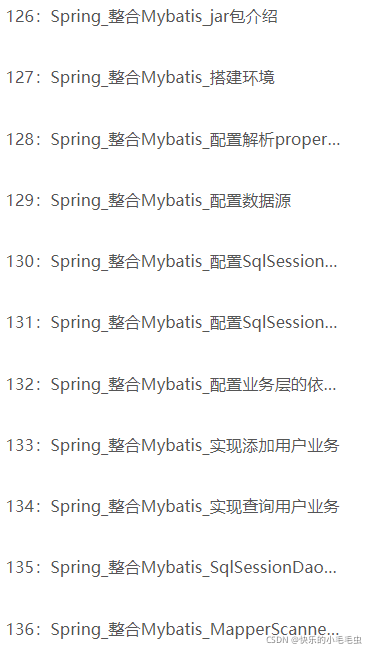
?
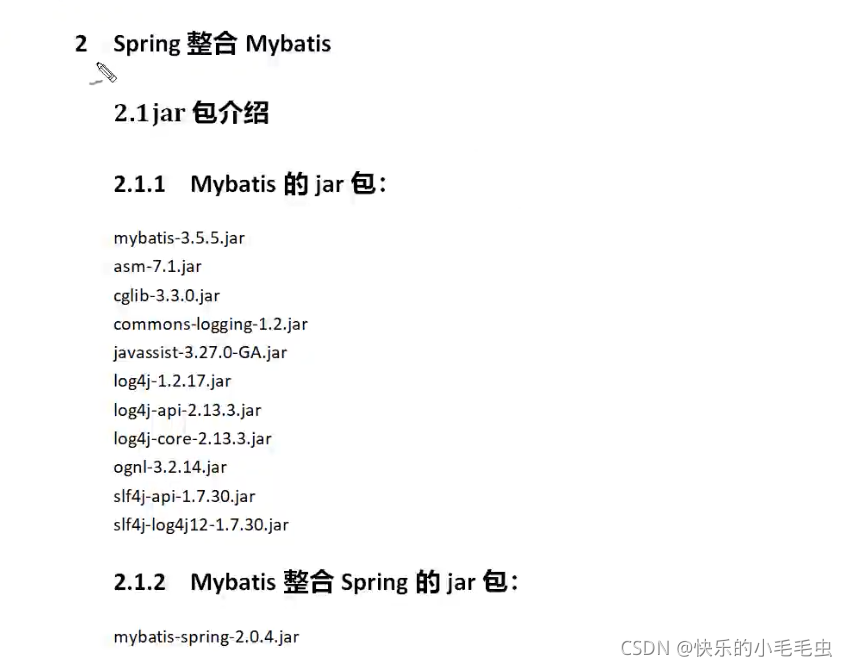
?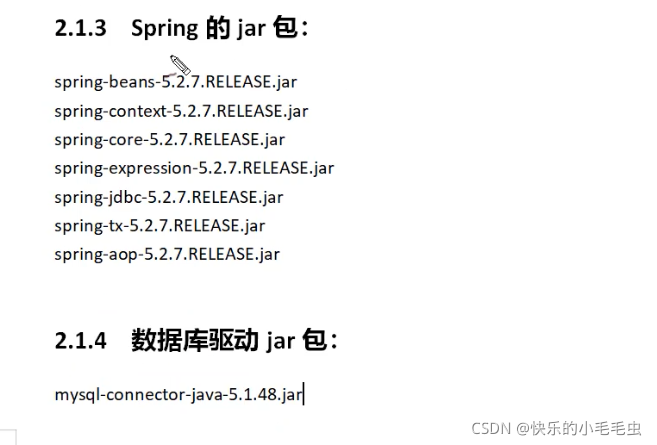
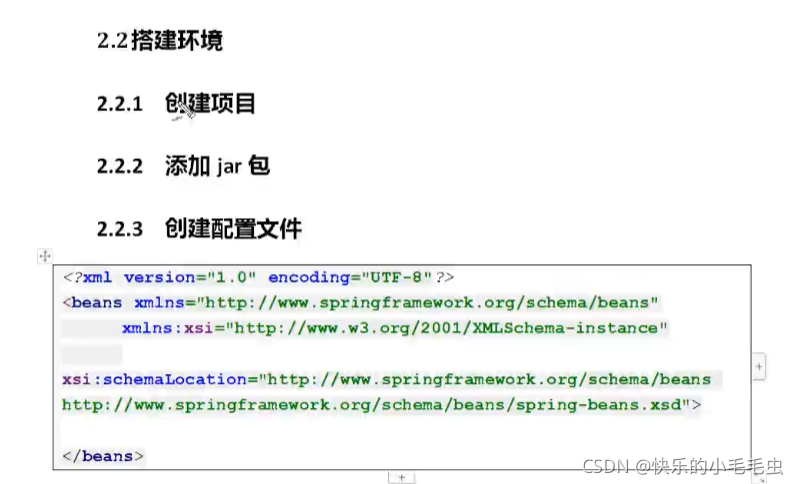
?
