Java
Java - 爪哇岛 盛产咖啡 Java之父 —— 高斯林
Write Once. Run anywhere. 一次运行,到处运行
环境:
JDK - Java开发者工具
IDEA - 集成开发环境
初识Java的main方法
public class HelloWorld {
public statci void main(String[] args) {
System.out.println("hello");
}
}

用Sublime Text3编译运行
如何编译
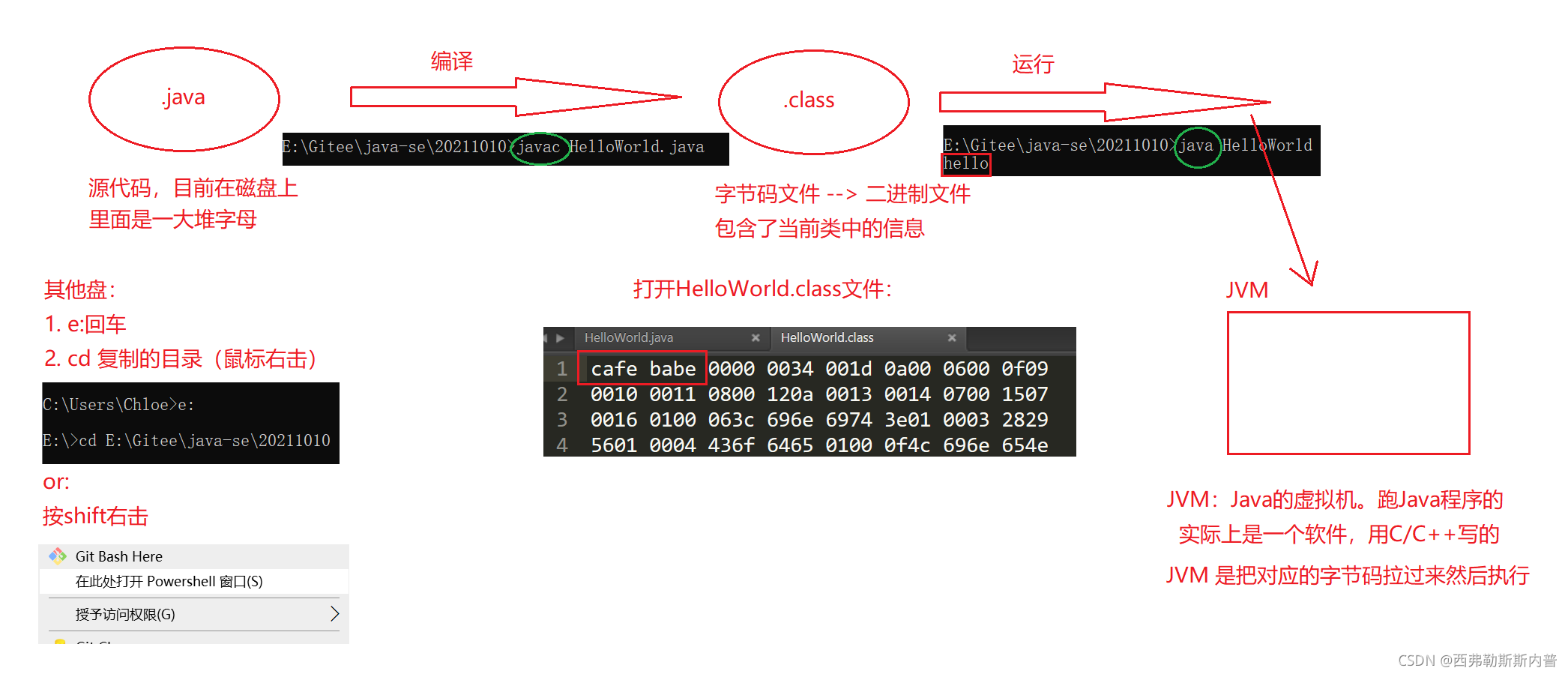
程序运行原理
JDK:Java开发者工具
JRE:Java运行环境
JVM:Java虚拟机
一次编译,到处运行,只要你安装了JDK 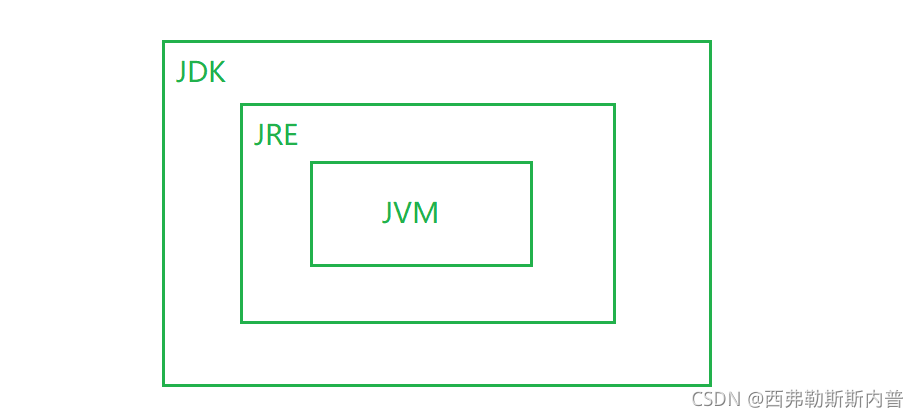
程序需注意的问题
1、每次写完代码,必须保存,然后重新编译!!
