sex) {
this.name = name;
this.age = age;
this.sex = sex;
}
public void eat() {
System.out.println(this.name+" 正在吃饭!");
}
public void show(){
System.out.println("name: "+this.name+" age: "+this.age+" sex:
"+this.sex);
this.eat();
}
}
public class Main{
public static void main(String[] args) {
Person p1 = new Person();
p1.show();
Person p2 = new Person("zhangfei",80,"男");
p2.show();
}
}
通过this调用自身的构造方法
这个方法有不少限制条件
class Person {
private String name;
private int age;
private String sex;
public Person() {
this("bit", 12, "man");
}
public Person(String name,int age,String sex) {
this.name = name;
this.age = age;
this.sex = sex;
}
public void show() {
System.out.println("name: "+name+" age: "+age+" sex: "+sex);
}
}
public class Main{
public static void main(String[] args) {
Person person = new Person();
person.show();
}
}
注意: 场景:需要在一个构造方法当中,调用当前类的另外一个构造方法的时候,通过this()的形式调用。 必须放在第一行,且只能调用一个 使用this调用构造方法的时候,只能在构造函数当中使用,不能再普通方法当中使用 举例:
public Person() {
this("caocao",12);
}
public Person(String name,int age,String sex) {
this.name = name;
this.age = age;
this.sex = sex; }
public Person(String name,int age ) {
this.name = name;
this.age = age; }
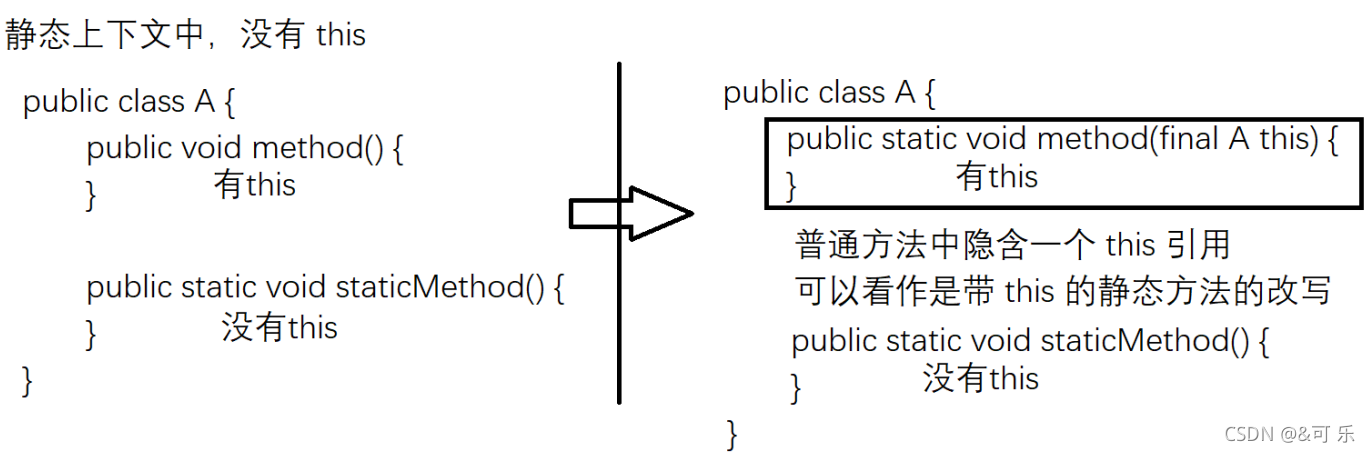
private关键字
private/ public 这两个关键字表示 “访问权限控制” 被 public 修饰的成员变量或者成员方法, 可以直接被类的调用者使用. 被 private 修饰的成员变量或者成员方法, 不能被类的调用者使用. 举例: 直接用public
class Person {
public String name = "张三";
public int age = 18; }
class Test {
public static void main(String[] args) {
Person person = new Person();
System.out.println("我叫" + person.name + ", 今年" + person.age + "岁");
}
}
举例:使用 private 修饰属性, 并提供 public 方法供类的调用者使用 当属性被private修饰之后,类外不可以直接进行访问。
class Person {
private String name = "张三";
private int age = 18;
public void show() {