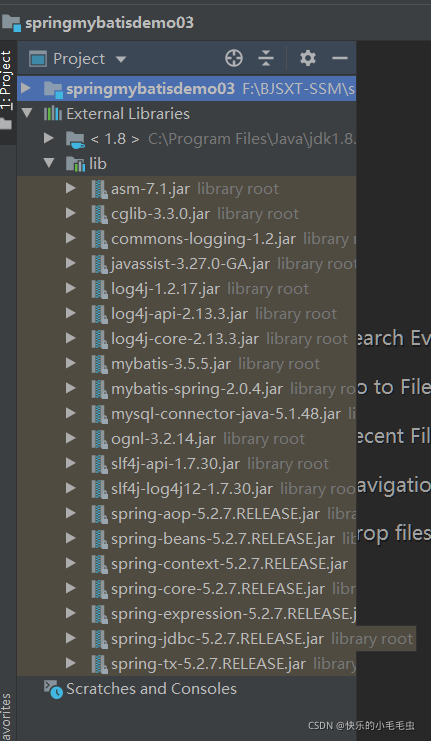
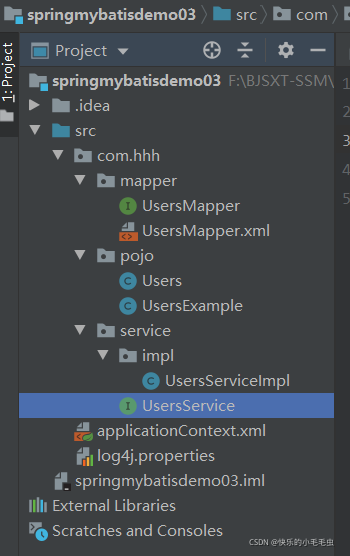
?下面是UsersMapper.xml
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd" >
<mapper namespace="com.hhh.mapper.UsersMapper" >
<resultMap id="BaseResultMap" type="com.hhh.pojo.Users" >
<id column="userid" property="userid" jdbcType="INTEGER" />
<result column="username" property="username" jdbcType="VARCHAR" />
<result column="usersex" property="usersex" jdbcType="VARCHAR" />
</resultMap>
<sql id="Example_Where_Clause" >
<where >
<foreach collection="oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Update_By_Example_Where_Clause" >
<where >
<foreach collection="example.oredCriteria" item="criteria" separator="or" >
<if test="criteria.valid" >
<trim prefix="(" suffix=")" prefixOverrides="and" >
<foreach collection="criteria.criteria" item="criterion" >
<choose >
<when test="criterion.noValue" >
and ${criterion.condition}
</when>
<when test="criterion.singleValue" >