2、如果这个类是public修饰的,类名需要和文件名一致 public class HelloWorld
3、方法需在类里 --> 类(方法)
4、不是一个文件对应一个字节码,而是一个类对应一个字节码
? 好处:用到哪个类,加载哪个类,而不是全加载!
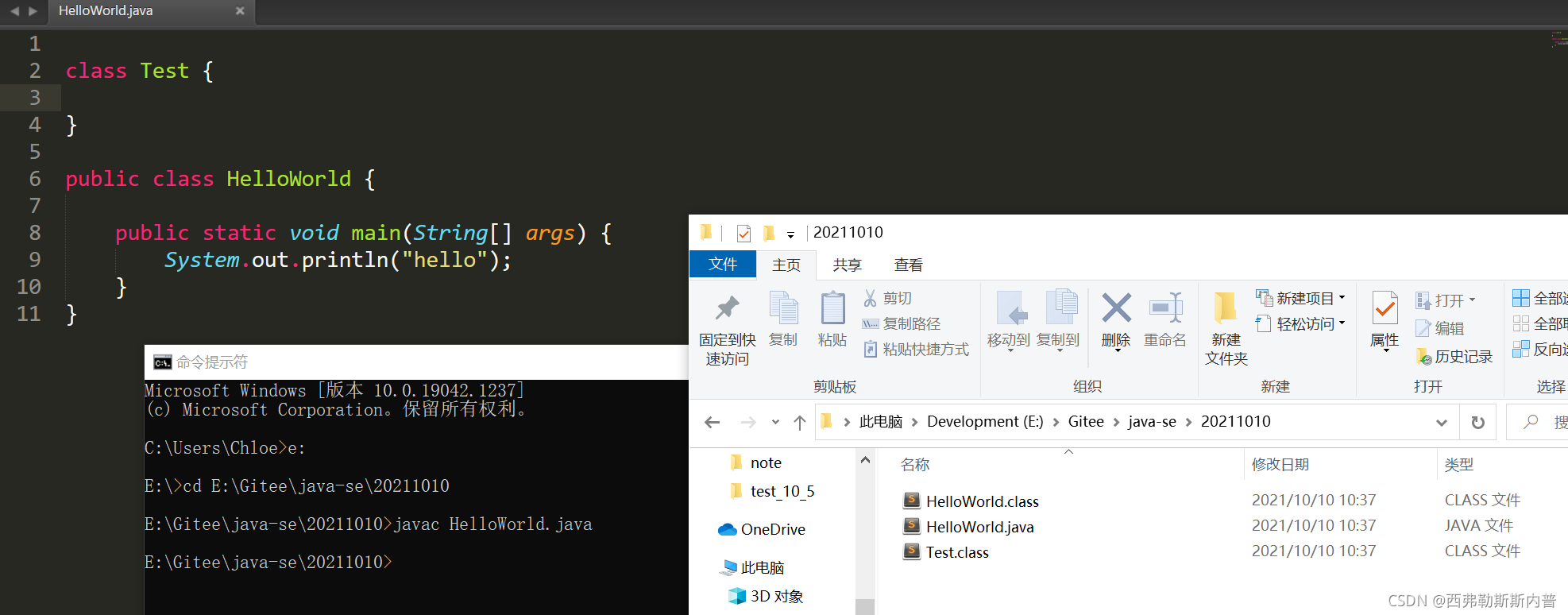
args
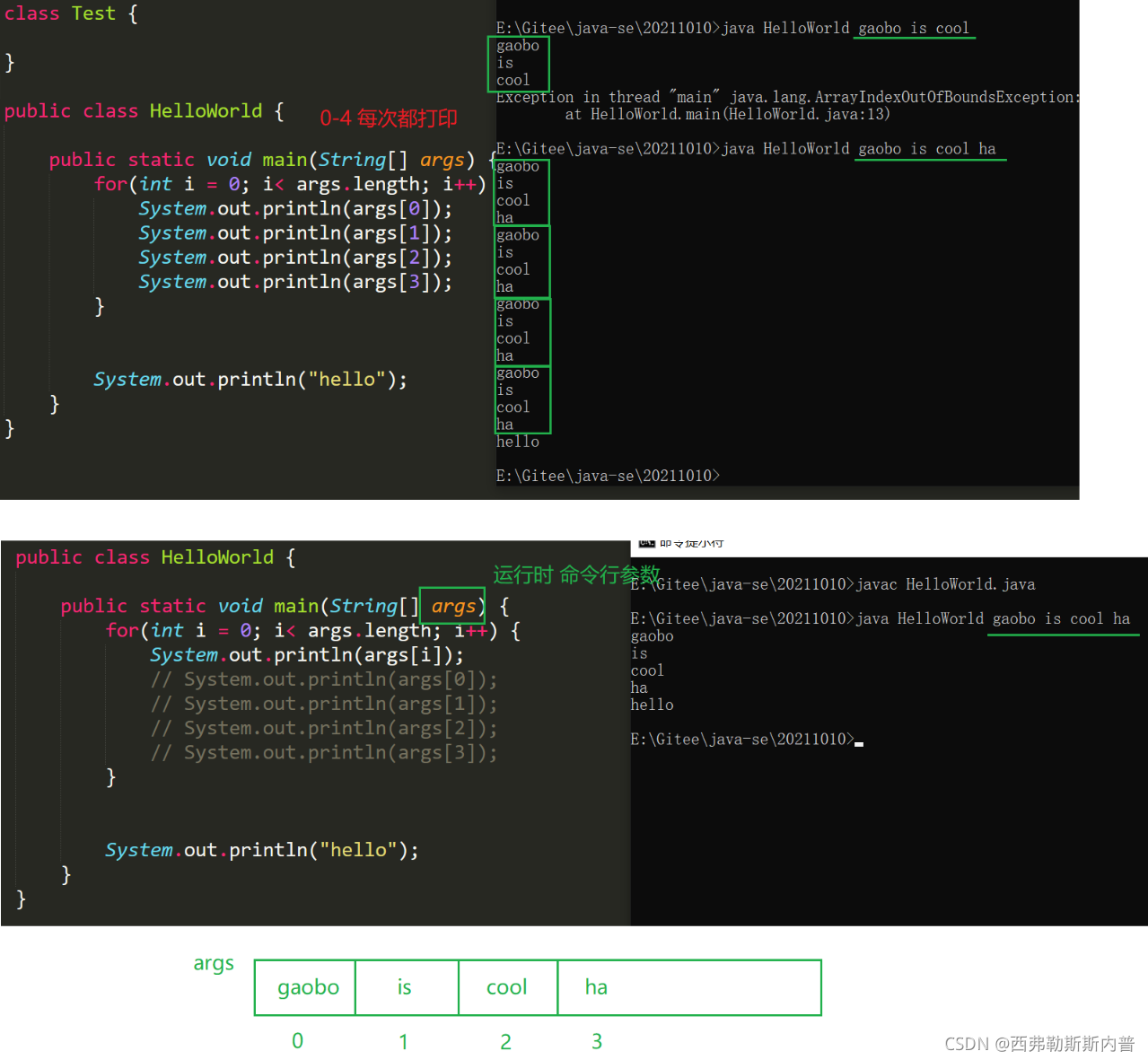
数组越界: 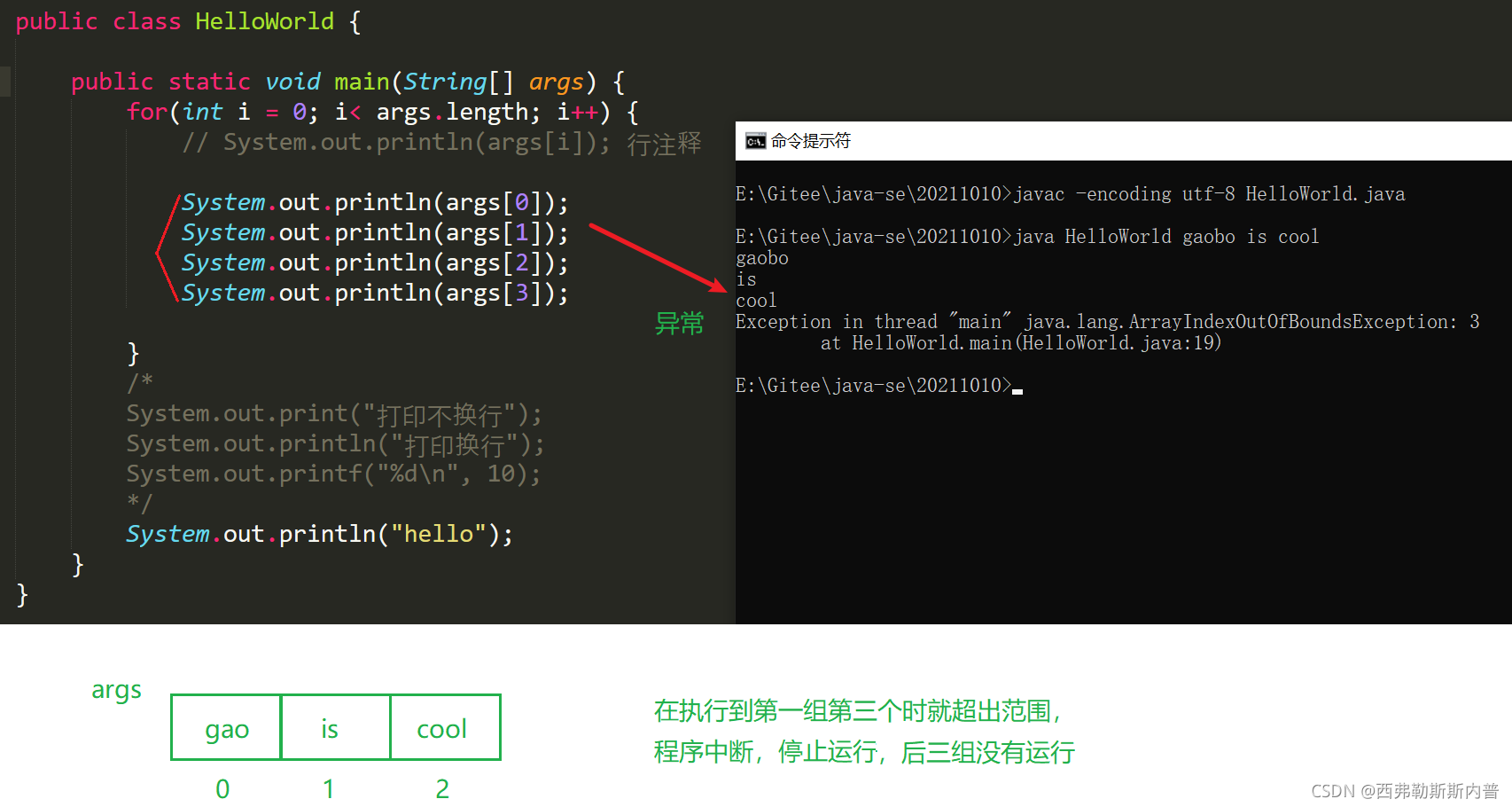
注释与打印
三种注释方法:
public class HelloWorld {
public static void main(String[] args) {
for(int i = 0; i< args.length; i++) {
}
System.out.println("hello");
}
}
运行此代码产生错误问题:
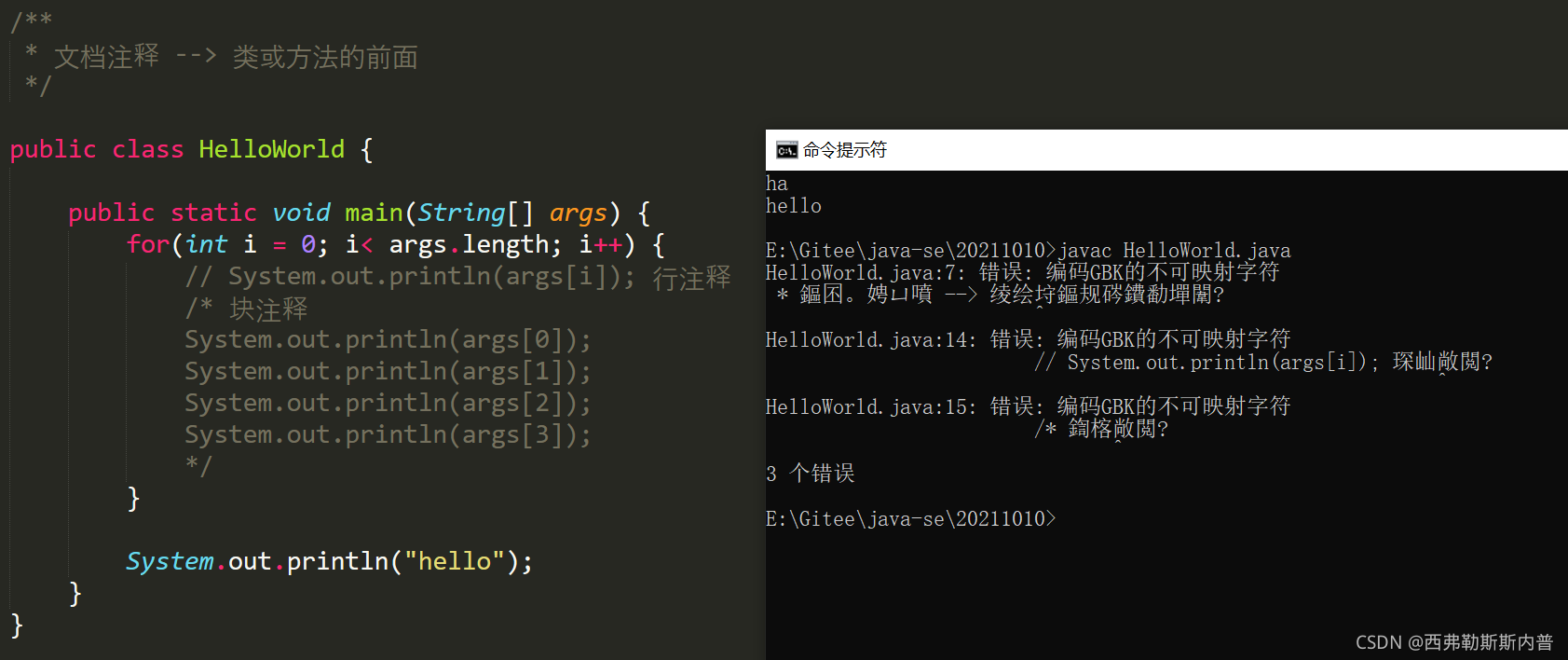
javac 在编译的时候,默认用GDK格式编译代码,
解决方法:javac -encoding utf-8 HelloWorld.java
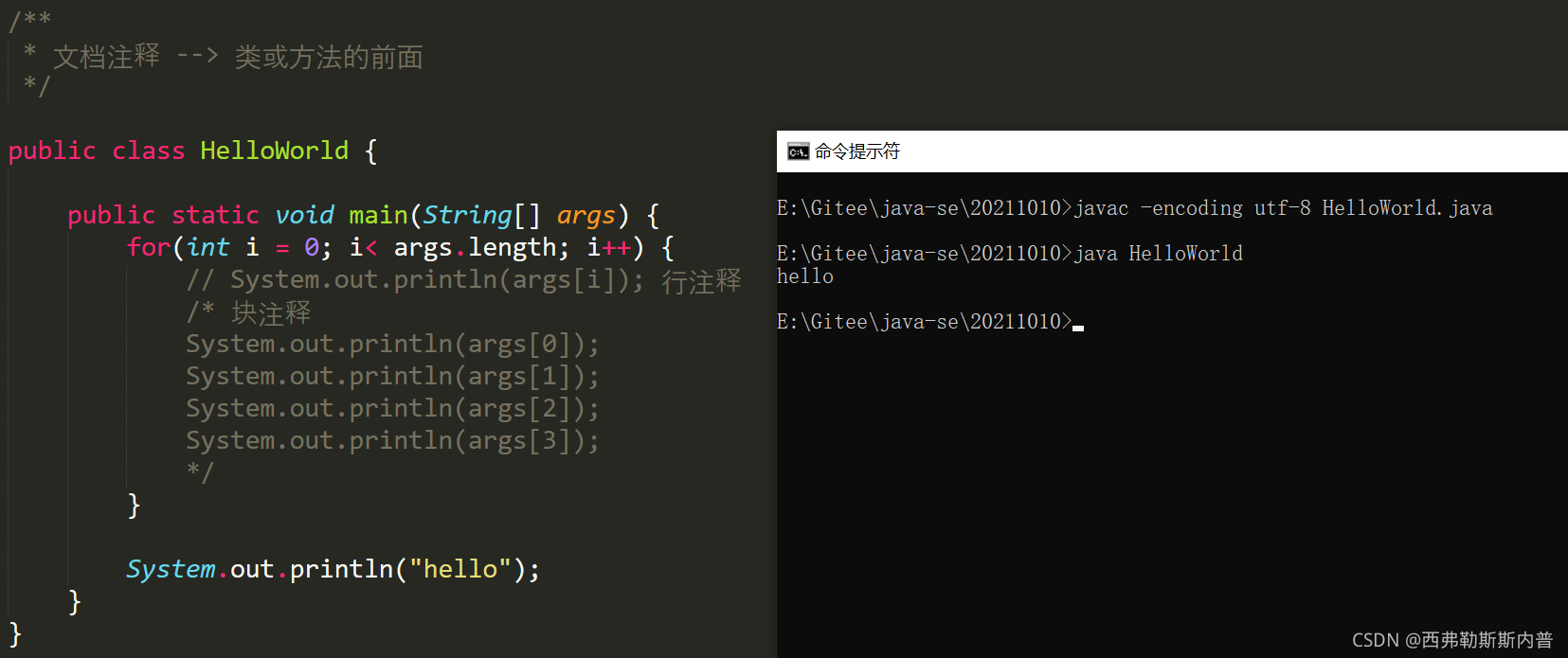