System.out.println("我叫" + name + ", 今年" + age + "岁");
}
}
class Test {
public static void main(String[] args) {
Person person = new Person();
person.show();
}
}
类的加载
虚拟机把描述类的数据从 Class 文件加载到内存,并对数据进行校验、转换解析和初始化,最终形成可以被虚拟机直接使用的 Java 类型,这就是虚拟机的类加载机制。 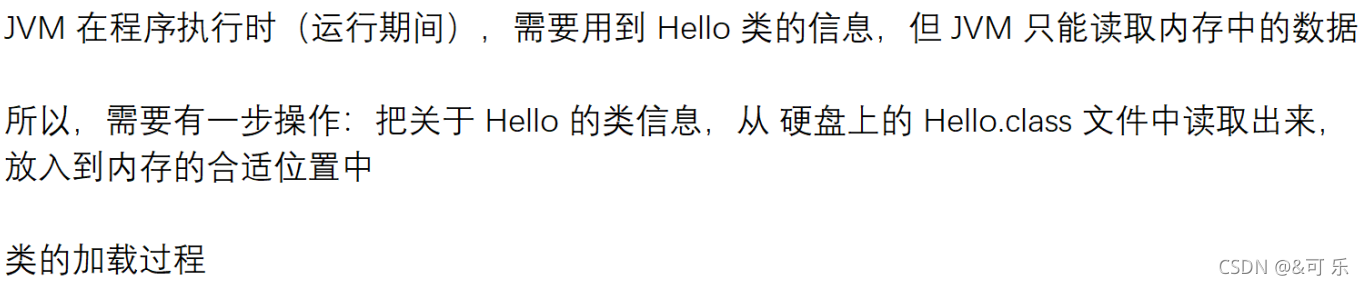 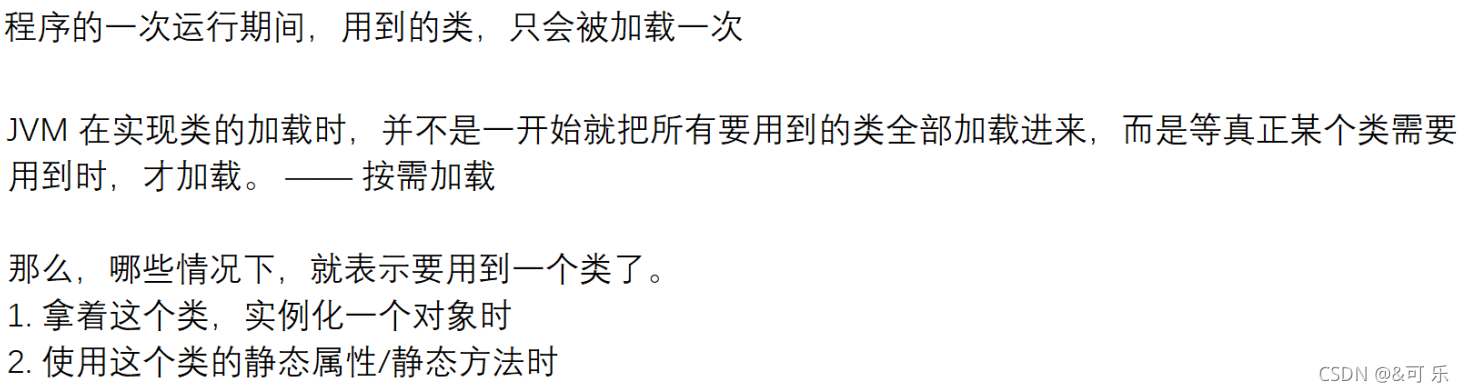
public class Hello {
static {
System.out.println("Hello 类被加载");
}
public static void main(String[] args) {
System.out.println("Hello");
new Hello();
}
}
toString方法将对象自动转成字符串
举例:先直接打印,这样出来是地址的哈希值
class Person {
private String name;
private int age;
public Person(String name,int age) {
this.age = age;
this.name = name;
}
public void show() {
System.out.println("name:"+name+" " + "age:"+age);
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("caocao",19);
person.show();
System.out.println(person);
}
}

用toString方法转
class Person {
private String name;