and ${criterion.condition} #{criterion.value}
</when>
<when test="criterion.betweenValue" >
and ${criterion.condition} #{criterion.value} and #{criterion.secondValue}
</when>
<when test="criterion.listValue" >
and ${criterion.condition}
<foreach collection="criterion.value" item="listItem" open="(" close=")" separator="," >
#{listItem}
</foreach>
</when>
</choose>
</foreach>
</trim>
</if>
</foreach>
</where>
</sql>
<sql id="Base_Column_List" >
userid, username, usersex
</sql>
<select id="selectByExample" resultMap="BaseResultMap" parameterType="com.hhh.pojo.UsersExample" >
select
<if test="distinct" >
distinct
</if>
<include refid="Base_Column_List" />
from users
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
<if test="orderByClause != null" >
order by ${orderByClause}
</if>
</select>
<select id="selectByPrimaryKey" resultMap="BaseResultMap" parameterType="java.lang.Integer" >
select
<include refid="Base_Column_List" />
from users
where userid = #{userid,jdbcType=INTEGER}
</select>
<delete id="deleteByPrimaryKey" parameterType="java.lang.Integer" >
delete from users
where userid = #{userid,jdbcType=INTEGER}
</delete>
<delete id="deleteByExample" parameterType="com.hhh.pojo.UsersExample" >
delete from users
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</delete>
<insert id="insert" parameterType="com.hhh.pojo.Users" >
insert into users (userid, username, usersex
)
values (#{userid,jdbcType=INTEGER}, #{username,jdbcType=VARCHAR}, #{usersex,jdbcType=VARCHAR}
)
</insert>
<insert id="insertSelective" parameterType="com.hhh.pojo.Users" >
insert into users
<trim prefix="(" suffix=")" suffixOverrides="," >
<if test="userid != null" >
userid,
</if>
<if test="username != null" >
username,
</if>
<if test="usersex != null" >
usersex,
</if>
</trim>
<trim prefix="values (" suffix=")" suffixOverrides="," >
<if test="userid != null" >
#{userid,jdbcType=INTEGER},
</if>