打印方法:
public class HelloWorld {
public static void main(String[] args) {
for(int i = 0; i< args.length; i++) {
System.out.print("打印不换行");
System.out.println("打印换行");
System.out.printf("%d\n", 10);
}
}
变量与类型
类型特性
1、int 多少字节?
int 4字节,无关多少位系统 — 可移植性高
Linux Mac Win 可要有字节码文件,就能编译 — 跨平台
public class HelloWorld {
public static void main(String[] args) {
int a = 10;
}
}
2、有无符号?
Java 中的 int,没有所谓的无符号类型,统一是有符号的
public class HelloWorld {
public static void main(String[] args) {
int a = 10;
System.out.println(a);
}
}
3、取值范围?
-
最高位为有符号位,剩余31位是数值位 这31位可表示 2^31 = 2,147,483,648 个数值 -
int 取值范围:-2^31 -> 2^31-1 -2147483648 — 0 — 2147483647
public class HelloWorld {
public static void main(String[] args) {
int a = 10;
System.out.println(a);
System.out.println(Integer.MAX_VALUE);
System.out.println(Integer.MIN_VALUE);
}
}
变量类型
1、变量命名
小驼峰:以(数字 字母 下划线 美元符号)组成,不能以数字开头
public class HelloWorld {
public static void main(String[] args) {
int maxMax = 10;
int max_num = 10;
int $max3num = 10;
int ___________ = 100;
System.out.println(___________);
int 钱 = 300;
System.out.println(钱);
}
}
2、变量能否不初始化?
Java 比较安全,如果不初始化就使用,编译器就会报错,不是警告!
int num; // err