private int age;
public Person(String name,int age) {
this.age = age;
this.name = name;
}
public void show() {
System.out.println("name:"+name+" " + "age:"+age);
}
@Override
public String toString() {
return "Person{" +
"name='" + name + '\'' +
", age=" + age +
'}';
}
}
public class Main {
public static void main(String[] args) {
Person person = new Person("caocao",19);
person.show();
System.out.println(person);
}
}
name:caocao age:19
Person{name='caocao', age=19}
注意: toString 方法会在 println 的时候被自动调用. 将对象转成字符串这样的操作我们称为 序列化
小结
一个类可以产生无数的对象,类就是模板,对象就是具体的实例。 类中定义的属性,大概分为几类:类属性,对象属性。其中被static所修饰的数据属性称为类属性, static修饰的方法称为类方法,特点是不依赖于对象,我们只需要通过类名就可以调用其属性或者 方法。 this关键字代表的是当前对象的引用。并不是当前对象。 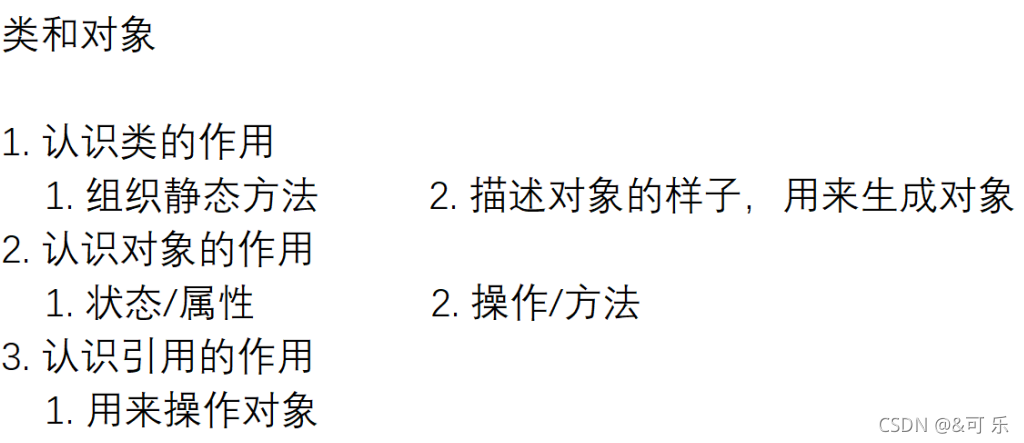
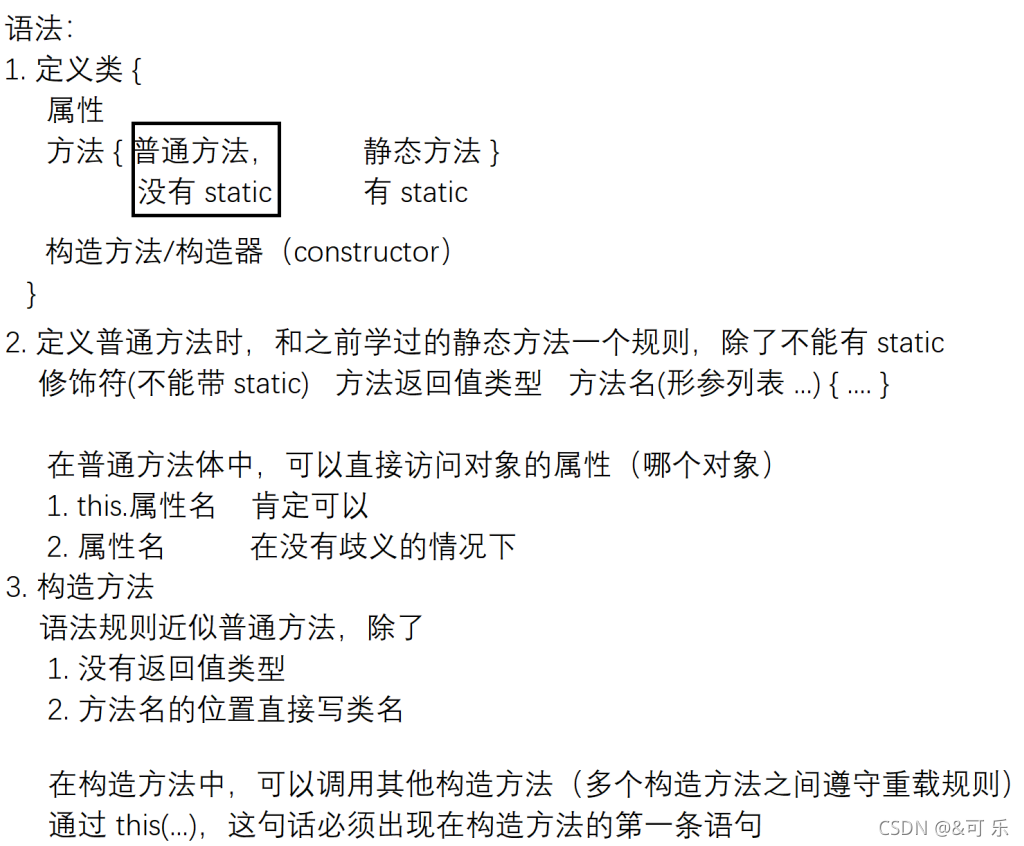 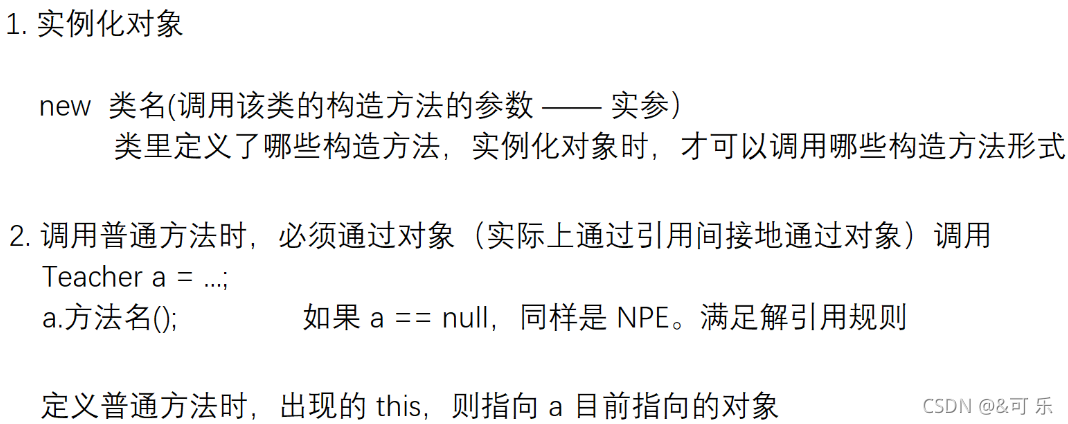 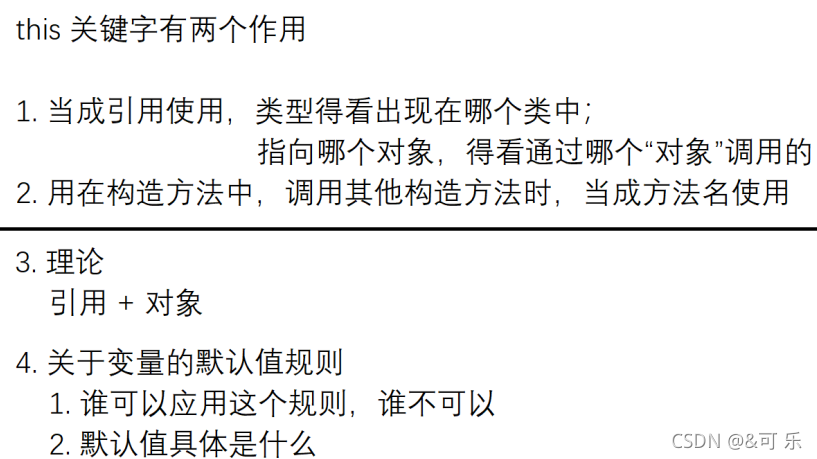
|