<if test="username != null" >
#{username,jdbcType=VARCHAR},
</if>
<if test="usersex != null" >
#{usersex,jdbcType=VARCHAR},
</if>
</trim>
</insert>
<select id="countByExample" parameterType="com.hhh.pojo.UsersExample" resultType="java.lang.Integer" >
select count(*) from users
<if test="_parameter != null" >
<include refid="Example_Where_Clause" />
</if>
</select>
<update id="updateByExampleSelective" parameterType="map" >
update users
<set >
<if test="record.userid != null" >
userid = #{record.userid,jdbcType=INTEGER},
</if>
<if test="record.username != null" >
username = #{record.username,jdbcType=VARCHAR},
</if>
<if test="record.usersex != null" >
usersex = #{record.usersex,jdbcType=VARCHAR},
</if>
</set>
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByExample" parameterType="map" >
update users
set userid = #{record.userid,jdbcType=INTEGER},
username = #{record.username,jdbcType=VARCHAR},
usersex = #{record.usersex,jdbcType=VARCHAR}
<if test="_parameter != null" >
<include refid="Update_By_Example_Where_Clause" />
</if>
</update>
<update id="updateByPrimaryKeySelective" parameterType="com.hhh.pojo.Users" >
update users
<set >
<if test="username != null" >
username = #{username,jdbcType=VARCHAR},
</if>
<if test="usersex != null" >
usersex = #{usersex,jdbcType=VARCHAR},
</if>
</set>
where userid = #{userid,jdbcType=INTEGER}
</update>
<update id="updateByPrimaryKey" parameterType="com.hhh.pojo.Users" >
update users
set username = #{username,jdbcType=VARCHAR},
usersex = #{usersex,jdbcType=VARCHAR}
where userid = #{userid,jdbcType=INTEGER}
</update>
</mapper>
下面是接口UsersMapper:
package com.hhh.mapper;
import com.hhh.pojo.Users;
import com.hhh.pojo.UsersExample;
import org.apache.ibatis.annotations.Param;
import java.util.List;
public interface UsersMapper {
int countByExample(UsersExample example);
int deleteByExample(UsersExample example);
int deleteByPrimaryKey(Integer userid);