3、long 长整形
8bit 64bit
long 的最大最小值:-2^63 2^63-1
取值范围:
public class HelloWorld {
public static void main(String[] args) {
long a = 10L;
System.out.println(Long.MAX_VALUE);
System.out.println(Long.MIN_VALUE);
}
}
4、双精度浮点型变量
注意问题1:

注意问题2:
小数本身是没有一个精准的数字的,只能精确到几位
public class HelloWorld {
public static void main(String[] args) {
double num = 1.1;
System.out.println(num*num);
}
}
5、单精度浮点型变量
public class HelloWorld {
public static void main(String[] args) {
float f = 10.5;
System.out.println(f);
}
}
6、 字符类型变量
public class HelloWorld {
public static void main(String[] args) {
char ch1 = 'a';
System.out.println(ch1);
char ch2 = '数';
System.out.println(ch2);
}
}
汉字2字节,代码无问题,由此可得 char 区别于C语言是2字节
在C语言中使用ASCII 表示字符,在Java 中使用Unicode 表示字符,表示的字符种类更多,包括中文
7、 字节类型
public class HelloWorld {
public static void main(String[] args) {
byte b = 10;
System.out.println(b1);
}
}
字节类型取值范围:
public class HelloWorld {
public static void main(String[] args) {
byte b1 = 130;
System.out.println(b2);
byte b2 = 127;
}
}
1字节 --> 8bit 最高位符号位,7位数值位
-2^7 - 2^7-1
-128 - 127
8、短整型
public class HelloWorld {
public static void main(String[] args) {
short s = 10;
}
}
|