int insert(Users record);
int insertSelective(Users record);
List<Users> selectByExample(UsersExample example);
Users selectByPrimaryKey(Integer userid);
int updateByExampleSelective(@Param("record") Users record, @Param("example") UsersExample example);
int updateByExample(@Param("record") Users record, @Param("example") UsersExample example);
int updateByPrimaryKeySelective(Users record);
int updateByPrimaryKey(Users record);
}
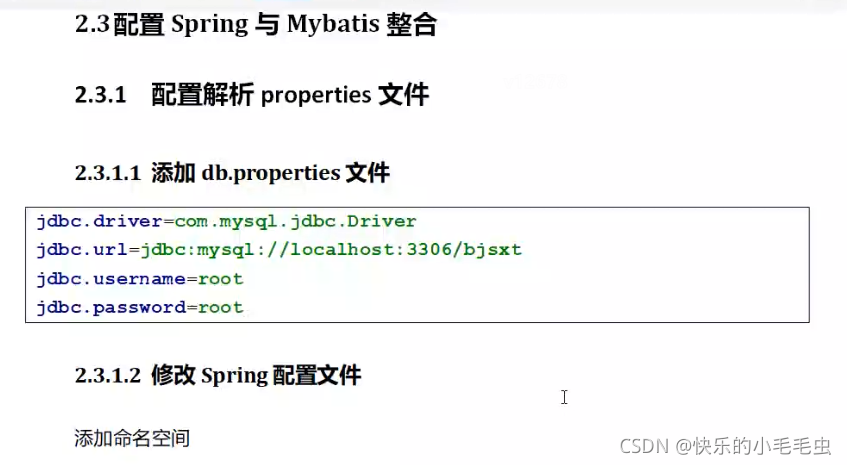
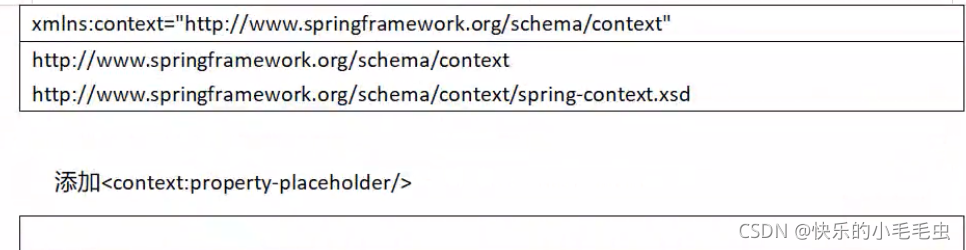
?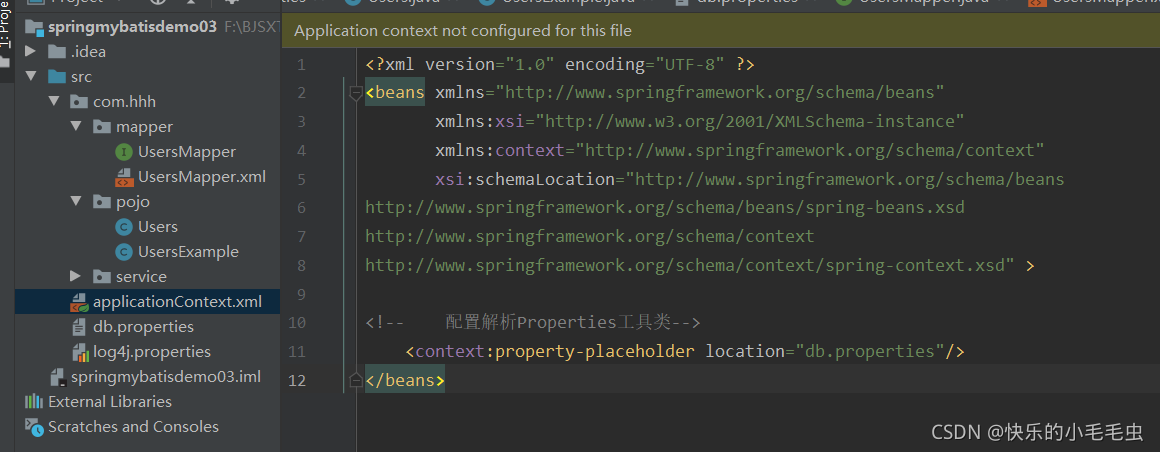
<?xml version="1.0" encoding="UTF-8" ?>
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xmlns:context="http://www.springframework.org/schema/context"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans.xsd
http://www.springframework.org/schema/context
http://www.springframework.org/schema/context/spring-context.xsd" >
<!-- 配置解析Properties工具类-->
<context:property-placeholder location="db.properties"/>
</beans>
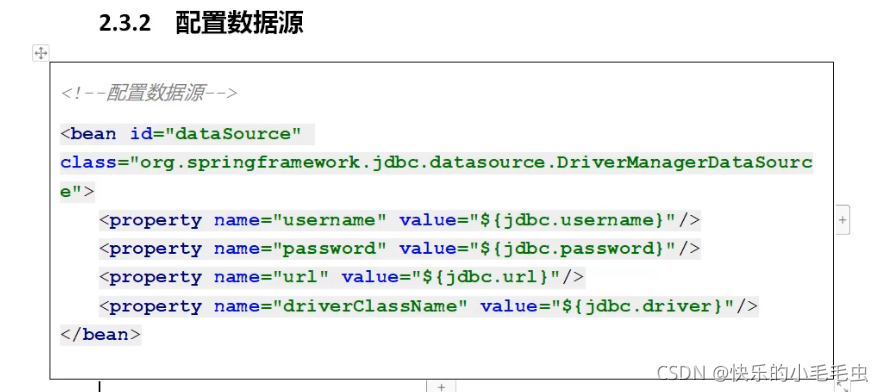
?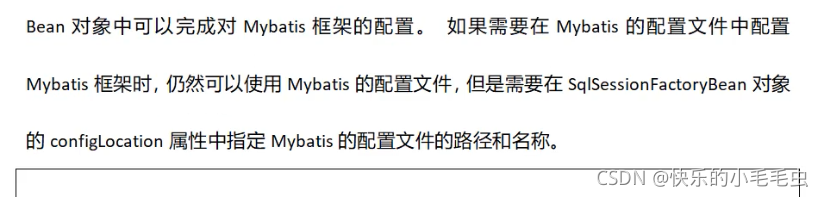
?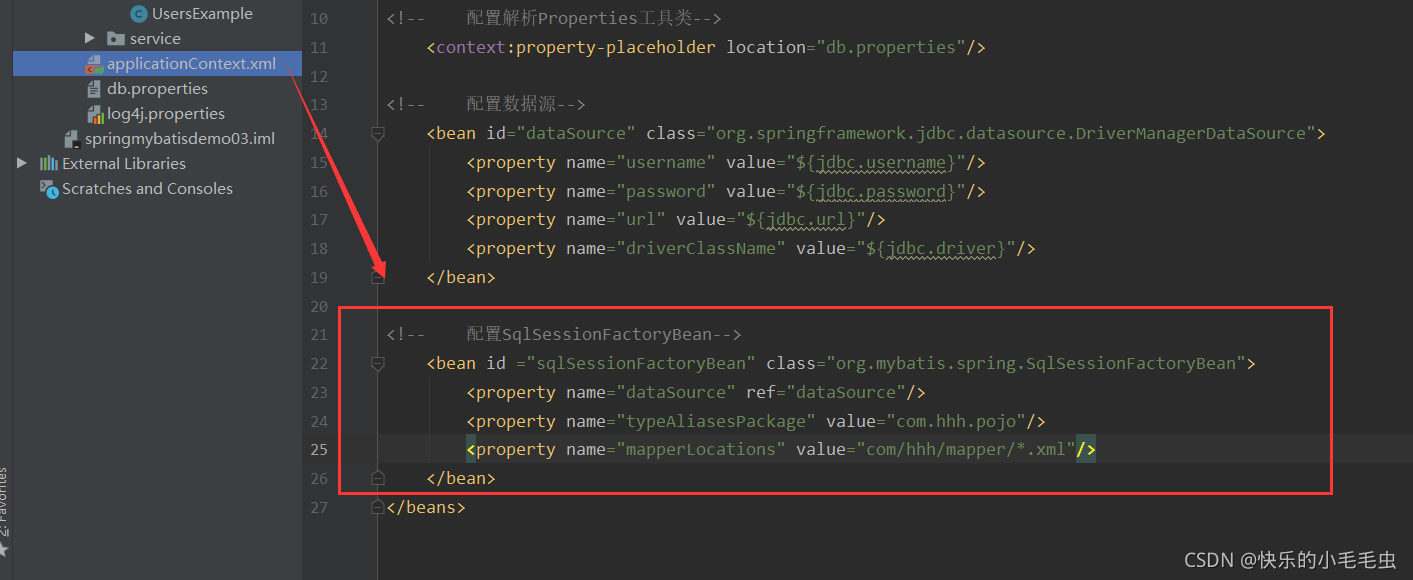
?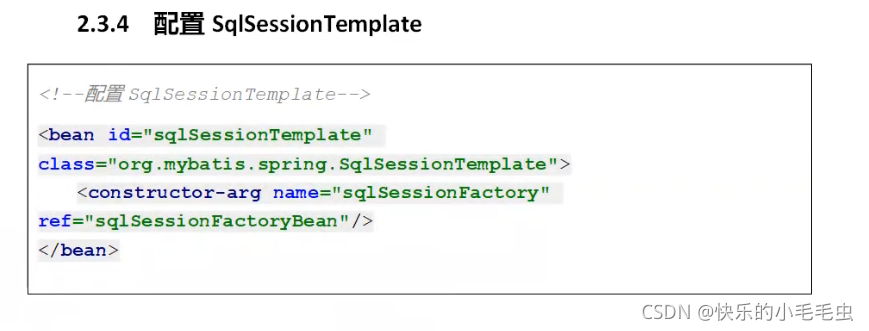
?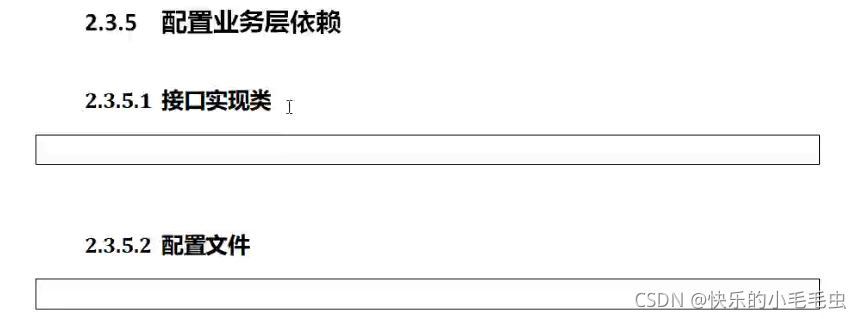
?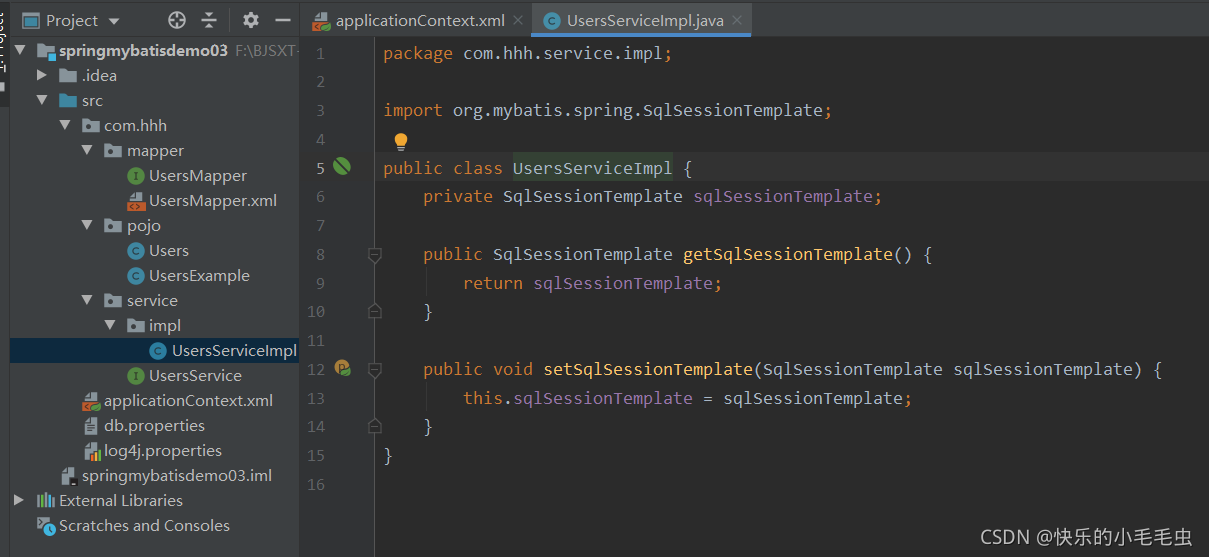
?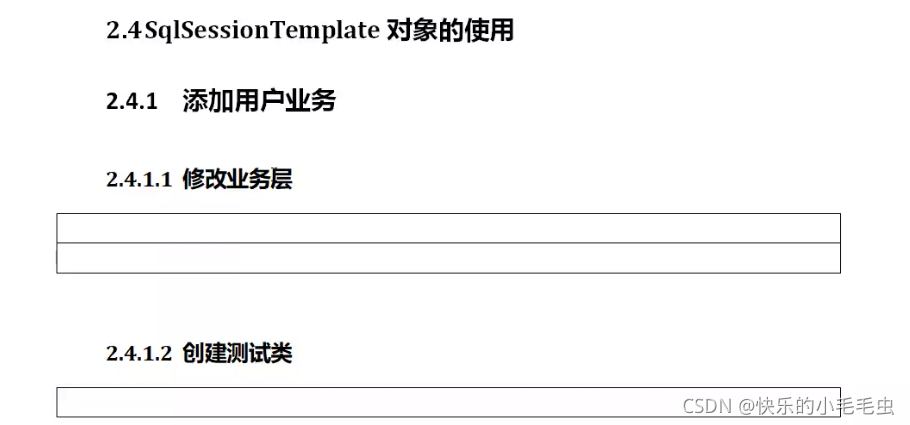
?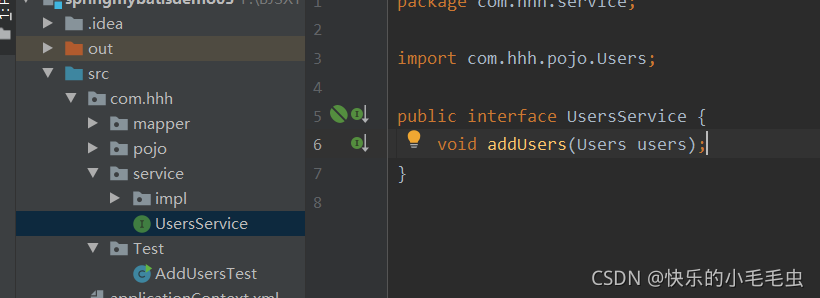
?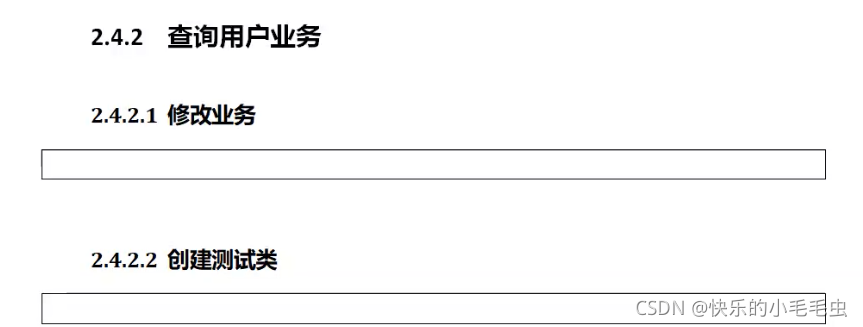
?修改业务层: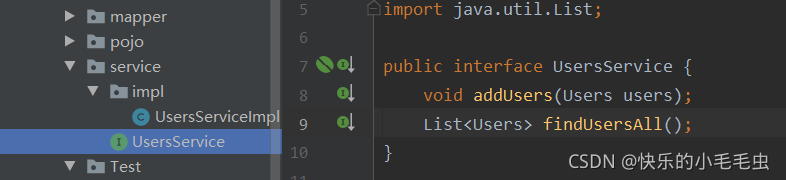
?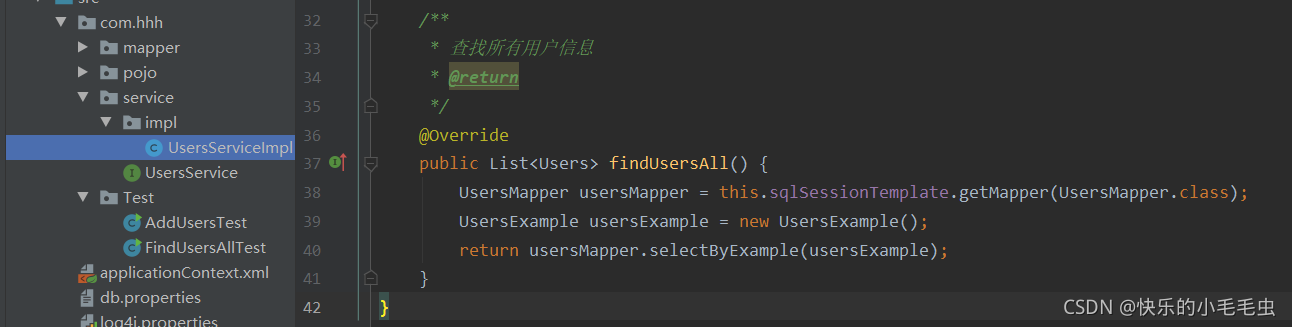
?测试:
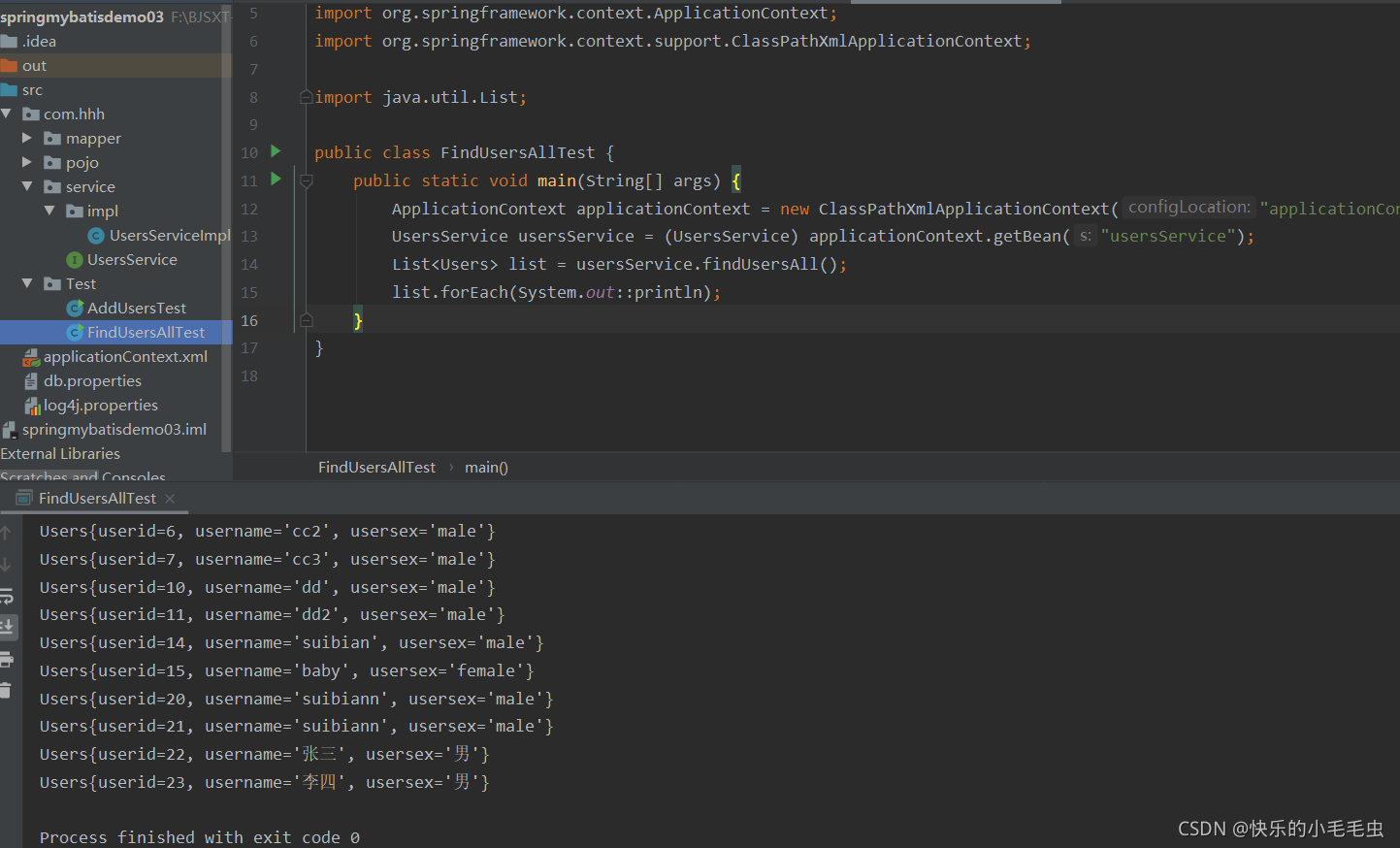
?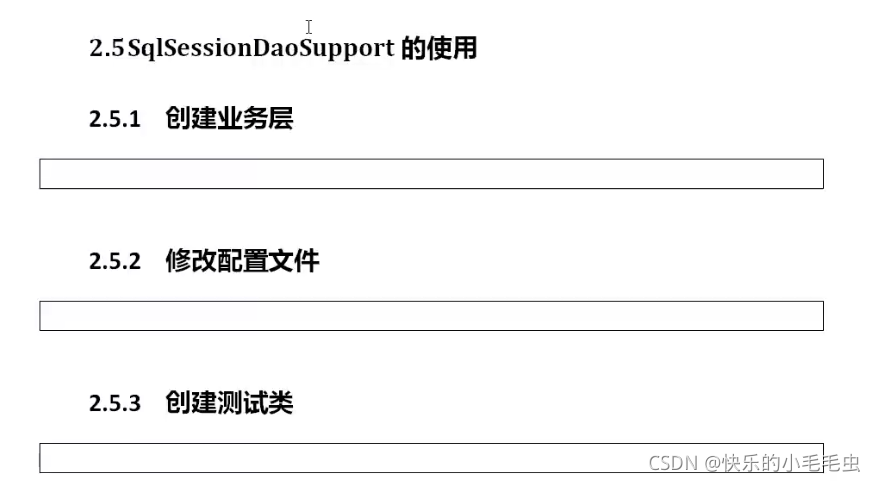
?创建业务层:直接通过SqlSessionDaoSupport来继承他的父类,来代替使用sqlSessionTemplate
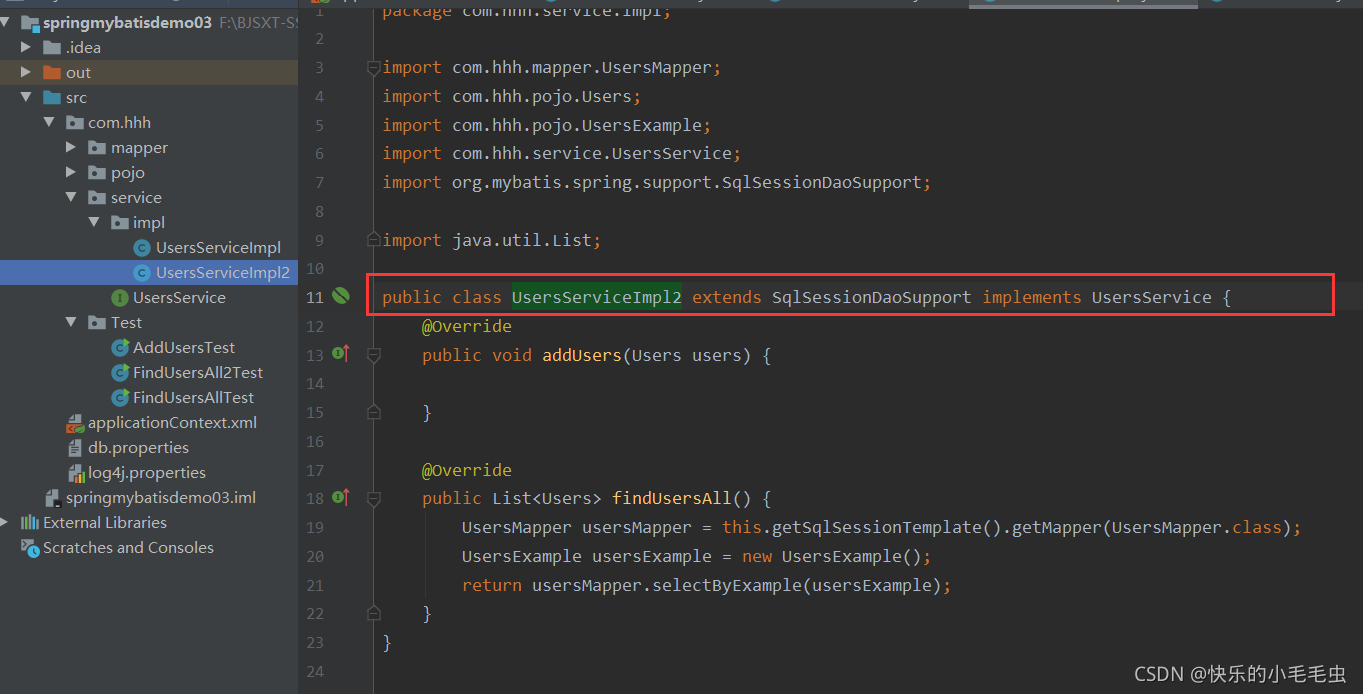
?修改配置文件:
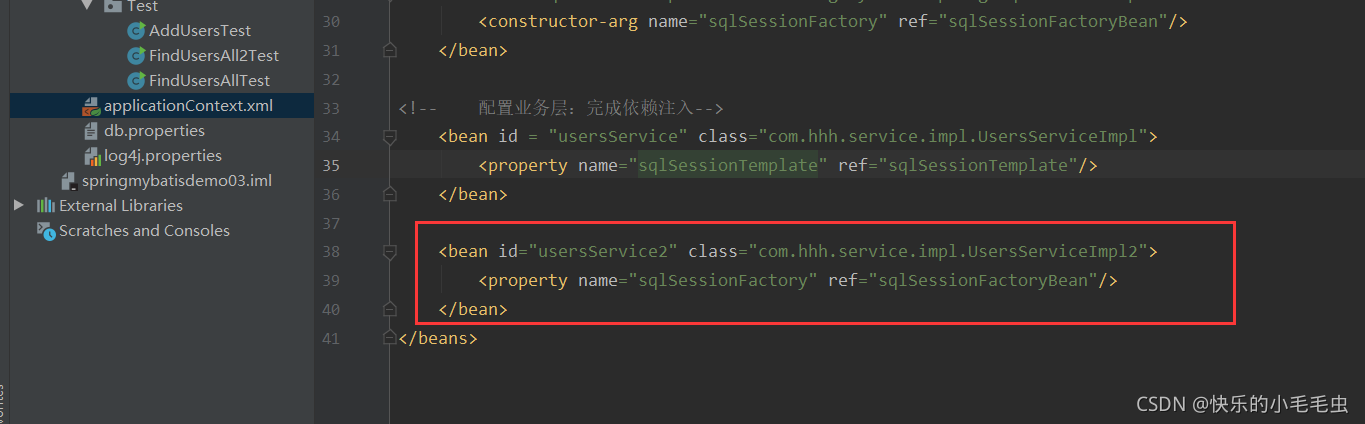
?测试:
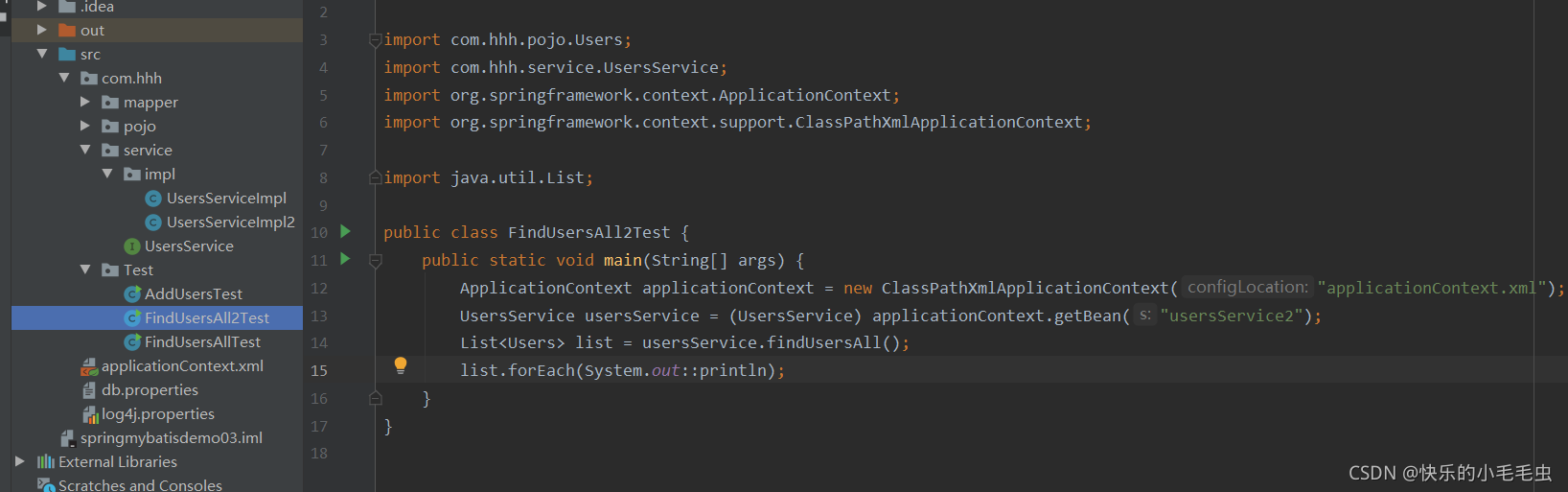
?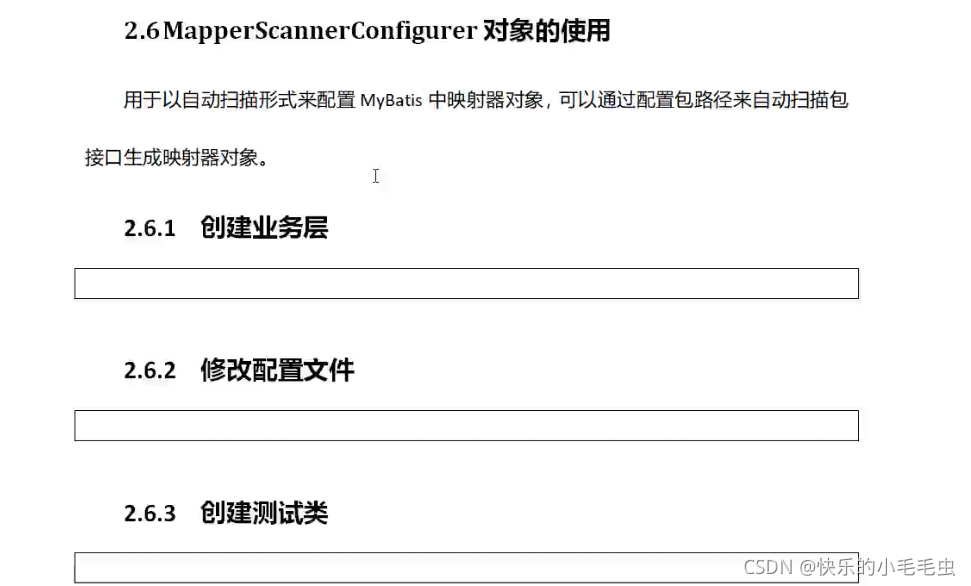
?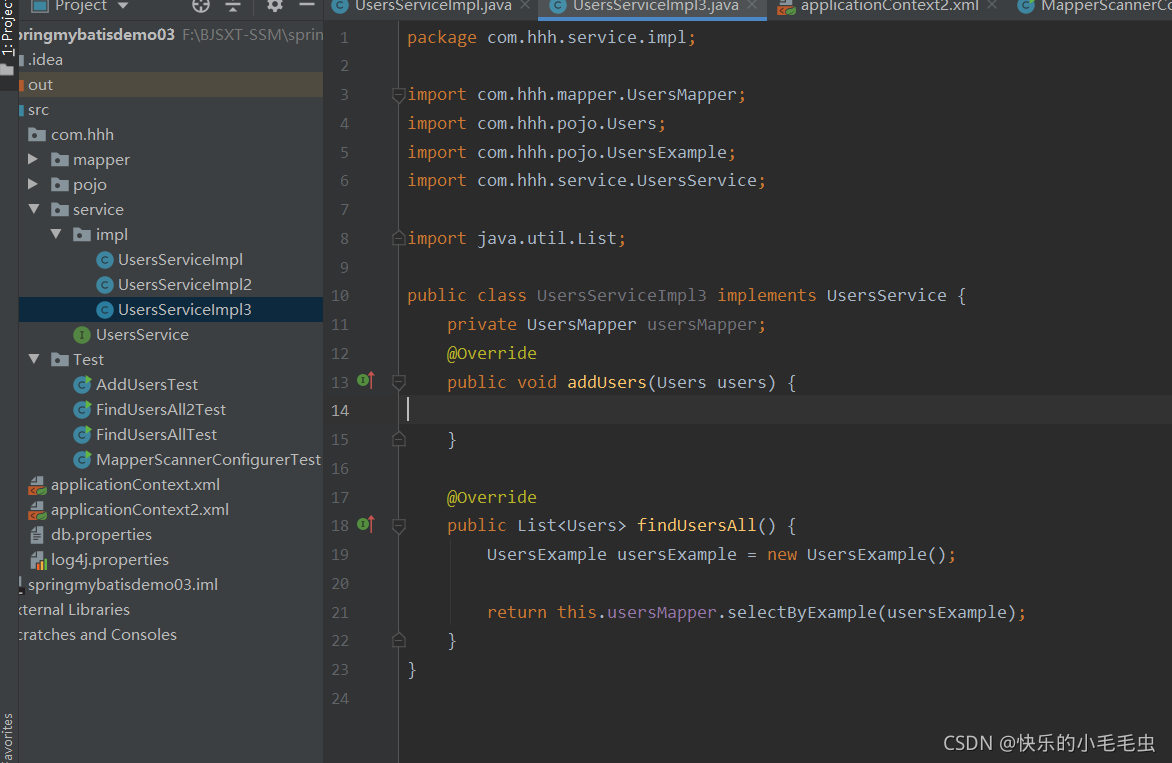
?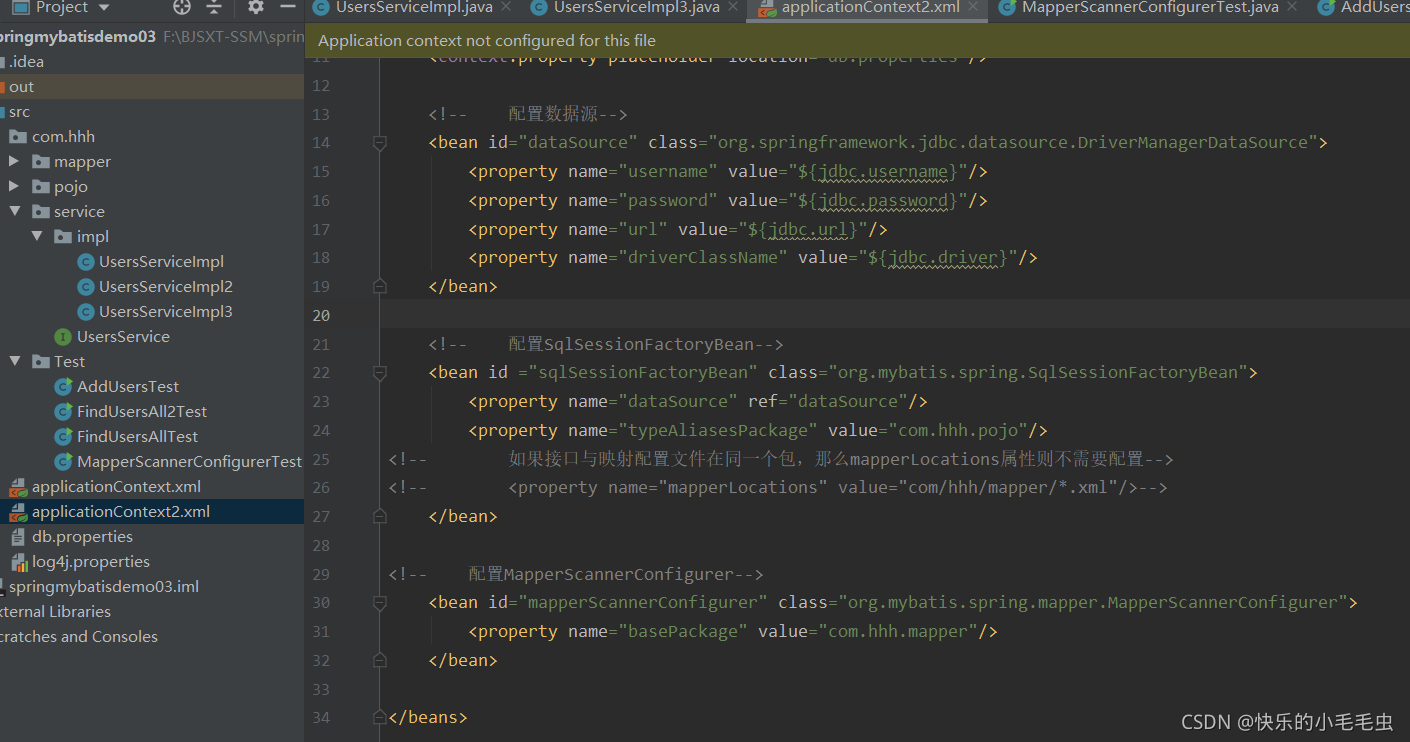
?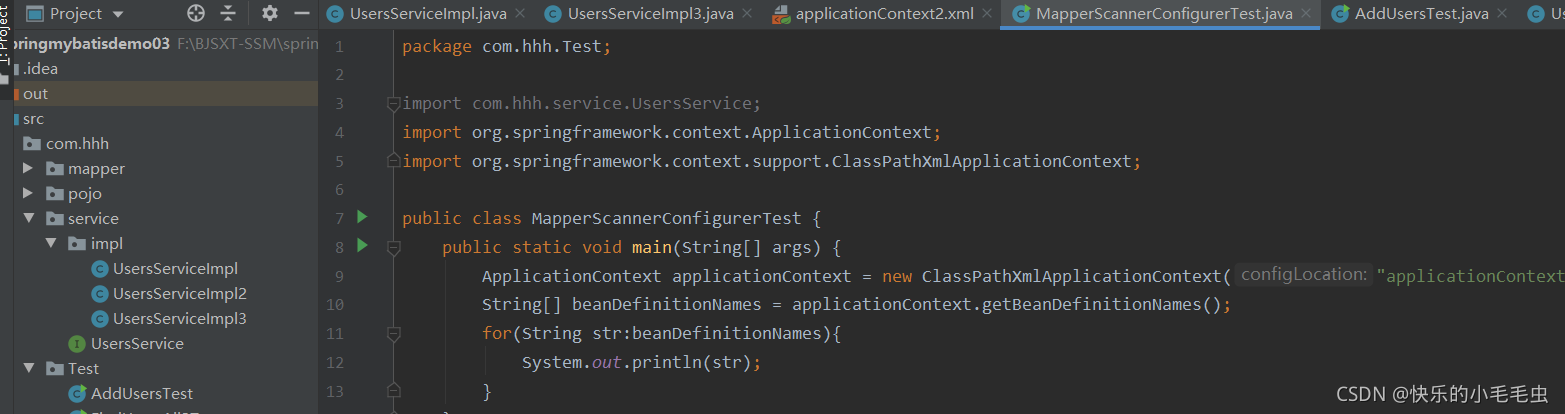
?
?
?
?
|